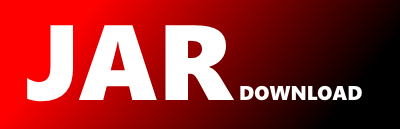
software.amazon.awssdk.services.ecs.model.AutoScalingGroupProviderUpdate Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of the Auto Scaling group capacity provider to update.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AutoScalingGroupProviderUpdate implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField MANAGED_SCALING_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("managedScaling")
.getter(getter(AutoScalingGroupProviderUpdate::managedScaling)).setter(setter(Builder::managedScaling))
.constructor(ManagedScaling::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("managedScaling").build()).build();
private static final SdkField MANAGED_TERMINATION_PROTECTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("managedTerminationProtection")
.getter(getter(AutoScalingGroupProviderUpdate::managedTerminationProtectionAsString))
.setter(setter(Builder::managedTerminationProtection))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("managedTerminationProtection")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MANAGED_SCALING_FIELD,
MANAGED_TERMINATION_PROTECTION_FIELD));
private static final long serialVersionUID = 1L;
private final ManagedScaling managedScaling;
private final String managedTerminationProtection;
private AutoScalingGroupProviderUpdate(BuilderImpl builder) {
this.managedScaling = builder.managedScaling;
this.managedTerminationProtection = builder.managedTerminationProtection;
}
/**
*
* The managed scaling settings for the Auto Scaling group capacity provider.
*
*
* @return The managed scaling settings for the Auto Scaling group capacity provider.
*/
public final ManagedScaling managedScaling() {
return managedScaling;
}
/**
*
* The managed termination protection setting to use for the Auto Scaling group capacity provider. This determines
* whether the Auto Scaling group has managed termination protection.
*
*
*
* When using managed termination protection, managed scaling must also be used otherwise managed termination
* protection doesn't work.
*
*
*
* When managed termination protection is on, Amazon ECS prevents the Amazon EC2 instances in an Auto Scaling group
* that contain tasks from being terminated during a scale-in action. The Auto Scaling group and each instance in
* the Auto Scaling group must have instance protection from scale-in actions on. For more information, see Instance Protection in the Auto Scaling User Guide.
*
*
* When managed termination protection is off, your Amazon EC2 instances aren't protected from termination when the
* Auto Scaling group scales in.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #managedTerminationProtection} will return {@link ManagedTerminationProtection#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #managedTerminationProtectionAsString}.
*
*
* @return The managed termination protection setting to use for the Auto Scaling group capacity provider. This
* determines whether the Auto Scaling group has managed termination protection.
*
* When using managed termination protection, managed scaling must also be used otherwise managed
* termination protection doesn't work.
*
*
*
* When managed termination protection is on, Amazon ECS prevents the Amazon EC2 instances in an Auto
* Scaling group that contain tasks from being terminated during a scale-in action. The Auto Scaling group
* and each instance in the Auto Scaling group must have instance protection from scale-in actions on. For
* more information, see Instance Protection in the Auto Scaling User Guide.
*
*
* When managed termination protection is off, your Amazon EC2 instances aren't protected from termination
* when the Auto Scaling group scales in.
* @see ManagedTerminationProtection
*/
public final ManagedTerminationProtection managedTerminationProtection() {
return ManagedTerminationProtection.fromValue(managedTerminationProtection);
}
/**
*
* The managed termination protection setting to use for the Auto Scaling group capacity provider. This determines
* whether the Auto Scaling group has managed termination protection.
*
*
*
* When using managed termination protection, managed scaling must also be used otherwise managed termination
* protection doesn't work.
*
*
*
* When managed termination protection is on, Amazon ECS prevents the Amazon EC2 instances in an Auto Scaling group
* that contain tasks from being terminated during a scale-in action. The Auto Scaling group and each instance in
* the Auto Scaling group must have instance protection from scale-in actions on. For more information, see Instance Protection in the Auto Scaling User Guide.
*
*
* When managed termination protection is off, your Amazon EC2 instances aren't protected from termination when the
* Auto Scaling group scales in.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #managedTerminationProtection} will return {@link ManagedTerminationProtection#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #managedTerminationProtectionAsString}.
*
*
* @return The managed termination protection setting to use for the Auto Scaling group capacity provider. This
* determines whether the Auto Scaling group has managed termination protection.
*
* When using managed termination protection, managed scaling must also be used otherwise managed
* termination protection doesn't work.
*
*
*
* When managed termination protection is on, Amazon ECS prevents the Amazon EC2 instances in an Auto
* Scaling group that contain tasks from being terminated during a scale-in action. The Auto Scaling group
* and each instance in the Auto Scaling group must have instance protection from scale-in actions on. For
* more information, see Instance Protection in the Auto Scaling User Guide.
*
*
* When managed termination protection is off, your Amazon EC2 instances aren't protected from termination
* when the Auto Scaling group scales in.
* @see ManagedTerminationProtection
*/
public final String managedTerminationProtectionAsString() {
return managedTerminationProtection;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(managedScaling());
hashCode = 31 * hashCode + Objects.hashCode(managedTerminationProtectionAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AutoScalingGroupProviderUpdate)) {
return false;
}
AutoScalingGroupProviderUpdate other = (AutoScalingGroupProviderUpdate) obj;
return Objects.equals(managedScaling(), other.managedScaling())
&& Objects.equals(managedTerminationProtectionAsString(), other.managedTerminationProtectionAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AutoScalingGroupProviderUpdate").add("ManagedScaling", managedScaling())
.add("ManagedTerminationProtection", managedTerminationProtectionAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "managedScaling":
return Optional.ofNullable(clazz.cast(managedScaling()));
case "managedTerminationProtection":
return Optional.ofNullable(clazz.cast(managedTerminationProtectionAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* When using managed termination protection, managed scaling must also be used otherwise managed
* termination protection doesn't work.
*
*
*
* When managed termination protection is on, Amazon ECS prevents the Amazon EC2 instances in an Auto
* Scaling group that contain tasks from being terminated during a scale-in action. The Auto Scaling
* group and each instance in the Auto Scaling group must have instance protection from scale-in actions
* on. For more information, see Instance Protection in the Auto Scaling User Guide.
*
*
* When managed termination protection is off, your Amazon EC2 instances aren't protected from
* termination when the Auto Scaling group scales in.
* @see ManagedTerminationProtection
* @return Returns a reference to this object so that method calls can be chained together.
* @see ManagedTerminationProtection
*/
Builder managedTerminationProtection(ManagedTerminationProtection managedTerminationProtection);
}
static final class BuilderImpl implements Builder {
private ManagedScaling managedScaling;
private String managedTerminationProtection;
private BuilderImpl() {
}
private BuilderImpl(AutoScalingGroupProviderUpdate model) {
managedScaling(model.managedScaling);
managedTerminationProtection(model.managedTerminationProtection);
}
public final ManagedScaling.Builder getManagedScaling() {
return managedScaling != null ? managedScaling.toBuilder() : null;
}
public final void setManagedScaling(ManagedScaling.BuilderImpl managedScaling) {
this.managedScaling = managedScaling != null ? managedScaling.build() : null;
}
@Override
public final Builder managedScaling(ManagedScaling managedScaling) {
this.managedScaling = managedScaling;
return this;
}
public final String getManagedTerminationProtection() {
return managedTerminationProtection;
}
public final void setManagedTerminationProtection(String managedTerminationProtection) {
this.managedTerminationProtection = managedTerminationProtection;
}
@Override
public final Builder managedTerminationProtection(String managedTerminationProtection) {
this.managedTerminationProtection = managedTerminationProtection;
return this;
}
@Override
public final Builder managedTerminationProtection(ManagedTerminationProtection managedTerminationProtection) {
this.managedTerminationProtection(managedTerminationProtection == null ? null : managedTerminationProtection
.toString());
return this;
}
@Override
public AutoScalingGroupProviderUpdate build() {
return new AutoScalingGroupProviderUpdate(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}