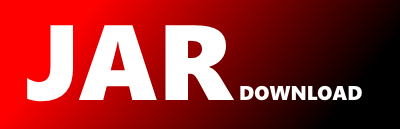
software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListContainerInstancesRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(ListContainerInstancesRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField FILTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("filter")
.getter(getter(ListContainerInstancesRequest::filter)).setter(setter(Builder::filter))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("filter").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("nextToken").getter(getter(ListContainerInstancesRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nextToken").build()).build();
private static final SdkField MAX_RESULTS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("maxResults").getter(getter(ListContainerInstancesRequest::maxResults))
.setter(setter(Builder::maxResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maxResults").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(ListContainerInstancesRequest::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD, FILTER_FIELD,
NEXT_TOKEN_FIELD, MAX_RESULTS_FIELD, STATUS_FIELD));
private final String cluster;
private final String filter;
private final String nextToken;
private final Integer maxResults;
private final String status;
private ListContainerInstancesRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.filter = builder.filter;
this.nextToken = builder.nextToken;
this.maxResults = builder.maxResults;
this.status = builder.status;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster that hosts the container instances to list. If
* you do not specify a cluster, the default cluster is assumed.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster that hosts the container instances to
* list. If you do not specify a cluster, the default cluster is assumed.
*/
public final String cluster() {
return cluster;
}
/**
*
* You can filter the results of a ListContainerInstances
operation with cluster query language
* statements. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
* @return You can filter the results of a ListContainerInstances
operation with cluster query language
* statements. For more information, see Cluster
* Query Language in the Amazon Elastic Container Service Developer Guide.
*/
public final String filter() {
return filter;
}
/**
*
* The nextToken
value returned from a ListContainerInstances
request indicating that more
* results are available to fulfill the request and further calls are needed. If maxResults
was
* provided, it's possible the number of results to be fewer than maxResults
.
*
*
*
* This token should be treated as an opaque identifier that is only used to retrieve the next items in a list and
* not for other programmatic purposes.
*
*
*
* @return The nextToken
value returned from a ListContainerInstances
request indicating
* that more results are available to fulfill the request and further calls are needed. If
* maxResults
was provided, it's possible the number of results to be fewer than
* maxResults
.
*
* This token should be treated as an opaque identifier that is only used to retrieve the next items in a
* list and not for other programmatic purposes.
*
*/
public final String nextToken() {
return nextToken;
}
/**
*
* The maximum number of container instance results that ListContainerInstances
returned in paginated
* output. When this parameter is used, ListContainerInstances
only returns maxResults
* results in a single page along with a nextToken
response element. The remaining results of the
* initial request can be seen by sending another ListContainerInstances
request with the returned
* nextToken
value. This value can be between 1 and 100. If this parameter isn't used, then
* ListContainerInstances
returns up to 100 results and a nextToken
value if applicable.
*
*
* @return The maximum number of container instance results that ListContainerInstances
returned in
* paginated output. When this parameter is used, ListContainerInstances
only returns
* maxResults
results in a single page along with a nextToken
response element.
* The remaining results of the initial request can be seen by sending another
* ListContainerInstances
request with the returned nextToken
value. This value
* can be between 1 and 100. If this parameter isn't used, then ListContainerInstances
returns
* up to 100 results and a nextToken
value if applicable.
*/
public final Integer maxResults() {
return maxResults;
}
/**
*
* Filters the container instances by status. For example, if you specify the DRAINING
status, the
* results include only container instances that have been set to DRAINING
using
* UpdateContainerInstancesState. If you don't specify this parameter, the default is to include container
* instances set to all states other than INACTIVE
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ContainerInstanceStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return Filters the container instances by status. For example, if you specify the DRAINING
status,
* the results include only container instances that have been set to DRAINING
using
* UpdateContainerInstancesState. If you don't specify this parameter, the default is to include
* container instances set to all states other than INACTIVE
.
* @see ContainerInstanceStatus
*/
public final ContainerInstanceStatus status() {
return ContainerInstanceStatus.fromValue(status);
}
/**
*
* Filters the container instances by status. For example, if you specify the DRAINING
status, the
* results include only container instances that have been set to DRAINING
using
* UpdateContainerInstancesState. If you don't specify this parameter, the default is to include container
* instances set to all states other than INACTIVE
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ContainerInstanceStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return Filters the container instances by status. For example, if you specify the DRAINING
status,
* the results include only container instances that have been set to DRAINING
using
* UpdateContainerInstancesState. If you don't specify this parameter, the default is to include
* container instances set to all states other than INACTIVE
.
* @see ContainerInstanceStatus
*/
public final String statusAsString() {
return status;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(filter());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(maxResults());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListContainerInstancesRequest)) {
return false;
}
ListContainerInstancesRequest other = (ListContainerInstancesRequest) obj;
return Objects.equals(cluster(), other.cluster()) && Objects.equals(filter(), other.filter())
&& Objects.equals(nextToken(), other.nextToken()) && Objects.equals(maxResults(), other.maxResults())
&& Objects.equals(statusAsString(), other.statusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListContainerInstancesRequest").add("Cluster", cluster()).add("Filter", filter())
.add("NextToken", nextToken()).add("MaxResults", maxResults()).add("Status", statusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "filter":
return Optional.ofNullable(clazz.cast(filter()));
case "nextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "maxResults":
return Optional.ofNullable(clazz.cast(maxResults()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function