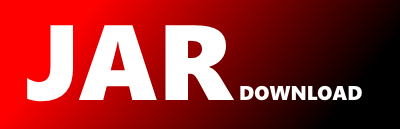
software.amazon.awssdk.services.ecs.model.ServiceRegistry Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details for the service registry.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServiceRegistry implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField REGISTRY_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("registryArn").getter(getter(ServiceRegistry::registryArn)).setter(setter(Builder::registryArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registryArn").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("port")
.getter(getter(ServiceRegistry::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("port").build()).build();
private static final SdkField CONTAINER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerName").getter(getter(ServiceRegistry::containerName)).setter(setter(Builder::containerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerName").build()).build();
private static final SdkField CONTAINER_PORT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("containerPort").getter(getter(ServiceRegistry::containerPort)).setter(setter(Builder::containerPort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerPort").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REGISTRY_ARN_FIELD,
PORT_FIELD, CONTAINER_NAME_FIELD, CONTAINER_PORT_FIELD));
private static final long serialVersionUID = 1L;
private final String registryArn;
private final Integer port;
private final String containerName;
private final Integer containerPort;
private ServiceRegistry(BuilderImpl builder) {
this.registryArn = builder.registryArn;
this.port = builder.port;
this.containerName = builder.containerName;
this.containerPort = builder.containerPort;
}
/**
*
* The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud Map.
* For more information, see CreateService.
*
*
* @return The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud
* Map. For more information, see CreateService.
*/
public final String registryArn() {
return registryArn;
}
/**
*
* The port value used if your service discovery service specified an SRV record. This field might be used if both
* the awsvpc
network mode and SRV records are used.
*
*
* @return The port value used if your service discovery service specified an SRV record. This field might be used
* if both the awsvpc
network mode and SRV records are used.
*/
public final Integer port() {
return port;
}
/**
*
* The container name value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and containerPort
* combination from the task definition. If the task definition that your service task specifies uses the
* awsvpc
network mode and a type SRV DNS record is used, you must specify either a
* containerName
and containerPort
combination or a port
value. However, you
* can't specify both.
*
*
* @return The container name value to be used for your service discovery service. It's already specified in the
* task definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and
* containerPort
combination from the task definition. If the task definition that your service
* task specifies uses the awsvpc
network mode and a type SRV DNS record is used, you must
* specify either a containerName
and containerPort
combination or a
* port
value. However, you can't specify both.
*/
public final String containerName() {
return containerName;
}
/**
*
* The port value to be used for your service discovery service. It's already specified in the task definition. If
* the task definition your service task specifies uses the bridge
or host
network mode,
* you must specify a containerName
and containerPort
combination from the task
* definition. If the task definition your service task specifies uses the awsvpc
network mode and a
* type SRV DNS record is used, you must specify either a containerName
and containerPort
* combination or a port
value. However, you can't specify both.
*
*
* @return The port value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and
* containerPort
combination from the task definition. If the task definition your service task
* specifies uses the awsvpc
network mode and a type SRV DNS record is used, you must specify
* either a containerName
and containerPort
combination or a port
* value. However, you can't specify both.
*/
public final Integer containerPort() {
return containerPort;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(registryArn());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(containerName());
hashCode = 31 * hashCode + Objects.hashCode(containerPort());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceRegistry)) {
return false;
}
ServiceRegistry other = (ServiceRegistry) obj;
return Objects.equals(registryArn(), other.registryArn()) && Objects.equals(port(), other.port())
&& Objects.equals(containerName(), other.containerName())
&& Objects.equals(containerPort(), other.containerPort());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServiceRegistry").add("RegistryArn", registryArn()).add("Port", port())
.add("ContainerName", containerName()).add("ContainerPort", containerPort()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "registryArn":
return Optional.ofNullable(clazz.cast(registryArn()));
case "port":
return Optional.ofNullable(clazz.cast(port()));
case "containerName":
return Optional.ofNullable(clazz.cast(containerName()));
case "containerPort":
return Optional.ofNullable(clazz.cast(containerPort()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function