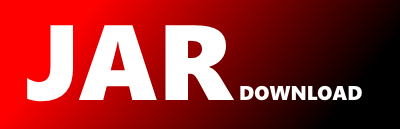
software.amazon.awssdk.services.ecs.model.Service Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details on a service within a cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Service implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SERVICE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceArn").getter(getter(Service::serviceArn)).setter(setter(Builder::serviceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceArn").build()).build();
private static final SdkField SERVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceName").getter(getter(Service::serviceName)).setter(setter(Builder::serviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceName").build()).build();
private static final SdkField CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clusterArn").getter(getter(Service::clusterArn)).setter(setter(Builder::clusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clusterArn").build()).build();
private static final SdkField> LOAD_BALANCERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("loadBalancers")
.getter(getter(Service::loadBalancers))
.setter(setter(Builder::loadBalancers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("loadBalancers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LoadBalancer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SERVICE_REGISTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("serviceRegistries")
.getter(getter(Service::serviceRegistries))
.setter(setter(Builder::serviceRegistries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRegistries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceRegistry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(Service::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField DESIRED_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("desiredCount").getter(getter(Service::desiredCount)).setter(setter(Builder::desiredCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("desiredCount").build()).build();
private static final SdkField RUNNING_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("runningCount").getter(getter(Service::runningCount)).setter(setter(Builder::runningCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runningCount").build()).build();
private static final SdkField PENDING_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("pendingCount").getter(getter(Service::pendingCount)).setter(setter(Builder::pendingCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pendingCount").build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("launchType").getter(getter(Service::launchTypeAsString)).setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final SdkField> CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviderStrategy")
.getter(getter(Service::capacityProviderStrategy))
.setter(setter(Builder::capacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformVersion").getter(getter(Service::platformVersion)).setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField PLATFORM_FAMILY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformFamily").getter(getter(Service::platformFamily)).setter(setter(Builder::platformFamily))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformFamily").build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinition").getter(getter(Service::taskDefinition)).setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final SdkField DEPLOYMENT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deploymentConfiguration")
.getter(getter(Service::deploymentConfiguration)).setter(setter(Builder::deploymentConfiguration))
.constructor(DeploymentConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentConfiguration").build())
.build();
private static final SdkField> TASK_SETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("taskSets")
.getter(getter(Service::taskSets))
.setter(setter(Builder::taskSets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskSets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TaskSet::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DEPLOYMENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("deployments")
.getter(getter(Service::deployments))
.setter(setter(Builder::deployments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deployments").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Deployment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("roleArn").getter(getter(Service::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("roleArn").build()).build();
private static final SdkField> EVENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("events")
.getter(getter(Service::events))
.setter(setter(Builder::events))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("events").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceEvent::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdAt").getter(getter(Service::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField> PLACEMENT_CONSTRAINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("placementConstraints")
.getter(getter(Service::placementConstraints))
.setter(setter(Builder::placementConstraints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementConstraints").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementConstraint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PLACEMENT_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("placementStrategy")
.getter(getter(Service::placementStrategy))
.setter(setter(Builder::placementStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementStrategy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkConfiguration")
.getter(getter(Service::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField HEALTH_CHECK_GRACE_PERIOD_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("healthCheckGracePeriodSeconds")
.getter(getter(Service::healthCheckGracePeriodSeconds))
.setter(setter(Builder::healthCheckGracePeriodSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("healthCheckGracePeriodSeconds")
.build()).build();
private static final SdkField SCHEDULING_STRATEGY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("schedulingStrategy").getter(getter(Service::schedulingStrategyAsString))
.setter(setter(Builder::schedulingStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("schedulingStrategy").build())
.build();
private static final SdkField DEPLOYMENT_CONTROLLER_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deploymentController")
.getter(getter(Service::deploymentController)).setter(setter(Builder::deploymentController))
.constructor(DeploymentController::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentController").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(Service::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("createdBy").getter(getter(Service::createdBy)).setter(setter(Builder::createdBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdBy").build()).build();
private static final SdkField ENABLE_ECS_MANAGED_TAGS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableECSManagedTags").getter(getter(Service::enableECSManagedTags))
.setter(setter(Builder::enableECSManagedTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableECSManagedTags").build())
.build();
private static final SdkField PROPAGATE_TAGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("propagateTags").getter(getter(Service::propagateTagsAsString)).setter(setter(Builder::propagateTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("propagateTags").build()).build();
private static final SdkField ENABLE_EXECUTE_COMMAND_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableExecuteCommand").getter(getter(Service::enableExecuteCommand))
.setter(setter(Builder::enableExecuteCommand))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableExecuteCommand").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SERVICE_ARN_FIELD,
SERVICE_NAME_FIELD, CLUSTER_ARN_FIELD, LOAD_BALANCERS_FIELD, SERVICE_REGISTRIES_FIELD, STATUS_FIELD,
DESIRED_COUNT_FIELD, RUNNING_COUNT_FIELD, PENDING_COUNT_FIELD, LAUNCH_TYPE_FIELD, CAPACITY_PROVIDER_STRATEGY_FIELD,
PLATFORM_VERSION_FIELD, PLATFORM_FAMILY_FIELD, TASK_DEFINITION_FIELD, DEPLOYMENT_CONFIGURATION_FIELD,
TASK_SETS_FIELD, DEPLOYMENTS_FIELD, ROLE_ARN_FIELD, EVENTS_FIELD, CREATED_AT_FIELD, PLACEMENT_CONSTRAINTS_FIELD,
PLACEMENT_STRATEGY_FIELD, NETWORK_CONFIGURATION_FIELD, HEALTH_CHECK_GRACE_PERIOD_SECONDS_FIELD,
SCHEDULING_STRATEGY_FIELD, DEPLOYMENT_CONTROLLER_FIELD, TAGS_FIELD, CREATED_BY_FIELD, ENABLE_ECS_MANAGED_TAGS_FIELD,
PROPAGATE_TAGS_FIELD, ENABLE_EXECUTE_COMMAND_FIELD));
private static final long serialVersionUID = 1L;
private final String serviceArn;
private final String serviceName;
private final String clusterArn;
private final List loadBalancers;
private final List serviceRegistries;
private final String status;
private final Integer desiredCount;
private final Integer runningCount;
private final Integer pendingCount;
private final String launchType;
private final List capacityProviderStrategy;
private final String platformVersion;
private final String platformFamily;
private final String taskDefinition;
private final DeploymentConfiguration deploymentConfiguration;
private final List taskSets;
private final List deployments;
private final String roleArn;
private final List events;
private final Instant createdAt;
private final List placementConstraints;
private final List placementStrategy;
private final NetworkConfiguration networkConfiguration;
private final Integer healthCheckGracePeriodSeconds;
private final String schedulingStrategy;
private final DeploymentController deploymentController;
private final List tags;
private final String createdBy;
private final Boolean enableECSManagedTags;
private final String propagateTags;
private final Boolean enableExecuteCommand;
private Service(BuilderImpl builder) {
this.serviceArn = builder.serviceArn;
this.serviceName = builder.serviceName;
this.clusterArn = builder.clusterArn;
this.loadBalancers = builder.loadBalancers;
this.serviceRegistries = builder.serviceRegistries;
this.status = builder.status;
this.desiredCount = builder.desiredCount;
this.runningCount = builder.runningCount;
this.pendingCount = builder.pendingCount;
this.launchType = builder.launchType;
this.capacityProviderStrategy = builder.capacityProviderStrategy;
this.platformVersion = builder.platformVersion;
this.platformFamily = builder.platformFamily;
this.taskDefinition = builder.taskDefinition;
this.deploymentConfiguration = builder.deploymentConfiguration;
this.taskSets = builder.taskSets;
this.deployments = builder.deployments;
this.roleArn = builder.roleArn;
this.events = builder.events;
this.createdAt = builder.createdAt;
this.placementConstraints = builder.placementConstraints;
this.placementStrategy = builder.placementStrategy;
this.networkConfiguration = builder.networkConfiguration;
this.healthCheckGracePeriodSeconds = builder.healthCheckGracePeriodSeconds;
this.schedulingStrategy = builder.schedulingStrategy;
this.deploymentController = builder.deploymentController;
this.tags = builder.tags;
this.createdBy = builder.createdBy;
this.enableECSManagedTags = builder.enableECSManagedTags;
this.propagateTags = builder.propagateTags;
this.enableExecuteCommand = builder.enableExecuteCommand;
}
/**
*
* The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @return The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*/
public final String serviceArn() {
return serviceArn;
}
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. Service names must be unique within a cluster. However, you can have similarly named services in
* multiple clusters within a Region or across multiple Regions.
*
*
* @return The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens
* are allowed. Service names must be unique within a cluster. However, you can have similarly named
* services in multiple clusters within a Region or across multiple Regions.
*/
public final String serviceName() {
return serviceName;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*
* @return The Amazon Resource Name (ARN) of the cluster that hosts the service.
*/
public final String clusterArn() {
return clusterArn;
}
/**
* For responses, this returns true if the service returned a value for the LoadBalancers property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLoadBalancers() {
return loadBalancers != null && !(loadBalancers instanceof SdkAutoConstructList);
}
/**
*
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container name,
* and the container port to access from the load balancer. The container name is as it appears in a container
* definition.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLoadBalancers} method.
*
*
* @return A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container
* name, and the container port to access from the load balancer. The container name is as it appears in a
* container definition.
*/
public final List loadBalancers() {
return loadBalancers;
}
/**
* For responses, this returns true if the service returned a value for the ServiceRegistries property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasServiceRegistries() {
return serviceRegistries != null && !(serviceRegistries instanceof SdkAutoConstructList);
}
/**
*
* The details for the service discovery registries to assign to this service. For more information, see Service Discovery.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasServiceRegistries} method.
*
*
* @return The details for the service discovery registries to assign to this service. For more information, see Service
* Discovery.
*/
public final List serviceRegistries() {
return serviceRegistries;
}
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*
* @return The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*/
public final String status() {
return status;
}
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*
* @return The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*/
public final Integer desiredCount() {
return desiredCount;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @return The number of tasks in the cluster that are in the RUNNING
state.
*/
public final Integer runningCount() {
return runningCount;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @return The number of tasks in the cluster that are in the PENDING
state.
*/
public final Integer pendingCount() {
return pendingCount;
}
/**
*
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the service
* was created using a capacity provider strategy.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type the service is using. When using the DescribeServices API, this field is omitted if the
* service was created using a capacity provider strategy.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the service
* was created using a capacity provider strategy.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type the service is using. When using the DescribeServices API, this field is omitted if the
* service was created using a capacity provider strategy.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
/**
* For responses, this returns true if the service returned a value for the CapacityProviderStrategy property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCapacityProviderStrategy() {
return capacityProviderStrategy != null && !(capacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is omitted if
* the service was created using a launch type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapacityProviderStrategy} method.
*
*
* @return The capacity provider strategy the service uses. When using the DescribeServices API, this field is
* omitted if the service was created using a launch type.
*/
public final List capacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* The platform version to run your service on. A platform version is only specified for tasks that are hosted on
* Fargate. If one isn't specified, the LATEST
platform version is used. For more information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version to run your service on. A platform version is only specified for tasks that are
* hosted on Fargate. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* The operating system that your tasks in the service run on. A platform family is specified only for tasks using
* the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service
* (for example, LINUX
).
*
*
* @return The operating system that your tasks in the service run on. A platform family is specified only for tasks
* using the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service (for example, LINUX
).
*/
public final String platformFamily() {
return platformFamily;
}
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*
* @return The task definition to use for tasks in the service. This value is specified when the service is created
* with CreateService, and it can be modified with UpdateService.
*/
public final String taskDefinition() {
return taskDefinition;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @return Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
*/
public final DeploymentConfiguration deploymentConfiguration() {
return deploymentConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the TaskSets property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasTaskSets() {
return taskSets != null && !(taskSets instanceof SdkAutoConstructList);
}
/**
*
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
deployment. An
* Amazon ECS task set includes details such as the desired number of tasks, how many tasks are running, and whether
* the task set serves production traffic.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTaskSets} method.
*
*
* @return Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
* deployment. An Amazon ECS task set includes details such as the desired number of tasks, how many tasks
* are running, and whether the task set serves production traffic.
*/
public final List taskSets() {
return taskSets;
}
/**
* For responses, this returns true if the service returned a value for the Deployments property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDeployments() {
return deployments != null && !(deployments instanceof SdkAutoConstructList);
}
/**
*
* The current state of deployments for the service.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDeployments} method.
*
*
* @return The current state of deployments for the service.
*/
public final List deployments() {
return deployments;
}
/**
*
* The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to register
* container instances with an Elastic Load Balancing load balancer.
*
*
* @return The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to
* register container instances with an Elastic Load Balancing load balancer.
*/
public final String roleArn() {
return roleArn;
}
/**
* For responses, this returns true if the service returned a value for the Events property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasEvents() {
return events != null && !(events instanceof SdkAutoConstructList);
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEvents} method.
*
*
* @return The event stream for your service. A maximum of 100 of the latest events are displayed.
*/
public final List events() {
return events;
}
/**
*
* The Unix timestamp for the time when the service was created.
*
*
* @return The Unix timestamp for the time when the service was created.
*/
public final Instant createdAt() {
return createdAt;
}
/**
* For responses, this returns true if the service returned a value for the PlacementConstraints property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPlacementConstraints() {
return placementConstraints != null && !(placementConstraints instanceof SdkAutoConstructList);
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPlacementConstraints} method.
*
*
* @return The placement constraints for the tasks in the service.
*/
public final List placementConstraints() {
return placementConstraints;
}
/**
* For responses, this returns true if the service returned a value for the PlacementStrategy property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPlacementStrategy() {
return placementStrategy != null && !(placementStrategy instanceof SdkAutoConstructList);
}
/**
*
* The placement strategy that determines how tasks for the service are placed.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPlacementStrategy} method.
*
*
* @return The placement strategy that determines how tasks for the service are placed.
*/
public final List placementStrategy() {
return placementStrategy;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @return The VPC subnet and security group configuration for tasks that receive their own elastic network
* interface by using the awsvpc
networking mode.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
*
* The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load Balancing
* target health checks after a task has first started.
*
*
* @return The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load
* Balancing target health checks after a task has first started.
*/
public final Integer healthCheckGracePeriodSeconds() {
return healthCheckGracePeriodSeconds;
}
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance.
* This task meets all of the task placement constraints that you specify in your cluster. The service scheduler
* also evaluates the task placement constraints for running tasks. It stop tasks that don't meet the placement
* constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #schedulingStrategy} will return {@link SchedulingStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #schedulingStrategyAsString}.
*
*
* @return The scheduling strategy to use for the service. For more information, see Services.
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks
* across your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can
* use task placement strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container
* instance. This task meets all of the task placement constraints that you specify in your cluster. The
* service scheduler also evaluates the task placement constraints for running tasks. It stop tasks that
* don't meet the placement constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
* @see SchedulingStrategy
*/
public final SchedulingStrategy schedulingStrategy() {
return SchedulingStrategy.fromValue(schedulingStrategy);
}
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance.
* This task meets all of the task placement constraints that you specify in your cluster. The service scheduler
* also evaluates the task placement constraints for running tasks. It stop tasks that don't meet the placement
* constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #schedulingStrategy} will return {@link SchedulingStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #schedulingStrategyAsString}.
*
*
* @return The scheduling strategy to use for the service. For more information, see Services.
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks
* across your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can
* use task placement strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container
* instance. This task meets all of the task placement constraints that you specify in your cluster. The
* service scheduler also evaluates the task placement constraints for running tasks. It stop tasks that
* don't meet the placement constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
* @see SchedulingStrategy
*/
public final String schedulingStrategyAsString() {
return schedulingStrategy;
}
/**
*
* The deployment controller type the service is using.
*
*
* @return The deployment controller type the service is using.
*/
public final DeploymentController deploymentController() {
return deploymentController;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of a key
* and an optional value. You define bot the key and value.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The metadata that you apply to the service to help you categorize and organize them. Each tag consists of
* a key and an optional value. You define bot the key and value.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete
* tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource
* limit.
*
*
*/
public final List tags() {
return tags;
}
/**
*
* The principal that created the service.
*
*
* @return The principal that created the service.
*/
public final String createdBy() {
return createdBy;
}
/**
*
* Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see
* Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public final Boolean enableECSManagedTags() {
return enableECSManagedTags;
}
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Determines whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
* @see PropagateTags
*/
public final PropagateTags propagateTags() {
return PropagateTags.fromValue(propagateTags);
}
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Determines whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
* @see PropagateTags
*/
public final String propagateTagsAsString() {
return propagateTags;
}
/**
*
* Determines whether the execute command functionality is turned on for the service. If true
, the
* execute command functionality is turned on for all containers in tasks as part of the service.
*
*
* @return Determines whether the execute command functionality is turned on for the service. If true
,
* the execute command functionality is turned on for all containers in tasks as part of the service.
*/
public final Boolean enableExecuteCommand() {
return enableExecuteCommand;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(serviceArn());
hashCode = 31 * hashCode + Objects.hashCode(serviceName());
hashCode = 31 * hashCode + Objects.hashCode(clusterArn());
hashCode = 31 * hashCode + Objects.hashCode(hasLoadBalancers() ? loadBalancers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasServiceRegistries() ? serviceRegistries() : null);
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(desiredCount());
hashCode = 31 * hashCode + Objects.hashCode(runningCount());
hashCode = 31 * hashCode + Objects.hashCode(pendingCount());
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviderStrategy() ? capacityProviderStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(platformFamily());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
hashCode = 31 * hashCode + Objects.hashCode(deploymentConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasTaskSets() ? taskSets() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDeployments() ? deployments() : null);
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(hasEvents() ? events() : null);
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementConstraints() ? placementConstraints() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementStrategy() ? placementStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckGracePeriodSeconds());
hashCode = 31 * hashCode + Objects.hashCode(schedulingStrategyAsString());
hashCode = 31 * hashCode + Objects.hashCode(deploymentController());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(createdBy());
hashCode = 31 * hashCode + Objects.hashCode(enableECSManagedTags());
hashCode = 31 * hashCode + Objects.hashCode(propagateTagsAsString());
hashCode = 31 * hashCode + Objects.hashCode(enableExecuteCommand());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Service)) {
return false;
}
Service other = (Service) obj;
return Objects.equals(serviceArn(), other.serviceArn()) && Objects.equals(serviceName(), other.serviceName())
&& Objects.equals(clusterArn(), other.clusterArn()) && hasLoadBalancers() == other.hasLoadBalancers()
&& Objects.equals(loadBalancers(), other.loadBalancers())
&& hasServiceRegistries() == other.hasServiceRegistries()
&& Objects.equals(serviceRegistries(), other.serviceRegistries()) && Objects.equals(status(), other.status())
&& Objects.equals(desiredCount(), other.desiredCount()) && Objects.equals(runningCount(), other.runningCount())
&& Objects.equals(pendingCount(), other.pendingCount())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString())
&& hasCapacityProviderStrategy() == other.hasCapacityProviderStrategy()
&& Objects.equals(capacityProviderStrategy(), other.capacityProviderStrategy())
&& Objects.equals(platformVersion(), other.platformVersion())
&& Objects.equals(platformFamily(), other.platformFamily())
&& Objects.equals(taskDefinition(), other.taskDefinition())
&& Objects.equals(deploymentConfiguration(), other.deploymentConfiguration())
&& hasTaskSets() == other.hasTaskSets() && Objects.equals(taskSets(), other.taskSets())
&& hasDeployments() == other.hasDeployments() && Objects.equals(deployments(), other.deployments())
&& Objects.equals(roleArn(), other.roleArn()) && hasEvents() == other.hasEvents()
&& Objects.equals(events(), other.events()) && Objects.equals(createdAt(), other.createdAt())
&& hasPlacementConstraints() == other.hasPlacementConstraints()
&& Objects.equals(placementConstraints(), other.placementConstraints())
&& hasPlacementStrategy() == other.hasPlacementStrategy()
&& Objects.equals(placementStrategy(), other.placementStrategy())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& Objects.equals(healthCheckGracePeriodSeconds(), other.healthCheckGracePeriodSeconds())
&& Objects.equals(schedulingStrategyAsString(), other.schedulingStrategyAsString())
&& Objects.equals(deploymentController(), other.deploymentController()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(createdBy(), other.createdBy())
&& Objects.equals(enableECSManagedTags(), other.enableECSManagedTags())
&& Objects.equals(propagateTagsAsString(), other.propagateTagsAsString())
&& Objects.equals(enableExecuteCommand(), other.enableExecuteCommand());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Service").add("ServiceArn", serviceArn()).add("ServiceName", serviceName())
.add("ClusterArn", clusterArn()).add("LoadBalancers", hasLoadBalancers() ? loadBalancers() : null)
.add("ServiceRegistries", hasServiceRegistries() ? serviceRegistries() : null).add("Status", status())
.add("DesiredCount", desiredCount()).add("RunningCount", runningCount()).add("PendingCount", pendingCount())
.add("LaunchType", launchTypeAsString())
.add("CapacityProviderStrategy", hasCapacityProviderStrategy() ? capacityProviderStrategy() : null)
.add("PlatformVersion", platformVersion()).add("PlatformFamily", platformFamily())
.add("TaskDefinition", taskDefinition()).add("DeploymentConfiguration", deploymentConfiguration())
.add("TaskSets", hasTaskSets() ? taskSets() : null).add("Deployments", hasDeployments() ? deployments() : null)
.add("RoleArn", roleArn()).add("Events", hasEvents() ? events() : null).add("CreatedAt", createdAt())
.add("PlacementConstraints", hasPlacementConstraints() ? placementConstraints() : null)
.add("PlacementStrategy", hasPlacementStrategy() ? placementStrategy() : null)
.add("NetworkConfiguration", networkConfiguration())
.add("HealthCheckGracePeriodSeconds", healthCheckGracePeriodSeconds())
.add("SchedulingStrategy", schedulingStrategyAsString()).add("DeploymentController", deploymentController())
.add("Tags", hasTags() ? tags() : null).add("CreatedBy", createdBy())
.add("EnableECSManagedTags", enableECSManagedTags()).add("PropagateTags", propagateTagsAsString())
.add("EnableExecuteCommand", enableExecuteCommand()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "serviceArn":
return Optional.ofNullable(clazz.cast(serviceArn()));
case "serviceName":
return Optional.ofNullable(clazz.cast(serviceName()));
case "clusterArn":
return Optional.ofNullable(clazz.cast(clusterArn()));
case "loadBalancers":
return Optional.ofNullable(clazz.cast(loadBalancers()));
case "serviceRegistries":
return Optional.ofNullable(clazz.cast(serviceRegistries()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "desiredCount":
return Optional.ofNullable(clazz.cast(desiredCount()));
case "runningCount":
return Optional.ofNullable(clazz.cast(runningCount()));
case "pendingCount":
return Optional.ofNullable(clazz.cast(pendingCount()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "capacityProviderStrategy":
return Optional.ofNullable(clazz.cast(capacityProviderStrategy()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "platformFamily":
return Optional.ofNullable(clazz.cast(platformFamily()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
case "deploymentConfiguration":
return Optional.ofNullable(clazz.cast(deploymentConfiguration()));
case "taskSets":
return Optional.ofNullable(clazz.cast(taskSets()));
case "deployments":
return Optional.ofNullable(clazz.cast(deployments()));
case "roleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "events":
return Optional.ofNullable(clazz.cast(events()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "placementConstraints":
return Optional.ofNullable(clazz.cast(placementConstraints()));
case "placementStrategy":
return Optional.ofNullable(clazz.cast(placementStrategy()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "healthCheckGracePeriodSeconds":
return Optional.ofNullable(clazz.cast(healthCheckGracePeriodSeconds()));
case "schedulingStrategy":
return Optional.ofNullable(clazz.cast(schedulingStrategyAsString()));
case "deploymentController":
return Optional.ofNullable(clazz.cast(deploymentController()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "createdBy":
return Optional.ofNullable(clazz.cast(createdBy()));
case "enableECSManagedTags":
return Optional.ofNullable(clazz.cast(enableECSManagedTags()));
case "propagateTags":
return Optional.ofNullable(clazz.cast(propagateTagsAsString()));
case "enableExecuteCommand":
return Optional.ofNullable(clazz.cast(enableExecuteCommand()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function