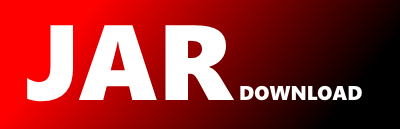
software.amazon.awssdk.services.ecs.model.Attribute Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An attribute is a name-value pair that's associated with an Amazon ECS object. Use attributes to extend the Amazon
* ECS data model by adding custom metadata to your resources. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Attribute implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Attribute::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField VALUE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("value")
.getter(getter(Attribute::value)).setter(setter(Builder::value))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("value").build()).build();
private static final SdkField TARGET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("targetType").getter(getter(Attribute::targetTypeAsString)).setter(setter(Builder::targetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetType").build()).build();
private static final SdkField TARGET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("targetId").getter(getter(Attribute::targetId)).setter(setter(Builder::targetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, VALUE_FIELD,
TARGET_TYPE_FIELD, TARGET_ID_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final String value;
private final String targetType;
private final String targetId;
private Attribute(BuilderImpl builder) {
this.name = builder.name;
this.value = builder.value;
this.targetType = builder.targetType;
this.targetId = builder.targetId;
}
/**
*
* The name of the attribute. The name
must contain between 1 and 128 characters. The name may contain
* letters (uppercase and lowercase), numbers, hyphens (-), underscores (_), forward slashes (/), back slashes (\),
* or periods (.).
*
*
* @return The name of the attribute. The name
must contain between 1 and 128 characters. The name may
* contain letters (uppercase and lowercase), numbers, hyphens (-), underscores (_), forward slashes (/),
* back slashes (\), or periods (.).
*/
public final String name() {
return name;
}
/**
*
* The value of the attribute. The value
must contain between 1 and 128 characters. It can contain
* letters (uppercase and lowercase), numbers, hyphens (-), underscores (_), periods (.), at signs (@), forward
* slashes (/), back slashes (\), colons (:), or spaces. The value can't start or end with a space.
*
*
* @return The value of the attribute. The value
must contain between 1 and 128 characters. It can
* contain letters (uppercase and lowercase), numbers, hyphens (-), underscores (_), periods (.), at signs
* (@), forward slashes (/), back slashes (\), colons (:), or spaces. The value can't start or end with a
* space.
*/
public final String value() {
return value;
}
/**
*
* The type of the target to attach the attribute with. This parameter is required if you use the short form ID for
* a resource instead of the full ARN.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #targetType} will
* return {@link TargetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #targetTypeAsString}.
*
*
* @return The type of the target to attach the attribute with. This parameter is required if you use the short form
* ID for a resource instead of the full ARN.
* @see TargetType
*/
public final TargetType targetType() {
return TargetType.fromValue(targetType);
}
/**
*
* The type of the target to attach the attribute with. This parameter is required if you use the short form ID for
* a resource instead of the full ARN.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #targetType} will
* return {@link TargetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #targetTypeAsString}.
*
*
* @return The type of the target to attach the attribute with. This parameter is required if you use the short form
* ID for a resource instead of the full ARN.
* @see TargetType
*/
public final String targetTypeAsString() {
return targetType;
}
/**
*
* The ID of the target. You can specify the short form ID for a resource or the full Amazon Resource Name (ARN).
*
*
* @return The ID of the target. You can specify the short form ID for a resource or the full Amazon Resource Name
* (ARN).
*/
public final String targetId() {
return targetId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(value());
hashCode = 31 * hashCode + Objects.hashCode(targetTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(targetId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Attribute)) {
return false;
}
Attribute other = (Attribute) obj;
return Objects.equals(name(), other.name()) && Objects.equals(value(), other.value())
&& Objects.equals(targetTypeAsString(), other.targetTypeAsString())
&& Objects.equals(targetId(), other.targetId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Attribute").add("Name", name()).add("Value", value()).add("TargetType", targetTypeAsString())
.add("TargetId", targetId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "value":
return Optional.ofNullable(clazz.cast(value()));
case "targetType":
return Optional.ofNullable(clazz.cast(targetTypeAsString()));
case "targetId":
return Optional.ofNullable(clazz.cast(targetId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function