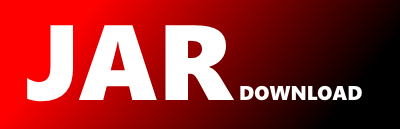
software.amazon.awssdk.services.ecs.model.StartTaskRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartTaskRequest extends EcsRequest implements ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(StartTaskRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField> CONTAINER_INSTANCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("containerInstances")
.getter(getter(StartTaskRequest::containerInstances))
.setter(setter(Builder::containerInstances))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerInstances").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ENABLE_ECS_MANAGED_TAGS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableECSManagedTags").getter(getter(StartTaskRequest::enableECSManagedTags))
.setter(setter(Builder::enableECSManagedTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableECSManagedTags").build())
.build();
private static final SdkField ENABLE_EXECUTE_COMMAND_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableExecuteCommand").getter(getter(StartTaskRequest::enableExecuteCommand))
.setter(setter(Builder::enableExecuteCommand))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableExecuteCommand").build())
.build();
private static final SdkField GROUP_FIELD = SdkField. builder(MarshallingType.STRING).memberName("group")
.getter(getter(StartTaskRequest::group)).setter(setter(Builder::group))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("group").build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkConfiguration")
.getter(getter(StartTaskRequest::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField OVERRIDES_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("overrides").getter(getter(StartTaskRequest::overrides)).setter(setter(Builder::overrides))
.constructor(TaskOverride::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("overrides").build()).build();
private static final SdkField PROPAGATE_TAGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("propagateTags").getter(getter(StartTaskRequest::propagateTagsAsString))
.setter(setter(Builder::propagateTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("propagateTags").build()).build();
private static final SdkField REFERENCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("referenceId").getter(getter(StartTaskRequest::referenceId)).setter(setter(Builder::referenceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("referenceId").build()).build();
private static final SdkField STARTED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("startedBy").getter(getter(StartTaskRequest::startedBy)).setter(setter(Builder::startedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startedBy").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(StartTaskRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinition").getter(getter(StartTaskRequest::taskDefinition))
.setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD,
CONTAINER_INSTANCES_FIELD, ENABLE_ECS_MANAGED_TAGS_FIELD, ENABLE_EXECUTE_COMMAND_FIELD, GROUP_FIELD,
NETWORK_CONFIGURATION_FIELD, OVERRIDES_FIELD, PROPAGATE_TAGS_FIELD, REFERENCE_ID_FIELD, STARTED_BY_FIELD, TAGS_FIELD,
TASK_DEFINITION_FIELD));
private final String cluster;
private final List containerInstances;
private final Boolean enableECSManagedTags;
private final Boolean enableExecuteCommand;
private final String group;
private final NetworkConfiguration networkConfiguration;
private final TaskOverride overrides;
private final String propagateTags;
private final String referenceId;
private final String startedBy;
private final List tags;
private final String taskDefinition;
private StartTaskRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.containerInstances = builder.containerInstances;
this.enableECSManagedTags = builder.enableECSManagedTags;
this.enableExecuteCommand = builder.enableExecuteCommand;
this.group = builder.group;
this.networkConfiguration = builder.networkConfiguration;
this.overrides = builder.overrides;
this.propagateTags = builder.propagateTags;
this.referenceId = builder.referenceId;
this.startedBy = builder.startedBy;
this.tags = builder.tags;
this.taskDefinition = builder.taskDefinition;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster where to start your task. If you do not specify
* a cluster, the default cluster is assumed.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster where to start your task. If you do not
* specify a cluster, the default cluster is assumed.
*/
public final String cluster() {
return cluster;
}
/**
* For responses, this returns true if the service returned a value for the ContainerInstances property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasContainerInstances() {
return containerInstances != null && !(containerInstances instanceof SdkAutoConstructList);
}
/**
*
* The container instance IDs or full ARN entries for the container instances where you would like to place your
* task. You can specify up to 10 container instances.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasContainerInstances} method.
*
*
* @return The container instance IDs or full ARN entries for the container instances where you would like to place
* your task. You can specify up to 10 container instances.
*/
public final List containerInstances() {
return containerInstances;
}
/**
*
* Specifies whether to use Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies whether to use Amazon ECS managed tags for the task. For more information, see Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public final Boolean enableECSManagedTags() {
return enableECSManagedTags;
}
/**
*
* Whether or not the execute command functionality is turned on for the task. If true
, this turns on
* the execute command functionality on all containers in the task.
*
*
* @return Whether or not the execute command functionality is turned on for the task. If true
, this
* turns on the execute command functionality on all containers in the task.
*/
public final Boolean enableExecuteCommand() {
return enableExecuteCommand;
}
/**
*
* The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*
*
* @return The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*/
public final String group() {
return group;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @return The VPC subnet and security group configuration for tasks that receive their own elastic network
* interface by using the awsvpc
networking mode.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
*
* A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it receives. You can override the default command for a container (that's specified
* in the task definition or Docker image) with a command
override. You can also override existing
* environment variables (that are specified in the task definition or Docker image) on a container or add new
* environment variables to it with an environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters of the
* override structure.
*
*
*
* @return A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it receives. You can override the default command for a container (that's
* specified in the task definition or Docker image) with a command
override. You can also
* override existing environment variables (that are specified in the task definition or Docker image) on a
* container or add new environment variables to it with an environment
override.
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters
* of the override structure.
*
*/
public final TaskOverride overrides() {
return overrides;
}
/**
*
* Specifies whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Specifies whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
* @see PropagateTags
*/
public final PropagateTags propagateTags() {
return PropagateTags.fromValue(propagateTags);
}
/**
*
* Specifies whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Specifies whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
* @see PropagateTags
*/
public final String propagateTagsAsString() {
return propagateTags;
}
/**
*
* The reference ID to use for the task.
*
*
* @return The reference ID to use for the task.
*/
public final String referenceId() {
return referenceId;
}
/**
*
* An optional tag specified when a task is started. For example, if you automatically trigger a task to run a batch
* process job, you could apply a unique identifier for that job to your task with the startedBy
* parameter. You can then identify which tasks belong to that job by filtering the results of a ListTasks
* call with the startedBy
value. Up to 36 letters (uppercase and lowercase), numbers, hyphens (-), and
* underscores (_) are allowed.
*
*
* If a task is started by an Amazon ECS service, the startedBy
parameter contains the deployment ID of
* the service that starts it.
*
*
* @return An optional tag specified when a task is started. For example, if you automatically trigger a task to run
* a batch process job, you could apply a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks belong to that job by filtering the
* results of a ListTasks call with the startedBy
value. Up to 36 letters (uppercase and
* lowercase), numbers, hyphens (-), and underscores (_) are allowed.
*
* If a task is started by an Amazon ECS service, the startedBy
parameter contains the
* deployment ID of the service that starts it.
*/
public final String startedBy() {
return startedBy;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete
* tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource
* limit.
*
*
*/
public final List tags() {
return tags;
}
/**
*
* The family
and revision
(family:revision
) or full ARN of the task
* definition to start. If a revision
isn't specified, the latest ACTIVE
revision is used.
*
*
* @return The family
and revision
(family:revision
) or full ARN of the task
* definition to start. If a revision
isn't specified, the latest ACTIVE
revision
* is used.
*/
public final String taskDefinition() {
return taskDefinition;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(hasContainerInstances() ? containerInstances() : null);
hashCode = 31 * hashCode + Objects.hashCode(enableECSManagedTags());
hashCode = 31 * hashCode + Objects.hashCode(enableExecuteCommand());
hashCode = 31 * hashCode + Objects.hashCode(group());
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(overrides());
hashCode = 31 * hashCode + Objects.hashCode(propagateTagsAsString());
hashCode = 31 * hashCode + Objects.hashCode(referenceId());
hashCode = 31 * hashCode + Objects.hashCode(startedBy());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartTaskRequest)) {
return false;
}
StartTaskRequest other = (StartTaskRequest) obj;
return Objects.equals(cluster(), other.cluster()) && hasContainerInstances() == other.hasContainerInstances()
&& Objects.equals(containerInstances(), other.containerInstances())
&& Objects.equals(enableECSManagedTags(), other.enableECSManagedTags())
&& Objects.equals(enableExecuteCommand(), other.enableExecuteCommand()) && Objects.equals(group(), other.group())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& Objects.equals(overrides(), other.overrides())
&& Objects.equals(propagateTagsAsString(), other.propagateTagsAsString())
&& Objects.equals(referenceId(), other.referenceId()) && Objects.equals(startedBy(), other.startedBy())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(taskDefinition(), other.taskDefinition());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartTaskRequest").add("Cluster", cluster())
.add("ContainerInstances", hasContainerInstances() ? containerInstances() : null)
.add("EnableECSManagedTags", enableECSManagedTags()).add("EnableExecuteCommand", enableExecuteCommand())
.add("Group", group()).add("NetworkConfiguration", networkConfiguration()).add("Overrides", overrides())
.add("PropagateTags", propagateTagsAsString()).add("ReferenceId", referenceId()).add("StartedBy", startedBy())
.add("Tags", hasTags() ? tags() : null).add("TaskDefinition", taskDefinition()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "containerInstances":
return Optional.ofNullable(clazz.cast(containerInstances()));
case "enableECSManagedTags":
return Optional.ofNullable(clazz.cast(enableECSManagedTags()));
case "enableExecuteCommand":
return Optional.ofNullable(clazz.cast(enableExecuteCommand()));
case "group":
return Optional.ofNullable(clazz.cast(group()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "overrides":
return Optional.ofNullable(clazz.cast(overrides()));
case "propagateTags":
return Optional.ofNullable(clazz.cast(propagateTagsAsString()));
case "referenceId":
return Optional.ofNullable(clazz.cast(referenceId()));
case "startedBy":
return Optional.ofNullable(clazz.cast(startedBy()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function