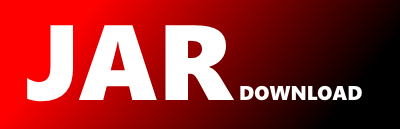
software.amazon.awssdk.services.ecs.model.UpdateClusterRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateClusterRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(UpdateClusterRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField> SETTINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("settings")
.getter(getter(UpdateClusterRequest::settings))
.setter(setter(Builder::settings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("settings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ClusterSetting::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("configuration")
.getter(getter(UpdateClusterRequest::configuration)).setter(setter(Builder::configuration))
.constructor(ClusterConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("configuration").build()).build();
private static final SdkField SERVICE_CONNECT_DEFAULTS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("serviceConnectDefaults")
.getter(getter(UpdateClusterRequest::serviceConnectDefaults)).setter(setter(Builder::serviceConnectDefaults))
.constructor(ClusterServiceConnectDefaultsRequest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceConnectDefaults").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD, SETTINGS_FIELD,
CONFIGURATION_FIELD, SERVICE_CONNECT_DEFAULTS_FIELD));
private final String cluster;
private final List settings;
private final ClusterConfiguration configuration;
private final ClusterServiceConnectDefaultsRequest serviceConnectDefaults;
private UpdateClusterRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.settings = builder.settings;
this.configuration = builder.configuration;
this.serviceConnectDefaults = builder.serviceConnectDefaults;
}
/**
*
* The name of the cluster to modify the settings for.
*
*
* @return The name of the cluster to modify the settings for.
*/
public final String cluster() {
return cluster;
}
/**
* For responses, this returns true if the service returned a value for the Settings property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasSettings() {
return settings != null && !(settings instanceof SdkAutoConstructList);
}
/**
*
* The cluster settings for your cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSettings} method.
*
*
* @return The cluster settings for your cluster.
*/
public final List settings() {
return settings;
}
/**
*
* The execute command configuration for the cluster.
*
*
* @return The execute command configuration for the cluster.
*/
public final ClusterConfiguration configuration() {
return configuration;
}
/**
*
* Use this parameter to set a default Service Connect namespace. After you set a default Service Connect namespace,
* any new services with Service Connect turned on that are created in the cluster are added as client services in
* the namespace. This setting only applies to new services that set the enabled
parameter to
* true
in the ServiceConnectConfiguration
. You can set the namespace of each service
* individually in the ServiceConnectConfiguration
to override this default parameter.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return Use this parameter to set a default Service Connect namespace. After you set a default Service Connect
* namespace, any new services with Service Connect turned on that are created in the cluster are added as
* client services in the namespace. This setting only applies to new services that set the
* enabled
parameter to true
in the ServiceConnectConfiguration
. You
* can set the namespace of each service individually in the ServiceConnectConfiguration
to
* override this default parameter.
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
*/
public final ClusterServiceConnectDefaultsRequest serviceConnectDefaults() {
return serviceConnectDefaults;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(hasSettings() ? settings() : null);
hashCode = 31 * hashCode + Objects.hashCode(configuration());
hashCode = 31 * hashCode + Objects.hashCode(serviceConnectDefaults());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateClusterRequest)) {
return false;
}
UpdateClusterRequest other = (UpdateClusterRequest) obj;
return Objects.equals(cluster(), other.cluster()) && hasSettings() == other.hasSettings()
&& Objects.equals(settings(), other.settings()) && Objects.equals(configuration(), other.configuration())
&& Objects.equals(serviceConnectDefaults(), other.serviceConnectDefaults());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateClusterRequest").add("Cluster", cluster())
.add("Settings", hasSettings() ? settings() : null).add("Configuration", configuration())
.add("ServiceConnectDefaults", serviceConnectDefaults()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "settings":
return Optional.ofNullable(clazz.cast(settings()));
case "configuration":
return Optional.ofNullable(clazz.cast(configuration()));
case "serviceConnectDefaults":
return Optional.ofNullable(clazz.cast(serviceConnectDefaults()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function