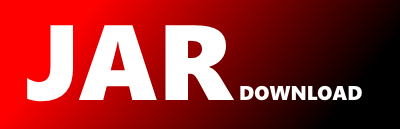
software.amazon.awssdk.services.ecs.model.Deployment Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of an Amazon ECS service deployment. This is used only when a service uses the ECS
* deployment controller type.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Deployment implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("id")
.getter(getter(Deployment::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(Deployment::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinition").getter(getter(Deployment::taskDefinition)).setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final SdkField DESIRED_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("desiredCount").getter(getter(Deployment::desiredCount)).setter(setter(Builder::desiredCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("desiredCount").build()).build();
private static final SdkField PENDING_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("pendingCount").getter(getter(Deployment::pendingCount)).setter(setter(Builder::pendingCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pendingCount").build()).build();
private static final SdkField RUNNING_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("runningCount").getter(getter(Deployment::runningCount)).setter(setter(Builder::runningCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runningCount").build()).build();
private static final SdkField FAILED_TASKS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("failedTasks").getter(getter(Deployment::failedTasks)).setter(setter(Builder::failedTasks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failedTasks").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdAt").getter(getter(Deployment::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField UPDATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updatedAt").getter(getter(Deployment::updatedAt)).setter(setter(Builder::updatedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updatedAt").build()).build();
private static final SdkField> CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviderStrategy")
.getter(getter(Deployment::capacityProviderStrategy))
.setter(setter(Builder::capacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("launchType").getter(getter(Deployment::launchTypeAsString)).setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformVersion").getter(getter(Deployment::platformVersion)).setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField PLATFORM_FAMILY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformFamily").getter(getter(Deployment::platformFamily)).setter(setter(Builder::platformFamily))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformFamily").build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkConfiguration")
.getter(getter(Deployment::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField ROLLOUT_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("rolloutState").getter(getter(Deployment::rolloutStateAsString)).setter(setter(Builder::rolloutState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rolloutState").build()).build();
private static final SdkField ROLLOUT_STATE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("rolloutStateReason").getter(getter(Deployment::rolloutStateReason))
.setter(setter(Builder::rolloutStateReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rolloutStateReason").build())
.build();
private static final SdkField SERVICE_CONNECT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("serviceConnectConfiguration")
.getter(getter(Deployment::serviceConnectConfiguration))
.setter(setter(Builder::serviceConnectConfiguration))
.constructor(ServiceConnectConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceConnectConfiguration")
.build()).build();
private static final SdkField> SERVICE_CONNECT_RESOURCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("serviceConnectResources")
.getter(getter(Deployment::serviceConnectResources))
.setter(setter(Builder::serviceConnectResources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceConnectResources").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceConnectServiceResource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, STATUS_FIELD,
TASK_DEFINITION_FIELD, DESIRED_COUNT_FIELD, PENDING_COUNT_FIELD, RUNNING_COUNT_FIELD, FAILED_TASKS_FIELD,
CREATED_AT_FIELD, UPDATED_AT_FIELD, CAPACITY_PROVIDER_STRATEGY_FIELD, LAUNCH_TYPE_FIELD, PLATFORM_VERSION_FIELD,
PLATFORM_FAMILY_FIELD, NETWORK_CONFIGURATION_FIELD, ROLLOUT_STATE_FIELD, ROLLOUT_STATE_REASON_FIELD,
SERVICE_CONNECT_CONFIGURATION_FIELD, SERVICE_CONNECT_RESOURCES_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String status;
private final String taskDefinition;
private final Integer desiredCount;
private final Integer pendingCount;
private final Integer runningCount;
private final Integer failedTasks;
private final Instant createdAt;
private final Instant updatedAt;
private final List capacityProviderStrategy;
private final String launchType;
private final String platformVersion;
private final String platformFamily;
private final NetworkConfiguration networkConfiguration;
private final String rolloutState;
private final String rolloutStateReason;
private final ServiceConnectConfiguration serviceConnectConfiguration;
private final List serviceConnectResources;
private Deployment(BuilderImpl builder) {
this.id = builder.id;
this.status = builder.status;
this.taskDefinition = builder.taskDefinition;
this.desiredCount = builder.desiredCount;
this.pendingCount = builder.pendingCount;
this.runningCount = builder.runningCount;
this.failedTasks = builder.failedTasks;
this.createdAt = builder.createdAt;
this.updatedAt = builder.updatedAt;
this.capacityProviderStrategy = builder.capacityProviderStrategy;
this.launchType = builder.launchType;
this.platformVersion = builder.platformVersion;
this.platformFamily = builder.platformFamily;
this.networkConfiguration = builder.networkConfiguration;
this.rolloutState = builder.rolloutState;
this.rolloutStateReason = builder.rolloutStateReason;
this.serviceConnectConfiguration = builder.serviceConnectConfiguration;
this.serviceConnectResources = builder.serviceConnectResources;
}
/**
*
* The ID of the deployment.
*
*
* @return The ID of the deployment.
*/
public final String id() {
return id;
}
/**
*
* The status of the deployment. The following describes each state.
*
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
*
*
* @return The status of the deployment. The following describes each state.
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
*/
public final String status() {
return status;
}
/**
*
* The most recent task definition that was specified for the tasks in the service to use.
*
*
* @return The most recent task definition that was specified for the tasks in the service to use.
*/
public final String taskDefinition() {
return taskDefinition;
}
/**
*
* The most recent desired count of tasks that was specified for the service to deploy or maintain.
*
*
* @return The most recent desired count of tasks that was specified for the service to deploy or maintain.
*/
public final Integer desiredCount() {
return desiredCount;
}
/**
*
* The number of tasks in the deployment that are in the PENDING
status.
*
*
* @return The number of tasks in the deployment that are in the PENDING
status.
*/
public final Integer pendingCount() {
return pendingCount;
}
/**
*
* The number of tasks in the deployment that are in the RUNNING
status.
*
*
* @return The number of tasks in the deployment that are in the RUNNING
status.
*/
public final Integer runningCount() {
return runningCount;
}
/**
*
* The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it fails any
* of its defined health checks and is stopped.
*
*
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to zero and
* stops being evaluated.
*
*
*
* @return The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it
* fails any of its defined health checks and is stopped.
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to
* zero and stops being evaluated.
*
*/
public final Integer failedTasks() {
return failedTasks;
}
/**
*
* The Unix timestamp for the time when the service deployment was created.
*
*
* @return The Unix timestamp for the time when the service deployment was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The Unix timestamp for the time when the service deployment was last updated.
*
*
* @return The Unix timestamp for the time when the service deployment was last updated.
*/
public final Instant updatedAt() {
return updatedAt;
}
/**
* For responses, this returns true if the service returned a value for the CapacityProviderStrategy property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCapacityProviderStrategy() {
return capacityProviderStrategy != null && !(capacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The capacity provider strategy that the deployment is using.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapacityProviderStrategy} method.
*
*
* @return The capacity provider strategy that the deployment is using.
*/
public final List capacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type the tasks in the service are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type the tasks in the service are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
/**
*
* The platform version that your tasks in the service run on. A platform version is only specified for tasks using
* the Fargate launch type. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version that your tasks in the service run on. A platform version is only specified for
* tasks using the Fargate launch type. If one isn't specified, the LATEST
platform version is
* used. For more information, see Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* The operating system that your tasks in the service, or tasks are running on. A platform family is specified only
* for tasks using the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service,
* for example, LINUX.
.
*
*
* @return The operating system that your tasks in the service, or tasks are running on. A platform family is
* specified only for tasks using the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service, for example, LINUX.
.
*/
public final String platformFamily() {
return platformFamily;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @return The VPC subnet and security group configuration for tasks that receive their own elastic network
* interface by using the awsvpc
networking mode.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
*
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned on, the
* deployment transitions to a FAILED
state. A deployment in FAILED
state doesn't launch
* any new tasks. For more information, see DeploymentCircuitBreaker.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rolloutState} will
* return {@link DeploymentRolloutState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #rolloutStateAsString}.
*
*
* @return
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned
* on, the deployment transitions to a FAILED
state. A deployment in FAILED
state
* doesn't launch any new tasks. For more information, see DeploymentCircuitBreaker.
* @see DeploymentRolloutState
*/
public final DeploymentRolloutState rolloutState() {
return DeploymentRolloutState.fromValue(rolloutState);
}
/**
*
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned on, the
* deployment transitions to a FAILED
state. A deployment in FAILED
state doesn't launch
* any new tasks. For more information, see DeploymentCircuitBreaker.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rolloutState} will
* return {@link DeploymentRolloutState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #rolloutStateAsString}.
*
*
* @return
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned
* on, the deployment transitions to a FAILED
state. A deployment in FAILED
state
* doesn't launch any new tasks. For more information, see DeploymentCircuitBreaker.
* @see DeploymentRolloutState
*/
public final String rolloutStateAsString() {
return rolloutState;
}
/**
*
* A description of the rollout state of a deployment.
*
*
* @return A description of the rollout state of a deployment.
*/
public final String rolloutStateReason() {
return rolloutStateReason;
}
/**
*
* The details of the Service Connect configuration that's used by this deployment. Compare the configuration
* between multiple deployments when troubleshooting issues with new deployments.
*
*
* The configuration for this service to discover and connect to services, and be discovered by, and connected from,
* other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The details of the Service Connect configuration that's used by this deployment. Compare the
* configuration between multiple deployments when troubleshooting issues with new deployments.
*
* The configuration for this service to discover and connect to services, and be discovered by, and
* connected from, other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
*/
public final ServiceConnectConfiguration serviceConnectConfiguration() {
return serviceConnectConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the ServiceConnectResources property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasServiceConnectResources() {
return serviceConnectResources != null && !(serviceConnectResources instanceof SdkAutoConstructList);
}
/**
*
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a discovery
* name to a Cloud Map service name.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasServiceConnectResources} method.
*
*
* @return The list of Service Connect resources that are associated with this deployment. Each list entry maps a
* discovery name to a Cloud Map service name.
*/
public final List serviceConnectResources() {
return serviceConnectResources;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
hashCode = 31 * hashCode + Objects.hashCode(desiredCount());
hashCode = 31 * hashCode + Objects.hashCode(pendingCount());
hashCode = 31 * hashCode + Objects.hashCode(runningCount());
hashCode = 31 * hashCode + Objects.hashCode(failedTasks());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(updatedAt());
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviderStrategy() ? capacityProviderStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(platformFamily());
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(rolloutStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(rolloutStateReason());
hashCode = 31 * hashCode + Objects.hashCode(serviceConnectConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasServiceConnectResources() ? serviceConnectResources() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Deployment)) {
return false;
}
Deployment other = (Deployment) obj;
return Objects.equals(id(), other.id()) && Objects.equals(status(), other.status())
&& Objects.equals(taskDefinition(), other.taskDefinition())
&& Objects.equals(desiredCount(), other.desiredCount()) && Objects.equals(pendingCount(), other.pendingCount())
&& Objects.equals(runningCount(), other.runningCount()) && Objects.equals(failedTasks(), other.failedTasks())
&& Objects.equals(createdAt(), other.createdAt()) && Objects.equals(updatedAt(), other.updatedAt())
&& hasCapacityProviderStrategy() == other.hasCapacityProviderStrategy()
&& Objects.equals(capacityProviderStrategy(), other.capacityProviderStrategy())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString())
&& Objects.equals(platformVersion(), other.platformVersion())
&& Objects.equals(platformFamily(), other.platformFamily())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& Objects.equals(rolloutStateAsString(), other.rolloutStateAsString())
&& Objects.equals(rolloutStateReason(), other.rolloutStateReason())
&& Objects.equals(serviceConnectConfiguration(), other.serviceConnectConfiguration())
&& hasServiceConnectResources() == other.hasServiceConnectResources()
&& Objects.equals(serviceConnectResources(), other.serviceConnectResources());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Deployment").add("Id", id()).add("Status", status()).add("TaskDefinition", taskDefinition())
.add("DesiredCount", desiredCount()).add("PendingCount", pendingCount()).add("RunningCount", runningCount())
.add("FailedTasks", failedTasks()).add("CreatedAt", createdAt()).add("UpdatedAt", updatedAt())
.add("CapacityProviderStrategy", hasCapacityProviderStrategy() ? capacityProviderStrategy() : null)
.add("LaunchType", launchTypeAsString()).add("PlatformVersion", platformVersion())
.add("PlatformFamily", platformFamily()).add("NetworkConfiguration", networkConfiguration())
.add("RolloutState", rolloutStateAsString()).add("RolloutStateReason", rolloutStateReason())
.add("ServiceConnectConfiguration", serviceConnectConfiguration())
.add("ServiceConnectResources", hasServiceConnectResources() ? serviceConnectResources() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "id":
return Optional.ofNullable(clazz.cast(id()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
case "desiredCount":
return Optional.ofNullable(clazz.cast(desiredCount()));
case "pendingCount":
return Optional.ofNullable(clazz.cast(pendingCount()));
case "runningCount":
return Optional.ofNullable(clazz.cast(runningCount()));
case "failedTasks":
return Optional.ofNullable(clazz.cast(failedTasks()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "updatedAt":
return Optional.ofNullable(clazz.cast(updatedAt()));
case "capacityProviderStrategy":
return Optional.ofNullable(clazz.cast(capacityProviderStrategy()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "platformFamily":
return Optional.ofNullable(clazz.cast(platformFamily()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "rolloutState":
return Optional.ofNullable(clazz.cast(rolloutStateAsString()));
case "rolloutStateReason":
return Optional.ofNullable(clazz.cast(rolloutStateReason()));
case "serviceConnectConfiguration":
return Optional.ofNullable(clazz.cast(serviceConnectConfiguration()));
case "serviceConnectResources":
return Optional.ofNullable(clazz.cast(serviceConnectResources()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function