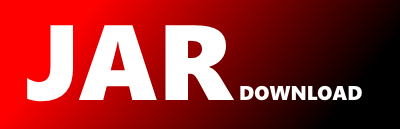
software.amazon.awssdk.services.ecs.model.Container Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A Docker container that's part of a task.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Container implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CONTAINER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerArn").getter(getter(Container::containerArn)).setter(setter(Builder::containerArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerArn").build()).build();
private static final SdkField TASK_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskArn").getter(getter(Container::taskArn)).setter(setter(Builder::taskArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskArn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Container::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField IMAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("image")
.getter(getter(Container::image)).setter(setter(Builder::image))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("image").build()).build();
private static final SdkField IMAGE_DIGEST_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("imageDigest").getter(getter(Container::imageDigest)).setter(setter(Builder::imageDigest))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imageDigest").build()).build();
private static final SdkField RUNTIME_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("runtimeId").getter(getter(Container::runtimeId)).setter(setter(Builder::runtimeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runtimeId").build()).build();
private static final SdkField LAST_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("lastStatus").getter(getter(Container::lastStatus)).setter(setter(Builder::lastStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastStatus").build()).build();
private static final SdkField EXIT_CODE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("exitCode").getter(getter(Container::exitCode)).setter(setter(Builder::exitCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("exitCode").build()).build();
private static final SdkField REASON_FIELD = SdkField. builder(MarshallingType.STRING).memberName("reason")
.getter(getter(Container::reason)).setter(setter(Builder::reason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("reason").build()).build();
private static final SdkField> NETWORK_BINDINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("networkBindings")
.getter(getter(Container::networkBindings))
.setter(setter(Builder::networkBindings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkBindings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NetworkBinding::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> NETWORK_INTERFACES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("networkInterfaces")
.getter(getter(Container::networkInterfaces))
.setter(setter(Builder::networkInterfaces))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkInterfaces").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(NetworkInterface::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField HEALTH_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("healthStatus").getter(getter(Container::healthStatusAsString)).setter(setter(Builder::healthStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("healthStatus").build()).build();
private static final SdkField> MANAGED_AGENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("managedAgents")
.getter(getter(Container::managedAgents))
.setter(setter(Builder::managedAgents))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("managedAgents").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ManagedAgent::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CPU_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cpu")
.getter(getter(Container::cpu)).setter(setter(Builder::cpu))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cpu").build()).build();
private static final SdkField MEMORY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("memory")
.getter(getter(Container::memory)).setter(setter(Builder::memory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memory").build()).build();
private static final SdkField MEMORY_RESERVATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("memoryReservation").getter(getter(Container::memoryReservation))
.setter(setter(Builder::memoryReservation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memoryReservation").build()).build();
private static final SdkField> GPU_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("gpuIds")
.getter(getter(Container::gpuIds))
.setter(setter(Builder::gpuIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("gpuIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTAINER_ARN_FIELD,
TASK_ARN_FIELD, NAME_FIELD, IMAGE_FIELD, IMAGE_DIGEST_FIELD, RUNTIME_ID_FIELD, LAST_STATUS_FIELD, EXIT_CODE_FIELD,
REASON_FIELD, NETWORK_BINDINGS_FIELD, NETWORK_INTERFACES_FIELD, HEALTH_STATUS_FIELD, MANAGED_AGENTS_FIELD, CPU_FIELD,
MEMORY_FIELD, MEMORY_RESERVATION_FIELD, GPU_IDS_FIELD));
private static final long serialVersionUID = 1L;
private final String containerArn;
private final String taskArn;
private final String name;
private final String image;
private final String imageDigest;
private final String runtimeId;
private final String lastStatus;
private final Integer exitCode;
private final String reason;
private final List networkBindings;
private final List networkInterfaces;
private final String healthStatus;
private final List managedAgents;
private final String cpu;
private final String memory;
private final String memoryReservation;
private final List gpuIds;
private Container(BuilderImpl builder) {
this.containerArn = builder.containerArn;
this.taskArn = builder.taskArn;
this.name = builder.name;
this.image = builder.image;
this.imageDigest = builder.imageDigest;
this.runtimeId = builder.runtimeId;
this.lastStatus = builder.lastStatus;
this.exitCode = builder.exitCode;
this.reason = builder.reason;
this.networkBindings = builder.networkBindings;
this.networkInterfaces = builder.networkInterfaces;
this.healthStatus = builder.healthStatus;
this.managedAgents = builder.managedAgents;
this.cpu = builder.cpu;
this.memory = builder.memory;
this.memoryReservation = builder.memoryReservation;
this.gpuIds = builder.gpuIds;
}
/**
*
* The Amazon Resource Name (ARN) of the container.
*
*
* @return The Amazon Resource Name (ARN) of the container.
*/
public final String containerArn() {
return containerArn;
}
/**
*
* The ARN of the task.
*
*
* @return The ARN of the task.
*/
public final String taskArn() {
return taskArn;
}
/**
*
* The name of the container.
*
*
* @return The name of the container.
*/
public final String name() {
return name;
}
/**
*
* The image used for the container.
*
*
* @return The image used for the container.
*/
public final String image() {
return image;
}
/**
*
* The container image manifest digest.
*
*
* @return The container image manifest digest.
*/
public final String imageDigest() {
return imageDigest;
}
/**
*
* The ID of the Docker container.
*
*
* @return The ID of the Docker container.
*/
public final String runtimeId() {
return runtimeId;
}
/**
*
* The last known status of the container.
*
*
* @return The last known status of the container.
*/
public final String lastStatus() {
return lastStatus;
}
/**
*
* The exit code returned from the container.
*
*
* @return The exit code returned from the container.
*/
public final Integer exitCode() {
return exitCode;
}
/**
*
* A short (255 max characters) human-readable string to provide additional details about a running or stopped
* container.
*
*
* @return A short (255 max characters) human-readable string to provide additional details about a running or
* stopped container.
*/
public final String reason() {
return reason;
}
/**
* For responses, this returns true if the service returned a value for the NetworkBindings property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNetworkBindings() {
return networkBindings != null && !(networkBindings instanceof SdkAutoConstructList);
}
/**
*
* The network bindings associated with the container.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNetworkBindings} method.
*
*
* @return The network bindings associated with the container.
*/
public final List networkBindings() {
return networkBindings;
}
/**
* For responses, this returns true if the service returned a value for the NetworkInterfaces property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasNetworkInterfaces() {
return networkInterfaces != null && !(networkInterfaces instanceof SdkAutoConstructList);
}
/**
*
* The network interfaces associated with the container.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasNetworkInterfaces} method.
*
*
* @return The network interfaces associated with the container.
*/
public final List networkInterfaces() {
return networkInterfaces;
}
/**
*
* The health status of the container. If health checks aren't configured for this container in its task definition,
* then it reports the health status as UNKNOWN
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #healthStatus} will
* return {@link HealthStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #healthStatusAsString}.
*
*
* @return The health status of the container. If health checks aren't configured for this container in its task
* definition, then it reports the health status as UNKNOWN
.
* @see HealthStatus
*/
public final HealthStatus healthStatus() {
return HealthStatus.fromValue(healthStatus);
}
/**
*
* The health status of the container. If health checks aren't configured for this container in its task definition,
* then it reports the health status as UNKNOWN
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #healthStatus} will
* return {@link HealthStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #healthStatusAsString}.
*
*
* @return The health status of the container. If health checks aren't configured for this container in its task
* definition, then it reports the health status as UNKNOWN
.
* @see HealthStatus
*/
public final String healthStatusAsString() {
return healthStatus;
}
/**
* For responses, this returns true if the service returned a value for the ManagedAgents property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasManagedAgents() {
return managedAgents != null && !(managedAgents instanceof SdkAutoConstructList);
}
/**
*
* The details of any Amazon ECS managed agents associated with the container.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasManagedAgents} method.
*
*
* @return The details of any Amazon ECS managed agents associated with the container.
*/
public final List managedAgents() {
return managedAgents;
}
/**
*
* The number of CPU units set for the container. The value is 0
if no value was specified in the
* container definition when the task definition was registered.
*
*
* @return The number of CPU units set for the container. The value is 0
if no value was specified in
* the container definition when the task definition was registered.
*/
public final String cpu() {
return cpu;
}
/**
*
* The hard limit (in MiB) of memory set for the container.
*
*
* @return The hard limit (in MiB) of memory set for the container.
*/
public final String memory() {
return memory;
}
/**
*
* The soft limit (in MiB) of memory set for the container.
*
*
* @return The soft limit (in MiB) of memory set for the container.
*/
public final String memoryReservation() {
return memoryReservation;
}
/**
* For responses, this returns true if the service returned a value for the GpuIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasGpuIds() {
return gpuIds != null && !(gpuIds instanceof SdkAutoConstructList);
}
/**
*
* The IDs of each GPU assigned to the container.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasGpuIds} method.
*
*
* @return The IDs of each GPU assigned to the container.
*/
public final List gpuIds() {
return gpuIds;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(containerArn());
hashCode = 31 * hashCode + Objects.hashCode(taskArn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(image());
hashCode = 31 * hashCode + Objects.hashCode(imageDigest());
hashCode = 31 * hashCode + Objects.hashCode(runtimeId());
hashCode = 31 * hashCode + Objects.hashCode(lastStatus());
hashCode = 31 * hashCode + Objects.hashCode(exitCode());
hashCode = 31 * hashCode + Objects.hashCode(reason());
hashCode = 31 * hashCode + Objects.hashCode(hasNetworkBindings() ? networkBindings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasNetworkInterfaces() ? networkInterfaces() : null);
hashCode = 31 * hashCode + Objects.hashCode(healthStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasManagedAgents() ? managedAgents() : null);
hashCode = 31 * hashCode + Objects.hashCode(cpu());
hashCode = 31 * hashCode + Objects.hashCode(memory());
hashCode = 31 * hashCode + Objects.hashCode(memoryReservation());
hashCode = 31 * hashCode + Objects.hashCode(hasGpuIds() ? gpuIds() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Container)) {
return false;
}
Container other = (Container) obj;
return Objects.equals(containerArn(), other.containerArn()) && Objects.equals(taskArn(), other.taskArn())
&& Objects.equals(name(), other.name()) && Objects.equals(image(), other.image())
&& Objects.equals(imageDigest(), other.imageDigest()) && Objects.equals(runtimeId(), other.runtimeId())
&& Objects.equals(lastStatus(), other.lastStatus()) && Objects.equals(exitCode(), other.exitCode())
&& Objects.equals(reason(), other.reason()) && hasNetworkBindings() == other.hasNetworkBindings()
&& Objects.equals(networkBindings(), other.networkBindings())
&& hasNetworkInterfaces() == other.hasNetworkInterfaces()
&& Objects.equals(networkInterfaces(), other.networkInterfaces())
&& Objects.equals(healthStatusAsString(), other.healthStatusAsString())
&& hasManagedAgents() == other.hasManagedAgents() && Objects.equals(managedAgents(), other.managedAgents())
&& Objects.equals(cpu(), other.cpu()) && Objects.equals(memory(), other.memory())
&& Objects.equals(memoryReservation(), other.memoryReservation()) && hasGpuIds() == other.hasGpuIds()
&& Objects.equals(gpuIds(), other.gpuIds());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Container").add("ContainerArn", containerArn()).add("TaskArn", taskArn()).add("Name", name())
.add("Image", image()).add("ImageDigest", imageDigest()).add("RuntimeId", runtimeId())
.add("LastStatus", lastStatus()).add("ExitCode", exitCode()).add("Reason", reason())
.add("NetworkBindings", hasNetworkBindings() ? networkBindings() : null)
.add("NetworkInterfaces", hasNetworkInterfaces() ? networkInterfaces() : null)
.add("HealthStatus", healthStatusAsString()).add("ManagedAgents", hasManagedAgents() ? managedAgents() : null)
.add("Cpu", cpu()).add("Memory", memory()).add("MemoryReservation", memoryReservation())
.add("GpuIds", hasGpuIds() ? gpuIds() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "containerArn":
return Optional.ofNullable(clazz.cast(containerArn()));
case "taskArn":
return Optional.ofNullable(clazz.cast(taskArn()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "image":
return Optional.ofNullable(clazz.cast(image()));
case "imageDigest":
return Optional.ofNullable(clazz.cast(imageDigest()));
case "runtimeId":
return Optional.ofNullable(clazz.cast(runtimeId()));
case "lastStatus":
return Optional.ofNullable(clazz.cast(lastStatus()));
case "exitCode":
return Optional.ofNullable(clazz.cast(exitCode()));
case "reason":
return Optional.ofNullable(clazz.cast(reason()));
case "networkBindings":
return Optional.ofNullable(clazz.cast(networkBindings()));
case "networkInterfaces":
return Optional.ofNullable(clazz.cast(networkInterfaces()));
case "healthStatus":
return Optional.ofNullable(clazz.cast(healthStatusAsString()));
case "managedAgents":
return Optional.ofNullable(clazz.cast(managedAgents()));
case "cpu":
return Optional.ofNullable(clazz.cast(cpu()));
case "memory":
return Optional.ofNullable(clazz.cast(memory()));
case "memoryReservation":
return Optional.ofNullable(clazz.cast(memoryReservation()));
case "gpuIds":
return Optional.ofNullable(clazz.cast(gpuIds()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function