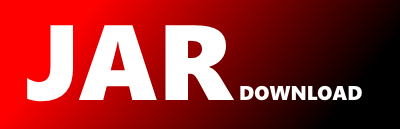
software.amazon.awssdk.services.ecs.model.DeploymentCircuitBreaker Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*
* The deployment circuit breaker can only be used for services using the rolling update (ECS
) deployment
* type.
*
*
*
* The deployment circuit breaker determines whether a service deployment will fail if the service can't reach a
* steady state. If it is turned on, a service deployment will transition to a failed state and stop launching new
* tasks. You can also configure Amazon ECS to roll back your service to the last completed deployment after a failure.
* For more information, see Rolling update in the
* Amazon Elastic Container Service Developer Guide.
*
*
* For more information about API failure reasons, see API failure reasons
* in the Amazon Elastic Container Service Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DeploymentCircuitBreaker implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ENABLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enable").getter(getter(DeploymentCircuitBreaker::enable)).setter(setter(Builder::enable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enable").build()).build();
private static final SdkField ROLLBACK_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("rollback").getter(getter(DeploymentCircuitBreaker::rollback)).setter(setter(Builder::rollback))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rollback").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENABLE_FIELD, ROLLBACK_FIELD));
private static final long serialVersionUID = 1L;
private final Boolean enable;
private final Boolean rollback;
private DeploymentCircuitBreaker(BuilderImpl builder) {
this.enable = builder.enable;
this.rollback = builder.rollback;
}
/**
*
* Determines whether to use the deployment circuit breaker logic for the service.
*
*
* @return Determines whether to use the deployment circuit breaker logic for the service.
*/
public final Boolean enable() {
return enable;
}
/**
*
* Determines whether to configure Amazon ECS to roll back the service if a service deployment fails. If rollback is
* on, when a service deployment fails, the service is rolled back to the last deployment that completed
* successfully.
*
*
* @return Determines whether to configure Amazon ECS to roll back the service if a service deployment fails. If
* rollback is on, when a service deployment fails, the service is rolled back to the last deployment that
* completed successfully.
*/
public final Boolean rollback() {
return rollback;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(enable());
hashCode = 31 * hashCode + Objects.hashCode(rollback());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DeploymentCircuitBreaker)) {
return false;
}
DeploymentCircuitBreaker other = (DeploymentCircuitBreaker) obj;
return Objects.equals(enable(), other.enable()) && Objects.equals(rollback(), other.rollback());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DeploymentCircuitBreaker").add("Enable", enable()).add("Rollback", rollback()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "enable":
return Optional.ofNullable(clazz.cast(enable()));
case "rollback":
return Optional.ofNullable(clazz.cast(rollback()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function