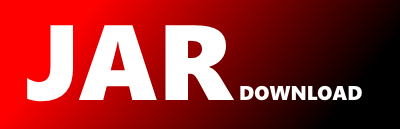
software.amazon.awssdk.services.ecs.model.EnvironmentFile Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A list of files containing the environment variables to pass to a container. You can specify up to ten environment
* files. The file must have a .env
file extension. Each line in an environment file should contain an
* environment variable in VARIABLE=VALUE
format. Lines beginning with #
are treated as
* comments and are ignored. For more information about the environment variable file syntax, see Declare default environment variables in file.
*
*
* If there are environment variables specified using the environment
parameter in a container definition,
* they take precedence over the variables contained within an environment file. If multiple environment files are
* specified that contain the same variable, they're processed from the top down. We recommend that you use unique
* variable names. For more information, see Specifying environment
* variables in the Amazon Elastic Container Service Developer Guide.
*
*
* You must use the following platforms for the Fargate launch type:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EnvironmentFile implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField VALUE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("value")
.getter(getter(EnvironmentFile::value)).setter(setter(Builder::value))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("value").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(EnvironmentFile::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VALUE_FIELD, TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String value;
private final String type;
private EnvironmentFile(BuilderImpl builder) {
this.value = builder.value;
this.type = builder.type;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon S3 object containing the environment variable file.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon S3 object containing the environment variable file.
*/
public final String value() {
return value;
}
/**
*
* The file type to use. The only supported value is s3
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link EnvironmentFileType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The file type to use. The only supported value is s3
.
* @see EnvironmentFileType
*/
public final EnvironmentFileType type() {
return EnvironmentFileType.fromValue(type);
}
/**
*
* The file type to use. The only supported value is s3
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link EnvironmentFileType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The file type to use. The only supported value is s3
.
* @see EnvironmentFileType
*/
public final String typeAsString() {
return type;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(value());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EnvironmentFile)) {
return false;
}
EnvironmentFile other = (EnvironmentFile) obj;
return Objects.equals(value(), other.value()) && Objects.equals(typeAsString(), other.typeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EnvironmentFile").add("Value", value()).add("Type", typeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "value":
return Optional.ofNullable(clazz.cast(value()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function