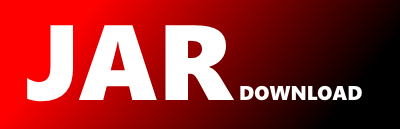
software.amazon.awssdk.services.ecs.model.Volume Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A data volume that's used in a task definition. For tasks that use the Amazon Elastic File System (Amazon EFS),
* specify an efsVolumeConfiguration
. For Windows tasks that use Amazon FSx for Windows File Server file
* system, specify a fsxWindowsFileServerVolumeConfiguration
. For tasks that use a Docker volume, specify a
* DockerVolumeConfiguration
. For tasks that use a bind mount host volume, specify a host
and
* optional sourcePath
. For more information, see Using Data Volumes in
* Tasks.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Volume implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Volume::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField HOST_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("host").getter(getter(Volume::host))
.setter(setter(Builder::host)).constructor(HostVolumeProperties::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("host").build()).build();
private static final SdkField DOCKER_VOLUME_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("dockerVolumeConfiguration")
.getter(getter(Volume::dockerVolumeConfiguration)).setter(setter(Builder::dockerVolumeConfiguration))
.constructor(DockerVolumeConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dockerVolumeConfiguration").build())
.build();
private static final SdkField EFS_VOLUME_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("efsVolumeConfiguration")
.getter(getter(Volume::efsVolumeConfiguration)).setter(setter(Builder::efsVolumeConfiguration))
.constructor(EFSVolumeConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("efsVolumeConfiguration").build())
.build();
private static final SdkField FSX_WINDOWS_FILE_SERVER_VOLUME_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("fsxWindowsFileServerVolumeConfiguration")
.getter(getter(Volume::fsxWindowsFileServerVolumeConfiguration))
.setter(setter(Builder::fsxWindowsFileServerVolumeConfiguration))
.constructor(FSxWindowsFileServerVolumeConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("fsxWindowsFileServerVolumeConfiguration").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays
.asList(NAME_FIELD, HOST_FIELD, DOCKER_VOLUME_CONFIGURATION_FIELD, EFS_VOLUME_CONFIGURATION_FIELD,
FSX_WINDOWS_FILE_SERVER_VOLUME_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final HostVolumeProperties host;
private final DockerVolumeConfiguration dockerVolumeConfiguration;
private final EFSVolumeConfiguration efsVolumeConfiguration;
private final FSxWindowsFileServerVolumeConfiguration fsxWindowsFileServerVolumeConfiguration;
private Volume(BuilderImpl builder) {
this.name = builder.name;
this.host = builder.host;
this.dockerVolumeConfiguration = builder.dockerVolumeConfiguration;
this.efsVolumeConfiguration = builder.efsVolumeConfiguration;
this.fsxWindowsFileServerVolumeConfiguration = builder.fsxWindowsFileServerVolumeConfiguration;
}
/**
*
* The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. This name is referenced in the sourceVolume
parameter of container definition
* mountPoints
.
*
*
* This is required wwhen you use an Amazon EFS volume.
*
*
* @return The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens
* are allowed. This name is referenced in the sourceVolume
parameter of container definition
* mountPoints
.
*
* This is required wwhen you use an Amazon EFS volume.
*/
public final String name() {
return name;
}
/**
*
* This parameter is specified when you use bind mount host volumes. The contents of the host
parameter
* determine whether your bind mount host volume persists on the host container instance and where it's stored. If
* the host
parameter is empty, then the Docker daemon assigns a host path for your data volume.
* However, the data isn't guaranteed to persist after the containers that are associated with it stop running.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives. For example, you
* can mount C:\my\path:C:\my\path
and D:\:D:\
, but not D:\my\path:C:\my\path
* or D:\:C:\my\path
.
*
*
* @return This parameter is specified when you use bind mount host volumes. The contents of the host
* parameter determine whether your bind mount host volume persists on the host container instance and where
* it's stored. If the host
parameter is empty, then the Docker daemon assigns a host path for
* your data volume. However, the data isn't guaranteed to persist after the containers that are associated
* with it stop running.
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
.
* Windows containers can't mount directories on a different drive, and mount point can't be across drives.
* For example, you can mount C:\my\path:C:\my\path
and D:\:D:\
, but not
* D:\my\path:C:\my\path
or D:\:C:\my\path
.
*/
public final HostVolumeProperties host() {
return host;
}
/**
*
* This parameter is specified when you use Docker volumes.
*
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
*
*
* @return This parameter is specified when you use Docker volumes.
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
*/
public final DockerVolumeConfiguration dockerVolumeConfiguration() {
return dockerVolumeConfiguration;
}
/**
*
* This parameter is specified when you use an Amazon Elastic File System file system for task storage.
*
*
* @return This parameter is specified when you use an Amazon Elastic File System file system for task storage.
*/
public final EFSVolumeConfiguration efsVolumeConfiguration() {
return efsVolumeConfiguration;
}
/**
*
* This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
*
*
* @return This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
*/
public final FSxWindowsFileServerVolumeConfiguration fsxWindowsFileServerVolumeConfiguration() {
return fsxWindowsFileServerVolumeConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(host());
hashCode = 31 * hashCode + Objects.hashCode(dockerVolumeConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(efsVolumeConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(fsxWindowsFileServerVolumeConfiguration());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Volume)) {
return false;
}
Volume other = (Volume) obj;
return Objects.equals(name(), other.name()) && Objects.equals(host(), other.host())
&& Objects.equals(dockerVolumeConfiguration(), other.dockerVolumeConfiguration())
&& Objects.equals(efsVolumeConfiguration(), other.efsVolumeConfiguration())
&& Objects.equals(fsxWindowsFileServerVolumeConfiguration(), other.fsxWindowsFileServerVolumeConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Volume").add("Name", name()).add("Host", host())
.add("DockerVolumeConfiguration", dockerVolumeConfiguration())
.add("EfsVolumeConfiguration", efsVolumeConfiguration())
.add("FsxWindowsFileServerVolumeConfiguration", fsxWindowsFileServerVolumeConfiguration()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "host":
return Optional.ofNullable(clazz.cast(host()));
case "dockerVolumeConfiguration":
return Optional.ofNullable(clazz.cast(dockerVolumeConfiguration()));
case "efsVolumeConfiguration":
return Optional.ofNullable(clazz.cast(efsVolumeConfiguration()));
case "fsxWindowsFileServerVolumeConfiguration":
return Optional.ofNullable(clazz.cast(fsxWindowsFileServerVolumeConfiguration()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function