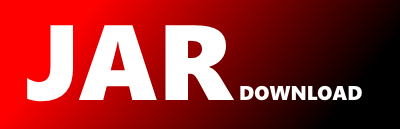
software.amazon.awssdk.services.ecs.model.Task Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Details on a task in a cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Task implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField> ATTACHMENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("attachments")
.getter(getter(Task::attachments))
.setter(setter(Builder::attachments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attachments").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Attachment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ATTRIBUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("attributes")
.getter(getter(Task::attributes))
.setter(setter(Builder::attributes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attributes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Attribute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("availabilityZone").getter(getter(Task::availabilityZone)).setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("availabilityZone").build()).build();
private static final SdkField CAPACITY_PROVIDER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("capacityProviderName").getter(getter(Task::capacityProviderName))
.setter(setter(Builder::capacityProviderName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderName").build())
.build();
private static final SdkField CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clusterArn").getter(getter(Task::clusterArn)).setter(setter(Builder::clusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clusterArn").build()).build();
private static final SdkField CONNECTIVITY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("connectivity").getter(getter(Task::connectivityAsString)).setter(setter(Builder::connectivity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("connectivity").build()).build();
private static final SdkField CONNECTIVITY_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("connectivityAt").getter(getter(Task::connectivityAt)).setter(setter(Builder::connectivityAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("connectivityAt").build()).build();
private static final SdkField CONTAINER_INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerInstanceArn").getter(getter(Task::containerInstanceArn))
.setter(setter(Builder::containerInstanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerInstanceArn").build())
.build();
private static final SdkField> CONTAINERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("containers")
.getter(getter(Task::containers))
.setter(setter(Builder::containers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Container::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CPU_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cpu")
.getter(getter(Task::cpu)).setter(setter(Builder::cpu))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cpu").build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdAt").getter(getter(Task::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField DESIRED_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("desiredStatus").getter(getter(Task::desiredStatus)).setter(setter(Builder::desiredStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("desiredStatus").build()).build();
private static final SdkField ENABLE_EXECUTE_COMMAND_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableExecuteCommand").getter(getter(Task::enableExecuteCommand))
.setter(setter(Builder::enableExecuteCommand))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableExecuteCommand").build())
.build();
private static final SdkField EXECUTION_STOPPED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("executionStoppedAt").getter(getter(Task::executionStoppedAt))
.setter(setter(Builder::executionStoppedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionStoppedAt").build())
.build();
private static final SdkField GROUP_FIELD = SdkField. builder(MarshallingType.STRING).memberName("group")
.getter(getter(Task::group)).setter(setter(Builder::group))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("group").build()).build();
private static final SdkField HEALTH_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("healthStatus").getter(getter(Task::healthStatusAsString)).setter(setter(Builder::healthStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("healthStatus").build()).build();
private static final SdkField> INFERENCE_ACCELERATORS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("inferenceAccelerators")
.getter(getter(Task::inferenceAccelerators))
.setter(setter(Builder::inferenceAccelerators))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inferenceAccelerators").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InferenceAccelerator::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LAST_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("lastStatus").getter(getter(Task::lastStatus)).setter(setter(Builder::lastStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastStatus").build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("launchType").getter(getter(Task::launchTypeAsString)).setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final SdkField MEMORY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("memory")
.getter(getter(Task::memory)).setter(setter(Builder::memory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memory").build()).build();
private static final SdkField OVERRIDES_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("overrides").getter(getter(Task::overrides)).setter(setter(Builder::overrides))
.constructor(TaskOverride::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("overrides").build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformVersion").getter(getter(Task::platformVersion)).setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField PLATFORM_FAMILY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformFamily").getter(getter(Task::platformFamily)).setter(setter(Builder::platformFamily))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformFamily").build()).build();
private static final SdkField PULL_STARTED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("pullStartedAt").getter(getter(Task::pullStartedAt)).setter(setter(Builder::pullStartedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pullStartedAt").build()).build();
private static final SdkField PULL_STOPPED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("pullStoppedAt").getter(getter(Task::pullStoppedAt)).setter(setter(Builder::pullStoppedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pullStoppedAt").build()).build();
private static final SdkField STARTED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("startedAt").getter(getter(Task::startedAt)).setter(setter(Builder::startedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startedAt").build()).build();
private static final SdkField STARTED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("startedBy").getter(getter(Task::startedBy)).setter(setter(Builder::startedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startedBy").build()).build();
private static final SdkField STOP_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("stopCode").getter(getter(Task::stopCodeAsString)).setter(setter(Builder::stopCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stopCode").build()).build();
private static final SdkField STOPPED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("stoppedAt").getter(getter(Task::stoppedAt)).setter(setter(Builder::stoppedAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stoppedAt").build()).build();
private static final SdkField STOPPED_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("stoppedReason").getter(getter(Task::stoppedReason)).setter(setter(Builder::stoppedReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stoppedReason").build()).build();
private static final SdkField STOPPING_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("stoppingAt").getter(getter(Task::stoppingAt)).setter(setter(Builder::stoppingAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("stoppingAt").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(Task::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TASK_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskArn").getter(getter(Task::taskArn)).setter(setter(Builder::taskArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskArn").build()).build();
private static final SdkField TASK_DEFINITION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinitionArn").getter(getter(Task::taskDefinitionArn)).setter(setter(Builder::taskDefinitionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinitionArn").build()).build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.LONG).memberName("version")
.getter(getter(Task::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("version").build()).build();
private static final SdkField EPHEMERAL_STORAGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ephemeralStorage")
.getter(getter(Task::ephemeralStorage)).setter(setter(Builder::ephemeralStorage))
.constructor(EphemeralStorage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ephemeralStorage").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ATTACHMENTS_FIELD,
ATTRIBUTES_FIELD, AVAILABILITY_ZONE_FIELD, CAPACITY_PROVIDER_NAME_FIELD, CLUSTER_ARN_FIELD, CONNECTIVITY_FIELD,
CONNECTIVITY_AT_FIELD, CONTAINER_INSTANCE_ARN_FIELD, CONTAINERS_FIELD, CPU_FIELD, CREATED_AT_FIELD,
DESIRED_STATUS_FIELD, ENABLE_EXECUTE_COMMAND_FIELD, EXECUTION_STOPPED_AT_FIELD, GROUP_FIELD, HEALTH_STATUS_FIELD,
INFERENCE_ACCELERATORS_FIELD, LAST_STATUS_FIELD, LAUNCH_TYPE_FIELD, MEMORY_FIELD, OVERRIDES_FIELD,
PLATFORM_VERSION_FIELD, PLATFORM_FAMILY_FIELD, PULL_STARTED_AT_FIELD, PULL_STOPPED_AT_FIELD, STARTED_AT_FIELD,
STARTED_BY_FIELD, STOP_CODE_FIELD, STOPPED_AT_FIELD, STOPPED_REASON_FIELD, STOPPING_AT_FIELD, TAGS_FIELD,
TASK_ARN_FIELD, TASK_DEFINITION_ARN_FIELD, VERSION_FIELD, EPHEMERAL_STORAGE_FIELD));
private static final long serialVersionUID = 1L;
private final List attachments;
private final List attributes;
private final String availabilityZone;
private final String capacityProviderName;
private final String clusterArn;
private final String connectivity;
private final Instant connectivityAt;
private final String containerInstanceArn;
private final List containers;
private final String cpu;
private final Instant createdAt;
private final String desiredStatus;
private final Boolean enableExecuteCommand;
private final Instant executionStoppedAt;
private final String group;
private final String healthStatus;
private final List inferenceAccelerators;
private final String lastStatus;
private final String launchType;
private final String memory;
private final TaskOverride overrides;
private final String platformVersion;
private final String platformFamily;
private final Instant pullStartedAt;
private final Instant pullStoppedAt;
private final Instant startedAt;
private final String startedBy;
private final String stopCode;
private final Instant stoppedAt;
private final String stoppedReason;
private final Instant stoppingAt;
private final List tags;
private final String taskArn;
private final String taskDefinitionArn;
private final Long version;
private final EphemeralStorage ephemeralStorage;
private Task(BuilderImpl builder) {
this.attachments = builder.attachments;
this.attributes = builder.attributes;
this.availabilityZone = builder.availabilityZone;
this.capacityProviderName = builder.capacityProviderName;
this.clusterArn = builder.clusterArn;
this.connectivity = builder.connectivity;
this.connectivityAt = builder.connectivityAt;
this.containerInstanceArn = builder.containerInstanceArn;
this.containers = builder.containers;
this.cpu = builder.cpu;
this.createdAt = builder.createdAt;
this.desiredStatus = builder.desiredStatus;
this.enableExecuteCommand = builder.enableExecuteCommand;
this.executionStoppedAt = builder.executionStoppedAt;
this.group = builder.group;
this.healthStatus = builder.healthStatus;
this.inferenceAccelerators = builder.inferenceAccelerators;
this.lastStatus = builder.lastStatus;
this.launchType = builder.launchType;
this.memory = builder.memory;
this.overrides = builder.overrides;
this.platformVersion = builder.platformVersion;
this.platformFamily = builder.platformFamily;
this.pullStartedAt = builder.pullStartedAt;
this.pullStoppedAt = builder.pullStoppedAt;
this.startedAt = builder.startedAt;
this.startedBy = builder.startedBy;
this.stopCode = builder.stopCode;
this.stoppedAt = builder.stoppedAt;
this.stoppedReason = builder.stoppedReason;
this.stoppingAt = builder.stoppingAt;
this.tags = builder.tags;
this.taskArn = builder.taskArn;
this.taskDefinitionArn = builder.taskDefinitionArn;
this.version = builder.version;
this.ephemeralStorage = builder.ephemeralStorage;
}
/**
* For responses, this returns true if the service returned a value for the Attachments property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAttachments() {
return attachments != null && !(attachments instanceof SdkAutoConstructList);
}
/**
*
* The Elastic Network Adapter that's associated with the task if the task uses the awsvpc
network
* mode.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttachments} method.
*
*
* @return The Elastic Network Adapter that's associated with the task if the task uses the awsvpc
* network mode.
*/
public final List attachments() {
return attachments;
}
/**
* For responses, this returns true if the service returned a value for the Attributes property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAttributes() {
return attributes != null && !(attributes instanceof SdkAutoConstructList);
}
/**
*
* The attributes of the task
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttributes} method.
*
*
* @return The attributes of the task
*/
public final List attributes() {
return attributes;
}
/**
*
* The Availability Zone for the task.
*
*
* @return The Availability Zone for the task.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
*
* The capacity provider that's associated with the task.
*
*
* @return The capacity provider that's associated with the task.
*/
public final String capacityProviderName() {
return capacityProviderName;
}
/**
*
* The ARN of the cluster that hosts the task.
*
*
* @return The ARN of the cluster that hosts the task.
*/
public final String clusterArn() {
return clusterArn;
}
/**
*
* The connectivity status of a task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #connectivity} will
* return {@link Connectivity#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #connectivityAsString}.
*
*
* @return The connectivity status of a task.
* @see Connectivity
*/
public final Connectivity connectivity() {
return Connectivity.fromValue(connectivity);
}
/**
*
* The connectivity status of a task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #connectivity} will
* return {@link Connectivity#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #connectivityAsString}.
*
*
* @return The connectivity status of a task.
* @see Connectivity
*/
public final String connectivityAsString() {
return connectivity;
}
/**
*
* The Unix timestamp for the time when the task last went into CONNECTED
status.
*
*
* @return The Unix timestamp for the time when the task last went into CONNECTED
status.
*/
public final Instant connectivityAt() {
return connectivityAt;
}
/**
*
* The ARN of the container instances that host the task.
*
*
* @return The ARN of the container instances that host the task.
*/
public final String containerInstanceArn() {
return containerInstanceArn;
}
/**
* For responses, this returns true if the service returned a value for the Containers property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasContainers() {
return containers != null && !(containers instanceof SdkAutoConstructList);
}
/**
*
* The containers that's associated with the task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasContainers} method.
*
*
* @return The containers that's associated with the task.
*/
public final List containers() {
return containers;
}
/**
*
* The number of CPU units used by the task as expressed in a task definition. It can be expressed as an integer
* using CPU units (for example, 1024
). It can also be expressed as a string using vCPUs (for example,
* 1 vCPU
or 1 vcpu
). String values are converted to an integer that indicates the CPU
* units when the task definition is registered.
*
*
* If you use the EC2 launch type, this field is optional. Supported values are between 128
CPU units (
* 0.125
vCPUs) and 10240
CPU units (10
vCPUs).
*
*
* If you use the Fargate launch type, this field is required. You must use one of the following values. These
* values determine the range of supported values for the memory
parameter:
*
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6
* GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @return The number of CPU units used by the task as expressed in a task definition. It can be expressed as an
* integer using CPU units (for example, 1024
). It can also be expressed as a string using
* vCPUs (for example, 1 vCPU
or 1 vcpu
). String values are converted to an
* integer that indicates the CPU units when the task definition is registered.
*
* If you use the EC2 launch type, this field is optional. Supported values are between 128
CPU
* units (0.125
vCPUs) and 10240
CPU units (10
vCPUs).
*
*
* If you use the Fargate launch type, this field is required. You must use one of the following values.
* These values determine the range of supported values for the memory
parameter:
*
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB),
* 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*/
public final String cpu() {
return cpu;
}
/**
*
* The Unix timestamp for the time when the task was created. More specifically, it's for the time when the task
* entered the PENDING
state.
*
*
* @return The Unix timestamp for the time when the task was created. More specifically, it's for the time when the
* task entered the PENDING
state.
*/
public final Instant createdAt() {
return createdAt;
}
/**
*
* The desired status of the task. For more information, see Task Lifecycle.
*
*
* @return The desired status of the task. For more information, see Task
* Lifecycle.
*/
public final String desiredStatus() {
return desiredStatus;
}
/**
*
* Determines whether execute command functionality is turned on for this task. If true
, execute
* command functionality is turned on all the containers in the task.
*
*
* @return Determines whether execute command functionality is turned on for this task. If true
,
* execute command functionality is turned on all the containers in the task.
*/
public final Boolean enableExecuteCommand() {
return enableExecuteCommand;
}
/**
*
* The Unix timestamp for the time when the task execution stopped.
*
*
* @return The Unix timestamp for the time when the task execution stopped.
*/
public final Instant executionStoppedAt() {
return executionStoppedAt;
}
/**
*
* The name of the task group that's associated with the task.
*
*
* @return The name of the task group that's associated with the task.
*/
public final String group() {
return group;
}
/**
*
* The health status for the task. It's determined by the health of the essential containers in the task. If all
* essential containers in the task are reporting as HEALTHY
, the task status also reports as
* HEALTHY
. If any essential containers in the task are reporting as UNHEALTHY
or
* UNKNOWN
, the task status also reports as UNHEALTHY
or UNKNOWN
.
*
*
*
* The Amazon ECS container agent doesn't monitor or report on Docker health checks that are embedded in a container
* image and not specified in the container definition. For example, this includes those specified in a parent image
* or from the image's Dockerfile. Health check parameters that are specified in a container definition override any
* Docker health checks that are found in the container image.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #healthStatus} will
* return {@link HealthStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #healthStatusAsString}.
*
*
* @return The health status for the task. It's determined by the health of the essential containers in the task. If
* all essential containers in the task are reporting as HEALTHY
, the task status also reports
* as HEALTHY
. If any essential containers in the task are reporting as UNHEALTHY
* or UNKNOWN
, the task status also reports as UNHEALTHY
or UNKNOWN
* .
*
* The Amazon ECS container agent doesn't monitor or report on Docker health checks that are embedded in a
* container image and not specified in the container definition. For example, this includes those specified
* in a parent image or from the image's Dockerfile. Health check parameters that are specified in a
* container definition override any Docker health checks that are found in the container image.
*
* @see HealthStatus
*/
public final HealthStatus healthStatus() {
return HealthStatus.fromValue(healthStatus);
}
/**
*
* The health status for the task. It's determined by the health of the essential containers in the task. If all
* essential containers in the task are reporting as HEALTHY
, the task status also reports as
* HEALTHY
. If any essential containers in the task are reporting as UNHEALTHY
or
* UNKNOWN
, the task status also reports as UNHEALTHY
or UNKNOWN
.
*
*
*
* The Amazon ECS container agent doesn't monitor or report on Docker health checks that are embedded in a container
* image and not specified in the container definition. For example, this includes those specified in a parent image
* or from the image's Dockerfile. Health check parameters that are specified in a container definition override any
* Docker health checks that are found in the container image.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #healthStatus} will
* return {@link HealthStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #healthStatusAsString}.
*
*
* @return The health status for the task. It's determined by the health of the essential containers in the task. If
* all essential containers in the task are reporting as HEALTHY
, the task status also reports
* as HEALTHY
. If any essential containers in the task are reporting as UNHEALTHY
* or UNKNOWN
, the task status also reports as UNHEALTHY
or UNKNOWN
* .
*
* The Amazon ECS container agent doesn't monitor or report on Docker health checks that are embedded in a
* container image and not specified in the container definition. For example, this includes those specified
* in a parent image or from the image's Dockerfile. Health check parameters that are specified in a
* container definition override any Docker health checks that are found in the container image.
*
* @see HealthStatus
*/
public final String healthStatusAsString() {
return healthStatus;
}
/**
* For responses, this returns true if the service returned a value for the InferenceAccelerators property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasInferenceAccelerators() {
return inferenceAccelerators != null && !(inferenceAccelerators instanceof SdkAutoConstructList);
}
/**
*
* The Elastic Inference accelerator that's associated with the task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInferenceAccelerators} method.
*
*
* @return The Elastic Inference accelerator that's associated with the task.
*/
public final List inferenceAccelerators() {
return inferenceAccelerators;
}
/**
*
* The last known status for the task. For more information, see Task Lifecycle.
*
*
* @return The last known status for the task. For more information, see Task
* Lifecycle.
*/
public final String lastStatus() {
return lastStatus;
}
/**
*
* The infrastructure where your task runs on. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The infrastructure where your task runs on. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The infrastructure where your task runs on. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The infrastructure where your task runs on. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
/**
*
* The amount of memory (in MiB) that the task uses as expressed in a task definition. It can be expressed as an
* integer using MiB (for example, 1024
). If it's expressed as a string using GB (for example,
* 1GB
or 1 GB
), it's converted to an integer indicating the MiB when the task definition
* is registered.
*
*
* If you use the EC2 launch type, this field is optional.
*
*
* If you use the Fargate launch type, this field is required. You must use one of the following values. The value
* that you choose determines the range of supported values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values: 2048 (2
* vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values: 4096 (4
* vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @return The amount of memory (in MiB) that the task uses as expressed in a task definition. It can be expressed
* as an integer using MiB (for example, 1024
). If it's expressed as a string using GB (for
* example, 1GB
or 1 GB
), it's converted to an integer indicating the MiB when the
* task definition is registered.
*
* If you use the EC2 launch type, this field is optional.
*
*
* If you use the Fargate launch type, this field is required. You must use one of the following values. The
* value that you choose determines the range of supported values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 2048 (2 vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 4096 (4 vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*/
public final String memory() {
return memory;
}
/**
*
* One or more container overrides.
*
*
* @return One or more container overrides.
*/
public final TaskOverride overrides() {
return overrides;
}
/**
*
* The platform version where your task runs on. A platform version is only specified for tasks that use the Fargate
* launch type. If you didn't specify one, the LATEST
platform version is used. For more information,
* see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version where your task runs on. A platform version is only specified for tasks that use the
* Fargate launch type. If you didn't specify one, the LATEST
platform version is used. For
* more information, see Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* The operating system that your tasks are running on. A platform family is specified only for tasks that use the
* Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service
* (for example, LINUX.
).
*
*
* @return The operating system that your tasks are running on. A platform family is specified only for tasks that
* use the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service (for example, LINUX.
).
*/
public final String platformFamily() {
return platformFamily;
}
/**
*
* The Unix timestamp for the time when the container image pull began.
*
*
* @return The Unix timestamp for the time when the container image pull began.
*/
public final Instant pullStartedAt() {
return pullStartedAt;
}
/**
*
* The Unix timestamp for the time when the container image pull completed.
*
*
* @return The Unix timestamp for the time when the container image pull completed.
*/
public final Instant pullStoppedAt() {
return pullStoppedAt;
}
/**
*
* The Unix timestamp for the time when the task started. More specifically, it's for the time when the task
* transitioned from the PENDING
state to the RUNNING
state.
*
*
* @return The Unix timestamp for the time when the task started. More specifically, it's for the time when the task
* transitioned from the PENDING
state to the RUNNING
state.
*/
public final Instant startedAt() {
return startedAt;
}
/**
*
* The tag specified when a task is started. If an Amazon ECS service started the task, the startedBy
* parameter contains the deployment ID of that service.
*
*
* @return The tag specified when a task is started. If an Amazon ECS service started the task, the
* startedBy
parameter contains the deployment ID of that service.
*/
public final String startedBy() {
return startedBy;
}
/**
*
* The stop code indicating why a task was stopped. The stoppedReason
might contain additional details.
*
*
* For more information about stop code, see Stopped tasks error
* codes in the Amazon ECS User Guide.
*
*
* The following are valid values:
*
*
* -
*
* TaskFailedToStart
*
*
* -
*
* EssentialContainerExited
*
*
* -
*
* UserInitiated
*
*
* -
*
* TerminationNotice
*
*
* -
*
* ServiceSchedulerInitiated
*
*
* -
*
* SpotInterruption
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stopCode} will
* return {@link TaskStopCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stopCodeAsString}.
*
*
* @return The stop code indicating why a task was stopped. The stoppedReason
might contain additional
* details.
*
* For more information about stop code, see Stopped tasks
* error codes in the Amazon ECS User Guide.
*
*
* The following are valid values:
*
*
* -
*
* TaskFailedToStart
*
*
* -
*
* EssentialContainerExited
*
*
* -
*
* UserInitiated
*
*
* -
*
* TerminationNotice
*
*
* -
*
* ServiceSchedulerInitiated
*
*
* -
*
* SpotInterruption
*
*
* @see TaskStopCode
*/
public final TaskStopCode stopCode() {
return TaskStopCode.fromValue(stopCode);
}
/**
*
* The stop code indicating why a task was stopped. The stoppedReason
might contain additional details.
*
*
* For more information about stop code, see Stopped tasks error
* codes in the Amazon ECS User Guide.
*
*
* The following are valid values:
*
*
* -
*
* TaskFailedToStart
*
*
* -
*
* EssentialContainerExited
*
*
* -
*
* UserInitiated
*
*
* -
*
* TerminationNotice
*
*
* -
*
* ServiceSchedulerInitiated
*
*
* -
*
* SpotInterruption
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #stopCode} will
* return {@link TaskStopCode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stopCodeAsString}.
*
*
* @return The stop code indicating why a task was stopped. The stoppedReason
might contain additional
* details.
*
* For more information about stop code, see Stopped tasks
* error codes in the Amazon ECS User Guide.
*
*
* The following are valid values:
*
*
* -
*
* TaskFailedToStart
*
*
* -
*
* EssentialContainerExited
*
*
* -
*
* UserInitiated
*
*
* -
*
* TerminationNotice
*
*
* -
*
* ServiceSchedulerInitiated
*
*
* -
*
* SpotInterruption
*
*
* @see TaskStopCode
*/
public final String stopCodeAsString() {
return stopCode;
}
/**
*
* The Unix timestamp for the time when the task was stopped. More specifically, it's for the time when the task
* transitioned from the RUNNING
state to the STOPPED
state.
*
*
* @return The Unix timestamp for the time when the task was stopped. More specifically, it's for the time when the
* task transitioned from the RUNNING
state to the STOPPED
state.
*/
public final Instant stoppedAt() {
return stoppedAt;
}
/**
*
* The reason that the task was stopped.
*
*
* @return The reason that the task was stopped.
*/
public final String stoppedReason() {
return stoppedReason;
}
/**
*
* The Unix timestamp for the time when the task stops. More specifically, it's for the time when the task
* transitions from the RUNNING
state to STOPPING
.
*
*
* @return The Unix timestamp for the time when the task stops. More specifically, it's for the time when the task
* transitions from the RUNNING
state to STOPPING
.
*/
public final Instant stoppingAt() {
return stoppingAt;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the task to help you categorize and organize the task. Each tag consists of a key
* and an optional value. You define both the key and value.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The metadata that you apply to the task to help you categorize and organize the task. Each tag consists
* of a key and an optional value. You define both the key and value.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete
* tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource
* limit.
*
*
*/
public final List tags() {
return tags;
}
/**
*
* The Amazon Resource Name (ARN) of the task.
*
*
* @return The Amazon Resource Name (ARN) of the task.
*/
public final String taskArn() {
return taskArn;
}
/**
*
* The ARN of the task definition that creates the task.
*
*
* @return The ARN of the task definition that creates the task.
*/
public final String taskDefinitionArn() {
return taskDefinitionArn;
}
/**
*
* The version counter for the task. Every time a task experiences a change that starts a CloudWatch event, the
* version counter is incremented. If you replicate your Amazon ECS task state with CloudWatch Events, you can
* compare the version of a task reported by the Amazon ECS API actions with the version reported in CloudWatch
* Events for the task (inside the detail
object) to verify that the version in your event stream is
* current.
*
*
* @return The version counter for the task. Every time a task experiences a change that starts a CloudWatch event,
* the version counter is incremented. If you replicate your Amazon ECS task state with CloudWatch Events,
* you can compare the version of a task reported by the Amazon ECS API actions with the version reported in
* CloudWatch Events for the task (inside the detail
object) to verify that the version in your
* event stream is current.
*/
public final Long version() {
return version;
}
/**
*
* The ephemeral storage settings for the task.
*
*
* @return The ephemeral storage settings for the task.
*/
public final EphemeralStorage ephemeralStorage() {
return ephemeralStorage;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasAttachments() ? attachments() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAttributes() ? attributes() : null);
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(capacityProviderName());
hashCode = 31 * hashCode + Objects.hashCode(clusterArn());
hashCode = 31 * hashCode + Objects.hashCode(connectivityAsString());
hashCode = 31 * hashCode + Objects.hashCode(connectivityAt());
hashCode = 31 * hashCode + Objects.hashCode(containerInstanceArn());
hashCode = 31 * hashCode + Objects.hashCode(hasContainers() ? containers() : null);
hashCode = 31 * hashCode + Objects.hashCode(cpu());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(desiredStatus());
hashCode = 31 * hashCode + Objects.hashCode(enableExecuteCommand());
hashCode = 31 * hashCode + Objects.hashCode(executionStoppedAt());
hashCode = 31 * hashCode + Objects.hashCode(group());
hashCode = 31 * hashCode + Objects.hashCode(healthStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasInferenceAccelerators() ? inferenceAccelerators() : null);
hashCode = 31 * hashCode + Objects.hashCode(lastStatus());
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(memory());
hashCode = 31 * hashCode + Objects.hashCode(overrides());
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(platformFamily());
hashCode = 31 * hashCode + Objects.hashCode(pullStartedAt());
hashCode = 31 * hashCode + Objects.hashCode(pullStoppedAt());
hashCode = 31 * hashCode + Objects.hashCode(startedAt());
hashCode = 31 * hashCode + Objects.hashCode(startedBy());
hashCode = 31 * hashCode + Objects.hashCode(stopCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(stoppedAt());
hashCode = 31 * hashCode + Objects.hashCode(stoppedReason());
hashCode = 31 * hashCode + Objects.hashCode(stoppingAt());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(taskArn());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinitionArn());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(ephemeralStorage());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Task)) {
return false;
}
Task other = (Task) obj;
return hasAttachments() == other.hasAttachments() && Objects.equals(attachments(), other.attachments())
&& hasAttributes() == other.hasAttributes() && Objects.equals(attributes(), other.attributes())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& Objects.equals(capacityProviderName(), other.capacityProviderName())
&& Objects.equals(clusterArn(), other.clusterArn())
&& Objects.equals(connectivityAsString(), other.connectivityAsString())
&& Objects.equals(connectivityAt(), other.connectivityAt())
&& Objects.equals(containerInstanceArn(), other.containerInstanceArn())
&& hasContainers() == other.hasContainers() && Objects.equals(containers(), other.containers())
&& Objects.equals(cpu(), other.cpu()) && Objects.equals(createdAt(), other.createdAt())
&& Objects.equals(desiredStatus(), other.desiredStatus())
&& Objects.equals(enableExecuteCommand(), other.enableExecuteCommand())
&& Objects.equals(executionStoppedAt(), other.executionStoppedAt()) && Objects.equals(group(), other.group())
&& Objects.equals(healthStatusAsString(), other.healthStatusAsString())
&& hasInferenceAccelerators() == other.hasInferenceAccelerators()
&& Objects.equals(inferenceAccelerators(), other.inferenceAccelerators())
&& Objects.equals(lastStatus(), other.lastStatus())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString()) && Objects.equals(memory(), other.memory())
&& Objects.equals(overrides(), other.overrides()) && Objects.equals(platformVersion(), other.platformVersion())
&& Objects.equals(platformFamily(), other.platformFamily())
&& Objects.equals(pullStartedAt(), other.pullStartedAt())
&& Objects.equals(pullStoppedAt(), other.pullStoppedAt()) && Objects.equals(startedAt(), other.startedAt())
&& Objects.equals(startedBy(), other.startedBy()) && Objects.equals(stopCodeAsString(), other.stopCodeAsString())
&& Objects.equals(stoppedAt(), other.stoppedAt()) && Objects.equals(stoppedReason(), other.stoppedReason())
&& Objects.equals(stoppingAt(), other.stoppingAt()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(taskArn(), other.taskArn())
&& Objects.equals(taskDefinitionArn(), other.taskDefinitionArn()) && Objects.equals(version(), other.version())
&& Objects.equals(ephemeralStorage(), other.ephemeralStorage());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Task").add("Attachments", hasAttachments() ? attachments() : null)
.add("Attributes", hasAttributes() ? attributes() : null).add("AvailabilityZone", availabilityZone())
.add("CapacityProviderName", capacityProviderName()).add("ClusterArn", clusterArn())
.add("Connectivity", connectivityAsString()).add("ConnectivityAt", connectivityAt())
.add("ContainerInstanceArn", containerInstanceArn()).add("Containers", hasContainers() ? containers() : null)
.add("Cpu", cpu()).add("CreatedAt", createdAt()).add("DesiredStatus", desiredStatus())
.add("EnableExecuteCommand", enableExecuteCommand()).add("ExecutionStoppedAt", executionStoppedAt())
.add("Group", group()).add("HealthStatus", healthStatusAsString())
.add("InferenceAccelerators", hasInferenceAccelerators() ? inferenceAccelerators() : null)
.add("LastStatus", lastStatus()).add("LaunchType", launchTypeAsString()).add("Memory", memory())
.add("Overrides", overrides()).add("PlatformVersion", platformVersion()).add("PlatformFamily", platformFamily())
.add("PullStartedAt", pullStartedAt()).add("PullStoppedAt", pullStoppedAt()).add("StartedAt", startedAt())
.add("StartedBy", startedBy()).add("StopCode", stopCodeAsString()).add("StoppedAt", stoppedAt())
.add("StoppedReason", stoppedReason()).add("StoppingAt", stoppingAt()).add("Tags", hasTags() ? tags() : null)
.add("TaskArn", taskArn()).add("TaskDefinitionArn", taskDefinitionArn()).add("Version", version())
.add("EphemeralStorage", ephemeralStorage()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "attachments":
return Optional.ofNullable(clazz.cast(attachments()));
case "attributes":
return Optional.ofNullable(clazz.cast(attributes()));
case "availabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "capacityProviderName":
return Optional.ofNullable(clazz.cast(capacityProviderName()));
case "clusterArn":
return Optional.ofNullable(clazz.cast(clusterArn()));
case "connectivity":
return Optional.ofNullable(clazz.cast(connectivityAsString()));
case "connectivityAt":
return Optional.ofNullable(clazz.cast(connectivityAt()));
case "containerInstanceArn":
return Optional.ofNullable(clazz.cast(containerInstanceArn()));
case "containers":
return Optional.ofNullable(clazz.cast(containers()));
case "cpu":
return Optional.ofNullable(clazz.cast(cpu()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "desiredStatus":
return Optional.ofNullable(clazz.cast(desiredStatus()));
case "enableExecuteCommand":
return Optional.ofNullable(clazz.cast(enableExecuteCommand()));
case "executionStoppedAt":
return Optional.ofNullable(clazz.cast(executionStoppedAt()));
case "group":
return Optional.ofNullable(clazz.cast(group()));
case "healthStatus":
return Optional.ofNullable(clazz.cast(healthStatusAsString()));
case "inferenceAccelerators":
return Optional.ofNullable(clazz.cast(inferenceAccelerators()));
case "lastStatus":
return Optional.ofNullable(clazz.cast(lastStatus()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "memory":
return Optional.ofNullable(clazz.cast(memory()));
case "overrides":
return Optional.ofNullable(clazz.cast(overrides()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "platformFamily":
return Optional.ofNullable(clazz.cast(platformFamily()));
case "pullStartedAt":
return Optional.ofNullable(clazz.cast(pullStartedAt()));
case "pullStoppedAt":
return Optional.ofNullable(clazz.cast(pullStoppedAt()));
case "startedAt":
return Optional.ofNullable(clazz.cast(startedAt()));
case "startedBy":
return Optional.ofNullable(clazz.cast(startedBy()));
case "stopCode":
return Optional.ofNullable(clazz.cast(stopCodeAsString()));
case "stoppedAt":
return Optional.ofNullable(clazz.cast(stoppedAt()));
case "stoppedReason":
return Optional.ofNullable(clazz.cast(stoppedReason()));
case "stoppingAt":
return Optional.ofNullable(clazz.cast(stoppingAt()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "taskArn":
return Optional.ofNullable(clazz.cast(taskArn()));
case "taskDefinitionArn":
return Optional.ofNullable(clazz.cast(taskDefinitionArn()));
case "version":
return Optional.ofNullable(clazz.cast(version()));
case "ephemeralStorage":
return Optional.ofNullable(clazz.cast(ephemeralStorage()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function