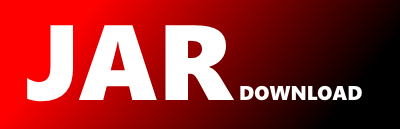
software.amazon.awssdk.services.ecs.model.ProxyConfiguration Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The configuration details for the App Mesh proxy.
*
*
* For tasks that use the EC2 launch type, the container instances require at least version 1.26.0 of the container
* agent and at least version 1.26.0-1 of the ecs-init
package to enable a proxy configuration. If your
* container instances are launched from the Amazon ECS optimized AMI version 20190301
or later, then they
* contain the required versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized Linux
* AMI
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProxyConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(ProxyConfiguration::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField CONTAINER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerName").getter(getter(ProxyConfiguration::containerName)).setter(setter(Builder::containerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerName").build()).build();
private static final SdkField> PROPERTIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("properties")
.getter(getter(ProxyConfiguration::properties))
.setter(setter(Builder::properties))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("properties").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(KeyValuePair::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD,
CONTAINER_NAME_FIELD, PROPERTIES_FIELD));
private static final long serialVersionUID = 1L;
private final String type;
private final String containerName;
private final List properties;
private ProxyConfiguration(BuilderImpl builder) {
this.type = builder.type;
this.containerName = builder.containerName;
this.properties = builder.properties;
}
/**
*
* The proxy type. The only supported value is APPMESH
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ProxyConfigurationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The proxy type. The only supported value is APPMESH
.
* @see ProxyConfigurationType
*/
public final ProxyConfigurationType type() {
return ProxyConfigurationType.fromValue(type);
}
/**
*
* The proxy type. The only supported value is APPMESH
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ProxyConfigurationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The proxy type. The only supported value is APPMESH
.
* @see ProxyConfigurationType
*/
public final String typeAsString() {
return type;
}
/**
*
* The name of the container that will serve as the App Mesh proxy.
*
*
* @return The name of the container that will serve as the App Mesh proxy.
*/
public final String containerName() {
return containerName;
}
/**
* For responses, this returns true if the service returned a value for the Properties property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasProperties() {
return properties != null && !(properties instanceof SdkAutoConstructList);
}
/**
*
* The set of network configuration parameters to provide the Container Network Interface (CNI) plugin, specified as
* key-value pairs.
*
*
* -
*
* IgnoredUID
- (Required) The user ID (UID) of the proxy container as defined by the user
* parameter in a container definition. This is used to ensure the proxy ignores its own traffic. If
* IgnoredGID
is specified, this field can be empty.
*
*
* -
*
* IgnoredGID
- (Required) The group ID (GID) of the proxy container as defined by the
* user
parameter in a container definition. This is used to ensure the proxy ignores its own traffic.
* If IgnoredUID
is specified, this field can be empty.
*
*
* -
*
* AppPorts
- (Required) The list of ports that the application uses. Network traffic to these ports is
* forwarded to the ProxyIngressPort
and ProxyEgressPort
.
*
*
* -
*
* ProxyIngressPort
- (Required) Specifies the port that incoming traffic to the AppPorts
* is directed to.
*
*
* -
*
* ProxyEgressPort
- (Required) Specifies the port that outgoing traffic from the AppPorts
* is directed to.
*
*
* -
*
* EgressIgnoredPorts
- (Required) The egress traffic going to the specified ports is ignored and not
* redirected to the ProxyEgressPort
. It can be an empty list.
*
*
* -
*
* EgressIgnoredIPs
- (Required) The egress traffic going to the specified IP addresses is ignored and
* not redirected to the ProxyEgressPort
. It can be an empty list.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasProperties} method.
*
*
* @return The set of network configuration parameters to provide the Container Network Interface (CNI) plugin,
* specified as key-value pairs.
*
* -
*
* IgnoredUID
- (Required) The user ID (UID) of the proxy container as defined by the
* user
parameter in a container definition. This is used to ensure the proxy ignores its own
* traffic. If IgnoredGID
is specified, this field can be empty.
*
*
* -
*
* IgnoredGID
- (Required) The group ID (GID) of the proxy container as defined by the
* user
parameter in a container definition. This is used to ensure the proxy ignores its own
* traffic. If IgnoredUID
is specified, this field can be empty.
*
*
* -
*
* AppPorts
- (Required) The list of ports that the application uses. Network traffic to these
* ports is forwarded to the ProxyIngressPort
and ProxyEgressPort
.
*
*
* -
*
* ProxyIngressPort
- (Required) Specifies the port that incoming traffic to the
* AppPorts
is directed to.
*
*
* -
*
* ProxyEgressPort
- (Required) Specifies the port that outgoing traffic from the
* AppPorts
is directed to.
*
*
* -
*
* EgressIgnoredPorts
- (Required) The egress traffic going to the specified ports is ignored
* and not redirected to the ProxyEgressPort
. It can be an empty list.
*
*
* -
*
* EgressIgnoredIPs
- (Required) The egress traffic going to the specified IP addresses is
* ignored and not redirected to the ProxyEgressPort
. It can be an empty list.
*
*
*/
public final List properties() {
return properties;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(containerName());
hashCode = 31 * hashCode + Objects.hashCode(hasProperties() ? properties() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProxyConfiguration)) {
return false;
}
ProxyConfiguration other = (ProxyConfiguration) obj;
return Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(containerName(), other.containerName())
&& hasProperties() == other.hasProperties() && Objects.equals(properties(), other.properties());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ProxyConfiguration").add("Type", typeAsString()).add("ContainerName", containerName())
.add("Properties", hasProperties() ? properties() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "containerName":
return Optional.ofNullable(clazz.cast(containerName()));
case "properties":
return Optional.ofNullable(clazz.cast(properties()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function