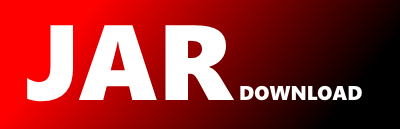
software.amazon.awssdk.services.ecs.model.DeploymentConfiguration Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Optional deployment parameters that control how many tasks run during a deployment and the ordering of stopping and
* starting tasks.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeploymentConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DEPLOYMENT_CIRCUIT_BREAKER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deploymentCircuitBreaker")
.getter(getter(DeploymentConfiguration::deploymentCircuitBreaker)).setter(setter(Builder::deploymentCircuitBreaker))
.constructor(DeploymentCircuitBreaker::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentCircuitBreaker").build())
.build();
private static final SdkField MAXIMUM_PERCENT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("maximumPercent").getter(getter(DeploymentConfiguration::maximumPercent))
.setter(setter(Builder::maximumPercent))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maximumPercent").build()).build();
private static final SdkField MINIMUM_HEALTHY_PERCENT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("minimumHealthyPercent").getter(getter(DeploymentConfiguration::minimumHealthyPercent))
.setter(setter(Builder::minimumHealthyPercent))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("minimumHealthyPercent").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
DEPLOYMENT_CIRCUIT_BREAKER_FIELD, MAXIMUM_PERCENT_FIELD, MINIMUM_HEALTHY_PERCENT_FIELD));
private static final long serialVersionUID = 1L;
private final DeploymentCircuitBreaker deploymentCircuitBreaker;
private final Integer maximumPercent;
private final Integer minimumHealthyPercent;
private DeploymentConfiguration(BuilderImpl builder) {
this.deploymentCircuitBreaker = builder.deploymentCircuitBreaker;
this.maximumPercent = builder.maximumPercent;
this.minimumHealthyPercent = builder.minimumHealthyPercent;
}
/**
*
*
* The deployment circuit breaker can only be used for services using the rolling update (ECS
)
* deployment type.
*
*
*
* The deployment circuit breaker determines whether a service deployment will fail if the service can't
* reach a steady state. If deployment circuit breaker is enabled, a service deployment will transition to a failed
* state and stop launching new tasks. If rollback is enabled, when a service deployment fails, the service is
* rolled back to the last deployment that completed successfully.
*
*
* @return
* The deployment circuit breaker can only be used for services using the rolling update (ECS
)
* deployment type.
*
*
*
* The deployment circuit breaker determines whether a service deployment will fail if the service
* can't reach a steady state. If deployment circuit breaker is enabled, a service deployment will
* transition to a failed state and stop launching new tasks. If rollback is enabled, when a service
* deployment fails, the service is rolled back to the last deployment that completed successfully.
*/
public final DeploymentCircuitBreaker deploymentCircuitBreaker() {
return deploymentCircuitBreaker;
}
/**
*
* If a service is using the rolling update (ECS
) deployment type, the maximum percent parameter
* represents an upper limit on the number of tasks in a service that are allowed in the RUNNING
or
* PENDING
state during a deployment, as a percentage of the desired number of tasks (rounded down to
* the nearest integer), and while any container instances are in the DRAINING
state if the service
* contains tasks using the EC2 launch type. This parameter enables you to define the deployment batch size. For
* example, if your service has a desired number of four tasks and a maximum percent value of 200%, the scheduler
* may start four new tasks before stopping the four older tasks (provided that the cluster resources required to do
* this are available). The default value for maximum percent is 200%.
*
*
* If a service is using the blue/green (CODE_DEPLOY
) or EXTERNAL
deployment types and
* tasks that use the EC2 launch type, the maximum percent value is set to the default value and is used to
* define the upper limit on the number of the tasks in the service that remain in the RUNNING
state
* while the container instances are in the DRAINING
state. If the tasks in the service use the Fargate
* launch type, the maximum percent value is not used, although it is returned when describing your service.
*
*
* @return If a service is using the rolling update (ECS
) deployment type, the maximum percent
* parameter represents an upper limit on the number of tasks in a service that are allowed in the
* RUNNING
or PENDING
state during a deployment, as a percentage of the desired
* number of tasks (rounded down to the nearest integer), and while any container instances are in the
* DRAINING
state if the service contains tasks using the EC2 launch type. This parameter
* enables you to define the deployment batch size. For example, if your service has a desired number of
* four tasks and a maximum percent value of 200%, the scheduler may start four new tasks before stopping
* the four older tasks (provided that the cluster resources required to do this are available). The default
* value for maximum percent is 200%.
*
* If a service is using the blue/green (CODE_DEPLOY
) or EXTERNAL
deployment types
* and tasks that use the EC2 launch type, the maximum percent value is set to the default value and
* is used to define the upper limit on the number of the tasks in the service that remain in the
* RUNNING
state while the container instances are in the DRAINING
state. If the
* tasks in the service use the Fargate launch type, the maximum percent value is not used, although it is
* returned when describing your service.
*/
public final Integer maximumPercent() {
return maximumPercent;
}
/**
*
* If a service is using the rolling update (ECS
) deployment type, the minimum healthy percent
* represents a lower limit on the number of tasks in a service that must remain in the RUNNING
state
* during a deployment, as a percentage of the desired number of tasks (rounded up to the nearest integer), and
* while any container instances are in the DRAINING
state if the service contains tasks using the EC2
* launch type. This parameter enables you to deploy without using additional cluster capacity. For example, if your
* service has a desired number of four tasks and a minimum healthy percent of 50%, the scheduler may stop two
* existing tasks to free up cluster capacity before starting two new tasks. Tasks for services that do not
* use a load balancer are considered healthy if they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the RUNNING
state and they are
* reported as healthy by the load balancer. The default value for minimum healthy percent is 100%.
*
*
* If a service is using the blue/green (CODE_DEPLOY
) or EXTERNAL
deployment types and
* tasks that use the EC2 launch type, the minimum healthy percent value is set to the default value and is
* used to define the lower limit on the number of the tasks in the service that remain in the RUNNING
* state while the container instances are in the DRAINING
state. If the tasks in the service use the
* Fargate launch type, the minimum healthy percent value is not used, although it is returned when describing your
* service.
*
*
* @return If a service is using the rolling update (ECS
) deployment type, the minimum healthy
* percent represents a lower limit on the number of tasks in a service that must remain in the
* RUNNING
state during a deployment, as a percentage of the desired number of tasks (rounded
* up to the nearest integer), and while any container instances are in the DRAINING
state if
* the service contains tasks using the EC2 launch type. This parameter enables you to deploy without using
* additional cluster capacity. For example, if your service has a desired number of four tasks and a
* minimum healthy percent of 50%, the scheduler may stop two existing tasks to free up cluster capacity
* before starting two new tasks. Tasks for services that do not use a load balancer are considered
* healthy if they are in the RUNNING
state; tasks for services that do use a load
* balancer are considered healthy if they are in the RUNNING
state and they are reported as
* healthy by the load balancer. The default value for minimum healthy percent is 100%.
*
* If a service is using the blue/green (CODE_DEPLOY
) or EXTERNAL
deployment types
* and tasks that use the EC2 launch type, the minimum healthy percent value is set to the default
* value and is used to define the lower limit on the number of the tasks in the service that remain in the
* RUNNING
state while the container instances are in the DRAINING
state. If the
* tasks in the service use the Fargate launch type, the minimum healthy percent value is not used, although
* it is returned when describing your service.
*/
public final Integer minimumHealthyPercent() {
return minimumHealthyPercent;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(deploymentCircuitBreaker());
hashCode = 31 * hashCode + Objects.hashCode(maximumPercent());
hashCode = 31 * hashCode + Objects.hashCode(minimumHealthyPercent());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeploymentConfiguration)) {
return false;
}
DeploymentConfiguration other = (DeploymentConfiguration) obj;
return Objects.equals(deploymentCircuitBreaker(), other.deploymentCircuitBreaker())
&& Objects.equals(maximumPercent(), other.maximumPercent())
&& Objects.equals(minimumHealthyPercent(), other.minimumHealthyPercent());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeploymentConfiguration").add("DeploymentCircuitBreaker", deploymentCircuitBreaker())
.add("MaximumPercent", maximumPercent()).add("MinimumHealthyPercent", minimumHealthyPercent()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "deploymentCircuitBreaker":
return Optional.ofNullable(clazz.cast(deploymentCircuitBreaker()));
case "maximumPercent":
return Optional.ofNullable(clazz.cast(maximumPercent()));
case "minimumHealthyPercent":
return Optional.ofNullable(clazz.cast(minimumHealthyPercent()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If a service is using the blue/green (CODE_DEPLOY
) or EXTERNAL
deployment
* types and tasks that use the EC2 launch type, the maximum percent value is set to the default
* value and is used to define the upper limit on the number of the tasks in the service that remain in
* the RUNNING
state while the container instances are in the DRAINING
state.
* If the tasks in the service use the Fargate launch type, the maximum percent value is not used,
* although it is returned when describing your service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maximumPercent(Integer maximumPercent);
/**
*
* If a service is using the rolling update (ECS
) deployment type, the minimum healthy
* percent represents a lower limit on the number of tasks in a service that must remain in the
* RUNNING
state during a deployment, as a percentage of the desired number of tasks (rounded up to
* the nearest integer), and while any container instances are in the DRAINING
state if the service
* contains tasks using the EC2 launch type. This parameter enables you to deploy without using additional
* cluster capacity. For example, if your service has a desired number of four tasks and a minimum healthy
* percent of 50%, the scheduler may stop two existing tasks to free up cluster capacity before starting two new
* tasks. Tasks for services that do not use a load balancer are considered healthy if they are in the
* RUNNING
state; tasks for services that do use a load balancer are considered healthy if
* they are in the RUNNING
state and they are reported as healthy by the load balancer. The default
* value for minimum healthy percent is 100%.
*
*
* If a service is using the blue/green (CODE_DEPLOY
) or EXTERNAL
deployment types and
* tasks that use the EC2 launch type, the minimum healthy percent value is set to the default value and
* is used to define the lower limit on the number of the tasks in the service that remain in the
* RUNNING
state while the container instances are in the DRAINING
state. If the tasks
* in the service use the Fargate launch type, the minimum healthy percent value is not used, although it is
* returned when describing your service.
*
*
* @param minimumHealthyPercent
* If a service is using the rolling update (ECS
) deployment type, the minimum healthy
* percent represents a lower limit on the number of tasks in a service that must remain in the
* RUNNING
state during a deployment, as a percentage of the desired number of tasks
* (rounded up to the nearest integer), and while any container instances are in the
* DRAINING
state if the service contains tasks using the EC2 launch type. This parameter
* enables you to deploy without using additional cluster capacity. For example, if your service has a
* desired number of four tasks and a minimum healthy percent of 50%, the scheduler may stop two existing
* tasks to free up cluster capacity before starting two new tasks. Tasks for services that do not
* use a load balancer are considered healthy if they are in the RUNNING
state; tasks for
* services that do use a load balancer are considered healthy if they are in the
* RUNNING
state and they are reported as healthy by the load balancer. The default value
* for minimum healthy percent is 100%.
*
* If a service is using the blue/green (CODE_DEPLOY
) or EXTERNAL
deployment
* types and tasks that use the EC2 launch type, the minimum healthy percent value is set to the
* default value and is used to define the lower limit on the number of the tasks in the service that
* remain in the RUNNING
state while the container instances are in the
* DRAINING
state. If the tasks in the service use the Fargate launch type, the minimum
* healthy percent value is not used, although it is returned when describing your service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder minimumHealthyPercent(Integer minimumHealthyPercent);
}
static final class BuilderImpl implements Builder {
private DeploymentCircuitBreaker deploymentCircuitBreaker;
private Integer maximumPercent;
private Integer minimumHealthyPercent;
private BuilderImpl() {
}
private BuilderImpl(DeploymentConfiguration model) {
deploymentCircuitBreaker(model.deploymentCircuitBreaker);
maximumPercent(model.maximumPercent);
minimumHealthyPercent(model.minimumHealthyPercent);
}
public final DeploymentCircuitBreaker.Builder getDeploymentCircuitBreaker() {
return deploymentCircuitBreaker != null ? deploymentCircuitBreaker.toBuilder() : null;
}
public final void setDeploymentCircuitBreaker(DeploymentCircuitBreaker.BuilderImpl deploymentCircuitBreaker) {
this.deploymentCircuitBreaker = deploymentCircuitBreaker != null ? deploymentCircuitBreaker.build() : null;
}
@Override
@Transient
public final Builder deploymentCircuitBreaker(DeploymentCircuitBreaker deploymentCircuitBreaker) {
this.deploymentCircuitBreaker = deploymentCircuitBreaker;
return this;
}
public final Integer getMaximumPercent() {
return maximumPercent;
}
public final void setMaximumPercent(Integer maximumPercent) {
this.maximumPercent = maximumPercent;
}
@Override
@Transient
public final Builder maximumPercent(Integer maximumPercent) {
this.maximumPercent = maximumPercent;
return this;
}
public final Integer getMinimumHealthyPercent() {
return minimumHealthyPercent;
}
public final void setMinimumHealthyPercent(Integer minimumHealthyPercent) {
this.minimumHealthyPercent = minimumHealthyPercent;
}
@Override
@Transient
public final Builder minimumHealthyPercent(Integer minimumHealthyPercent) {
this.minimumHealthyPercent = minimumHealthyPercent;
return this;
}
@Override
public DeploymentConfiguration build() {
return new DeploymentConfiguration(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}