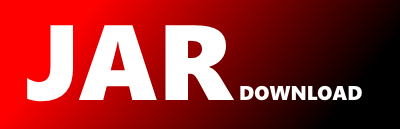
software.amazon.awssdk.services.ecs.model.CreateTaskSetRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateTaskSetRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField SERVICE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("service")
.getter(getter(CreateTaskSetRequest::service)).setter(setter(Builder::service))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("service").build()).build();
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(CreateTaskSetRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField EXTERNAL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("externalId").getter(getter(CreateTaskSetRequest::externalId)).setter(setter(Builder::externalId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("externalId").build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinition").getter(getter(CreateTaskSetRequest::taskDefinition))
.setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkConfiguration")
.getter(getter(CreateTaskSetRequest::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField> LOAD_BALANCERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("loadBalancers")
.getter(getter(CreateTaskSetRequest::loadBalancers))
.setter(setter(Builder::loadBalancers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("loadBalancers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LoadBalancer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SERVICE_REGISTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("serviceRegistries")
.getter(getter(CreateTaskSetRequest::serviceRegistries))
.setter(setter(Builder::serviceRegistries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRegistries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceRegistry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("launchType").getter(getter(CreateTaskSetRequest::launchTypeAsString))
.setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final SdkField> CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviderStrategy")
.getter(getter(CreateTaskSetRequest::capacityProviderStrategy))
.setter(setter(Builder::capacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformVersion").getter(getter(CreateTaskSetRequest::platformVersion))
.setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField SCALE_FIELD = SdkField. builder(MarshallingType.SDK_POJO).memberName("scale")
.getter(getter(CreateTaskSetRequest::scale)).setter(setter(Builder::scale)).constructor(Scale::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("scale").build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clientToken").getter(getter(CreateTaskSetRequest::clientToken)).setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientToken").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(CreateTaskSetRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SERVICE_FIELD, CLUSTER_FIELD,
EXTERNAL_ID_FIELD, TASK_DEFINITION_FIELD, NETWORK_CONFIGURATION_FIELD, LOAD_BALANCERS_FIELD,
SERVICE_REGISTRIES_FIELD, LAUNCH_TYPE_FIELD, CAPACITY_PROVIDER_STRATEGY_FIELD, PLATFORM_VERSION_FIELD, SCALE_FIELD,
CLIENT_TOKEN_FIELD, TAGS_FIELD));
private final String service;
private final String cluster;
private final String externalId;
private final String taskDefinition;
private final NetworkConfiguration networkConfiguration;
private final List loadBalancers;
private final List serviceRegistries;
private final String launchType;
private final List capacityProviderStrategy;
private final String platformVersion;
private final Scale scale;
private final String clientToken;
private final List tags;
private CreateTaskSetRequest(BuilderImpl builder) {
super(builder);
this.service = builder.service;
this.cluster = builder.cluster;
this.externalId = builder.externalId;
this.taskDefinition = builder.taskDefinition;
this.networkConfiguration = builder.networkConfiguration;
this.loadBalancers = builder.loadBalancers;
this.serviceRegistries = builder.serviceRegistries;
this.launchType = builder.launchType;
this.capacityProviderStrategy = builder.capacityProviderStrategy;
this.platformVersion = builder.platformVersion;
this.scale = builder.scale;
this.clientToken = builder.clientToken;
this.tags = builder.tags;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the service to create the task set in.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the service to create the task set in.
*/
public final String service() {
return service;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster that hosts the service to create the task set
* in.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster that hosts the service to create the
* task set in.
*/
public final String cluster() {
return cluster;
}
/**
*
* An optional non-unique tag that identifies this task set in external systems. If the task set is associated with
* a service discovery registry, the tasks in this task set will have the ECS_TASK_SET_EXTERNAL_ID
* Cloud Map attribute set to the provided value.
*
*
* @return An optional non-unique tag that identifies this task set in external systems. If the task set is
* associated with a service discovery registry, the tasks in this task set will have the
* ECS_TASK_SET_EXTERNAL_ID
Cloud Map attribute set to the provided value.
*/
public final String externalId() {
return externalId;
}
/**
*
* The task definition for the tasks in the task set to use.
*
*
* @return The task definition for the tasks in the task set to use.
*/
public final String taskDefinition() {
return taskDefinition;
}
/**
*
* An object representing the network configuration for a task set.
*
*
* @return An object representing the network configuration for a task set.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the LoadBalancers property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLoadBalancers() {
return loadBalancers != null && !(loadBalancers instanceof SdkAutoConstructList);
}
/**
*
* A load balancer object representing the load balancer to use with the task set. The supported load balancer types
* are either an Application Load Balancer or a Network Load Balancer.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLoadBalancers} method.
*
*
* @return A load balancer object representing the load balancer to use with the task set. The supported load
* balancer types are either an Application Load Balancer or a Network Load Balancer.
*/
public final List loadBalancers() {
return loadBalancers;
}
/**
* For responses, this returns true if the service returned a value for the ServiceRegistries property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasServiceRegistries() {
return serviceRegistries != null && !(serviceRegistries instanceof SdkAutoConstructList);
}
/**
*
* The details of the service discovery registries to assign to this task set. For more information, see Service Discovery.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasServiceRegistries} method.
*
*
* @return The details of the service discovery registries to assign to this task set. For more information, see Service
* Discovery.
*/
public final List serviceRegistries() {
return serviceRegistries;
}
/**
*
* The launch type that new tasks in the task set will use. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type that new tasks in the task set will use. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be
* omitted.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The launch type that new tasks in the task set will use. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type that new tasks in the task set will use. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be
* omitted.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
/**
* For responses, this returns true if the service returned a value for the CapacityProviderStrategy property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCapacityProviderStrategy() {
return capacityProviderStrategy != null && !(capacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The capacity provider strategy to use for the task set.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the base
and
* weight
to assign to them. A capacity provider must be associated with the cluster to be used in a
* capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider
* with a cluster. Only capacity providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be created.
* New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
capacity
* providers. The Fargate capacity providers are available to all accounts and only need to be associated with a
* cluster to be used.
*
*
* The PutClusterCapacityProviders API operation is used to update the list of available capacity providers
* for a cluster after the cluster is created.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapacityProviderStrategy} method.
*
*
* @return The capacity provider strategy to use for the task set.
*
* A capacity provider strategy consists of one or more capacity providers along with the base
* and weight
to assign to them. A capacity provider must be associated with the cluster to be
* used in a capacity provider strategy. The PutClusterCapacityProviders API is used to associate a
* capacity provider with a cluster. Only capacity providers with an ACTIVE
or
* UPDATING
status can be used.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be
* created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
* capacity providers. The Fargate capacity providers are available to all accounts and only need to be
* associated with a cluster to be used.
*
*
* The PutClusterCapacityProviders API operation is used to update the list of available capacity
* providers for a cluster after the cluster is created.
*/
public final List capacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* The platform version that the tasks in the task set should use. A platform version is specified only for tasks
* using the Fargate launch type. If one isn't specified, the LATEST
platform version is used by
* default.
*
*
* @return The platform version that the tasks in the task set should use. A platform version is specified only for
* tasks using the Fargate launch type. If one isn't specified, the LATEST
platform version is
* used by default.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*
*
* @return A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*/
public final Scale scale() {
return scale;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Up to 32 ASCII
* characters are allowed.
*
*
* @return Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. Up to 32
* ASCII characters are allowed.
*/
public final String clientToken() {
return clientToken;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the task set to help you categorize and organize them. Each tag consists of a key
* and an optional value, both of which you define. When a service is deleted, the tags are deleted as well.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The metadata that you apply to the task set to help you categorize and organize them. Each tag consists
* of a key and an optional value, both of which you define. When a service is deleted, the tags are deleted
* as well.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete
* tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource
* limit.
*
*
*/
public final List tags() {
return tags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(service());
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(externalId());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasLoadBalancers() ? loadBalancers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasServiceRegistries() ? serviceRegistries() : null);
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviderStrategy() ? capacityProviderStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(scale());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateTaskSetRequest)) {
return false;
}
CreateTaskSetRequest other = (CreateTaskSetRequest) obj;
return Objects.equals(service(), other.service()) && Objects.equals(cluster(), other.cluster())
&& Objects.equals(externalId(), other.externalId()) && Objects.equals(taskDefinition(), other.taskDefinition())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& hasLoadBalancers() == other.hasLoadBalancers() && Objects.equals(loadBalancers(), other.loadBalancers())
&& hasServiceRegistries() == other.hasServiceRegistries()
&& Objects.equals(serviceRegistries(), other.serviceRegistries())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString())
&& hasCapacityProviderStrategy() == other.hasCapacityProviderStrategy()
&& Objects.equals(capacityProviderStrategy(), other.capacityProviderStrategy())
&& Objects.equals(platformVersion(), other.platformVersion()) && Objects.equals(scale(), other.scale())
&& Objects.equals(clientToken(), other.clientToken()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateTaskSetRequest").add("Service", service()).add("Cluster", cluster())
.add("ExternalId", externalId()).add("TaskDefinition", taskDefinition())
.add("NetworkConfiguration", networkConfiguration())
.add("LoadBalancers", hasLoadBalancers() ? loadBalancers() : null)
.add("ServiceRegistries", hasServiceRegistries() ? serviceRegistries() : null)
.add("LaunchType", launchTypeAsString())
.add("CapacityProviderStrategy", hasCapacityProviderStrategy() ? capacityProviderStrategy() : null)
.add("PlatformVersion", platformVersion()).add("Scale", scale()).add("ClientToken", clientToken())
.add("Tags", hasTags() ? tags() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "service":
return Optional.ofNullable(clazz.cast(service()));
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "externalId":
return Optional.ofNullable(clazz.cast(externalId()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "loadBalancers":
return Optional.ofNullable(clazz.cast(loadBalancers()));
case "serviceRegistries":
return Optional.ofNullable(clazz.cast(serviceRegistries()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "capacityProviderStrategy":
return Optional.ofNullable(clazz.cast(capacityProviderStrategy()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "scale":
return Optional.ofNullable(clazz.cast(scale()));
case "clientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function