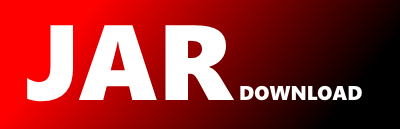
software.amazon.awssdk.services.ecs.model.PlacementStrategy Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The task placement strategy for a task or service. For more information, see Task placement
* strategies in the Amazon Elastic Container Service Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PlacementStrategy implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(PlacementStrategy::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField FIELD_FIELD = SdkField. builder(MarshallingType.STRING).memberName("field")
.getter(getter(PlacementStrategy::field)).setter(setter(Builder::field))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("field").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TYPE_FIELD, FIELD_FIELD));
private static final long serialVersionUID = 1L;
private final String type;
private final String field;
private PlacementStrategy(BuilderImpl builder) {
this.type = builder.type;
this.field = builder.field;
}
/**
*
* The type of placement strategy. The random
placement strategy randomly places tasks on available
* candidates. The spread
placement strategy spreads placement across available candidates evenly based
* on the field
parameter. The binpack
strategy places tasks on available candidates that
* have the least available amount of the resource that's specified with the field
parameter. For
* example, if you binpack on memory, a task is placed on the instance with the least amount of remaining memory but
* still enough to run the task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link PlacementStrategyType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of placement strategy. The random
placement strategy randomly places tasks on
* available candidates. The spread
placement strategy spreads placement across available
* candidates evenly based on the field
parameter. The binpack
strategy places
* tasks on available candidates that have the least available amount of the resource that's specified with
* the field
parameter. For example, if you binpack on memory, a task is placed on the instance
* with the least amount of remaining memory but still enough to run the task.
* @see PlacementStrategyType
*/
public final PlacementStrategyType type() {
return PlacementStrategyType.fromValue(type);
}
/**
*
* The type of placement strategy. The random
placement strategy randomly places tasks on available
* candidates. The spread
placement strategy spreads placement across available candidates evenly based
* on the field
parameter. The binpack
strategy places tasks on available candidates that
* have the least available amount of the resource that's specified with the field
parameter. For
* example, if you binpack on memory, a task is placed on the instance with the least amount of remaining memory but
* still enough to run the task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link PlacementStrategyType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of placement strategy. The random
placement strategy randomly places tasks on
* available candidates. The spread
placement strategy spreads placement across available
* candidates evenly based on the field
parameter. The binpack
strategy places
* tasks on available candidates that have the least available amount of the resource that's specified with
* the field
parameter. For example, if you binpack on memory, a task is placed on the instance
* with the least amount of remaining memory but still enough to run the task.
* @see PlacementStrategyType
*/
public final String typeAsString() {
return type;
}
/**
*
* The field to apply the placement strategy against. For the spread
placement strategy, valid values
* are instanceId
(or host
, which has the same effect), or any platform or custom
* attribute that's applied to a container instance, such as attribute:ecs.availability-zone
. For the
* binpack
placement strategy, valid values are cpu
and memory
. For the
* random
placement strategy, this field is not used.
*
*
* @return The field to apply the placement strategy against. For the spread
placement strategy, valid
* values are instanceId
(or host
, which has the same effect), or any platform or
* custom attribute that's applied to a container instance, such as
* attribute:ecs.availability-zone
. For the binpack
placement strategy, valid
* values are cpu
and memory
. For the random
placement strategy, this
* field is not used.
*/
public final String field() {
return field;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(field());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PlacementStrategy)) {
return false;
}
PlacementStrategy other = (PlacementStrategy) obj;
return Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(field(), other.field());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PlacementStrategy").add("Type", typeAsString()).add("Field", field()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "field":
return Optional.ofNullable(clazz.cast(field()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function