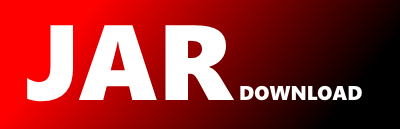
software.amazon.awssdk.services.ecs.model.ExecuteCommandConfiguration Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of the execute command configuration.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ExecuteCommandConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("kmsKeyId").getter(getter(ExecuteCommandConfiguration::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsKeyId").build()).build();
private static final SdkField LOGGING_FIELD = SdkField. builder(MarshallingType.STRING).memberName("logging")
.getter(getter(ExecuteCommandConfiguration::loggingAsString)).setter(setter(Builder::logging))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logging").build()).build();
private static final SdkField LOG_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("logConfiguration")
.getter(getter(ExecuteCommandConfiguration::logConfiguration)).setter(setter(Builder::logConfiguration))
.constructor(ExecuteCommandLogConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logConfiguration").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(KMS_KEY_ID_FIELD,
LOGGING_FIELD, LOG_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final String kmsKeyId;
private final String logging;
private final ExecuteCommandLogConfiguration logConfiguration;
private ExecuteCommandConfiguration(BuilderImpl builder) {
this.kmsKeyId = builder.kmsKeyId;
this.logging = builder.logging;
this.logConfiguration = builder.logConfiguration;
}
/**
*
* Specify an Key Management Service key ID to encrypt the data between the local client and the container.
*
*
* @return Specify an Key Management Service key ID to encrypt the data between the local client and the container.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The log setting to use for redirecting logs for your execute command results. The following log settings are
* available.
*
*
* -
*
* NONE
: The execute command session is not logged.
*
*
* -
*
* DEFAULT
: The awslogs
configuration in the task definition is used. If no logging
* parameter is specified, it defaults to this value. If no awslogs
log driver is configured in the
* task definition, the output won't be logged.
*
*
* -
*
* OVERRIDE
: Specify the logging details as a part of logConfiguration
. If the
* OVERRIDE
logging option is specified, the logConfiguration
is required.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #logging} will
* return {@link ExecuteCommandLogging#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #loggingAsString}.
*
*
* @return The log setting to use for redirecting logs for your execute command results. The following log settings
* are available.
*
* -
*
* NONE
: The execute command session is not logged.
*
*
* -
*
* DEFAULT
: The awslogs
configuration in the task definition is used. If no
* logging parameter is specified, it defaults to this value. If no awslogs
log driver is
* configured in the task definition, the output won't be logged.
*
*
* -
*
* OVERRIDE
: Specify the logging details as a part of logConfiguration
. If the
* OVERRIDE
logging option is specified, the logConfiguration
is required.
*
*
* @see ExecuteCommandLogging
*/
public final ExecuteCommandLogging logging() {
return ExecuteCommandLogging.fromValue(logging);
}
/**
*
* The log setting to use for redirecting logs for your execute command results. The following log settings are
* available.
*
*
* -
*
* NONE
: The execute command session is not logged.
*
*
* -
*
* DEFAULT
: The awslogs
configuration in the task definition is used. If no logging
* parameter is specified, it defaults to this value. If no awslogs
log driver is configured in the
* task definition, the output won't be logged.
*
*
* -
*
* OVERRIDE
: Specify the logging details as a part of logConfiguration
. If the
* OVERRIDE
logging option is specified, the logConfiguration
is required.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #logging} will
* return {@link ExecuteCommandLogging#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #loggingAsString}.
*
*
* @return The log setting to use for redirecting logs for your execute command results. The following log settings
* are available.
*
* -
*
* NONE
: The execute command session is not logged.
*
*
* -
*
* DEFAULT
: The awslogs
configuration in the task definition is used. If no
* logging parameter is specified, it defaults to this value. If no awslogs
log driver is
* configured in the task definition, the output won't be logged.
*
*
* -
*
* OVERRIDE
: Specify the logging details as a part of logConfiguration
. If the
* OVERRIDE
logging option is specified, the logConfiguration
is required.
*
*
* @see ExecuteCommandLogging
*/
public final String loggingAsString() {
return logging;
}
/**
*
* The log configuration for the results of the execute command actions. The logs can be sent to CloudWatch Logs or
* an Amazon S3 bucket. When logging=OVERRIDE
is specified, a logConfiguration
must be
* provided.
*
*
* @return The log configuration for the results of the execute command actions. The logs can be sent to CloudWatch
* Logs or an Amazon S3 bucket. When logging=OVERRIDE
is specified, a
* logConfiguration
must be provided.
*/
public final ExecuteCommandLogConfiguration logConfiguration() {
return logConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(loggingAsString());
hashCode = 31 * hashCode + Objects.hashCode(logConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ExecuteCommandConfiguration)) {
return false;
}
ExecuteCommandConfiguration other = (ExecuteCommandConfiguration) obj;
return Objects.equals(kmsKeyId(), other.kmsKeyId()) && Objects.equals(loggingAsString(), other.loggingAsString())
&& Objects.equals(logConfiguration(), other.logConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ExecuteCommandConfiguration").add("KmsKeyId", kmsKeyId()).add("Logging", loggingAsString())
.add("LogConfiguration", logConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "kmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "logging":
return Optional.ofNullable(clazz.cast(loggingAsString()));
case "logConfiguration":
return Optional.ofNullable(clazz.cast(logConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function