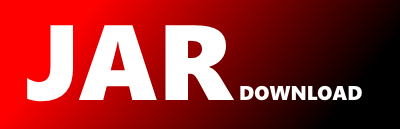
software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of a capacity provider strategy. A capacity provider strategy can be set when using the RunTask or
* CreateCluster APIs or as the default capacity provider strategy for a cluster with the CreateCluster
* API.
*
*
* Only capacity providers that are already associated with a cluster and have an ACTIVE
or
* UPDATING
status can be used in a capacity provider strategy. The PutClusterCapacityProviders API
* is used to associate a capacity provider with a cluster.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be created. New
* Auto Scaling group capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
capacity
* providers. The Fargate capacity providers are available to all accounts and only need to be associated with a cluster
* to be used in a capacity provider strategy.
*
*
* A capacity provider strategy may contain a maximum of 6 capacity providers.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CapacityProviderStrategyItem implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CAPACITY_PROVIDER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("capacityProvider").getter(getter(CapacityProviderStrategyItem::capacityProvider))
.setter(setter(Builder::capacityProvider))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProvider").build()).build();
private static final SdkField WEIGHT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("weight").getter(getter(CapacityProviderStrategyItem::weight)).setter(setter(Builder::weight))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("weight").build()).build();
private static final SdkField BASE_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("base")
.getter(getter(CapacityProviderStrategyItem::base)).setter(setter(Builder::base))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("base").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CAPACITY_PROVIDER_FIELD,
WEIGHT_FIELD, BASE_FIELD));
private static final long serialVersionUID = 1L;
private final String capacityProvider;
private final Integer weight;
private final Integer base;
private CapacityProviderStrategyItem(BuilderImpl builder) {
this.capacityProvider = builder.capacityProvider;
this.weight = builder.weight;
this.base = builder.base;
}
/**
*
* The short name of the capacity provider.
*
*
* @return The short name of the capacity provider.
*/
public final String capacityProvider() {
return capacityProvider;
}
/**
*
* The weight value designates the relative percentage of the total number of tasks launched that should use
* the specified capacity provider. The weight
value is taken into consideration after the
* base
value, if defined, is satisfied.
*
*
* If no weight
value is specified, the default value of 0
is used. When multiple capacity
* providers are specified within a capacity provider strategy, at least one of the capacity providers must have a
* weight value greater than zero and any capacity providers with a weight of 0
can't be used to place
* tasks. If you specify multiple capacity providers in a strategy that all have a weight of 0
, any
* RunTask
or CreateService
actions using the capacity provider strategy will fail.
*
*
* An example scenario for using weights is defining a strategy that contains two capacity providers and both have a
* weight of 1
, then when the base
is satisfied, the tasks will be split evenly across the
* two capacity providers. Using that same logic, if you specify a weight of 1
for
* capacityProviderA and a weight of 4
for capacityProviderB, then for every one task
* that's run using capacityProviderA, four tasks would use capacityProviderB.
*
*
* @return The weight value designates the relative percentage of the total number of tasks launched that
* should use the specified capacity provider. The weight
value is taken into consideration
* after the base
value, if defined, is satisfied.
*
* If no weight
value is specified, the default value of 0
is used. When multiple
* capacity providers are specified within a capacity provider strategy, at least one of the capacity
* providers must have a weight value greater than zero and any capacity providers with a weight of
* 0
can't be used to place tasks. If you specify multiple capacity providers in a strategy
* that all have a weight of 0
, any RunTask
or CreateService
actions
* using the capacity provider strategy will fail.
*
*
* An example scenario for using weights is defining a strategy that contains two capacity providers and
* both have a weight of 1
, then when the base
is satisfied, the tasks will be
* split evenly across the two capacity providers. Using that same logic, if you specify a weight of
* 1
for capacityProviderA and a weight of 4
for capacityProviderB,
* then for every one task that's run using capacityProviderA, four tasks would use
* capacityProviderB.
*/
public final Integer weight() {
return weight;
}
/**
*
* The base value designates how many tasks, at a minimum, to run on the specified capacity provider. Only
* one capacity provider in a capacity provider strategy can have a base defined. If no value is specified,
* the default value of 0
is used.
*
*
* @return The base value designates how many tasks, at a minimum, to run on the specified capacity provider.
* Only one capacity provider in a capacity provider strategy can have a base defined. If no value is
* specified, the default value of 0
is used.
*/
public final Integer base() {
return base;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(capacityProvider());
hashCode = 31 * hashCode + Objects.hashCode(weight());
hashCode = 31 * hashCode + Objects.hashCode(base());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CapacityProviderStrategyItem)) {
return false;
}
CapacityProviderStrategyItem other = (CapacityProviderStrategyItem) obj;
return Objects.equals(capacityProvider(), other.capacityProvider()) && Objects.equals(weight(), other.weight())
&& Objects.equals(base(), other.base());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CapacityProviderStrategyItem").add("CapacityProvider", capacityProvider())
.add("Weight", weight()).add("Base", base()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "capacityProvider":
return Optional.ofNullable(clazz.cast(capacityProvider()));
case "weight":
return Optional.ofNullable(clazz.cast(weight()));
case "base":
return Optional.ofNullable(clazz.cast(base()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function