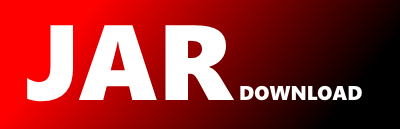
software.amazon.awssdk.services.ecs.model.EFSVolumeConfiguration Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* This parameter is specified when you're using an Amazon Elastic File System file system for task storage. For more
* information, see Amazon EFS
* volumes in the Amazon Elastic Container Service Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EFSVolumeConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField FILE_SYSTEM_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("fileSystemId").getter(getter(EFSVolumeConfiguration::fileSystemId))
.setter(setter(Builder::fileSystemId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fileSystemId").build()).build();
private static final SdkField ROOT_DIRECTORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("rootDirectory").getter(getter(EFSVolumeConfiguration::rootDirectory))
.setter(setter(Builder::rootDirectory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rootDirectory").build()).build();
private static final SdkField TRANSIT_ENCRYPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("transitEncryption").getter(getter(EFSVolumeConfiguration::transitEncryptionAsString))
.setter(setter(Builder::transitEncryption))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("transitEncryption").build()).build();
private static final SdkField TRANSIT_ENCRYPTION_PORT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("transitEncryptionPort").getter(getter(EFSVolumeConfiguration::transitEncryptionPort))
.setter(setter(Builder::transitEncryptionPort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("transitEncryptionPort").build())
.build();
private static final SdkField AUTHORIZATION_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("authorizationConfig")
.getter(getter(EFSVolumeConfiguration::authorizationConfig)).setter(setter(Builder::authorizationConfig))
.constructor(EFSAuthorizationConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("authorizationConfig").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FILE_SYSTEM_ID_FIELD,
ROOT_DIRECTORY_FIELD, TRANSIT_ENCRYPTION_FIELD, TRANSIT_ENCRYPTION_PORT_FIELD, AUTHORIZATION_CONFIG_FIELD));
private static final long serialVersionUID = 1L;
private final String fileSystemId;
private final String rootDirectory;
private final String transitEncryption;
private final Integer transitEncryptionPort;
private final EFSAuthorizationConfig authorizationConfig;
private EFSVolumeConfiguration(BuilderImpl builder) {
this.fileSystemId = builder.fileSystemId;
this.rootDirectory = builder.rootDirectory;
this.transitEncryption = builder.transitEncryption;
this.transitEncryptionPort = builder.transitEncryptionPort;
this.authorizationConfig = builder.authorizationConfig;
}
/**
*
* The Amazon EFS file system ID to use.
*
*
* @return The Amazon EFS file system ID to use.
*/
public final String fileSystemId() {
return fileSystemId;
}
/**
*
* The directory within the Amazon EFS file system to mount as the root directory inside the host. If this parameter
* is omitted, the root of the Amazon EFS volume will be used. Specifying /
will have the same effect
* as omitting this parameter.
*
*
*
* If an EFS access point is specified in the authorizationConfig
, the root directory parameter must
* either be omitted or set to /
which will enforce the path set on the EFS access point.
*
*
*
* @return The directory within the Amazon EFS file system to mount as the root directory inside the host. If this
* parameter is omitted, the root of the Amazon EFS volume will be used. Specifying /
will have
* the same effect as omitting this parameter.
*
* If an EFS access point is specified in the authorizationConfig
, the root directory parameter
* must either be omitted or set to /
which will enforce the path set on the EFS access point.
*
*/
public final String rootDirectory() {
return rootDirectory;
}
/**
*
* Determines whether to use encryption for Amazon EFS data in transit between the Amazon ECS host and the Amazon
* EFS server. Transit encryption must be turned on if Amazon EFS IAM authorization is used. If this parameter is
* omitted, the default value of DISABLED
is used. For more information, see Encrypting data in transit in the
* Amazon Elastic File System User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #transitEncryption}
* will return {@link EFSTransitEncryption#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #transitEncryptionAsString}.
*
*
* @return Determines whether to use encryption for Amazon EFS data in transit between the Amazon ECS host and the
* Amazon EFS server. Transit encryption must be turned on if Amazon EFS IAM authorization is used. If this
* parameter is omitted, the default value of DISABLED
is used. For more information, see Encrypting data in
* transit in the Amazon Elastic File System User Guide.
* @see EFSTransitEncryption
*/
public final EFSTransitEncryption transitEncryption() {
return EFSTransitEncryption.fromValue(transitEncryption);
}
/**
*
* Determines whether to use encryption for Amazon EFS data in transit between the Amazon ECS host and the Amazon
* EFS server. Transit encryption must be turned on if Amazon EFS IAM authorization is used. If this parameter is
* omitted, the default value of DISABLED
is used. For more information, see Encrypting data in transit in the
* Amazon Elastic File System User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #transitEncryption}
* will return {@link EFSTransitEncryption#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #transitEncryptionAsString}.
*
*
* @return Determines whether to use encryption for Amazon EFS data in transit between the Amazon ECS host and the
* Amazon EFS server. Transit encryption must be turned on if Amazon EFS IAM authorization is used. If this
* parameter is omitted, the default value of DISABLED
is used. For more information, see Encrypting data in
* transit in the Amazon Elastic File System User Guide.
* @see EFSTransitEncryption
*/
public final String transitEncryptionAsString() {
return transitEncryption;
}
/**
*
* The port to use when sending encrypted data between the Amazon ECS host and the Amazon EFS server. If you do not
* specify a transit encryption port, it will use the port selection strategy that the Amazon EFS mount helper uses.
* For more information, see EFS mount
* helper in the Amazon Elastic File System User Guide.
*
*
* @return The port to use when sending encrypted data between the Amazon ECS host and the Amazon EFS server. If you
* do not specify a transit encryption port, it will use the port selection strategy that the Amazon EFS
* mount helper uses. For more information, see EFS mount helper in the
* Amazon Elastic File System User Guide.
*/
public final Integer transitEncryptionPort() {
return transitEncryptionPort;
}
/**
*
* The authorization configuration details for the Amazon EFS file system.
*
*
* @return The authorization configuration details for the Amazon EFS file system.
*/
public final EFSAuthorizationConfig authorizationConfig() {
return authorizationConfig;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(fileSystemId());
hashCode = 31 * hashCode + Objects.hashCode(rootDirectory());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionAsString());
hashCode = 31 * hashCode + Objects.hashCode(transitEncryptionPort());
hashCode = 31 * hashCode + Objects.hashCode(authorizationConfig());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EFSVolumeConfiguration)) {
return false;
}
EFSVolumeConfiguration other = (EFSVolumeConfiguration) obj;
return Objects.equals(fileSystemId(), other.fileSystemId()) && Objects.equals(rootDirectory(), other.rootDirectory())
&& Objects.equals(transitEncryptionAsString(), other.transitEncryptionAsString())
&& Objects.equals(transitEncryptionPort(), other.transitEncryptionPort())
&& Objects.equals(authorizationConfig(), other.authorizationConfig());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EFSVolumeConfiguration").add("FileSystemId", fileSystemId())
.add("RootDirectory", rootDirectory()).add("TransitEncryption", transitEncryptionAsString())
.add("TransitEncryptionPort", transitEncryptionPort()).add("AuthorizationConfig", authorizationConfig()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "fileSystemId":
return Optional.ofNullable(clazz.cast(fileSystemId()));
case "rootDirectory":
return Optional.ofNullable(clazz.cast(rootDirectory()));
case "transitEncryption":
return Optional.ofNullable(clazz.cast(transitEncryptionAsString()));
case "transitEncryptionPort":
return Optional.ofNullable(clazz.cast(transitEncryptionPort()));
case "authorizationConfig":
return Optional.ofNullable(clazz.cast(authorizationConfig()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function