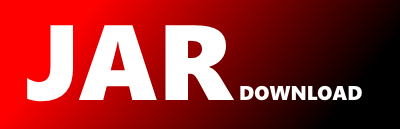
software.amazon.awssdk.services.ecs.model.ManagedAgentStateChange Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object representing a change in state for a managed agent.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ManagedAgentStateChange implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CONTAINER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerName").getter(getter(ManagedAgentStateChange::containerName))
.setter(setter(Builder::containerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerName").build()).build();
private static final SdkField MANAGED_AGENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("managedAgentName").getter(getter(ManagedAgentStateChange::managedAgentNameAsString))
.setter(setter(Builder::managedAgentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("managedAgentName").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(ManagedAgentStateChange::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField REASON_FIELD = SdkField. builder(MarshallingType.STRING).memberName("reason")
.getter(getter(ManagedAgentStateChange::reason)).setter(setter(Builder::reason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("reason").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTAINER_NAME_FIELD,
MANAGED_AGENT_NAME_FIELD, STATUS_FIELD, REASON_FIELD));
private static final long serialVersionUID = 1L;
private final String containerName;
private final String managedAgentName;
private final String status;
private final String reason;
private ManagedAgentStateChange(BuilderImpl builder) {
this.containerName = builder.containerName;
this.managedAgentName = builder.managedAgentName;
this.status = builder.status;
this.reason = builder.reason;
}
/**
*
* The name of the container that's associated with the managed agent.
*
*
* @return The name of the container that's associated with the managed agent.
*/
public final String containerName() {
return containerName;
}
/**
*
* The name of the managed agent.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #managedAgentName}
* will return {@link ManagedAgentName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #managedAgentNameAsString}.
*
*
* @return The name of the managed agent.
* @see ManagedAgentName
*/
public final ManagedAgentName managedAgentName() {
return ManagedAgentName.fromValue(managedAgentName);
}
/**
*
* The name of the managed agent.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #managedAgentName}
* will return {@link ManagedAgentName#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #managedAgentNameAsString}.
*
*
* @return The name of the managed agent.
* @see ManagedAgentName
*/
public final String managedAgentNameAsString() {
return managedAgentName;
}
/**
*
* The status of the managed agent.
*
*
* @return The status of the managed agent.
*/
public final String status() {
return status;
}
/**
*
* The reason for the status of the managed agent.
*
*
* @return The reason for the status of the managed agent.
*/
public final String reason() {
return reason;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(containerName());
hashCode = 31 * hashCode + Objects.hashCode(managedAgentNameAsString());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(reason());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ManagedAgentStateChange)) {
return false;
}
ManagedAgentStateChange other = (ManagedAgentStateChange) obj;
return Objects.equals(containerName(), other.containerName())
&& Objects.equals(managedAgentNameAsString(), other.managedAgentNameAsString())
&& Objects.equals(status(), other.status()) && Objects.equals(reason(), other.reason());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ManagedAgentStateChange").add("ContainerName", containerName())
.add("ManagedAgentName", managedAgentNameAsString()).add("Status", status()).add("Reason", reason()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "containerName":
return Optional.ofNullable(clazz.cast(containerName()));
case "managedAgentName":
return Optional.ofNullable(clazz.cast(managedAgentNameAsString()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "reason":
return Optional.ofNullable(clazz.cast(reason()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function