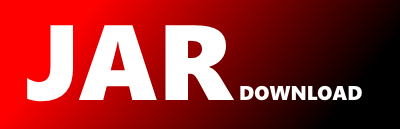
software.amazon.awssdk.services.ecs.model.KernelCapabilities Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The Linux capabilities for the container that are added to or dropped from the default configuration provided by
* Docker. For more information about the default capabilities and the non-default available capabilities, see Runtime privilege and
* Linux capabilities in the Docker run reference. For more detailed information about these Linux
* capabilities, see the capabilities(7) Linux
* manual page.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class KernelCapabilities implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> ADD_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("add")
.getter(getter(KernelCapabilities::add))
.setter(setter(Builder::add))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("add").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DROP_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("drop")
.getter(getter(KernelCapabilities::drop))
.setter(setter(Builder::drop))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("drop").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ADD_FIELD, DROP_FIELD));
private static final long serialVersionUID = 1L;
private final List add;
private final List drop;
private KernelCapabilities(BuilderImpl builder) {
this.add = builder.add;
this.drop = builder.drop;
}
/**
* For responses, this returns true if the service returned a value for the Add property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasAdd() {
return add != null && !(add instanceof SdkAutoConstructList);
}
/**
*
* The Linux capabilities for the container that have been added to the default configuration provided by Docker.
* This parameter maps to CapAdd
in the Create a container section of the
* Docker Remote API and the --cap-add
option
* to docker run.
*
*
*
* Tasks launched on Fargate only support adding the SYS_PTRACE
kernel capability.
*
*
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAdd} method.
*
*
* @return The Linux capabilities for the container that have been added to the default configuration provided by
* Docker. This parameter maps to CapAdd
in the Create a container section
* of the Docker Remote API and the
* --cap-add
option to docker run.
*
* Tasks launched on Fargate only support adding the SYS_PTRACE
kernel capability.
*
*
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
*/
public final List add() {
return add;
}
/**
* For responses, this returns true if the service returned a value for the Drop property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasDrop() {
return drop != null && !(drop instanceof SdkAutoConstructList);
}
/**
*
* The Linux capabilities for the container that have been removed from the default configuration provided by
* Docker. This parameter maps to CapDrop
in the Create a container section of the
* Docker Remote API and the --cap-drop
option
* to docker run.
*
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDrop} method.
*
*
* @return The Linux capabilities for the container that have been removed from the default configuration provided
* by Docker. This parameter maps to CapDrop
in the Create a container section
* of the Docker Remote API and the
* --cap-drop
option to docker run.
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
*/
public final List drop() {
return drop;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasAdd() ? add() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDrop() ? drop() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof KernelCapabilities)) {
return false;
}
KernelCapabilities other = (KernelCapabilities) obj;
return hasAdd() == other.hasAdd() && Objects.equals(add(), other.add()) && hasDrop() == other.hasDrop()
&& Objects.equals(drop(), other.drop());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("KernelCapabilities").add("Add", hasAdd() ? add() : null).add("Drop", hasDrop() ? drop() : null)
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "add":
return Optional.ofNullable(clazz.cast(add()));
case "drop":
return Optional.ofNullable(clazz.cast(drop()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Tasks launched on Fargate only support adding the SYS_PTRACE
kernel capability.
*
*
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder add(Collection add);
/**
*
* The Linux capabilities for the container that have been added to the default configuration provided by
* Docker. This parameter maps to CapAdd
in the Create a container section of
* the Docker Remote API and the --cap-add
* option to docker run.
*
*
*
* Tasks launched on Fargate only support adding the SYS_PTRACE
kernel capability.
*
*
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
*
*
* @param add
* The Linux capabilities for the container that have been added to the default configuration provided by
* Docker. This parameter maps to CapAdd
in the Create a container
* section of the Docker Remote API and the
* --cap-add
option to docker run.
*
* Tasks launched on Fargate only support adding the SYS_PTRACE
kernel capability.
*
*
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder add(String... add);
/**
*
* The Linux capabilities for the container that have been removed from the default configuration provided by
* Docker. This parameter maps to CapDrop
in the Create a container section of
* the Docker Remote API and the --cap-drop
* option to docker run.
*
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
*
*
* @param drop
* The Linux capabilities for the container that have been removed from the default configuration
* provided by Docker. This parameter maps to CapDrop
in the Create a container
* section of the Docker Remote API and the
* --cap-drop
option to docker run.
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder drop(Collection drop);
/**
*
* The Linux capabilities for the container that have been removed from the default configuration provided by
* Docker. This parameter maps to CapDrop
in the Create a container section of
* the Docker Remote API and the --cap-drop
* option to docker run.
*
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
*
*
* @param drop
* The Linux capabilities for the container that have been removed from the default configuration
* provided by Docker. This parameter maps to CapDrop
in the Create a container
* section of the Docker Remote API and the
* --cap-drop
option to docker run.
*
* Valid values:
* "ALL" | "AUDIT_CONTROL" | "AUDIT_WRITE" | "BLOCK_SUSPEND" | "CHOWN" | "DAC_OVERRIDE" | "DAC_READ_SEARCH" | "FOWNER" | "FSETID" | "IPC_LOCK" | "IPC_OWNER" | "KILL" | "LEASE" | "LINUX_IMMUTABLE" | "MAC_ADMIN" | "MAC_OVERRIDE" | "MKNOD" | "NET_ADMIN" | "NET_BIND_SERVICE" | "NET_BROADCAST" | "NET_RAW" | "SETFCAP" | "SETGID" | "SETPCAP" | "SETUID" | "SYS_ADMIN" | "SYS_BOOT" | "SYS_CHROOT" | "SYS_MODULE" | "SYS_NICE" | "SYS_PACCT" | "SYS_PTRACE" | "SYS_RAWIO" | "SYS_RESOURCE" | "SYS_TIME" | "SYS_TTY_CONFIG" | "SYSLOG" | "WAKE_ALARM"
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder drop(String... drop);
}
static final class BuilderImpl implements Builder {
private List add = DefaultSdkAutoConstructList.getInstance();
private List drop = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(KernelCapabilities model) {
add(model.add);
drop(model.drop);
}
public final Collection getAdd() {
if (add instanceof SdkAutoConstructList) {
return null;
}
return add;
}
public final void setAdd(Collection add) {
this.add = StringListCopier.copy(add);
}
@Override
@Transient
public final Builder add(Collection add) {
this.add = StringListCopier.copy(add);
return this;
}
@Override
@Transient
@SafeVarargs
public final Builder add(String... add) {
add(Arrays.asList(add));
return this;
}
public final Collection getDrop() {
if (drop instanceof SdkAutoConstructList) {
return null;
}
return drop;
}
public final void setDrop(Collection drop) {
this.drop = StringListCopier.copy(drop);
}
@Override
@Transient
public final Builder drop(Collection drop) {
this.drop = StringListCopier.copy(drop);
return this;
}
@Override
@Transient
@SafeVarargs
public final Builder drop(String... drop) {
drop(Arrays.asList(drop));
return this;
}
@Override
public KernelCapabilities build() {
return new KernelCapabilities(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}