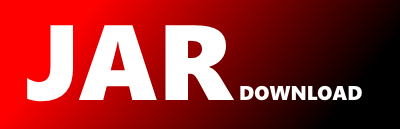
software.amazon.awssdk.services.ecs.EcsAsyncClient Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.ecs.model.CreateCapacityProviderRequest;
import software.amazon.awssdk.services.ecs.model.CreateCapacityProviderResponse;
import software.amazon.awssdk.services.ecs.model.CreateClusterRequest;
import software.amazon.awssdk.services.ecs.model.CreateClusterResponse;
import software.amazon.awssdk.services.ecs.model.CreateServiceRequest;
import software.amazon.awssdk.services.ecs.model.CreateServiceResponse;
import software.amazon.awssdk.services.ecs.model.CreateTaskSetRequest;
import software.amazon.awssdk.services.ecs.model.CreateTaskSetResponse;
import software.amazon.awssdk.services.ecs.model.DeleteAccountSettingRequest;
import software.amazon.awssdk.services.ecs.model.DeleteAccountSettingResponse;
import software.amazon.awssdk.services.ecs.model.DeleteAttributesRequest;
import software.amazon.awssdk.services.ecs.model.DeleteAttributesResponse;
import software.amazon.awssdk.services.ecs.model.DeleteCapacityProviderRequest;
import software.amazon.awssdk.services.ecs.model.DeleteCapacityProviderResponse;
import software.amazon.awssdk.services.ecs.model.DeleteClusterRequest;
import software.amazon.awssdk.services.ecs.model.DeleteClusterResponse;
import software.amazon.awssdk.services.ecs.model.DeleteServiceRequest;
import software.amazon.awssdk.services.ecs.model.DeleteServiceResponse;
import software.amazon.awssdk.services.ecs.model.DeleteTaskSetRequest;
import software.amazon.awssdk.services.ecs.model.DeleteTaskSetResponse;
import software.amazon.awssdk.services.ecs.model.DeregisterContainerInstanceRequest;
import software.amazon.awssdk.services.ecs.model.DeregisterContainerInstanceResponse;
import software.amazon.awssdk.services.ecs.model.DeregisterTaskDefinitionRequest;
import software.amazon.awssdk.services.ecs.model.DeregisterTaskDefinitionResponse;
import software.amazon.awssdk.services.ecs.model.DescribeCapacityProvidersRequest;
import software.amazon.awssdk.services.ecs.model.DescribeCapacityProvidersResponse;
import software.amazon.awssdk.services.ecs.model.DescribeClustersRequest;
import software.amazon.awssdk.services.ecs.model.DescribeClustersResponse;
import software.amazon.awssdk.services.ecs.model.DescribeContainerInstancesRequest;
import software.amazon.awssdk.services.ecs.model.DescribeContainerInstancesResponse;
import software.amazon.awssdk.services.ecs.model.DescribeServicesRequest;
import software.amazon.awssdk.services.ecs.model.DescribeServicesResponse;
import software.amazon.awssdk.services.ecs.model.DescribeTaskDefinitionRequest;
import software.amazon.awssdk.services.ecs.model.DescribeTaskDefinitionResponse;
import software.amazon.awssdk.services.ecs.model.DescribeTaskSetsRequest;
import software.amazon.awssdk.services.ecs.model.DescribeTaskSetsResponse;
import software.amazon.awssdk.services.ecs.model.DescribeTasksRequest;
import software.amazon.awssdk.services.ecs.model.DescribeTasksResponse;
import software.amazon.awssdk.services.ecs.model.DiscoverPollEndpointRequest;
import software.amazon.awssdk.services.ecs.model.DiscoverPollEndpointResponse;
import software.amazon.awssdk.services.ecs.model.ExecuteCommandRequest;
import software.amazon.awssdk.services.ecs.model.ExecuteCommandResponse;
import software.amazon.awssdk.services.ecs.model.ListAccountSettingsRequest;
import software.amazon.awssdk.services.ecs.model.ListAccountSettingsResponse;
import software.amazon.awssdk.services.ecs.model.ListAttributesRequest;
import software.amazon.awssdk.services.ecs.model.ListAttributesResponse;
import software.amazon.awssdk.services.ecs.model.ListClustersRequest;
import software.amazon.awssdk.services.ecs.model.ListClustersResponse;
import software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest;
import software.amazon.awssdk.services.ecs.model.ListContainerInstancesResponse;
import software.amazon.awssdk.services.ecs.model.ListServicesRequest;
import software.amazon.awssdk.services.ecs.model.ListServicesResponse;
import software.amazon.awssdk.services.ecs.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ecs.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesRequest;
import software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesResponse;
import software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsRequest;
import software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsResponse;
import software.amazon.awssdk.services.ecs.model.ListTasksRequest;
import software.amazon.awssdk.services.ecs.model.ListTasksResponse;
import software.amazon.awssdk.services.ecs.model.PutAccountSettingDefaultRequest;
import software.amazon.awssdk.services.ecs.model.PutAccountSettingDefaultResponse;
import software.amazon.awssdk.services.ecs.model.PutAccountSettingRequest;
import software.amazon.awssdk.services.ecs.model.PutAccountSettingResponse;
import software.amazon.awssdk.services.ecs.model.PutAttributesRequest;
import software.amazon.awssdk.services.ecs.model.PutAttributesResponse;
import software.amazon.awssdk.services.ecs.model.PutClusterCapacityProvidersRequest;
import software.amazon.awssdk.services.ecs.model.PutClusterCapacityProvidersResponse;
import software.amazon.awssdk.services.ecs.model.RegisterContainerInstanceRequest;
import software.amazon.awssdk.services.ecs.model.RegisterContainerInstanceResponse;
import software.amazon.awssdk.services.ecs.model.RegisterTaskDefinitionRequest;
import software.amazon.awssdk.services.ecs.model.RegisterTaskDefinitionResponse;
import software.amazon.awssdk.services.ecs.model.RunTaskRequest;
import software.amazon.awssdk.services.ecs.model.RunTaskResponse;
import software.amazon.awssdk.services.ecs.model.StartTaskRequest;
import software.amazon.awssdk.services.ecs.model.StartTaskResponse;
import software.amazon.awssdk.services.ecs.model.StopTaskRequest;
import software.amazon.awssdk.services.ecs.model.StopTaskResponse;
import software.amazon.awssdk.services.ecs.model.SubmitAttachmentStateChangesRequest;
import software.amazon.awssdk.services.ecs.model.SubmitAttachmentStateChangesResponse;
import software.amazon.awssdk.services.ecs.model.SubmitContainerStateChangeRequest;
import software.amazon.awssdk.services.ecs.model.SubmitContainerStateChangeResponse;
import software.amazon.awssdk.services.ecs.model.SubmitTaskStateChangeRequest;
import software.amazon.awssdk.services.ecs.model.SubmitTaskStateChangeResponse;
import software.amazon.awssdk.services.ecs.model.TagResourceRequest;
import software.amazon.awssdk.services.ecs.model.TagResourceResponse;
import software.amazon.awssdk.services.ecs.model.UntagResourceRequest;
import software.amazon.awssdk.services.ecs.model.UntagResourceResponse;
import software.amazon.awssdk.services.ecs.model.UpdateCapacityProviderRequest;
import software.amazon.awssdk.services.ecs.model.UpdateCapacityProviderResponse;
import software.amazon.awssdk.services.ecs.model.UpdateClusterRequest;
import software.amazon.awssdk.services.ecs.model.UpdateClusterResponse;
import software.amazon.awssdk.services.ecs.model.UpdateClusterSettingsRequest;
import software.amazon.awssdk.services.ecs.model.UpdateClusterSettingsResponse;
import software.amazon.awssdk.services.ecs.model.UpdateContainerAgentRequest;
import software.amazon.awssdk.services.ecs.model.UpdateContainerAgentResponse;
import software.amazon.awssdk.services.ecs.model.UpdateContainerInstancesStateRequest;
import software.amazon.awssdk.services.ecs.model.UpdateContainerInstancesStateResponse;
import software.amazon.awssdk.services.ecs.model.UpdateServicePrimaryTaskSetRequest;
import software.amazon.awssdk.services.ecs.model.UpdateServicePrimaryTaskSetResponse;
import software.amazon.awssdk.services.ecs.model.UpdateServiceRequest;
import software.amazon.awssdk.services.ecs.model.UpdateServiceResponse;
import software.amazon.awssdk.services.ecs.model.UpdateTaskSetRequest;
import software.amazon.awssdk.services.ecs.model.UpdateTaskSetResponse;
import software.amazon.awssdk.services.ecs.paginators.ListAccountSettingsPublisher;
import software.amazon.awssdk.services.ecs.paginators.ListAttributesPublisher;
import software.amazon.awssdk.services.ecs.paginators.ListClustersPublisher;
import software.amazon.awssdk.services.ecs.paginators.ListContainerInstancesPublisher;
import software.amazon.awssdk.services.ecs.paginators.ListServicesPublisher;
import software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionFamiliesPublisher;
import software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionsPublisher;
import software.amazon.awssdk.services.ecs.paginators.ListTasksPublisher;
import software.amazon.awssdk.services.ecs.waiters.EcsAsyncWaiter;
/**
* Service client for accessing Amazon ECS asynchronously. This can be created using the static {@link #builder()}
* method.
*
* Amazon Elastic Container Service
*
* Amazon Elastic Container Service (Amazon ECS) is a highly scalable, fast, container management service. It makes it
* easy to run, stop, and manage Docker containers on a cluster. You can host your cluster on a serverless
* infrastructure that's managed by Amazon ECS by launching your services or tasks on Fargate. For more control, you can
* host your tasks on a cluster of Amazon Elastic Compute Cloud (Amazon EC2) instances that you manage.
*
*
* Amazon ECS makes it easy to launch and stop container-based applications with simple API calls. This makes it easy to
* get the state of your cluster from a centralized service, and gives you access to many familiar Amazon EC2 features.
*
*
* You can use Amazon ECS to schedule the placement of containers across your cluster based on your resource needs,
* isolation policies, and availability requirements. With Amazon ECS, you don't need to operate your own cluster
* management and configuration management systems. You also don't need to worry about scaling your management
* infrastructure.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface EcsAsyncClient extends SdkClient {
String SERVICE_NAME = "ecs";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "ecs";
/**
* Create a {@link EcsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static EcsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link EcsAsyncClient}.
*/
static EcsAsyncClientBuilder builder() {
return new DefaultEcsAsyncClientBuilder();
}
/**
*
* Creates a new capacity provider. Capacity providers are associated with an Amazon ECS cluster and are used in
* capacity provider strategies to facilitate cluster auto scaling.
*
*
* Only capacity providers that use an Auto Scaling group can be created. Amazon ECS tasks on Fargate use the
* FARGATE
and FARGATE_SPOT
capacity providers. These providers are available to all
* accounts in the Amazon Web Services Regions that Fargate supports.
*
*
* @param createCapacityProviderRequest
* @return A Java Future containing the result of the CreateCapacityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - LimitExceededException The limit for the resource was exceeded.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateCapacityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture createCapacityProvider(
CreateCapacityProviderRequest createCapacityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new capacity provider. Capacity providers are associated with an Amazon ECS cluster and are used in
* capacity provider strategies to facilitate cluster auto scaling.
*
*
* Only capacity providers that use an Auto Scaling group can be created. Amazon ECS tasks on Fargate use the
* FARGATE
and FARGATE_SPOT
capacity providers. These providers are available to all
* accounts in the Amazon Web Services Regions that Fargate supports.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCapacityProviderRequest.Builder} avoiding the
* need to create one manually via {@link CreateCapacityProviderRequest#builder()}
*
*
* @param createCapacityProviderRequest
* A {@link Consumer} that will call methods on {@link CreateCapacityProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateCapacityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - LimitExceededException The limit for the resource was exceeded.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateCapacityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture createCapacityProvider(
Consumer createCapacityProviderRequest) {
return createCapacityProvider(CreateCapacityProviderRequest.builder().applyMutation(createCapacityProviderRequest)
.build());
}
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a default
cluster when you
* launch your first container instance. However, you can create your own cluster with a unique name with the
* CreateCluster
action.
*
*
*
* When you call the CreateCluster API operation, Amazon ECS attempts to create the Amazon ECS service-linked
* role for your account. This is so that it can manage required resources in other Amazon Web Services services on
* your behalf. However, if the IAM user that makes the call doesn't have permissions to create the service-linked
* role, it isn't created. For more information, see Using
* Service-Linked Roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @param createClusterRequest
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createCluster(CreateClusterRequest createClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a default
cluster when you
* launch your first container instance. However, you can create your own cluster with a unique name with the
* CreateCluster
action.
*
*
*
* When you call the CreateCluster API operation, Amazon ECS attempts to create the Amazon ECS service-linked
* role for your account. This is so that it can manage required resources in other Amazon Web Services services on
* your behalf. However, if the IAM user that makes the call doesn't have permissions to create the service-linked
* role, it isn't created. For more information, see Using
* Service-Linked Roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateClusterRequest.Builder} avoiding the need to
* create one manually via {@link CreateClusterRequest#builder()}
*
*
* @param createClusterRequest
* A {@link Consumer} that will call methods on {@link CreateClusterRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createCluster(Consumer createClusterRequest) {
return createCluster(CreateClusterRequest.builder().applyMutation(createClusterRequest).build());
}
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a default
cluster when you
* launch your first container instance. However, you can create your own cluster with a unique name with the
* CreateCluster
action.
*
*
*
* When you call the CreateCluster API operation, Amazon ECS attempts to create the Amazon ECS service-linked
* role for your account. This is so that it can manage required resources in other Amazon Web Services services on
* your behalf. However, if the IAM user that makes the call doesn't have permissions to create the service-linked
* role, it isn't created. For more information, see Using
* Service-Linked Roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateCluster
* @see AWS API
* Documentation
*/
default CompletableFuture createCluster() {
return createCluster(CreateClusterRequest.builder().build());
}
/**
*
* Runs and maintains your desired number of tasks from a specified task definition. If the number of tasks running
* in a service drops below the desiredCount
, Amazon ECS runs another copy of the task in the specified
* cluster. To update an existing service, see the UpdateService action.
*
*
* In addition to maintaining the desired count of tasks in your service, you can optionally run your service behind
* one or more load balancers. The load balancers distribute traffic across the tasks that are associated with the
* service. For more information, see Service Load
* Balancing in the Amazon Elastic Container Service Developer Guide.
*
*
* Tasks for services that don't use a load balancer are considered healthy if they're in the RUNNING
* state. Tasks for services that use a load balancer are considered healthy if they're in the RUNNING
* state and the container instance that they're hosted on is reported as healthy by the load balancer.
*
*
* There are two service scheduler strategies available:
*
*
* -
*
* REPLICA
- The replica scheduling strategy places and maintains your desired number of tasks across
* your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task
* placement strategies and constraints to customize task placement decisions. For more information, see Service Scheduler
* Concepts in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* DAEMON
- The daemon scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that you specify in your cluster. The service scheduler also
* evaluates the task placement constraints for running tasks. It also stops tasks that don't meet the placement
* constraints. When using this strategy, you don't need to specify a desired number of tasks, a task placement
* strategy, or use Service Auto Scaling policies. For more information, see Service Scheduler
* Concepts in the Amazon Elastic Container Service Developer Guide.
*
*
*
*
* You can optionally specify a deployment configuration for your service. The deployment is initiated by changing
* properties. For example, the deployment might be initiated by the task definition or by your desired count of a
* service. This is done with an UpdateService operation. The default value for a replica service for
* minimumHealthyPercent
is 100%. The default value for a daemon service for
* minimumHealthyPercent
is 0%.
*
*
* If a service uses the ECS
deployment controller, the minimum healthy percent represents a lower
* limit on the number of tasks in a service that must remain in the RUNNING
state during a deployment.
* Specifically, it represents it as a percentage of your desired number of tasks (rounded up to the nearest
* integer). This happens when any of your container instances are in the DRAINING
state if the service
* contains tasks using the EC2 launch type. Using this parameter, you can deploy without using additional cluster
* capacity. For example, if you set your service to have desired number of four tasks and a minimum healthy percent
* of 50%, the scheduler might stop two existing tasks to free up cluster capacity before starting two new tasks. If
* they're in the RUNNING
state, tasks for services that don't use a load balancer are considered
* healthy . If they're in the RUNNING
state and reported as healthy by the load balancer, tasks for
* services that do use a load balancer are considered healthy . The default value for minimum healthy
* percent is 100%.
*
*
* If a service uses the ECS
deployment controller, the maximum percent parameter represents an
* upper limit on the number of tasks in a service that are allowed in the RUNNING
or
* PENDING
state during a deployment. Specifically, it represents it as a percentage of the desired
* number of tasks (rounded down to the nearest integer). This happens when any of your container instances are in
* the DRAINING
state if the service contains tasks using the EC2 launch type. Using this parameter,
* you can define the deployment batch size. For example, if your service has a desired number of four tasks and a
* maximum percent value of 200%, the scheduler may start four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available). The default value for maximum percent is
* 200%.
*
*
* If a service uses either the CODE_DEPLOY
or EXTERNAL
deployment controller types and
* tasks that use the EC2 launch type, the minimum healthy percent and maximum percent values are used
* only to define the lower and upper limit on the number of the tasks in the service that remain in the
* RUNNING
state. This is while the container instances are in the DRAINING
state. If the
* tasks in the service use the Fargate launch type, the minimum healthy percent and maximum percent values aren't
* used. This is the case even if they're currently visible when describing your service.
*
*
* When creating a service that uses the EXTERNAL
deployment controller, you can specify only
* parameters that aren't controlled at the task set level. The only required parameter is the service name. You
* control your services using the CreateTaskSet operation. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* When the service scheduler launches new tasks, it determines task placement in your cluster using the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support the task definition of your service. For
* example, they have the required CPU, memory, ports, and container instance attributes.
*
*
* -
*
* By default, the service scheduler attempts to balance tasks across Availability Zones in this manner. This is the
* case even if you can choose a different placement strategy with the placementStrategy
parameter.
*
*
* -
*
* Sort the valid container instances, giving priority to instances that have the fewest number of running tasks for
* this service in their respective Availability Zone. For example, if zone A has one running service task and zones
* B and C each have zero, valid container instances in either zone B or C are considered optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal Availability Zone based on the previous
* steps, favoring container instances with the fewest number of running tasks for this service.
*
*
*
*
*
*
* @param createServiceRequest
* @return A Java Future containing the result of the CreateService operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateService
* @see AWS API
* Documentation
*/
default CompletableFuture createService(CreateServiceRequest createServiceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Runs and maintains your desired number of tasks from a specified task definition. If the number of tasks running
* in a service drops below the desiredCount
, Amazon ECS runs another copy of the task in the specified
* cluster. To update an existing service, see the UpdateService action.
*
*
* In addition to maintaining the desired count of tasks in your service, you can optionally run your service behind
* one or more load balancers. The load balancers distribute traffic across the tasks that are associated with the
* service. For more information, see Service Load
* Balancing in the Amazon Elastic Container Service Developer Guide.
*
*
* Tasks for services that don't use a load balancer are considered healthy if they're in the RUNNING
* state. Tasks for services that use a load balancer are considered healthy if they're in the RUNNING
* state and the container instance that they're hosted on is reported as healthy by the load balancer.
*
*
* There are two service scheduler strategies available:
*
*
* -
*
* REPLICA
- The replica scheduling strategy places and maintains your desired number of tasks across
* your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task
* placement strategies and constraints to customize task placement decisions. For more information, see Service Scheduler
* Concepts in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* DAEMON
- The daemon scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that you specify in your cluster. The service scheduler also
* evaluates the task placement constraints for running tasks. It also stops tasks that don't meet the placement
* constraints. When using this strategy, you don't need to specify a desired number of tasks, a task placement
* strategy, or use Service Auto Scaling policies. For more information, see Service Scheduler
* Concepts in the Amazon Elastic Container Service Developer Guide.
*
*
*
*
* You can optionally specify a deployment configuration for your service. The deployment is initiated by changing
* properties. For example, the deployment might be initiated by the task definition or by your desired count of a
* service. This is done with an UpdateService operation. The default value for a replica service for
* minimumHealthyPercent
is 100%. The default value for a daemon service for
* minimumHealthyPercent
is 0%.
*
*
* If a service uses the ECS
deployment controller, the minimum healthy percent represents a lower
* limit on the number of tasks in a service that must remain in the RUNNING
state during a deployment.
* Specifically, it represents it as a percentage of your desired number of tasks (rounded up to the nearest
* integer). This happens when any of your container instances are in the DRAINING
state if the service
* contains tasks using the EC2 launch type. Using this parameter, you can deploy without using additional cluster
* capacity. For example, if you set your service to have desired number of four tasks and a minimum healthy percent
* of 50%, the scheduler might stop two existing tasks to free up cluster capacity before starting two new tasks. If
* they're in the RUNNING
state, tasks for services that don't use a load balancer are considered
* healthy . If they're in the RUNNING
state and reported as healthy by the load balancer, tasks for
* services that do use a load balancer are considered healthy . The default value for minimum healthy
* percent is 100%.
*
*
* If a service uses the ECS
deployment controller, the maximum percent parameter represents an
* upper limit on the number of tasks in a service that are allowed in the RUNNING
or
* PENDING
state during a deployment. Specifically, it represents it as a percentage of the desired
* number of tasks (rounded down to the nearest integer). This happens when any of your container instances are in
* the DRAINING
state if the service contains tasks using the EC2 launch type. Using this parameter,
* you can define the deployment batch size. For example, if your service has a desired number of four tasks and a
* maximum percent value of 200%, the scheduler may start four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available). The default value for maximum percent is
* 200%.
*
*
* If a service uses either the CODE_DEPLOY
or EXTERNAL
deployment controller types and
* tasks that use the EC2 launch type, the minimum healthy percent and maximum percent values are used
* only to define the lower and upper limit on the number of the tasks in the service that remain in the
* RUNNING
state. This is while the container instances are in the DRAINING
state. If the
* tasks in the service use the Fargate launch type, the minimum healthy percent and maximum percent values aren't
* used. This is the case even if they're currently visible when describing your service.
*
*
* When creating a service that uses the EXTERNAL
deployment controller, you can specify only
* parameters that aren't controlled at the task set level. The only required parameter is the service name. You
* control your services using the CreateTaskSet operation. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* When the service scheduler launches new tasks, it determines task placement in your cluster using the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support the task definition of your service. For
* example, they have the required CPU, memory, ports, and container instance attributes.
*
*
* -
*
* By default, the service scheduler attempts to balance tasks across Availability Zones in this manner. This is the
* case even if you can choose a different placement strategy with the placementStrategy
parameter.
*
*
* -
*
* Sort the valid container instances, giving priority to instances that have the fewest number of running tasks for
* this service in their respective Availability Zone. For example, if zone A has one running service task and zones
* B and C each have zero, valid container instances in either zone B or C are considered optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal Availability Zone based on the previous
* steps, favoring container instances with the fewest number of running tasks for this service.
*
*
*
*
*
*
*
* This is a convenience which creates an instance of the {@link CreateServiceRequest.Builder} avoiding the need to
* create one manually via {@link CreateServiceRequest#builder()}
*
*
* @param createServiceRequest
* A {@link Consumer} that will call methods on {@link CreateServiceRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateService operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateService
* @see AWS API
* Documentation
*/
default CompletableFuture createService(Consumer createServiceRequest) {
return createService(CreateServiceRequest.builder().applyMutation(createServiceRequest).build());
}
/**
*
* Create a task set in the specified cluster and service. This is used when a service uses the
* EXTERNAL
deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param createTaskSetRequest
* @return A Java Future containing the result of the CreateTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateTaskSet
* @see AWS API
* Documentation
*/
default CompletableFuture createTaskSet(CreateTaskSetRequest createTaskSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create a task set in the specified cluster and service. This is used when a service uses the
* EXTERNAL
deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link CreateTaskSetRequest.Builder} avoiding the need to
* create one manually via {@link CreateTaskSetRequest#builder()}
*
*
* @param createTaskSetRequest
* A {@link Consumer} that will call methods on {@link CreateTaskSetRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateTaskSet
* @see AWS API
* Documentation
*/
default CompletableFuture createTaskSet(Consumer createTaskSetRequest) {
return createTaskSet(CreateTaskSetRequest.builder().applyMutation(createTaskSetRequest).build());
}
/**
*
* Disables an account setting for a specified IAM user, IAM role, or the root user for an account.
*
*
* @param deleteAccountSettingRequest
* @return A Java Future containing the result of the DeleteAccountSetting operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteAccountSetting
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAccountSetting(
DeleteAccountSettingRequest deleteAccountSettingRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disables an account setting for a specified IAM user, IAM role, or the root user for an account.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAccountSettingRequest.Builder} avoiding the
* need to create one manually via {@link DeleteAccountSettingRequest#builder()}
*
*
* @param deleteAccountSettingRequest
* A {@link Consumer} that will call methods on {@link DeleteAccountSettingRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteAccountSetting operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteAccountSetting
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAccountSetting(
Consumer deleteAccountSettingRequest) {
return deleteAccountSetting(DeleteAccountSettingRequest.builder().applyMutation(deleteAccountSettingRequest).build());
}
/**
*
* Deletes one or more custom attributes from an Amazon ECS resource.
*
*
* @param deleteAttributesRequest
* @return A Java Future containing the result of the DeleteAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotFoundException The specified target wasn't found. You can view your available container
* instances with ListContainerInstances. Amazon ECS container instances are cluster-specific and
* Region-specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteAttributes
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAttributes(DeleteAttributesRequest deleteAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes one or more custom attributes from an Amazon ECS resource.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAttributesRequest.Builder} avoiding the need
* to create one manually via {@link DeleteAttributesRequest#builder()}
*
*
* @param deleteAttributesRequest
* A {@link Consumer} that will call methods on {@link DeleteAttributesRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotFoundException The specified target wasn't found. You can view your available container
* instances with ListContainerInstances. Amazon ECS container instances are cluster-specific and
* Region-specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteAttributes
* @see AWS API
* Documentation
*/
default CompletableFuture deleteAttributes(
Consumer deleteAttributesRequest) {
return deleteAttributes(DeleteAttributesRequest.builder().applyMutation(deleteAttributesRequest).build());
}
/**
*
* Deletes the specified capacity provider.
*
*
*
* The FARGATE
and FARGATE_SPOT
capacity providers are reserved and can't be deleted. You
* can disassociate them from a cluster using either the PutClusterCapacityProviders API or by deleting the
* cluster.
*
*
*
* Prior to a capacity provider being deleted, the capacity provider must be removed from the capacity provider
* strategy from all services. The UpdateService API can be used to remove a capacity provider from a
* service's capacity provider strategy. When updating a service, the forceNewDeployment
option can be
* used to ensure that any tasks using the Amazon EC2 instance capacity provided by the capacity provider are
* transitioned to use the capacity from the remaining capacity providers. Only capacity providers that aren't
* associated with a cluster can be deleted. To remove a capacity provider from a cluster, you can either use
* PutClusterCapacityProviders or delete the cluster.
*
*
* @param deleteCapacityProviderRequest
* @return A Java Future containing the result of the DeleteCapacityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteCapacityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture deleteCapacityProvider(
DeleteCapacityProviderRequest deleteCapacityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified capacity provider.
*
*
*
* The FARGATE
and FARGATE_SPOT
capacity providers are reserved and can't be deleted. You
* can disassociate them from a cluster using either the PutClusterCapacityProviders API or by deleting the
* cluster.
*
*
*
* Prior to a capacity provider being deleted, the capacity provider must be removed from the capacity provider
* strategy from all services. The UpdateService API can be used to remove a capacity provider from a
* service's capacity provider strategy. When updating a service, the forceNewDeployment
option can be
* used to ensure that any tasks using the Amazon EC2 instance capacity provided by the capacity provider are
* transitioned to use the capacity from the remaining capacity providers. Only capacity providers that aren't
* associated with a cluster can be deleted. To remove a capacity provider from a cluster, you can either use
* PutClusterCapacityProviders or delete the cluster.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCapacityProviderRequest.Builder} avoiding the
* need to create one manually via {@link DeleteCapacityProviderRequest#builder()}
*
*
* @param deleteCapacityProviderRequest
* A {@link Consumer} that will call methods on {@link DeleteCapacityProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteCapacityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteCapacityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture deleteCapacityProvider(
Consumer deleteCapacityProviderRequest) {
return deleteCapacityProvider(DeleteCapacityProviderRequest.builder().applyMutation(deleteCapacityProviderRequest)
.build());
}
/**
*
* Deletes the specified cluster. The cluster transitions to the INACTIVE
state. Clusters with an
* INACTIVE
status might remain discoverable in your account for a period of time. However, this
* behavior is subject to change in the future. We don't recommend that you rely on INACTIVE
clusters
* persisting.
*
*
* You must deregister all container instances from this cluster before you may delete it. You can list the
* container instances in a cluster with ListContainerInstances and deregister them with
* DeregisterContainerInstance.
*
*
* @param deleteClusterRequest
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ClusterContainsContainerInstancesException You can't delete a cluster that has registered container
* instances. First, deregister the container instances before you can delete the cluster. For more
* information, see DeregisterContainerInstance.
* - ClusterContainsServicesException You can't delete a cluster that contains services. First, update the
* service to reduce its desired task count to 0, and then delete the service. For more information, see
* UpdateService and DeleteService.
* - ClusterContainsTasksException You can't delete a cluster that has active tasks.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteCluster
* @see AWS API
* Documentation
*/
default CompletableFuture deleteCluster(DeleteClusterRequest deleteClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified cluster. The cluster transitions to the INACTIVE
state. Clusters with an
* INACTIVE
status might remain discoverable in your account for a period of time. However, this
* behavior is subject to change in the future. We don't recommend that you rely on INACTIVE
clusters
* persisting.
*
*
* You must deregister all container instances from this cluster before you may delete it. You can list the
* container instances in a cluster with ListContainerInstances and deregister them with
* DeregisterContainerInstance.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteClusterRequest.Builder} avoiding the need to
* create one manually via {@link DeleteClusterRequest#builder()}
*
*
* @param deleteClusterRequest
* A {@link Consumer} that will call methods on {@link DeleteClusterRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ClusterContainsContainerInstancesException You can't delete a cluster that has registered container
* instances. First, deregister the container instances before you can delete the cluster. For more
* information, see DeregisterContainerInstance.
* - ClusterContainsServicesException You can't delete a cluster that contains services. First, update the
* service to reduce its desired task count to 0, and then delete the service. For more information, see
* UpdateService and DeleteService.
* - ClusterContainsTasksException You can't delete a cluster that has active tasks.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteCluster
* @see AWS API
* Documentation
*/
default CompletableFuture deleteCluster(Consumer deleteClusterRequest) {
return deleteCluster(DeleteClusterRequest.builder().applyMutation(deleteClusterRequest).build());
}
/**
*
* Deletes a specified service within a cluster. You can delete a service if you have no running tasks in it and the
* desired task count is zero. If the service is actively maintaining tasks, you can't delete it, and you must
* update the service to a desired task count of zero. For more information, see UpdateService.
*
*
*
* When you delete a service, if there are still running tasks that require cleanup, the service status moves from
* ACTIVE
to DRAINING
, and the service is no longer visible in the console or in the
* ListServices API operation. After all tasks have transitioned to either STOPPING
or
* STOPPED
status, the service status moves from DRAINING
to INACTIVE
.
* Services in the DRAINING
or INACTIVE
status can still be viewed with the
* DescribeServices API operation. However, in the future, INACTIVE
services may be cleaned up
* and purged from Amazon ECS record keeping, and DescribeServices calls on those services return a
* ServiceNotFoundException
error.
*
*
*
* If you attempt to create a new service with the same name as an existing service in either ACTIVE
or
* DRAINING
status, you receive an error.
*
*
*
* @param deleteServiceRequest
* @return A Java Future containing the result of the DeleteService operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteService
* @see AWS API
* Documentation
*/
default CompletableFuture deleteService(DeleteServiceRequest deleteServiceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified service within a cluster. You can delete a service if you have no running tasks in it and the
* desired task count is zero. If the service is actively maintaining tasks, you can't delete it, and you must
* update the service to a desired task count of zero. For more information, see UpdateService.
*
*
*
* When you delete a service, if there are still running tasks that require cleanup, the service status moves from
* ACTIVE
to DRAINING
, and the service is no longer visible in the console or in the
* ListServices API operation. After all tasks have transitioned to either STOPPING
or
* STOPPED
status, the service status moves from DRAINING
to INACTIVE
.
* Services in the DRAINING
or INACTIVE
status can still be viewed with the
* DescribeServices API operation. However, in the future, INACTIVE
services may be cleaned up
* and purged from Amazon ECS record keeping, and DescribeServices calls on those services return a
* ServiceNotFoundException
error.
*
*
*
* If you attempt to create a new service with the same name as an existing service in either ACTIVE
or
* DRAINING
status, you receive an error.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServiceRequest.Builder} avoiding the need to
* create one manually via {@link DeleteServiceRequest#builder()}
*
*
* @param deleteServiceRequest
* A {@link Consumer} that will call methods on {@link DeleteServiceRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteService operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteService
* @see AWS API
* Documentation
*/
default CompletableFuture deleteService(Consumer deleteServiceRequest) {
return deleteService(DeleteServiceRequest.builder().applyMutation(deleteServiceRequest).build());
}
/**
*
* Deletes a specified task set within a service. This is used when a service uses the EXTERNAL
* deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param deleteTaskSetRequest
* @return A Java Future containing the result of the DeleteTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - TaskSetNotFoundException The specified task set wasn't found. You can view your available task sets
* with DescribeTaskSets. Task sets are specific to each cluster, service and Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteTaskSet
* @see AWS API
* Documentation
*/
default CompletableFuture deleteTaskSet(DeleteTaskSetRequest deleteTaskSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified task set within a service. This is used when a service uses the EXTERNAL
* deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteTaskSetRequest.Builder} avoiding the need to
* create one manually via {@link DeleteTaskSetRequest#builder()}
*
*
* @param deleteTaskSetRequest
* A {@link Consumer} that will call methods on {@link DeleteTaskSetRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - TaskSetNotFoundException The specified task set wasn't found. You can view your available task sets
* with DescribeTaskSets. Task sets are specific to each cluster, service and Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteTaskSet
* @see AWS API
* Documentation
*/
default CompletableFuture deleteTaskSet(Consumer deleteTaskSetRequest) {
return deleteTaskSet(DeleteTaskSetRequest.builder().applyMutation(deleteTaskSetRequest).build());
}
/**
*
* Deregisters an Amazon ECS container instance from the specified cluster. This instance is no longer available to
* run tasks.
*
*
* If you intend to use the container instance for some other purpose after deregistration, we recommend that you
* stop all of the tasks running on the container instance before deregistration. That prevents any orphaned tasks
* from consuming resources.
*
*
* Deregistering a container instance removes the instance from a cluster, but it doesn't terminate the EC2
* instance. If you are finished using the instance, be sure to terminate it in the Amazon EC2 console to stop
* billing.
*
*
*
* If you terminate a running container instance, Amazon ECS automatically deregisters the instance from your
* cluster (stopped container instances or instances with disconnected agents aren't automatically deregistered when
* terminated).
*
*
*
* @param deregisterContainerInstanceRequest
* @return A Java Future containing the result of the DeregisterContainerInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeregisterContainerInstance
* @see AWS API Documentation
*/
default CompletableFuture deregisterContainerInstance(
DeregisterContainerInstanceRequest deregisterContainerInstanceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters an Amazon ECS container instance from the specified cluster. This instance is no longer available to
* run tasks.
*
*
* If you intend to use the container instance for some other purpose after deregistration, we recommend that you
* stop all of the tasks running on the container instance before deregistration. That prevents any orphaned tasks
* from consuming resources.
*
*
* Deregistering a container instance removes the instance from a cluster, but it doesn't terminate the EC2
* instance. If you are finished using the instance, be sure to terminate it in the Amazon EC2 console to stop
* billing.
*
*
*
* If you terminate a running container instance, Amazon ECS automatically deregisters the instance from your
* cluster (stopped container instances or instances with disconnected agents aren't automatically deregistered when
* terminated).
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterContainerInstanceRequest.Builder}
* avoiding the need to create one manually via {@link DeregisterContainerInstanceRequest#builder()}
*
*
* @param deregisterContainerInstanceRequest
* A {@link Consumer} that will call methods on {@link DeregisterContainerInstanceRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeregisterContainerInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeregisterContainerInstance
* @see AWS API Documentation
*/
default CompletableFuture deregisterContainerInstance(
Consumer deregisterContainerInstanceRequest) {
return deregisterContainerInstance(DeregisterContainerInstanceRequest.builder()
.applyMutation(deregisterContainerInstanceRequest).build());
}
/**
*
* Deregisters the specified task definition by family and revision. Upon deregistration, the task definition is
* marked as INACTIVE
. Existing tasks and services that reference an INACTIVE
task
* definition continue to run without disruption. Existing services that reference an INACTIVE
task
* definition can still scale up or down by modifying the service's desired count.
*
*
* You can't use an INACTIVE
task definition to run new tasks or create new services, and you can't
* update an existing service to reference an INACTIVE
task definition. However, there may be up to a
* 10-minute window following deregistration where these restrictions have not yet taken effect.
*
*
*
* At this time, INACTIVE
task definitions remain discoverable in your account indefinitely. However,
* this behavior is subject to change in the future. We don't recommend that you rely on INACTIVE
task
* definitions persisting beyond the lifecycle of any associated tasks and services.
*
*
*
* @param deregisterTaskDefinitionRequest
* @return A Java Future containing the result of the DeregisterTaskDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeregisterTaskDefinition
* @see AWS
* API Documentation
*/
default CompletableFuture deregisterTaskDefinition(
DeregisterTaskDefinitionRequest deregisterTaskDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters the specified task definition by family and revision. Upon deregistration, the task definition is
* marked as INACTIVE
. Existing tasks and services that reference an INACTIVE
task
* definition continue to run without disruption. Existing services that reference an INACTIVE
task
* definition can still scale up or down by modifying the service's desired count.
*
*
* You can't use an INACTIVE
task definition to run new tasks or create new services, and you can't
* update an existing service to reference an INACTIVE
task definition. However, there may be up to a
* 10-minute window following deregistration where these restrictions have not yet taken effect.
*
*
*
* At this time, INACTIVE
task definitions remain discoverable in your account indefinitely. However,
* this behavior is subject to change in the future. We don't recommend that you rely on INACTIVE
task
* definitions persisting beyond the lifecycle of any associated tasks and services.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterTaskDefinitionRequest.Builder} avoiding
* the need to create one manually via {@link DeregisterTaskDefinitionRequest#builder()}
*
*
* @param deregisterTaskDefinitionRequest
* A {@link Consumer} that will call methods on {@link DeregisterTaskDefinitionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeregisterTaskDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeregisterTaskDefinition
* @see AWS
* API Documentation
*/
default CompletableFuture deregisterTaskDefinition(
Consumer deregisterTaskDefinitionRequest) {
return deregisterTaskDefinition(DeregisterTaskDefinitionRequest.builder().applyMutation(deregisterTaskDefinitionRequest)
.build());
}
/**
*
* Describes one or more of your capacity providers.
*
*
* @param describeCapacityProvidersRequest
* @return A Java Future containing the result of the DescribeCapacityProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeCapacityProviders
* @see AWS
* API Documentation
*/
default CompletableFuture describeCapacityProviders(
DescribeCapacityProvidersRequest describeCapacityProvidersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes one or more of your capacity providers.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCapacityProvidersRequest.Builder} avoiding
* the need to create one manually via {@link DescribeCapacityProvidersRequest#builder()}
*
*
* @param describeCapacityProvidersRequest
* A {@link Consumer} that will call methods on {@link DescribeCapacityProvidersRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeCapacityProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeCapacityProviders
* @see AWS
* API Documentation
*/
default CompletableFuture describeCapacityProviders(
Consumer describeCapacityProvidersRequest) {
return describeCapacityProviders(DescribeCapacityProvidersRequest.builder()
.applyMutation(describeCapacityProvidersRequest).build());
}
/**
*
* Describes one or more of your clusters.
*
*
* @param describeClustersRequest
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeClusters
* @see AWS API
* Documentation
*/
default CompletableFuture describeClusters(DescribeClustersRequest describeClustersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes one or more of your clusters.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeClustersRequest.Builder} avoiding the need
* to create one manually via {@link DescribeClustersRequest#builder()}
*
*
* @param describeClustersRequest
* A {@link Consumer} that will call methods on {@link DescribeClustersRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeClusters
* @see AWS API
* Documentation
*/
default CompletableFuture describeClusters(
Consumer describeClustersRequest) {
return describeClusters(DescribeClustersRequest.builder().applyMutation(describeClustersRequest).build());
}
/**
*
* Describes one or more of your clusters.
*
*
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeClusters
* @see AWS API
* Documentation
*/
default CompletableFuture describeClusters() {
return describeClusters(DescribeClustersRequest.builder().build());
}
/**
*
* Describes one or more container instances. Returns metadata about each container instance requested.
*
*
* @param describeContainerInstancesRequest
* @return A Java Future containing the result of the DescribeContainerInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeContainerInstances
* @see AWS API Documentation
*/
default CompletableFuture describeContainerInstances(
DescribeContainerInstancesRequest describeContainerInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes one or more container instances. Returns metadata about each container instance requested.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeContainerInstancesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeContainerInstancesRequest#builder()}
*
*
* @param describeContainerInstancesRequest
* A {@link Consumer} that will call methods on {@link DescribeContainerInstancesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeContainerInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeContainerInstances
* @see AWS API Documentation
*/
default CompletableFuture describeContainerInstances(
Consumer describeContainerInstancesRequest) {
return describeContainerInstances(DescribeContainerInstancesRequest.builder()
.applyMutation(describeContainerInstancesRequest).build());
}
/**
*
* Describes the specified services running in your cluster.
*
*
* @param describeServicesRequest
* @return A Java Future containing the result of the DescribeServices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeServices
* @see AWS API
* Documentation
*/
default CompletableFuture describeServices(DescribeServicesRequest describeServicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified services running in your cluster.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServicesRequest.Builder} avoiding the need
* to create one manually via {@link DescribeServicesRequest#builder()}
*
*
* @param describeServicesRequest
* A {@link Consumer} that will call methods on {@link DescribeServicesRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeServices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeServices
* @see AWS API
* Documentation
*/
default CompletableFuture describeServices(
Consumer describeServicesRequest) {
return describeServices(DescribeServicesRequest.builder().applyMutation(describeServicesRequest).build());
}
/**
*
* Describes a task definition. You can specify a family
and revision
to find information
* about a specific task definition, or you can simply specify the family to find the latest ACTIVE
* revision in that family.
*
*
*
* You can only describe INACTIVE
task definitions while an active task or service references them.
*
*
*
* @param describeTaskDefinitionRequest
* @return A Java Future containing the result of the DescribeTaskDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTaskDefinition
* @see AWS
* API Documentation
*/
default CompletableFuture describeTaskDefinition(
DescribeTaskDefinitionRequest describeTaskDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a task definition. You can specify a family
and revision
to find information
* about a specific task definition, or you can simply specify the family to find the latest ACTIVE
* revision in that family.
*
*
*
* You can only describe INACTIVE
task definitions while an active task or service references them.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTaskDefinitionRequest.Builder} avoiding the
* need to create one manually via {@link DescribeTaskDefinitionRequest#builder()}
*
*
* @param describeTaskDefinitionRequest
* A {@link Consumer} that will call methods on {@link DescribeTaskDefinitionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeTaskDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTaskDefinition
* @see AWS
* API Documentation
*/
default CompletableFuture describeTaskDefinition(
Consumer describeTaskDefinitionRequest) {
return describeTaskDefinition(DescribeTaskDefinitionRequest.builder().applyMutation(describeTaskDefinitionRequest)
.build());
}
/**
*
* Describes the task sets in the specified cluster and service. This is used when a service uses the
* EXTERNAL
deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param describeTaskSetsRequest
* @return A Java Future containing the result of the DescribeTaskSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTaskSets
* @see AWS API
* Documentation
*/
default CompletableFuture describeTaskSets(DescribeTaskSetsRequest describeTaskSetsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the task sets in the specified cluster and service. This is used when a service uses the
* EXTERNAL
deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTaskSetsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeTaskSetsRequest#builder()}
*
*
* @param describeTaskSetsRequest
* A {@link Consumer} that will call methods on {@link DescribeTaskSetsRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeTaskSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTaskSets
* @see AWS API
* Documentation
*/
default CompletableFuture describeTaskSets(
Consumer describeTaskSetsRequest) {
return describeTaskSets(DescribeTaskSetsRequest.builder().applyMutation(describeTaskSetsRequest).build());
}
/**
*
* Describes a specified task or tasks.
*
*
* @param describeTasksRequest
* @return A Java Future containing the result of the DescribeTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTasks
* @see AWS API
* Documentation
*/
default CompletableFuture describeTasks(DescribeTasksRequest describeTasksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes a specified task or tasks.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTasksRequest.Builder} avoiding the need to
* create one manually via {@link DescribeTasksRequest#builder()}
*
*
* @param describeTasksRequest
* A {@link Consumer} that will call methods on {@link DescribeTasksRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTasks
* @see AWS API
* Documentation
*/
default CompletableFuture describeTasks(Consumer describeTasksRequest) {
return describeTasks(DescribeTasksRequest.builder().applyMutation(describeTasksRequest).build());
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Returns an endpoint for the Amazon ECS agent to poll for updates.
*
*
* @param discoverPollEndpointRequest
* @return A Java Future containing the result of the DiscoverPollEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DiscoverPollEndpoint
* @see AWS API
* Documentation
*/
default CompletableFuture discoverPollEndpoint(
DiscoverPollEndpointRequest discoverPollEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Returns an endpoint for the Amazon ECS agent to poll for updates.
*
*
*
* This is a convenience which creates an instance of the {@link DiscoverPollEndpointRequest.Builder} avoiding the
* need to create one manually via {@link DiscoverPollEndpointRequest#builder()}
*
*
* @param discoverPollEndpointRequest
* A {@link Consumer} that will call methods on {@link DiscoverPollEndpointRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DiscoverPollEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DiscoverPollEndpoint
* @see AWS API
* Documentation
*/
default CompletableFuture discoverPollEndpoint(
Consumer discoverPollEndpointRequest) {
return discoverPollEndpoint(DiscoverPollEndpointRequest.builder().applyMutation(discoverPollEndpointRequest).build());
}
/**
*
* Runs a command remotely on a container within a task.
*
*
* @param executeCommandRequest
* @return A Java Future containing the result of the ExecuteCommand operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotConnectedException The target container isn't properly configured with the execute command
* agent or the container is no longer active or running.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ExecuteCommand
* @see AWS API
* Documentation
*/
default CompletableFuture executeCommand(ExecuteCommandRequest executeCommandRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Runs a command remotely on a container within a task.
*
*
*
* This is a convenience which creates an instance of the {@link ExecuteCommandRequest.Builder} avoiding the need to
* create one manually via {@link ExecuteCommandRequest#builder()}
*
*
* @param executeCommandRequest
* A {@link Consumer} that will call methods on {@link ExecuteCommandRequest.Builder} to create a request.
* @return A Java Future containing the result of the ExecuteCommand operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotConnectedException The target container isn't properly configured with the execute command
* agent or the container is no longer active or running.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ExecuteCommand
* @see AWS API
* Documentation
*/
default CompletableFuture executeCommand(Consumer executeCommandRequest) {
return executeCommand(ExecuteCommandRequest.builder().applyMutation(executeCommandRequest).build());
}
/**
*
* Lists the account settings for a specified principal.
*
*
* @param listAccountSettingsRequest
* @return A Java Future containing the result of the ListAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAccountSettings
* @see AWS API
* Documentation
*/
default CompletableFuture listAccountSettings(
ListAccountSettingsRequest listAccountSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the account settings for a specified principal.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccountSettingsRequest.Builder} avoiding the
* need to create one manually via {@link ListAccountSettingsRequest#builder()}
*
*
* @param listAccountSettingsRequest
* A {@link Consumer} that will call methods on {@link ListAccountSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAccountSettings
* @see AWS API
* Documentation
*/
default CompletableFuture listAccountSettings(
Consumer listAccountSettingsRequest) {
return listAccountSettings(ListAccountSettingsRequest.builder().applyMutation(listAccountSettingsRequest).build());
}
/**
*
* Lists the account settings for a specified principal.
*
*
* @return A Java Future containing the result of the ListAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAccountSettings
* @see AWS API
* Documentation
*/
default CompletableFuture listAccountSettings() {
return listAccountSettings(ListAccountSettingsRequest.builder().build());
}
/**
*
* Lists the account settings for a specified principal.
*
*
*
* This is a variant of
* {@link #listAccountSettings(software.amazon.awssdk.services.ecs.model.ListAccountSettingsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAccountSettingsPublisher publisher = client.listAccountSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAccountSettingsPublisher publisher = client.listAccountSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListAccountSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountSettings(software.amazon.awssdk.services.ecs.model.ListAccountSettingsRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAccountSettings
* @see AWS API
* Documentation
*/
default ListAccountSettingsPublisher listAccountSettingsPaginator() {
return listAccountSettingsPaginator(ListAccountSettingsRequest.builder().build());
}
/**
*
* Lists the account settings for a specified principal.
*
*
*
* This is a variant of
* {@link #listAccountSettings(software.amazon.awssdk.services.ecs.model.ListAccountSettingsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAccountSettingsPublisher publisher = client.listAccountSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAccountSettingsPublisher publisher = client.listAccountSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListAccountSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountSettings(software.amazon.awssdk.services.ecs.model.ListAccountSettingsRequest)} operation.
*
*
* @param listAccountSettingsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAccountSettings
* @see AWS API
* Documentation
*/
default ListAccountSettingsPublisher listAccountSettingsPaginator(ListAccountSettingsRequest listAccountSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the account settings for a specified principal.
*
*
*
* This is a variant of
* {@link #listAccountSettings(software.amazon.awssdk.services.ecs.model.ListAccountSettingsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAccountSettingsPublisher publisher = client.listAccountSettingsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAccountSettingsPublisher publisher = client.listAccountSettingsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListAccountSettingsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountSettings(software.amazon.awssdk.services.ecs.model.ListAccountSettingsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAccountSettingsRequest.Builder} avoiding the
* need to create one manually via {@link ListAccountSettingsRequest#builder()}
*
*
* @param listAccountSettingsRequest
* A {@link Consumer} that will call methods on {@link ListAccountSettingsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAccountSettings
* @see AWS API
* Documentation
*/
default ListAccountSettingsPublisher listAccountSettingsPaginator(
Consumer listAccountSettingsRequest) {
return listAccountSettingsPaginator(ListAccountSettingsRequest.builder().applyMutation(listAccountSettingsRequest)
.build());
}
/**
*
* Lists the attributes for Amazon ECS resources within a specified target type and cluster. When you specify a
* target type and cluster, ListAttributes
returns a list of attribute objects, one for each attribute
* on each resource. You can filter the list of results to a single attribute name to only return results that have
* that name. You can also filter the results by attribute name and value. You can do this, for example, to see
* which container instances in a cluster are running a Linux AMI (ecs.os-type=linux
).
*
*
* @param listAttributesRequest
* @return A Java Future containing the result of the ListAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAttributes
* @see AWS API
* Documentation
*/
default CompletableFuture listAttributes(ListAttributesRequest listAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the attributes for Amazon ECS resources within a specified target type and cluster. When you specify a
* target type and cluster, ListAttributes
returns a list of attribute objects, one for each attribute
* on each resource. You can filter the list of results to a single attribute name to only return results that have
* that name. You can also filter the results by attribute name and value. You can do this, for example, to see
* which container instances in a cluster are running a Linux AMI (ecs.os-type=linux
).
*
*
*
* This is a convenience which creates an instance of the {@link ListAttributesRequest.Builder} avoiding the need to
* create one manually via {@link ListAttributesRequest#builder()}
*
*
* @param listAttributesRequest
* A {@link Consumer} that will call methods on {@link ListAttributesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAttributes
* @see AWS API
* Documentation
*/
default CompletableFuture listAttributes(Consumer listAttributesRequest) {
return listAttributes(ListAttributesRequest.builder().applyMutation(listAttributesRequest).build());
}
/**
*
* Lists the attributes for Amazon ECS resources within a specified target type and cluster. When you specify a
* target type and cluster, ListAttributes
returns a list of attribute objects, one for each attribute
* on each resource. You can filter the list of results to a single attribute name to only return results that have
* that name. You can also filter the results by attribute name and value. You can do this, for example, to see
* which container instances in a cluster are running a Linux AMI (ecs.os-type=linux
).
*
*
*
* This is a variant of {@link #listAttributes(software.amazon.awssdk.services.ecs.model.ListAttributesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAttributesPublisher publisher = client.listAttributesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAttributesPublisher publisher = client.listAttributesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListAttributesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttributes(software.amazon.awssdk.services.ecs.model.ListAttributesRequest)} operation.
*
*
* @param listAttributesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAttributes
* @see AWS API
* Documentation
*/
default ListAttributesPublisher listAttributesPaginator(ListAttributesRequest listAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the attributes for Amazon ECS resources within a specified target type and cluster. When you specify a
* target type and cluster, ListAttributes
returns a list of attribute objects, one for each attribute
* on each resource. You can filter the list of results to a single attribute name to only return results that have
* that name. You can also filter the results by attribute name and value. You can do this, for example, to see
* which container instances in a cluster are running a Linux AMI (ecs.os-type=linux
).
*
*
*
* This is a variant of {@link #listAttributes(software.amazon.awssdk.services.ecs.model.ListAttributesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAttributesPublisher publisher = client.listAttributesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListAttributesPublisher publisher = client.listAttributesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListAttributesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttributes(software.amazon.awssdk.services.ecs.model.ListAttributesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAttributesRequest.Builder} avoiding the need to
* create one manually via {@link ListAttributesRequest#builder()}
*
*
* @param listAttributesRequest
* A {@link Consumer} that will call methods on {@link ListAttributesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAttributes
* @see AWS API
* Documentation
*/
default ListAttributesPublisher listAttributesPaginator(Consumer listAttributesRequest) {
return listAttributesPaginator(ListAttributesRequest.builder().applyMutation(listAttributesRequest).build());
}
/**
*
* Returns a list of existing clusters.
*
*
* @param listClustersRequest
* @return A Java Future containing the result of the ListClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListClusters
* @see AWS API
* Documentation
*/
default CompletableFuture listClusters(ListClustersRequest listClustersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of existing clusters.
*
*
*
* This is a convenience which creates an instance of the {@link ListClustersRequest.Builder} avoiding the need to
* create one manually via {@link ListClustersRequest#builder()}
*
*
* @param listClustersRequest
* A {@link Consumer} that will call methods on {@link ListClustersRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListClusters
* @see AWS API
* Documentation
*/
default CompletableFuture listClusters(Consumer listClustersRequest) {
return listClusters(ListClustersRequest.builder().applyMutation(listClustersRequest).build());
}
/**
*
* Returns a list of existing clusters.
*
*
* @return A Java Future containing the result of the ListClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListClusters
* @see AWS API
* Documentation
*/
default CompletableFuture listClusters() {
return listClusters(ListClustersRequest.builder().build());
}
/**
*
* Returns a list of existing clusters.
*
*
*
* This is a variant of {@link #listClusters(software.amazon.awssdk.services.ecs.model.ListClustersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listClusters(software.amazon.awssdk.services.ecs.model.ListClustersRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListClusters
* @see AWS API
* Documentation
*/
default ListClustersPublisher listClustersPaginator() {
return listClustersPaginator(ListClustersRequest.builder().build());
}
/**
*
* Returns a list of existing clusters.
*
*
*
* This is a variant of {@link #listClusters(software.amazon.awssdk.services.ecs.model.ListClustersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listClusters(software.amazon.awssdk.services.ecs.model.ListClustersRequest)} operation.
*
*
* @param listClustersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListClusters
* @see AWS API
* Documentation
*/
default ListClustersPublisher listClustersPaginator(ListClustersRequest listClustersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of existing clusters.
*
*
*
* This is a variant of {@link #listClusters(software.amazon.awssdk.services.ecs.model.ListClustersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListClustersPublisher publisher = client.listClustersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListClustersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listClusters(software.amazon.awssdk.services.ecs.model.ListClustersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListClustersRequest.Builder} avoiding the need to
* create one manually via {@link ListClustersRequest#builder()}
*
*
* @param listClustersRequest
* A {@link Consumer} that will call methods on {@link ListClustersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListClusters
* @see AWS API
* Documentation
*/
default ListClustersPublisher listClustersPaginator(Consumer listClustersRequest) {
return listClustersPaginator(ListClustersRequest.builder().applyMutation(listClustersRequest).build());
}
/**
*
* Returns a list of container instances in a specified cluster. You can filter the results of a
* ListContainerInstances
operation with cluster query language statements inside the
* filter
parameter. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
* @param listContainerInstancesRequest
* @return A Java Future containing the result of the ListContainerInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListContainerInstances
* @see AWS
* API Documentation
*/
default CompletableFuture listContainerInstances(
ListContainerInstancesRequest listContainerInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of container instances in a specified cluster. You can filter the results of a
* ListContainerInstances
operation with cluster query language statements inside the
* filter
parameter. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListContainerInstancesRequest.Builder} avoiding the
* need to create one manually via {@link ListContainerInstancesRequest#builder()}
*
*
* @param listContainerInstancesRequest
* A {@link Consumer} that will call methods on {@link ListContainerInstancesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListContainerInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListContainerInstances
* @see AWS
* API Documentation
*/
default CompletableFuture listContainerInstances(
Consumer listContainerInstancesRequest) {
return listContainerInstances(ListContainerInstancesRequest.builder().applyMutation(listContainerInstancesRequest)
.build());
}
/**
*
* Returns a list of container instances in a specified cluster. You can filter the results of a
* ListContainerInstances
operation with cluster query language statements inside the
* filter
parameter. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
* @return A Java Future containing the result of the ListContainerInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListContainerInstances
* @see AWS
* API Documentation
*/
default CompletableFuture listContainerInstances() {
return listContainerInstances(ListContainerInstancesRequest.builder().build());
}
/**
*
* Returns a list of container instances in a specified cluster. You can filter the results of a
* ListContainerInstances
operation with cluster query language statements inside the
* filter
parameter. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a variant of
* {@link #listContainerInstances(software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListContainerInstancesPublisher publisher = client.listContainerInstancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListContainerInstancesPublisher publisher = client.listContainerInstancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListContainerInstancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listContainerInstances(software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListContainerInstances
* @see AWS
* API Documentation
*/
default ListContainerInstancesPublisher listContainerInstancesPaginator() {
return listContainerInstancesPaginator(ListContainerInstancesRequest.builder().build());
}
/**
*
* Returns a list of container instances in a specified cluster. You can filter the results of a
* ListContainerInstances
operation with cluster query language statements inside the
* filter
parameter. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a variant of
* {@link #listContainerInstances(software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListContainerInstancesPublisher publisher = client.listContainerInstancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListContainerInstancesPublisher publisher = client.listContainerInstancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListContainerInstancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listContainerInstances(software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest)}
* operation.
*
*
* @param listContainerInstancesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListContainerInstances
* @see AWS
* API Documentation
*/
default ListContainerInstancesPublisher listContainerInstancesPaginator(
ListContainerInstancesRequest listContainerInstancesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of container instances in a specified cluster. You can filter the results of a
* ListContainerInstances
operation with cluster query language statements inside the
* filter
parameter. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a variant of
* {@link #listContainerInstances(software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListContainerInstancesPublisher publisher = client.listContainerInstancesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListContainerInstancesPublisher publisher = client.listContainerInstancesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListContainerInstancesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listContainerInstances(software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListContainerInstancesRequest.Builder} avoiding the
* need to create one manually via {@link ListContainerInstancesRequest#builder()}
*
*
* @param listContainerInstancesRequest
* A {@link Consumer} that will call methods on {@link ListContainerInstancesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListContainerInstances
* @see AWS
* API Documentation
*/
default ListContainerInstancesPublisher listContainerInstancesPaginator(
Consumer listContainerInstancesRequest) {
return listContainerInstancesPaginator(ListContainerInstancesRequest.builder()
.applyMutation(listContainerInstancesRequest).build());
}
/**
*
* Returns a list of services. You can filter the results by cluster, launch type, and scheduling strategy.
*
*
* @param listServicesRequest
* @return A Java Future containing the result of the ListServices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListServices
* @see AWS API
* Documentation
*/
default CompletableFuture listServices(ListServicesRequest listServicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of services. You can filter the results by cluster, launch type, and scheduling strategy.
*
*
*
* This is a convenience which creates an instance of the {@link ListServicesRequest.Builder} avoiding the need to
* create one manually via {@link ListServicesRequest#builder()}
*
*
* @param listServicesRequest
* A {@link Consumer} that will call methods on {@link ListServicesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListServices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListServices
* @see AWS API
* Documentation
*/
default CompletableFuture listServices(Consumer listServicesRequest) {
return listServices(ListServicesRequest.builder().applyMutation(listServicesRequest).build());
}
/**
*
* Returns a list of services. You can filter the results by cluster, launch type, and scheduling strategy.
*
*
* @return A Java Future containing the result of the ListServices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListServices
* @see AWS API
* Documentation
*/
default CompletableFuture listServices() {
return listServices(ListServicesRequest.builder().build());
}
/**
*
* Returns a list of services. You can filter the results by cluster, launch type, and scheduling strategy.
*
*
*
* This is a variant of {@link #listServices(software.amazon.awssdk.services.ecs.model.ListServicesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListServicesPublisher publisher = client.listServicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListServicesPublisher publisher = client.listServicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListServicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServices(software.amazon.awssdk.services.ecs.model.ListServicesRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListServices
* @see AWS API
* Documentation
*/
default ListServicesPublisher listServicesPaginator() {
return listServicesPaginator(ListServicesRequest.builder().build());
}
/**
*
* Returns a list of services. You can filter the results by cluster, launch type, and scheduling strategy.
*
*
*
* This is a variant of {@link #listServices(software.amazon.awssdk.services.ecs.model.ListServicesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListServicesPublisher publisher = client.listServicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListServicesPublisher publisher = client.listServicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListServicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServices(software.amazon.awssdk.services.ecs.model.ListServicesRequest)} operation.
*
*
* @param listServicesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListServices
* @see AWS API
* Documentation
*/
default ListServicesPublisher listServicesPaginator(ListServicesRequest listServicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of services. You can filter the results by cluster, launch type, and scheduling strategy.
*
*
*
* This is a variant of {@link #listServices(software.amazon.awssdk.services.ecs.model.ListServicesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListServicesPublisher publisher = client.listServicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListServicesPublisher publisher = client.listServicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListServicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listServices(software.amazon.awssdk.services.ecs.model.ListServicesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListServicesRequest.Builder} avoiding the need to
* create one manually via {@link ListServicesRequest#builder()}
*
*
* @param listServicesRequest
* A {@link Consumer} that will call methods on {@link ListServicesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListServices
* @see AWS API
* Documentation
*/
default ListServicesPublisher listServicesPaginator(Consumer listServicesRequest) {
return listServicesPaginator(ListServicesRequest.builder().applyMutation(listServicesRequest).build());
}
/**
*
* List the tags for an Amazon ECS resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* List the tags for an Amazon ECS resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Returns a list of task definition families that are registered to your account. This list includes task
* definition families that no longer have any ACTIVE
task definition revisions.
*
*
* You can filter out task definition families that don't contain any ACTIVE
task definition revisions
* by setting the status
parameter to ACTIVE
. You can also filter the results with the
* familyPrefix
parameter.
*
*
* @param listTaskDefinitionFamiliesRequest
* @return A Java Future containing the result of the ListTaskDefinitionFamilies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitionFamilies
* @see AWS API Documentation
*/
default CompletableFuture listTaskDefinitionFamilies(
ListTaskDefinitionFamiliesRequest listTaskDefinitionFamiliesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of task definition families that are registered to your account. This list includes task
* definition families that no longer have any ACTIVE
task definition revisions.
*
*
* You can filter out task definition families that don't contain any ACTIVE
task definition revisions
* by setting the status
parameter to ACTIVE
. You can also filter the results with the
* familyPrefix
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListTaskDefinitionFamiliesRequest.Builder} avoiding
* the need to create one manually via {@link ListTaskDefinitionFamiliesRequest#builder()}
*
*
* @param listTaskDefinitionFamiliesRequest
* A {@link Consumer} that will call methods on {@link ListTaskDefinitionFamiliesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTaskDefinitionFamilies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitionFamilies
* @see AWS API Documentation
*/
default CompletableFuture listTaskDefinitionFamilies(
Consumer listTaskDefinitionFamiliesRequest) {
return listTaskDefinitionFamilies(ListTaskDefinitionFamiliesRequest.builder()
.applyMutation(listTaskDefinitionFamiliesRequest).build());
}
/**
*
* Returns a list of task definition families that are registered to your account. This list includes task
* definition families that no longer have any ACTIVE
task definition revisions.
*
*
* You can filter out task definition families that don't contain any ACTIVE
task definition revisions
* by setting the status
parameter to ACTIVE
. You can also filter the results with the
* familyPrefix
parameter.
*
*
* @return A Java Future containing the result of the ListTaskDefinitionFamilies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitionFamilies
* @see AWS API Documentation
*/
default CompletableFuture listTaskDefinitionFamilies() {
return listTaskDefinitionFamilies(ListTaskDefinitionFamiliesRequest.builder().build());
}
/**
*
* Returns a list of task definition families that are registered to your account. This list includes task
* definition families that no longer have any ACTIVE
task definition revisions.
*
*
* You can filter out task definition families that don't contain any ACTIVE
task definition revisions
* by setting the status
parameter to ACTIVE
. You can also filter the results with the
* familyPrefix
parameter.
*
*
*
* This is a variant of
* {@link #listTaskDefinitionFamilies(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionFamiliesPublisher publisher = client.listTaskDefinitionFamiliesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionFamiliesPublisher publisher = client.listTaskDefinitionFamiliesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTaskDefinitionFamilies(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesRequest)}
* operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitionFamilies
* @see AWS API Documentation
*/
default ListTaskDefinitionFamiliesPublisher listTaskDefinitionFamiliesPaginator() {
return listTaskDefinitionFamiliesPaginator(ListTaskDefinitionFamiliesRequest.builder().build());
}
/**
*
* Returns a list of task definition families that are registered to your account. This list includes task
* definition families that no longer have any ACTIVE
task definition revisions.
*
*
* You can filter out task definition families that don't contain any ACTIVE
task definition revisions
* by setting the status
parameter to ACTIVE
. You can also filter the results with the
* familyPrefix
parameter.
*
*
*
* This is a variant of
* {@link #listTaskDefinitionFamilies(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionFamiliesPublisher publisher = client.listTaskDefinitionFamiliesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionFamiliesPublisher publisher = client.listTaskDefinitionFamiliesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTaskDefinitionFamilies(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesRequest)}
* operation.
*
*
* @param listTaskDefinitionFamiliesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitionFamilies
* @see AWS API Documentation
*/
default ListTaskDefinitionFamiliesPublisher listTaskDefinitionFamiliesPaginator(
ListTaskDefinitionFamiliesRequest listTaskDefinitionFamiliesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of task definition families that are registered to your account. This list includes task
* definition families that no longer have any ACTIVE
task definition revisions.
*
*
* You can filter out task definition families that don't contain any ACTIVE
task definition revisions
* by setting the status
parameter to ACTIVE
. You can also filter the results with the
* familyPrefix
parameter.
*
*
*
* This is a variant of
* {@link #listTaskDefinitionFamilies(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionFamiliesPublisher publisher = client.listTaskDefinitionFamiliesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionFamiliesPublisher publisher = client.listTaskDefinitionFamiliesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTaskDefinitionFamilies(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListTaskDefinitionFamiliesRequest.Builder} avoiding
* the need to create one manually via {@link ListTaskDefinitionFamiliesRequest#builder()}
*
*
* @param listTaskDefinitionFamiliesRequest
* A {@link Consumer} that will call methods on {@link ListTaskDefinitionFamiliesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitionFamilies
* @see AWS API Documentation
*/
default ListTaskDefinitionFamiliesPublisher listTaskDefinitionFamiliesPaginator(
Consumer listTaskDefinitionFamiliesRequest) {
return listTaskDefinitionFamiliesPaginator(ListTaskDefinitionFamiliesRequest.builder()
.applyMutation(listTaskDefinitionFamiliesRequest).build());
}
/**
*
* Returns a list of task definitions that are registered to your account. You can filter the results by family name
* with the familyPrefix
parameter or by status with the status
parameter.
*
*
* @param listTaskDefinitionsRequest
* @return A Java Future containing the result of the ListTaskDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitions
* @see AWS API
* Documentation
*/
default CompletableFuture listTaskDefinitions(
ListTaskDefinitionsRequest listTaskDefinitionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of task definitions that are registered to your account. You can filter the results by family name
* with the familyPrefix
parameter or by status with the status
parameter.
*
*
*
* This is a convenience which creates an instance of the {@link ListTaskDefinitionsRequest.Builder} avoiding the
* need to create one manually via {@link ListTaskDefinitionsRequest#builder()}
*
*
* @param listTaskDefinitionsRequest
* A {@link Consumer} that will call methods on {@link ListTaskDefinitionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTaskDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitions
* @see AWS API
* Documentation
*/
default CompletableFuture listTaskDefinitions(
Consumer listTaskDefinitionsRequest) {
return listTaskDefinitions(ListTaskDefinitionsRequest.builder().applyMutation(listTaskDefinitionsRequest).build());
}
/**
*
* Returns a list of task definitions that are registered to your account. You can filter the results by family name
* with the familyPrefix
parameter or by status with the status
parameter.
*
*
* @return A Java Future containing the result of the ListTaskDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitions
* @see AWS API
* Documentation
*/
default CompletableFuture listTaskDefinitions() {
return listTaskDefinitions(ListTaskDefinitionsRequest.builder().build());
}
/**
*
* Returns a list of task definitions that are registered to your account. You can filter the results by family name
* with the familyPrefix
parameter or by status with the status
parameter.
*
*
*
* This is a variant of
* {@link #listTaskDefinitions(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionsPublisher publisher = client.listTaskDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionsPublisher publisher = client.listTaskDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTaskDefinitions(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitions
* @see AWS API
* Documentation
*/
default ListTaskDefinitionsPublisher listTaskDefinitionsPaginator() {
return listTaskDefinitionsPaginator(ListTaskDefinitionsRequest.builder().build());
}
/**
*
* Returns a list of task definitions that are registered to your account. You can filter the results by family name
* with the familyPrefix
parameter or by status with the status
parameter.
*
*
*
* This is a variant of
* {@link #listTaskDefinitions(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionsPublisher publisher = client.listTaskDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionsPublisher publisher = client.listTaskDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTaskDefinitions(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsRequest)} operation.
*
*
* @param listTaskDefinitionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitions
* @see AWS API
* Documentation
*/
default ListTaskDefinitionsPublisher listTaskDefinitionsPaginator(ListTaskDefinitionsRequest listTaskDefinitionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of task definitions that are registered to your account. You can filter the results by family name
* with the familyPrefix
parameter or by status with the status
parameter.
*
*
*
* This is a variant of
* {@link #listTaskDefinitions(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionsPublisher publisher = client.listTaskDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTaskDefinitionsPublisher publisher = client.listTaskDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTaskDefinitions(software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListTaskDefinitionsRequest.Builder} avoiding the
* need to create one manually via {@link ListTaskDefinitionsRequest#builder()}
*
*
* @param listTaskDefinitionsRequest
* A {@link Consumer} that will call methods on {@link ListTaskDefinitionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitions
* @see AWS API
* Documentation
*/
default ListTaskDefinitionsPublisher listTaskDefinitionsPaginator(
Consumer listTaskDefinitionsRequest) {
return listTaskDefinitionsPaginator(ListTaskDefinitionsRequest.builder().applyMutation(listTaskDefinitionsRequest)
.build());
}
/**
*
* Returns a list of tasks. You can filter the results by cluster, task definition family, container instance,
* launch type, what IAM principal started the task, or by the desired status of the task.
*
*
* Recently stopped tasks might appear in the returned results. Currently, stopped tasks appear in the returned
* results for at least one hour.
*
*
* @param listTasksRequest
* @return A Java Future containing the result of the ListTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTasks
* @see AWS API
* Documentation
*/
default CompletableFuture listTasks(ListTasksRequest listTasksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of tasks. You can filter the results by cluster, task definition family, container instance,
* launch type, what IAM principal started the task, or by the desired status of the task.
*
*
* Recently stopped tasks might appear in the returned results. Currently, stopped tasks appear in the returned
* results for at least one hour.
*
*
*
* This is a convenience which creates an instance of the {@link ListTasksRequest.Builder} avoiding the need to
* create one manually via {@link ListTasksRequest#builder()}
*
*
* @param listTasksRequest
* A {@link Consumer} that will call methods on {@link ListTasksRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTasks
* @see AWS API
* Documentation
*/
default CompletableFuture listTasks(Consumer listTasksRequest) {
return listTasks(ListTasksRequest.builder().applyMutation(listTasksRequest).build());
}
/**
*
* Returns a list of tasks. You can filter the results by cluster, task definition family, container instance,
* launch type, what IAM principal started the task, or by the desired status of the task.
*
*
* Recently stopped tasks might appear in the returned results. Currently, stopped tasks appear in the returned
* results for at least one hour.
*
*
* @return A Java Future containing the result of the ListTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTasks
* @see AWS API
* Documentation
*/
default CompletableFuture listTasks() {
return listTasks(ListTasksRequest.builder().build());
}
/**
*
* Returns a list of tasks. You can filter the results by cluster, task definition family, container instance,
* launch type, what IAM principal started the task, or by the desired status of the task.
*
*
* Recently stopped tasks might appear in the returned results. Currently, stopped tasks appear in the returned
* results for at least one hour.
*
*
*
* This is a variant of {@link #listTasks(software.amazon.awssdk.services.ecs.model.ListTasksRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTasksPublisher publisher = client.listTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTasksPublisher publisher = client.listTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTasks(software.amazon.awssdk.services.ecs.model.ListTasksRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTasks
* @see AWS API
* Documentation
*/
default ListTasksPublisher listTasksPaginator() {
return listTasksPaginator(ListTasksRequest.builder().build());
}
/**
*
* Returns a list of tasks. You can filter the results by cluster, task definition family, container instance,
* launch type, what IAM principal started the task, or by the desired status of the task.
*
*
* Recently stopped tasks might appear in the returned results. Currently, stopped tasks appear in the returned
* results for at least one hour.
*
*
*
* This is a variant of {@link #listTasks(software.amazon.awssdk.services.ecs.model.ListTasksRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTasksPublisher publisher = client.listTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTasksPublisher publisher = client.listTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTasks(software.amazon.awssdk.services.ecs.model.ListTasksRequest)} operation.
*
*
* @param listTasksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTasks
* @see AWS API
* Documentation
*/
default ListTasksPublisher listTasksPaginator(ListTasksRequest listTasksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of tasks. You can filter the results by cluster, task definition family, container instance,
* launch type, what IAM principal started the task, or by the desired status of the task.
*
*
* Recently stopped tasks might appear in the returned results. Currently, stopped tasks appear in the returned
* results for at least one hour.
*
*
*
* This is a variant of {@link #listTasks(software.amazon.awssdk.services.ecs.model.ListTasksRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTasksPublisher publisher = client.listTasksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ecs.paginators.ListTasksPublisher publisher = client.listTasksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ecs.model.ListTasksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTasks(software.amazon.awssdk.services.ecs.model.ListTasksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListTasksRequest.Builder} avoiding the need to
* create one manually via {@link ListTasksRequest#builder()}
*
*
* @param listTasksRequest
* A {@link Consumer} that will call methods on {@link ListTasksRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTasks
* @see AWS API
* Documentation
*/
default ListTasksPublisher listTasksPaginator(Consumer listTasksRequest) {
return listTasksPaginator(ListTasksRequest.builder().applyMutation(listTasksRequest).build());
}
/**
*
* Modifies an account setting. Account settings are set on a per-Region basis.
*
*
* If you change the account setting for the root user, the default settings for all of the IAM users and roles that
* no individual account setting was specified are reset for. For more information, see Account Settings
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When serviceLongArnFormat
, taskLongArnFormat
, or
* containerInstanceLongArnFormat
are specified, the Amazon Resource Name (ARN) and resource ID format
* of the resource type for a specified IAM user, IAM role, or the root user for an account is affected. The opt-in
* and opt-out account setting must be set for each Amazon ECS resource separately. The ARN and resource ID format
* of a resource is defined by the opt-in status of the IAM user or role that created the resource. You must enable
* this setting to use Amazon ECS features such as resource tagging.
*
*
* When awsvpcTrunking
is specified, the elastic network interface (ENI) limit for any new container
* instances that support the feature is changed. If awsvpcTrunking
is enabled, any new container
* instances that support the feature are launched have the increased ENI limits available to them. For more
* information, see Elastic Network
* Interface Trunking in the Amazon Elastic Container Service Developer Guide.
*
*
* When containerInsights
is specified, the default setting indicating whether CloudWatch Container
* Insights is enabled for your clusters is changed. If containerInsights
is enabled, any new clusters
* that are created will have Container Insights enabled unless you disable it during cluster creation. For more
* information, see CloudWatch
* Container Insights in the Amazon Elastic Container Service Developer Guide.
*
*
* @param putAccountSettingRequest
* @return A Java Future containing the result of the PutAccountSetting operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAccountSetting
* @see AWS API
* Documentation
*/
default CompletableFuture putAccountSetting(PutAccountSettingRequest putAccountSettingRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies an account setting. Account settings are set on a per-Region basis.
*
*
* If you change the account setting for the root user, the default settings for all of the IAM users and roles that
* no individual account setting was specified are reset for. For more information, see Account Settings
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When serviceLongArnFormat
, taskLongArnFormat
, or
* containerInstanceLongArnFormat
are specified, the Amazon Resource Name (ARN) and resource ID format
* of the resource type for a specified IAM user, IAM role, or the root user for an account is affected. The opt-in
* and opt-out account setting must be set for each Amazon ECS resource separately. The ARN and resource ID format
* of a resource is defined by the opt-in status of the IAM user or role that created the resource. You must enable
* this setting to use Amazon ECS features such as resource tagging.
*
*
* When awsvpcTrunking
is specified, the elastic network interface (ENI) limit for any new container
* instances that support the feature is changed. If awsvpcTrunking
is enabled, any new container
* instances that support the feature are launched have the increased ENI limits available to them. For more
* information, see Elastic Network
* Interface Trunking in the Amazon Elastic Container Service Developer Guide.
*
*
* When containerInsights
is specified, the default setting indicating whether CloudWatch Container
* Insights is enabled for your clusters is changed. If containerInsights
is enabled, any new clusters
* that are created will have Container Insights enabled unless you disable it during cluster creation. For more
* information, see CloudWatch
* Container Insights in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutAccountSettingRequest.Builder} avoiding the need
* to create one manually via {@link PutAccountSettingRequest#builder()}
*
*
* @param putAccountSettingRequest
* A {@link Consumer} that will call methods on {@link PutAccountSettingRequest.Builder} to create a request.
* @return A Java Future containing the result of the PutAccountSetting operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAccountSetting
* @see AWS API
* Documentation
*/
default CompletableFuture putAccountSetting(
Consumer putAccountSettingRequest) {
return putAccountSetting(PutAccountSettingRequest.builder().applyMutation(putAccountSettingRequest).build());
}
/**
*
* Modifies an account setting for all IAM users on an account for whom no individual account setting has been
* specified. Account settings are set on a per-Region basis.
*
*
* @param putAccountSettingDefaultRequest
* @return A Java Future containing the result of the PutAccountSettingDefault operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAccountSettingDefault
* @see AWS
* API Documentation
*/
default CompletableFuture putAccountSettingDefault(
PutAccountSettingDefaultRequest putAccountSettingDefaultRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies an account setting for all IAM users on an account for whom no individual account setting has been
* specified. Account settings are set on a per-Region basis.
*
*
*
* This is a convenience which creates an instance of the {@link PutAccountSettingDefaultRequest.Builder} avoiding
* the need to create one manually via {@link PutAccountSettingDefaultRequest#builder()}
*
*
* @param putAccountSettingDefaultRequest
* A {@link Consumer} that will call methods on {@link PutAccountSettingDefaultRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutAccountSettingDefault operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAccountSettingDefault
* @see AWS
* API Documentation
*/
default CompletableFuture putAccountSettingDefault(
Consumer putAccountSettingDefaultRequest) {
return putAccountSettingDefault(PutAccountSettingDefaultRequest.builder().applyMutation(putAccountSettingDefaultRequest)
.build());
}
/**
*
* Create or update an attribute on an Amazon ECS resource. If the attribute doesn't exist, it's created. If the
* attribute exists, its value is replaced with the specified value. To delete an attribute, use
* DeleteAttributes. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
* @param putAttributesRequest
* @return A Java Future containing the result of the PutAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotFoundException The specified target wasn't found. You can view your available container
* instances with ListContainerInstances. Amazon ECS container instances are cluster-specific and
* Region-specific.
* - AttributeLimitExceededException You can apply up to 10 custom attributes for each resource. You can
* view the attributes of a resource with ListAttributes. You can remove existing attributes on a
* resource with DeleteAttributes.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAttributes
* @see AWS API
* Documentation
*/
default CompletableFuture putAttributes(PutAttributesRequest putAttributesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create or update an attribute on an Amazon ECS resource. If the attribute doesn't exist, it's created. If the
* attribute exists, its value is replaced with the specified value. To delete an attribute, use
* DeleteAttributes. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutAttributesRequest.Builder} avoiding the need to
* create one manually via {@link PutAttributesRequest#builder()}
*
*
* @param putAttributesRequest
* A {@link Consumer} that will call methods on {@link PutAttributesRequest.Builder} to create a request.
* @return A Java Future containing the result of the PutAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotFoundException The specified target wasn't found. You can view your available container
* instances with ListContainerInstances. Amazon ECS container instances are cluster-specific and
* Region-specific.
* - AttributeLimitExceededException You can apply up to 10 custom attributes for each resource. You can
* view the attributes of a resource with ListAttributes. You can remove existing attributes on a
* resource with DeleteAttributes.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAttributes
* @see AWS API
* Documentation
*/
default CompletableFuture putAttributes(Consumer putAttributesRequest) {
return putAttributes(PutAttributesRequest.builder().applyMutation(putAttributesRequest).build());
}
/**
*
* Modifies the available capacity providers and the default capacity provider strategy for a cluster.
*
*
* You must specify both the available capacity providers and a default capacity provider strategy for the cluster.
* If the specified cluster has existing capacity providers associated with it, you must specify all existing
* capacity providers in addition to any new ones you want to add. Any existing capacity providers that are
* associated with a cluster that are omitted from a PutClusterCapacityProviders API call will be
* disassociated with the cluster. You can only disassociate an existing capacity provider from a cluster if it's
* not being used by any existing tasks.
*
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is specified, then
* the cluster's default capacity provider strategy is used. We recommend that you define a default capacity
* provider strategy for your cluster. However, you must specify an empty array ([]
) to bypass defining
* a default strategy.
*
*
* @param putClusterCapacityProvidersRequest
* @return A Java Future containing the result of the PutClusterCapacityProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ResourceInUseException The specified resource is in-use and can't be removed.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutClusterCapacityProviders
* @see AWS API Documentation
*/
default CompletableFuture putClusterCapacityProviders(
PutClusterCapacityProvidersRequest putClusterCapacityProvidersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the available capacity providers and the default capacity provider strategy for a cluster.
*
*
* You must specify both the available capacity providers and a default capacity provider strategy for the cluster.
* If the specified cluster has existing capacity providers associated with it, you must specify all existing
* capacity providers in addition to any new ones you want to add. Any existing capacity providers that are
* associated with a cluster that are omitted from a PutClusterCapacityProviders API call will be
* disassociated with the cluster. You can only disassociate an existing capacity provider from a cluster if it's
* not being used by any existing tasks.
*
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is specified, then
* the cluster's default capacity provider strategy is used. We recommend that you define a default capacity
* provider strategy for your cluster. However, you must specify an empty array ([]
) to bypass defining
* a default strategy.
*
*
*
* This is a convenience which creates an instance of the {@link PutClusterCapacityProvidersRequest.Builder}
* avoiding the need to create one manually via {@link PutClusterCapacityProvidersRequest#builder()}
*
*
* @param putClusterCapacityProvidersRequest
* A {@link Consumer} that will call methods on {@link PutClusterCapacityProvidersRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the PutClusterCapacityProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ResourceInUseException The specified resource is in-use and can't be removed.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutClusterCapacityProviders
* @see AWS API Documentation
*/
default CompletableFuture putClusterCapacityProviders(
Consumer putClusterCapacityProvidersRequest) {
return putClusterCapacityProviders(PutClusterCapacityProvidersRequest.builder()
.applyMutation(putClusterCapacityProvidersRequest).build());
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Registers an EC2 instance into the specified cluster. This instance becomes available to place containers on.
*
*
* @param registerContainerInstanceRequest
* @return A Java Future containing the result of the RegisterContainerInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.RegisterContainerInstance
* @see AWS
* API Documentation
*/
default CompletableFuture registerContainerInstance(
RegisterContainerInstanceRequest registerContainerInstanceRequest) {
throw new UnsupportedOperationException();
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Registers an EC2 instance into the specified cluster. This instance becomes available to place containers on.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterContainerInstanceRequest.Builder} avoiding
* the need to create one manually via {@link RegisterContainerInstanceRequest#builder()}
*
*
* @param registerContainerInstanceRequest
* A {@link Consumer} that will call methods on {@link RegisterContainerInstanceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the RegisterContainerInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.RegisterContainerInstance
* @see AWS
* API Documentation
*/
default CompletableFuture registerContainerInstance(
Consumer registerContainerInstanceRequest) {
return registerContainerInstance(RegisterContainerInstanceRequest.builder()
.applyMutation(registerContainerInstanceRequest).build());
}
/**
*
* Registers a new task definition from the supplied family
and containerDefinitions
.
* Optionally, you can add data volumes to your containers with the volumes
parameter. For more
* information about task definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* You can specify an IAM role for your task with the taskRoleArn
parameter. When you specify an IAM
* role for a task, its containers can then use the latest versions of the CLI or SDKs to make API requests to the
* Amazon Web Services services that are specified in the IAM policy that's associated with the role. For more
* information, see IAM
* Roles for Tasks in the Amazon Elastic Container Service Developer Guide.
*
*
* You can specify a Docker networking mode for the containers in your task definition with the
* networkMode
parameter. The available network modes correspond to those described in Network settings in the Docker run
* reference. If you specify the awsvpc
network mode, the task is allocated an elastic network
* interface, and you must specify a NetworkConfiguration when you create a service or run a task with the
* task definition. For more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param registerTaskDefinitionRequest
* @return A Java Future containing the result of the RegisterTaskDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.RegisterTaskDefinition
* @see AWS
* API Documentation
*/
default CompletableFuture registerTaskDefinition(
RegisterTaskDefinitionRequest registerTaskDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Registers a new task definition from the supplied family
and containerDefinitions
.
* Optionally, you can add data volumes to your containers with the volumes
parameter. For more
* information about task definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* You can specify an IAM role for your task with the taskRoleArn
parameter. When you specify an IAM
* role for a task, its containers can then use the latest versions of the CLI or SDKs to make API requests to the
* Amazon Web Services services that are specified in the IAM policy that's associated with the role. For more
* information, see IAM
* Roles for Tasks in the Amazon Elastic Container Service Developer Guide.
*
*
* You can specify a Docker networking mode for the containers in your task definition with the
* networkMode
parameter. The available network modes correspond to those described in Network settings in the Docker run
* reference. If you specify the awsvpc
network mode, the task is allocated an elastic network
* interface, and you must specify a NetworkConfiguration when you create a service or run a task with the
* task definition. For more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterTaskDefinitionRequest.Builder} avoiding the
* need to create one manually via {@link RegisterTaskDefinitionRequest#builder()}
*
*
* @param registerTaskDefinitionRequest
* A {@link Consumer} that will call methods on {@link RegisterTaskDefinitionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the RegisterTaskDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.RegisterTaskDefinition
* @see AWS
* API Documentation
*/
default CompletableFuture registerTaskDefinition(
Consumer registerTaskDefinitionRequest) {
return registerTaskDefinition(RegisterTaskDefinitionRequest.builder().applyMutation(registerTaskDefinitionRequest)
.build());
}
/**
*
* Starts a new task using the specified task definition.
*
*
* You can allow Amazon ECS to place tasks for you, or you can customize how Amazon ECS places tasks using placement
* constraints and placement strategies. For more information, see Scheduling Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* Alternatively, you can use StartTask to use your own scheduler or place tasks manually on specific
* container instances.
*
*
* The Amazon ECS API follows an eventual consistency model. This is because the distributed nature of the system
* supporting the API. This means that the result of an API command you run that affects your Amazon ECS resources
* might not be immediately visible to all subsequent commands you run. Keep this in mind when you carry out an API
* command that immediately follows a previous API command.
*
*
* To manage eventual consistency, you can do the following:
*
*
* -
*
* Confirm the state of the resource before you run a command to modify it. Run the DescribeTasks command using an
* exponential backoff algorithm to ensure that you allow enough time for the previous command to propagate through
* the system. To do this, run the DescribeTasks command repeatedly, starting with a couple of seconds of wait time
* and increasing gradually up to five minutes of wait time.
*
*
* -
*
* Add wait time between subsequent commands, even if the DescribeTasks command returns an accurate response. Apply
* an exponential backoff algorithm starting with a couple of seconds of wait time, and increase gradually up to
* about five minutes of wait time.
*
*
*
*
* @param runTaskRequest
* @return A Java Future containing the result of the RunTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - BlockedException Your Amazon Web Services account was blocked. For more information, contact Amazon Web Services Support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.RunTask
* @see AWS API
* Documentation
*/
default CompletableFuture runTask(RunTaskRequest runTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a new task using the specified task definition.
*
*
* You can allow Amazon ECS to place tasks for you, or you can customize how Amazon ECS places tasks using placement
* constraints and placement strategies. For more information, see Scheduling Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* Alternatively, you can use StartTask to use your own scheduler or place tasks manually on specific
* container instances.
*
*
* The Amazon ECS API follows an eventual consistency model. This is because the distributed nature of the system
* supporting the API. This means that the result of an API command you run that affects your Amazon ECS resources
* might not be immediately visible to all subsequent commands you run. Keep this in mind when you carry out an API
* command that immediately follows a previous API command.
*
*
* To manage eventual consistency, you can do the following:
*
*
* -
*
* Confirm the state of the resource before you run a command to modify it. Run the DescribeTasks command using an
* exponential backoff algorithm to ensure that you allow enough time for the previous command to propagate through
* the system. To do this, run the DescribeTasks command repeatedly, starting with a couple of seconds of wait time
* and increasing gradually up to five minutes of wait time.
*
*
* -
*
* Add wait time between subsequent commands, even if the DescribeTasks command returns an accurate response. Apply
* an exponential backoff algorithm starting with a couple of seconds of wait time, and increase gradually up to
* about five minutes of wait time.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link RunTaskRequest.Builder} avoiding the need to create
* one manually via {@link RunTaskRequest#builder()}
*
*
* @param runTaskRequest
* A {@link Consumer} that will call methods on {@link RunTaskRequest.Builder} to create a request.
* @return A Java Future containing the result of the RunTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - BlockedException Your Amazon Web Services account was blocked. For more information, contact Amazon Web Services Support.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.RunTask
* @see AWS API
* Documentation
*/
default CompletableFuture runTask(Consumer runTaskRequest) {
return runTask(RunTaskRequest.builder().applyMutation(runTaskRequest).build());
}
/**
*
* Starts a new task from the specified task definition on the specified container instance or instances.
*
*
* Alternatively, you can use RunTask to place tasks for you. For more information, see Scheduling Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param startTaskRequest
* @return A Java Future containing the result of the StartTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.StartTask
* @see AWS API
* Documentation
*/
default CompletableFuture startTask(StartTaskRequest startTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a new task from the specified task definition on the specified container instance or instances.
*
*
* Alternatively, you can use RunTask to place tasks for you. For more information, see Scheduling Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link StartTaskRequest.Builder} avoiding the need to
* create one manually via {@link StartTaskRequest#builder()}
*
*
* @param startTaskRequest
* A {@link Consumer} that will call methods on {@link StartTaskRequest.Builder} to create a request.
* @return A Java Future containing the result of the StartTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.StartTask
* @see AWS API
* Documentation
*/
default CompletableFuture startTask(Consumer startTaskRequest) {
return startTask(StartTaskRequest.builder().applyMutation(startTaskRequest).build());
}
/**
*
* Stops a running task. Any tags associated with the task will be deleted.
*
*
* When StopTask is called on a task, the equivalent of docker stop
is issued to the containers
* running in the task. This results in a SIGTERM
value and a default 30-second timeout, after which
* the SIGKILL
value is sent and the containers are forcibly stopped. If the container handles the
* SIGTERM
value gracefully and exits within 30 seconds from receiving it, no SIGKILL
* value is sent.
*
*
*
* The default 30-second timeout can be configured on the Amazon ECS container agent with the
* ECS_CONTAINER_STOP_TIMEOUT
variable. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @param stopTaskRequest
* @return A Java Future containing the result of the StopTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.StopTask
* @see AWS API
* Documentation
*/
default CompletableFuture stopTask(StopTaskRequest stopTaskRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops a running task. Any tags associated with the task will be deleted.
*
*
* When StopTask is called on a task, the equivalent of docker stop
is issued to the containers
* running in the task. This results in a SIGTERM
value and a default 30-second timeout, after which
* the SIGKILL
value is sent and the containers are forcibly stopped. If the container handles the
* SIGTERM
value gracefully and exits within 30 seconds from receiving it, no SIGKILL
* value is sent.
*
*
*
* The default 30-second timeout can be configured on the Amazon ECS container agent with the
* ECS_CONTAINER_STOP_TIMEOUT
variable. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link StopTaskRequest.Builder} avoiding the need to
* create one manually via {@link StopTaskRequest#builder()}
*
*
* @param stopTaskRequest
* A {@link Consumer} that will call methods on {@link StopTaskRequest.Builder} to create a request.
* @return A Java Future containing the result of the StopTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.StopTask
* @see AWS API
* Documentation
*/
default CompletableFuture stopTask(Consumer stopTaskRequest) {
return stopTask(StopTaskRequest.builder().applyMutation(stopTaskRequest).build());
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that an attachment changed states.
*
*
* @param submitAttachmentStateChangesRequest
* @return A Java Future containing the result of the SubmitAttachmentStateChanges operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.SubmitAttachmentStateChanges
* @see AWS API Documentation
*/
default CompletableFuture submitAttachmentStateChanges(
SubmitAttachmentStateChangesRequest submitAttachmentStateChangesRequest) {
throw new UnsupportedOperationException();
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that an attachment changed states.
*
*
*
* This is a convenience which creates an instance of the {@link SubmitAttachmentStateChangesRequest.Builder}
* avoiding the need to create one manually via {@link SubmitAttachmentStateChangesRequest#builder()}
*
*
* @param submitAttachmentStateChangesRequest
* A {@link Consumer} that will call methods on {@link SubmitAttachmentStateChangesRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the SubmitAttachmentStateChanges operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.SubmitAttachmentStateChanges
* @see AWS API Documentation
*/
default CompletableFuture submitAttachmentStateChanges(
Consumer submitAttachmentStateChangesRequest) {
return submitAttachmentStateChanges(SubmitAttachmentStateChangesRequest.builder()
.applyMutation(submitAttachmentStateChangesRequest).build());
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a container changed states.
*
*
* @param submitContainerStateChangeRequest
* @return A Java Future containing the result of the SubmitContainerStateChange operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.SubmitContainerStateChange
* @see AWS API Documentation
*/
default CompletableFuture submitContainerStateChange(
SubmitContainerStateChangeRequest submitContainerStateChangeRequest) {
throw new UnsupportedOperationException();
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a container changed states.
*
*
*
* This is a convenience which creates an instance of the {@link SubmitContainerStateChangeRequest.Builder} avoiding
* the need to create one manually via {@link SubmitContainerStateChangeRequest#builder()}
*
*
* @param submitContainerStateChangeRequest
* A {@link Consumer} that will call methods on {@link SubmitContainerStateChangeRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SubmitContainerStateChange operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.SubmitContainerStateChange
* @see AWS API Documentation
*/
default CompletableFuture submitContainerStateChange(
Consumer submitContainerStateChangeRequest) {
return submitContainerStateChange(SubmitContainerStateChangeRequest.builder()
.applyMutation(submitContainerStateChangeRequest).build());
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a task changed states.
*
*
* @param submitTaskStateChangeRequest
* @return A Java Future containing the result of the SubmitTaskStateChange operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.SubmitTaskStateChange
* @see AWS API
* Documentation
*/
default CompletableFuture submitTaskStateChange(
SubmitTaskStateChangeRequest submitTaskStateChangeRequest) {
throw new UnsupportedOperationException();
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a task changed states.
*
*
*
* This is a convenience which creates an instance of the {@link SubmitTaskStateChangeRequest.Builder} avoiding the
* need to create one manually via {@link SubmitTaskStateChangeRequest#builder()}
*
*
* @param submitTaskStateChangeRequest
* A {@link Consumer} that will call methods on {@link SubmitTaskStateChangeRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the SubmitTaskStateChange operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.SubmitTaskStateChange
* @see AWS API
* Documentation
*/
default CompletableFuture submitTaskStateChange(
Consumer submitTaskStateChangeRequest) {
return submitTaskStateChange(SubmitTaskStateChangeRequest.builder().applyMutation(submitTaskStateChangeRequest).build());
}
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource aren't specified in the request parameters, they aren't changed. When a resource is deleted, the tags
* that are associated with that resource are deleted as well.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ResourceNotFoundException The specified resource wasn't found.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource aren't specified in the request parameters, they aren't changed. When a resource is deleted, the tags
* that are associated with that resource are deleted as well.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ResourceNotFoundException The specified resource wasn't found.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ResourceNotFoundException The specified resource wasn't found.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes specified tags from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ResourceNotFoundException The specified resource wasn't found.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Modifies the parameters for a capacity provider.
*
*
* @param updateCapacityProviderRequest
* @return A Java Future containing the result of the UpdateCapacityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateCapacityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture updateCapacityProvider(
UpdateCapacityProviderRequest updateCapacityProviderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the parameters for a capacity provider.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateCapacityProviderRequest.Builder} avoiding the
* need to create one manually via {@link UpdateCapacityProviderRequest#builder()}
*
*
* @param updateCapacityProviderRequest
* A {@link Consumer} that will call methods on {@link UpdateCapacityProviderRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateCapacityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateCapacityProvider
* @see AWS
* API Documentation
*/
default CompletableFuture updateCapacityProvider(
Consumer updateCapacityProviderRequest) {
return updateCapacityProvider(UpdateCapacityProviderRequest.builder().applyMutation(updateCapacityProviderRequest)
.build());
}
/**
*
* Updates the cluster.
*
*
* @param updateClusterRequest
* @return A Java Future containing the result of the UpdateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateCluster
* @see AWS API
* Documentation
*/
default CompletableFuture updateCluster(UpdateClusterRequest updateClusterRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the cluster.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateClusterRequest.Builder} avoiding the need to
* create one manually via {@link UpdateClusterRequest#builder()}
*
*
* @param updateClusterRequest
* A {@link Consumer} that will call methods on {@link UpdateClusterRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateCluster
* @see AWS API
* Documentation
*/
default CompletableFuture updateCluster(Consumer updateClusterRequest) {
return updateCluster(UpdateClusterRequest.builder().applyMutation(updateClusterRequest).build());
}
/**
*
* Modifies the settings to use for a cluster.
*
*
* @param updateClusterSettingsRequest
* @return A Java Future containing the result of the UpdateClusterSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateClusterSettings
* @see AWS API
* Documentation
*/
default CompletableFuture updateClusterSettings(
UpdateClusterSettingsRequest updateClusterSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the settings to use for a cluster.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateClusterSettingsRequest.Builder} avoiding the
* need to create one manually via {@link UpdateClusterSettingsRequest#builder()}
*
*
* @param updateClusterSettingsRequest
* A {@link Consumer} that will call methods on {@link UpdateClusterSettingsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateClusterSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateClusterSettings
* @see AWS API
* Documentation
*/
default CompletableFuture updateClusterSettings(
Consumer updateClusterSettingsRequest) {
return updateClusterSettings(UpdateClusterSettingsRequest.builder().applyMutation(updateClusterSettingsRequest).build());
}
/**
*
* Updates the Amazon ECS container agent on a specified container instance. Updating the Amazon ECS container agent
* doesn't interrupt running tasks or services on the container instance. The process for updating the agent differs
* depending on whether your container instance was launched with the Amazon ECS-optimized AMI or another operating
* system.
*
*
*
* The UpdateContainerAgent
API isn't supported for container instances using the Amazon ECS-optimized
* Amazon Linux 2 (arm64) AMI. To update the container agent, you can update the ecs-init
package. This
* updates the agent. For more information, see Updating the Amazon
* ECS container agent in the Amazon Elastic Container Service Developer Guide.
*
*
*
* The UpdateContainerAgent
API requires an Amazon ECS-optimized AMI or Amazon Linux AMI with the
* ecs-init
service installed and running. For help updating the Amazon ECS container agent on other
* operating systems, see Manually updating the Amazon ECS container agent in the Amazon Elastic Container Service Developer
* Guide.
*
*
* @param updateContainerAgentRequest
* @return A Java Future containing the result of the UpdateContainerAgent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - NoUpdateAvailableException There's no update available for this Amazon ECS container agent. This
* might be because the agent is already running the latest version or because it's so old that there's no
* update path to the current version.
* - MissingVersionException Amazon ECS can't determine the current version of the Amazon ECS container
* agent on the container instance and doesn't have enough information to proceed with an update. This could
* be because the agent running on the container instance is a previous or custom version that doesn't use
* our version information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateContainerAgent
* @see AWS API
* Documentation
*/
default CompletableFuture updateContainerAgent(
UpdateContainerAgentRequest updateContainerAgentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the Amazon ECS container agent on a specified container instance. Updating the Amazon ECS container agent
* doesn't interrupt running tasks or services on the container instance. The process for updating the agent differs
* depending on whether your container instance was launched with the Amazon ECS-optimized AMI or another operating
* system.
*
*
*
* The UpdateContainerAgent
API isn't supported for container instances using the Amazon ECS-optimized
* Amazon Linux 2 (arm64) AMI. To update the container agent, you can update the ecs-init
package. This
* updates the agent. For more information, see Updating the Amazon
* ECS container agent in the Amazon Elastic Container Service Developer Guide.
*
*
*
* The UpdateContainerAgent
API requires an Amazon ECS-optimized AMI or Amazon Linux AMI with the
* ecs-init
service installed and running. For help updating the Amazon ECS container agent on other
* operating systems, see Manually updating the Amazon ECS container agent in the Amazon Elastic Container Service Developer
* Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateContainerAgentRequest.Builder} avoiding the
* need to create one manually via {@link UpdateContainerAgentRequest#builder()}
*
*
* @param updateContainerAgentRequest
* A {@link Consumer} that will call methods on {@link UpdateContainerAgentRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateContainerAgent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - NoUpdateAvailableException There's no update available for this Amazon ECS container agent. This
* might be because the agent is already running the latest version or because it's so old that there's no
* update path to the current version.
* - MissingVersionException Amazon ECS can't determine the current version of the Amazon ECS container
* agent on the container instance and doesn't have enough information to proceed with an update. This could
* be because the agent running on the container instance is a previous or custom version that doesn't use
* our version information.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateContainerAgent
* @see AWS API
* Documentation
*/
default CompletableFuture updateContainerAgent(
Consumer updateContainerAgentRequest) {
return updateContainerAgent(UpdateContainerAgentRequest.builder().applyMutation(updateContainerAgentRequest).build());
}
/**
*
* Modifies the status of an Amazon ECS container instance.
*
*
* Once a container instance has reached an ACTIVE
state, you can change the status of a container
* instance to DRAINING
to manually remove an instance from a cluster, for example to perform system
* updates, update the Docker daemon, or scale down the cluster size.
*
*
*
* A container instance can't be changed to DRAINING
until it has reached an ACTIVE
* status. If the instance is in any other status, an error will be received.
*
*
*
* When you set a container instance to DRAINING
, Amazon ECS prevents new tasks from being scheduled
* for placement on the container instance and replacement service tasks are started on other container instances in
* the cluster if the resources are available. Service tasks on the container instance that are in the
* PENDING
state are stopped immediately.
*
*
* Service tasks on the container instance that are in the RUNNING
state are stopped and replaced
* according to the service's deployment configuration parameters, minimumHealthyPercent
and
* maximumPercent
. You can change the deployment configuration of your service using
* UpdateService.
*
*
* -
*
* If minimumHealthyPercent
is below 100%, the scheduler can ignore desiredCount
* temporarily during task replacement. For example, desiredCount
is four tasks, a minimum of 50%
* allows the scheduler to stop two existing tasks before starting two new tasks. If the minimum is 100%, the
* service scheduler can't remove existing tasks until the replacement tasks are considered healthy. Tasks for
* services that do not use a load balancer are considered healthy if they're in the RUNNING
state.
* Tasks for services that use a load balancer are considered healthy if they're in the RUNNING
state
* and the container instance they're hosted on is reported as healthy by the load balancer.
*
*
* -
*
* The maximumPercent
parameter represents an upper limit on the number of running tasks during task
* replacement. You can use this to define the replacement batch size. For example, if desiredCount
is
* four tasks, a maximum of 200% starts four new tasks before stopping the four tasks to be drained, provided that
* the cluster resources required to do this are available. If the maximum is 100%, then replacement tasks can't
* start until the draining tasks have stopped.
*
*
*
*
* Any PENDING
or RUNNING
tasks that do not belong to a service aren't affected. You must
* wait for them to finish or stop them manually.
*
*
* A container instance has completed draining when it has no more RUNNING
tasks. You can verify this
* using ListTasks.
*
*
* When a container instance has been drained, you can set a container instance to ACTIVE
status and
* once it has reached that status the Amazon ECS scheduler can begin scheduling tasks on the instance again.
*
*
* @param updateContainerInstancesStateRequest
* @return A Java Future containing the result of the UpdateContainerInstancesState operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateContainerInstancesState
* @see AWS API Documentation
*/
default CompletableFuture updateContainerInstancesState(
UpdateContainerInstancesStateRequest updateContainerInstancesStateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the status of an Amazon ECS container instance.
*
*
* Once a container instance has reached an ACTIVE
state, you can change the status of a container
* instance to DRAINING
to manually remove an instance from a cluster, for example to perform system
* updates, update the Docker daemon, or scale down the cluster size.
*
*
*
* A container instance can't be changed to DRAINING
until it has reached an ACTIVE
* status. If the instance is in any other status, an error will be received.
*
*
*
* When you set a container instance to DRAINING
, Amazon ECS prevents new tasks from being scheduled
* for placement on the container instance and replacement service tasks are started on other container instances in
* the cluster if the resources are available. Service tasks on the container instance that are in the
* PENDING
state are stopped immediately.
*
*
* Service tasks on the container instance that are in the RUNNING
state are stopped and replaced
* according to the service's deployment configuration parameters, minimumHealthyPercent
and
* maximumPercent
. You can change the deployment configuration of your service using
* UpdateService.
*
*
* -
*
* If minimumHealthyPercent
is below 100%, the scheduler can ignore desiredCount
* temporarily during task replacement. For example, desiredCount
is four tasks, a minimum of 50%
* allows the scheduler to stop two existing tasks before starting two new tasks. If the minimum is 100%, the
* service scheduler can't remove existing tasks until the replacement tasks are considered healthy. Tasks for
* services that do not use a load balancer are considered healthy if they're in the RUNNING
state.
* Tasks for services that use a load balancer are considered healthy if they're in the RUNNING
state
* and the container instance they're hosted on is reported as healthy by the load balancer.
*
*
* -
*
* The maximumPercent
parameter represents an upper limit on the number of running tasks during task
* replacement. You can use this to define the replacement batch size. For example, if desiredCount
is
* four tasks, a maximum of 200% starts four new tasks before stopping the four tasks to be drained, provided that
* the cluster resources required to do this are available. If the maximum is 100%, then replacement tasks can't
* start until the draining tasks have stopped.
*
*
*
*
* Any PENDING
or RUNNING
tasks that do not belong to a service aren't affected. You must
* wait for them to finish or stop them manually.
*
*
* A container instance has completed draining when it has no more RUNNING
tasks. You can verify this
* using ListTasks.
*
*
* When a container instance has been drained, you can set a container instance to ACTIVE
status and
* once it has reached that status the Amazon ECS scheduler can begin scheduling tasks on the instance again.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateContainerInstancesStateRequest.Builder}
* avoiding the need to create one manually via {@link UpdateContainerInstancesStateRequest#builder()}
*
*
* @param updateContainerInstancesStateRequest
* A {@link Consumer} that will call methods on {@link UpdateContainerInstancesStateRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateContainerInstancesState operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateContainerInstancesState
* @see AWS API Documentation
*/
default CompletableFuture updateContainerInstancesState(
Consumer updateContainerInstancesStateRequest) {
return updateContainerInstancesState(UpdateContainerInstancesStateRequest.builder()
.applyMutation(updateContainerInstancesStateRequest).build());
}
/**
*
*
* Updating the task placement strategies and constraints on an Amazon ECS service remains in preview and is a Beta
* Service as defined by and subject to the Beta Service Participation Service Terms located at https://aws.amazon.com/service-terms ("Beta Terms"). These Beta
* Terms apply to your participation in this preview.
*
*
*
* Modifies the parameters of a service.
*
*
* For services using the rolling update (ECS
) deployment controller, the desired count, deployment
* configuration, network configuration, task placement constraints and strategies, or task definition used can be
* updated.
*
*
* For services using the blue/green (CODE_DEPLOY
) deployment controller, only the desired count,
* deployment configuration, task placement constraints and strategies, and health check grace period can be updated
* using this API. If the network configuration, platform version, or task definition need to be updated, a new
* CodeDeploy deployment is created. For more information, see CreateDeployment
* in the CodeDeploy API Reference.
*
*
* For services using an external deployment controller, you can update only the desired count, task placement
* constraints and strategies, and health check grace period using this API. If the launch type, load balancer,
* network configuration, platform version, or task definition need to be updated, create a new task set. For more
* information, see CreateTaskSet.
*
*
* You can add to or subtract from the number of instantiations of a task definition in a service by specifying the
* cluster that the service is running in and a new desiredCount
parameter.
*
*
* If you have updated the Docker image of your application, you can create a new task definition with that image
* and deploy it to your service. The service scheduler uses the minimum healthy percent and maximum percent
* parameters (in the service's deployment configuration) to determine the deployment strategy.
*
*
*
* If your updated Docker image uses the same tag as what is in the existing task definition for your service (for
* example, my_image:latest
), you don't need to create a new revision of your task definition. You can
* update the service using the forceNewDeployment
option. The new tasks launched by the deployment
* pull the current image/tag combination from your repository when they start.
*
*
*
* You can also update the deployment configuration of a service. When a deployment is triggered by updating the
* task definition of a service, the service scheduler uses the deployment configuration parameters,
* minimumHealthyPercent
and maximumPercent
, to determine the deployment strategy.
*
*
* -
*
* If minimumHealthyPercent
is below 100%, the scheduler can ignore desiredCount
* temporarily during a deployment. For example, if desiredCount
is four tasks, a minimum of 50% allows
* the scheduler to stop two existing tasks before starting two new tasks. Tasks for services that don't use a load
* balancer are considered healthy if they're in the RUNNING
state. Tasks for services that use a load
* balancer are considered healthy if they're in the RUNNING
state and the container instance they're
* hosted on is reported as healthy by the load balancer.
*
*
* -
*
* The maximumPercent
parameter represents an upper limit on the number of running tasks during a
* deployment. You can use it to define the deployment batch size. For example, if desiredCount
is four
* tasks, a maximum of 200% starts four new tasks before stopping the four older tasks (provided that the cluster
* resources required to do this are available).
*
*
*
*
* When UpdateService stops a task during a deployment, the equivalent of docker stop
is issued
* to the containers running in the task. This results in a SIGTERM
and a 30-second timeout. After
* this, SIGKILL
is sent and the containers are forcibly stopped. If the container handles the
* SIGTERM
gracefully and exits within 30 seconds from receiving it, no SIGKILL
is sent.
*
*
* When the service scheduler launches new tasks, it determines task placement in your cluster with the following
* logic.
*
*
* -
*
* Determine which of the container instances in your cluster can support your service's task definition. For
* example, they have the required CPU, memory, ports, and container instance attributes.
*
*
* -
*
* By default, the service scheduler attempts to balance tasks across Availability Zones in this manner even though
* you can choose a different placement strategy.
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks for this service in the same
* Availability Zone as the instance. For example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal Availability Zone (based on the previous
* steps), favoring container instances with the fewest number of running tasks for this service.
*
*
*
*
*
*
* When the service scheduler stops running tasks, it attempts to maintain balance across the Availability Zones in
* your cluster using the following logic:
*
*
* -
*
* Sort the container instances by the largest number of running tasks for this service in the same Availability
* Zone as the instance. For example, if zone A has one running service task and zones B and C each have two,
* container instances in either zone B or C are considered optimal for termination.
*
*
* -
*
* Stop the task on a container instance in an optimal Availability Zone (based on the previous steps), favoring
* container instances with the largest number of running tasks for this service.
*
*
*
*
* @param updateServiceRequest
* @return A Java Future containing the result of the UpdateService operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateService
* @see AWS API
* Documentation
*/
default CompletableFuture updateService(UpdateServiceRequest updateServiceRequest) {
throw new UnsupportedOperationException();
}
/**
*
*
* Updating the task placement strategies and constraints on an Amazon ECS service remains in preview and is a Beta
* Service as defined by and subject to the Beta Service Participation Service Terms located at https://aws.amazon.com/service-terms ("Beta Terms"). These Beta
* Terms apply to your participation in this preview.
*
*
*
* Modifies the parameters of a service.
*
*
* For services using the rolling update (ECS
) deployment controller, the desired count, deployment
* configuration, network configuration, task placement constraints and strategies, or task definition used can be
* updated.
*
*
* For services using the blue/green (CODE_DEPLOY
) deployment controller, only the desired count,
* deployment configuration, task placement constraints and strategies, and health check grace period can be updated
* using this API. If the network configuration, platform version, or task definition need to be updated, a new
* CodeDeploy deployment is created. For more information, see CreateDeployment
* in the CodeDeploy API Reference.
*
*
* For services using an external deployment controller, you can update only the desired count, task placement
* constraints and strategies, and health check grace period using this API. If the launch type, load balancer,
* network configuration, platform version, or task definition need to be updated, create a new task set. For more
* information, see CreateTaskSet.
*
*
* You can add to or subtract from the number of instantiations of a task definition in a service by specifying the
* cluster that the service is running in and a new desiredCount
parameter.
*
*
* If you have updated the Docker image of your application, you can create a new task definition with that image
* and deploy it to your service. The service scheduler uses the minimum healthy percent and maximum percent
* parameters (in the service's deployment configuration) to determine the deployment strategy.
*
*
*
* If your updated Docker image uses the same tag as what is in the existing task definition for your service (for
* example, my_image:latest
), you don't need to create a new revision of your task definition. You can
* update the service using the forceNewDeployment
option. The new tasks launched by the deployment
* pull the current image/tag combination from your repository when they start.
*
*
*
* You can also update the deployment configuration of a service. When a deployment is triggered by updating the
* task definition of a service, the service scheduler uses the deployment configuration parameters,
* minimumHealthyPercent
and maximumPercent
, to determine the deployment strategy.
*
*
* -
*
* If minimumHealthyPercent
is below 100%, the scheduler can ignore desiredCount
* temporarily during a deployment. For example, if desiredCount
is four tasks, a minimum of 50% allows
* the scheduler to stop two existing tasks before starting two new tasks. Tasks for services that don't use a load
* balancer are considered healthy if they're in the RUNNING
state. Tasks for services that use a load
* balancer are considered healthy if they're in the RUNNING
state and the container instance they're
* hosted on is reported as healthy by the load balancer.
*
*
* -
*
* The maximumPercent
parameter represents an upper limit on the number of running tasks during a
* deployment. You can use it to define the deployment batch size. For example, if desiredCount
is four
* tasks, a maximum of 200% starts four new tasks before stopping the four older tasks (provided that the cluster
* resources required to do this are available).
*
*
*
*
* When UpdateService stops a task during a deployment, the equivalent of docker stop
is issued
* to the containers running in the task. This results in a SIGTERM
and a 30-second timeout. After
* this, SIGKILL
is sent and the containers are forcibly stopped. If the container handles the
* SIGTERM
gracefully and exits within 30 seconds from receiving it, no SIGKILL
is sent.
*
*
* When the service scheduler launches new tasks, it determines task placement in your cluster with the following
* logic.
*
*
* -
*
* Determine which of the container instances in your cluster can support your service's task definition. For
* example, they have the required CPU, memory, ports, and container instance attributes.
*
*
* -
*
* By default, the service scheduler attempts to balance tasks across Availability Zones in this manner even though
* you can choose a different placement strategy.
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks for this service in the same
* Availability Zone as the instance. For example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal Availability Zone (based on the previous
* steps), favoring container instances with the fewest number of running tasks for this service.
*
*
*
*
*
*
* When the service scheduler stops running tasks, it attempts to maintain balance across the Availability Zones in
* your cluster using the following logic:
*
*
* -
*
* Sort the container instances by the largest number of running tasks for this service in the same Availability
* Zone as the instance. For example, if zone A has one running service task and zones B and C each have two,
* container instances in either zone B or C are considered optimal for termination.
*
*
* -
*
* Stop the task on a container instance in an optimal Availability Zone (based on the previous steps), favoring
* container instances with the largest number of running tasks for this service.
*
*
*
*
*
* This is a convenience which creates an instance of the {@link UpdateServiceRequest.Builder} avoiding the need to
* create one manually via {@link UpdateServiceRequest#builder()}
*
*
* @param updateServiceRequest
* A {@link Consumer} that will call methods on {@link UpdateServiceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateService operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateService
* @see AWS API
* Documentation
*/
default CompletableFuture updateService(Consumer updateServiceRequest) {
return updateService(UpdateServiceRequest.builder().applyMutation(updateServiceRequest).build());
}
/**
*
* Modifies which task set in a service is the primary task set. Any parameters that are updated on the primary task
* set in a service will transition to the service. This is used when a service uses the EXTERNAL
* deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param updateServicePrimaryTaskSetRequest
* @return A Java Future containing the result of the UpdateServicePrimaryTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - TaskSetNotFoundException The specified task set wasn't found. You can view your available task sets
* with DescribeTaskSets. Task sets are specific to each cluster, service and Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateServicePrimaryTaskSet
* @see AWS API Documentation
*/
default CompletableFuture updateServicePrimaryTaskSet(
UpdateServicePrimaryTaskSetRequest updateServicePrimaryTaskSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies which task set in a service is the primary task set. Any parameters that are updated on the primary task
* set in a service will transition to the service. This is used when a service uses the EXTERNAL
* deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateServicePrimaryTaskSetRequest.Builder}
* avoiding the need to create one manually via {@link UpdateServicePrimaryTaskSetRequest#builder()}
*
*
* @param updateServicePrimaryTaskSetRequest
* A {@link Consumer} that will call methods on {@link UpdateServicePrimaryTaskSetRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the UpdateServicePrimaryTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - TaskSetNotFoundException The specified task set wasn't found. You can view your available task sets
* with DescribeTaskSets. Task sets are specific to each cluster, service and Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateServicePrimaryTaskSet
* @see AWS API Documentation
*/
default CompletableFuture updateServicePrimaryTaskSet(
Consumer updateServicePrimaryTaskSetRequest) {
return updateServicePrimaryTaskSet(UpdateServicePrimaryTaskSetRequest.builder()
.applyMutation(updateServicePrimaryTaskSetRequest).build());
}
/**
*
* Modifies a task set. This is used when a service uses the EXTERNAL
deployment controller type. For
* more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param updateTaskSetRequest
* @return A Java Future containing the result of the UpdateTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - TaskSetNotFoundException The specified task set wasn't found. You can view your available task sets
* with DescribeTaskSets. Task sets are specific to each cluster, service and Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateTaskSet
* @see AWS API
* Documentation
*/
default CompletableFuture updateTaskSet(UpdateTaskSetRequest updateTaskSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Modifies a task set. This is used when a service uses the EXTERNAL
deployment controller type. For
* more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateTaskSetRequest.Builder} avoiding the need to
* create one manually via {@link UpdateTaskSetRequest#builder()}
*
*
* @param updateTaskSetRequest
* A {@link Consumer} that will call methods on {@link UpdateTaskSetRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource,.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - TaskSetNotFoundException The specified task set wasn't found. You can view your available task sets
* with DescribeTaskSets. Task sets are specific to each cluster, service and Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.UpdateTaskSet
* @see AWS API
* Documentation
*/
default CompletableFuture updateTaskSet(Consumer updateTaskSetRequest) {
return updateTaskSet(UpdateTaskSetRequest.builder().applyMutation(updateTaskSetRequest).build());
}
/**
* Create an instance of {@link EcsAsyncWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link EcsAsyncWaiter}
*/
default EcsAsyncWaiter waiter() {
throw new UnsupportedOperationException();
}
}