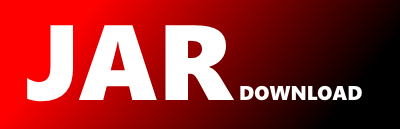
software.amazon.awssdk.services.ecs.model.PortMapping Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Port mappings allow containers to access ports on the host container instance to send or receive traffic. Port
* mappings are specified as part of the container definition.
*
*
* If you use containers in a task with the awsvpc
or host
network mode, specify the exposed
* ports using containerPort
. The hostPort
can be left blank or it must be the same value as
* the containerPort
.
*
*
* Most fields of this parameter (containerPort
, hostPort
, protocol
) maps to
* PortBindings
in the Create
* a container section of the Docker Remote API and the
* --publish
option to
* docker run
. If the network mode of a task definition is set to host
, host ports must
* either be undefined or match the container port in the port mapping.
*
*
*
* You can't expose the same container port for multiple protocols. If you attempt this, an error is returned.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port assignments are
* visible in the networkBindings
section of DescribeTasks API responses.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PortMapping implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CONTAINER_PORT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("containerPort").getter(getter(PortMapping::containerPort)).setter(setter(Builder::containerPort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerPort").build()).build();
private static final SdkField HOST_PORT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("hostPort").getter(getter(PortMapping::hostPort)).setter(setter(Builder::hostPort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("hostPort").build()).build();
private static final SdkField PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("protocol").getter(getter(PortMapping::protocolAsString)).setter(setter(Builder::protocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("protocol").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(PortMapping::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField APP_PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("appProtocol").getter(getter(PortMapping::appProtocolAsString)).setter(setter(Builder::appProtocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("appProtocol").build()).build();
private static final SdkField CONTAINER_PORT_RANGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerPortRange").getter(getter(PortMapping::containerPortRange))
.setter(setter(Builder::containerPortRange))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerPortRange").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTAINER_PORT_FIELD,
HOST_PORT_FIELD, PROTOCOL_FIELD, NAME_FIELD, APP_PROTOCOL_FIELD, CONTAINER_PORT_RANGE_FIELD));
private static final long serialVersionUID = 1L;
private final Integer containerPort;
private final Integer hostPort;
private final String protocol;
private final String name;
private final String appProtocol;
private final String containerPortRange;
private PortMapping(BuilderImpl builder) {
this.containerPort = builder.containerPort;
this.hostPort = builder.hostPort;
this.protocol = builder.protocol;
this.name = builder.name;
this.appProtocol = builder.appProtocol;
this.containerPortRange = builder.containerPortRange;
}
/**
*
* The port number on the container that's bound to the user-specified or automatically assigned host port.
*
*
* If you use containers in a task with the awsvpc
or host
network mode, specify the
* exposed ports using containerPort
.
*
*
* If you use containers in a task with the bridge
network mode and you specify a container port and
* not a host port, your container automatically receives a host port in the ephemeral port range. For more
* information, see hostPort
. Port mappings that are automatically assigned in this way do not count
* toward the 100 reserved ports limit of a container instance.
*
*
* @return The port number on the container that's bound to the user-specified or automatically assigned host
* port.
*
* If you use containers in a task with the awsvpc
or host
network mode, specify
* the exposed ports using containerPort
.
*
*
* If you use containers in a task with the bridge
network mode and you specify a container
* port and not a host port, your container automatically receives a host port in the ephemeral port range.
* For more information, see hostPort
. Port mappings that are automatically assigned in this
* way do not count toward the 100 reserved ports limit of a container instance.
*/
public final Integer containerPort() {
return containerPort;
}
/**
*
* The port number on the container instance to reserve for your container.
*
*
* If you specify a containerPortRange
, leave this field empty and the value of the
* hostPort
is set as follows:
*
*
* -
*
* For containers in a task with the awsvpc
network mode, the hostPort
is set to the same
* value as the containerPort
. This is a static mapping strategy.
*
*
* -
*
* For containers in a task with the bridge
network mode, the Amazon ECS agent finds open ports on the
* host and automatically binds them to the container ports. This is a dynamic mapping strategy.
*
*
*
*
* If you use containers in a task with the awsvpc
or host
network mode, the
* hostPort
can either be left blank or set to the same value as the containerPort
.
*
*
* If you use containers in a task with the bridge
network mode, you can specify a non-reserved host
* port for your container port mapping, or you can omit the hostPort
(or set it to 0
)
* while specifying a containerPort
and your container automatically receives a port in the ephemeral
* port range for your container instance operating system and Docker version.
*
*
* The default ephemeral port range for Docker version 1.6.0 and later is listed on the instance under
* /proc/sys/net/ipv4/ip_local_port_range
. If this kernel parameter is unavailable, the default
* ephemeral port range from 49153 through 65535 is used. Do not attempt to specify a host port in the ephemeral
* port range as these are reserved for automatic assignment. In general, ports below 32768 are outside of the
* ephemeral port range.
*
*
* The default reserved ports are 22 for SSH, the Docker ports 2375 and 2376, and the Amazon ECS container agent
* ports 51678-51680. Any host port that was previously specified in a running task is also reserved while the task
* is running. That is, after a task stops, the host port is released. The current reserved ports are displayed in
* the remainingResources
of DescribeContainerInstances output. A container instance can have up to 100 reserved ports at a time. This
* number includes the default reserved ports. Automatically assigned ports aren't included in the 100 reserved
* ports quota.
*
*
* @return The port number on the container instance to reserve for your container.
*
* If you specify a containerPortRange
, leave this field empty and the value of the
* hostPort
is set as follows:
*
*
* -
*
* For containers in a task with the awsvpc
network mode, the hostPort
is set to
* the same value as the containerPort
. This is a static mapping strategy.
*
*
* -
*
* For containers in a task with the bridge
network mode, the Amazon ECS agent finds open ports
* on the host and automatically binds them to the container ports. This is a dynamic mapping strategy.
*
*
*
*
* If you use containers in a task with the awsvpc
or host
network mode, the
* hostPort
can either be left blank or set to the same value as the containerPort
* .
*
*
* If you use containers in a task with the bridge
network mode, you can specify a non-reserved
* host port for your container port mapping, or you can omit the hostPort
(or set it to
* 0
) while specifying a containerPort
and your container automatically receives a
* port in the ephemeral port range for your container instance operating system and Docker version.
*
*
* The default ephemeral port range for Docker version 1.6.0 and later is listed on the instance under
* /proc/sys/net/ipv4/ip_local_port_range
. If this kernel parameter is unavailable, the default
* ephemeral port range from 49153 through 65535 is used. Do not attempt to specify a host port in the
* ephemeral port range as these are reserved for automatic assignment. In general, ports below 32768 are
* outside of the ephemeral port range.
*
*
* The default reserved ports are 22 for SSH, the Docker ports 2375 and 2376, and the Amazon ECS container
* agent ports 51678-51680. Any host port that was previously specified in a running task is also reserved
* while the task is running. That is, after a task stops, the host port is released. The current reserved
* ports are displayed in the remainingResources
of DescribeContainerInstances output. A container instance can have up to 100 reserved ports at a time.
* This number includes the default reserved ports. Automatically assigned ports aren't included in the 100
* reserved ports quota.
*/
public final Integer hostPort() {
return hostPort;
}
/**
*
* The protocol used for the port mapping. Valid values are tcp
and udp
. The default is
* tcp
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #protocol} will
* return {@link TransportProtocol#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #protocolAsString}.
*
*
* @return The protocol used for the port mapping. Valid values are tcp
and udp
. The
* default is tcp
.
* @see TransportProtocol
*/
public final TransportProtocol protocol() {
return TransportProtocol.fromValue(protocol);
}
/**
*
* The protocol used for the port mapping. Valid values are tcp
and udp
. The default is
* tcp
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #protocol} will
* return {@link TransportProtocol#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #protocolAsString}.
*
*
* @return The protocol used for the port mapping. Valid values are tcp
and udp
. The
* default is tcp
.
* @see TransportProtocol
*/
public final String protocolAsString() {
return protocol;
}
/**
*
* The name that's used for the port mapping. This parameter only applies to Service Connect. This parameter is the
* name that you use in the serviceConnectConfiguration
of a service. The name can include up to 64
* characters. The characters can include lowercase letters, numbers, underscores (_), and hyphens (-). The name
* can't start with a hyphen.
*
*
* For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The name that's used for the port mapping. This parameter only applies to Service Connect. This parameter
* is the name that you use in the serviceConnectConfiguration
of a service. The name can
* include up to 64 characters. The characters can include lowercase letters, numbers, underscores (_), and
* hyphens (-). The name can't start with a hyphen.
*
* For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
*/
public final String name() {
return name;
}
/**
*
* The application protocol that's used for the port mapping. This parameter only applies to Service Connect. We
* recommend that you set this parameter to be consistent with the protocol that your application uses. If you set
* this parameter, Amazon ECS adds protocol-specific connection handling to the Service Connect proxy. If you set
* this parameter, Amazon ECS adds protocol-specific telemetry in the Amazon ECS console and CloudWatch.
*
*
* If you don't set a value for this parameter, then TCP is used. However, Amazon ECS doesn't add protocol-specific
* telemetry for TCP.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #appProtocol} will
* return {@link ApplicationProtocol#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #appProtocolAsString}.
*
*
* @return The application protocol that's used for the port mapping. This parameter only applies to Service
* Connect. We recommend that you set this parameter to be consistent with the protocol that your
* application uses. If you set this parameter, Amazon ECS adds protocol-specific connection handling to the
* Service Connect proxy. If you set this parameter, Amazon ECS adds protocol-specific telemetry in the
* Amazon ECS console and CloudWatch.
*
* If you don't set a value for this parameter, then TCP is used. However, Amazon ECS doesn't add
* protocol-specific telemetry for TCP.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
* @see ApplicationProtocol
*/
public final ApplicationProtocol appProtocol() {
return ApplicationProtocol.fromValue(appProtocol);
}
/**
*
* The application protocol that's used for the port mapping. This parameter only applies to Service Connect. We
* recommend that you set this parameter to be consistent with the protocol that your application uses. If you set
* this parameter, Amazon ECS adds protocol-specific connection handling to the Service Connect proxy. If you set
* this parameter, Amazon ECS adds protocol-specific telemetry in the Amazon ECS console and CloudWatch.
*
*
* If you don't set a value for this parameter, then TCP is used. However, Amazon ECS doesn't add protocol-specific
* telemetry for TCP.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #appProtocol} will
* return {@link ApplicationProtocol#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #appProtocolAsString}.
*
*
* @return The application protocol that's used for the port mapping. This parameter only applies to Service
* Connect. We recommend that you set this parameter to be consistent with the protocol that your
* application uses. If you set this parameter, Amazon ECS adds protocol-specific connection handling to the
* Service Connect proxy. If you set this parameter, Amazon ECS adds protocol-specific telemetry in the
* Amazon ECS console and CloudWatch.
*
* If you don't set a value for this parameter, then TCP is used. However, Amazon ECS doesn't add
* protocol-specific telemetry for TCP.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
* @see ApplicationProtocol
*/
public final String appProtocolAsString() {
return appProtocol;
}
/**
*
* The port number range on the container that's bound to the dynamically mapped host port range.
*
*
* The following rules apply when you specify a containerPortRange
:
*
*
* -
*
* You must use either the bridge
network mode or the awsvpc
network mode.
*
*
* -
*
* This parameter is available for both the EC2 and Fargate launch types.
*
*
* -
*
* This parameter is available for both the Linux and Windows operating systems.
*
*
* -
*
* The container instance must have at least version 1.67.0 of the container agent and at least version 1.67.0-1 of
* the ecs-init
package
*
*
* -
*
* You can specify a maximum of 100 port ranges per container.
*
*
* -
*
* You do not specify a hostPortRange
. The value of the hostPortRange
is set as follows:
*
*
* -
*
* For containers in a task with the awsvpc
network mode, the hostPort
is set to the same
* value as the containerPort
. This is a static mapping strategy.
*
*
* -
*
* For containers in a task with the bridge
network mode, the Amazon ECS agent finds open host ports
* from the default ephemeral range and passes it to docker to bind them to the container ports.
*
*
*
*
* -
*
* The containerPortRange
valid values are between 1 and 65535.
*
*
* -
*
* A port can only be included in one port mapping per container.
*
*
* -
*
* You cannot specify overlapping port ranges.
*
*
* -
*
* The first port in the range must be less than last port in the range.
*
*
* -
*
* Docker recommends that you turn off the docker-proxy in the Docker daemon config file when you have a large
* number of ports.
*
*
* For more information, see Issue #11185 on the Github
* website.
*
*
* For information about how to turn off the docker-proxy in the Docker daemon config file, see Docker daemon in the Amazon ECS Developer Guide.
*
*
*
*
* You can call
* DescribeTasks
to view the hostPortRange
which are the host ports that are bound to
* the container ports.
*
*
* @return The port number range on the container that's bound to the dynamically mapped host port range.
*
* The following rules apply when you specify a containerPortRange
:
*
*
* -
*
* You must use either the bridge
network mode or the awsvpc
network mode.
*
*
* -
*
* This parameter is available for both the EC2 and Fargate launch types.
*
*
* -
*
* This parameter is available for both the Linux and Windows operating systems.
*
*
* -
*
* The container instance must have at least version 1.67.0 of the container agent and at least version
* 1.67.0-1 of the ecs-init
package
*
*
* -
*
* You can specify a maximum of 100 port ranges per container.
*
*
* -
*
* You do not specify a hostPortRange
. The value of the hostPortRange
is set as
* follows:
*
*
* -
*
* For containers in a task with the awsvpc
network mode, the hostPort
is set to
* the same value as the containerPort
. This is a static mapping strategy.
*
*
* -
*
* For containers in a task with the bridge
network mode, the Amazon ECS agent finds open host
* ports from the default ephemeral range and passes it to docker to bind them to the container ports.
*
*
*
*
* -
*
* The containerPortRange
valid values are between 1 and 65535.
*
*
* -
*
* A port can only be included in one port mapping per container.
*
*
* -
*
* You cannot specify overlapping port ranges.
*
*
* -
*
* The first port in the range must be less than last port in the range.
*
*
* -
*
* Docker recommends that you turn off the docker-proxy in the Docker daemon config file when you have a
* large number of ports.
*
*
* For more information, see Issue #11185 on the
* Github website.
*
*
* For information about how to turn off the docker-proxy in the Docker daemon config file, see Docker daemon in the Amazon ECS Developer Guide.
*
*
*
*
* You can call
* DescribeTasks
to view the hostPortRange
which are the host ports that are
* bound to the container ports.
*/
public final String containerPortRange() {
return containerPortRange;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(containerPort());
hashCode = 31 * hashCode + Objects.hashCode(hostPort());
hashCode = 31 * hashCode + Objects.hashCode(protocolAsString());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(appProtocolAsString());
hashCode = 31 * hashCode + Objects.hashCode(containerPortRange());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PortMapping)) {
return false;
}
PortMapping other = (PortMapping) obj;
return Objects.equals(containerPort(), other.containerPort()) && Objects.equals(hostPort(), other.hostPort())
&& Objects.equals(protocolAsString(), other.protocolAsString()) && Objects.equals(name(), other.name())
&& Objects.equals(appProtocolAsString(), other.appProtocolAsString())
&& Objects.equals(containerPortRange(), other.containerPortRange());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PortMapping").add("ContainerPort", containerPort()).add("HostPort", hostPort())
.add("Protocol", protocolAsString()).add("Name", name()).add("AppProtocol", appProtocolAsString())
.add("ContainerPortRange", containerPortRange()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "containerPort":
return Optional.ofNullable(clazz.cast(containerPort()));
case "hostPort":
return Optional.ofNullable(clazz.cast(hostPort()));
case "protocol":
return Optional.ofNullable(clazz.cast(protocolAsString()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "appProtocol":
return Optional.ofNullable(clazz.cast(appProtocolAsString()));
case "containerPortRange":
return Optional.ofNullable(clazz.cast(containerPortRange()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If you use containers in a task with the awsvpc
or host
network mode,
* specify the exposed ports using containerPort
.
*
*
* If you use containers in a task with the bridge
network mode and you specify a container
* port and not a host port, your container automatically receives a host port in the ephemeral port
* range. For more information, see hostPort
. Port mappings that are automatically assigned
* in this way do not count toward the 100 reserved ports limit of a container instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder containerPort(Integer containerPort);
/**
*
* The port number on the container instance to reserve for your container.
*
*
* If you specify a containerPortRange
, leave this field empty and the value of the
* hostPort
is set as follows:
*
*
* -
*
* For containers in a task with the awsvpc
network mode, the hostPort
is set to the
* same value as the containerPort
. This is a static mapping strategy.
*
*
* -
*
* For containers in a task with the bridge
network mode, the Amazon ECS agent finds open ports on
* the host and automatically binds them to the container ports. This is a dynamic mapping strategy.
*
*
*
*
* If you use containers in a task with the awsvpc
or host
network mode, the
* hostPort
can either be left blank or set to the same value as the containerPort
.
*
*
* If you use containers in a task with the bridge
network mode, you can specify a non-reserved
* host port for your container port mapping, or you can omit the hostPort
(or set it to
* 0
) while specifying a containerPort
and your container automatically receives a
* port in the ephemeral port range for your container instance operating system and Docker version.
*
*
* The default ephemeral port range for Docker version 1.6.0 and later is listed on the instance under
* /proc/sys/net/ipv4/ip_local_port_range
. If this kernel parameter is unavailable, the default
* ephemeral port range from 49153 through 65535 is used. Do not attempt to specify a host port in the ephemeral
* port range as these are reserved for automatic assignment. In general, ports below 32768 are outside of the
* ephemeral port range.
*
*
* The default reserved ports are 22 for SSH, the Docker ports 2375 and 2376, and the Amazon ECS container agent
* ports 51678-51680. Any host port that was previously specified in a running task is also reserved while the
* task is running. That is, after a task stops, the host port is released. The current reserved ports are
* displayed in the remainingResources
of DescribeContainerInstances output. A container instance can have up to 100 reserved ports at a time.
* This number includes the default reserved ports. Automatically assigned ports aren't included in the 100
* reserved ports quota.
*
*
* @param hostPort
* The port number on the container instance to reserve for your container.
*
* If you specify a containerPortRange
, leave this field empty and the value of the
* hostPort
is set as follows:
*
*
* -
*
* For containers in a task with the awsvpc
network mode, the hostPort
is set
* to the same value as the containerPort
. This is a static mapping strategy.
*
*
* -
*
* For containers in a task with the bridge
network mode, the Amazon ECS agent finds open
* ports on the host and automatically binds them to the container ports. This is a dynamic mapping
* strategy.
*
*
*
*
* If you use containers in a task with the awsvpc
or host
network mode, the
* hostPort
can either be left blank or set to the same value as the
* containerPort
.
*
*
* If you use containers in a task with the bridge
network mode, you can specify a
* non-reserved host port for your container port mapping, or you can omit the hostPort
(or
* set it to 0
) while specifying a containerPort
and your container
* automatically receives a port in the ephemeral port range for your container instance operating system
* and Docker version.
*
*
* The default ephemeral port range for Docker version 1.6.0 and later is listed on the instance under
* /proc/sys/net/ipv4/ip_local_port_range
. If this kernel parameter is unavailable, the
* default ephemeral port range from 49153 through 65535 is used. Do not attempt to specify a host port
* in the ephemeral port range as these are reserved for automatic assignment. In general, ports below
* 32768 are outside of the ephemeral port range.
*
*
* The default reserved ports are 22 for SSH, the Docker ports 2375 and 2376, and the Amazon ECS
* container agent ports 51678-51680. Any host port that was previously specified in a running task is
* also reserved while the task is running. That is, after a task stops, the host port is released. The
* current reserved ports are displayed in the remainingResources
of DescribeContainerInstances output. A container instance can have up to 100 reserved ports at a
* time. This number includes the default reserved ports. Automatically assigned ports aren't included in
* the 100 reserved ports quota.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder hostPort(Integer hostPort);
/**
*
* The protocol used for the port mapping. Valid values are tcp
and udp
. The default
* is tcp
.
*
*
* @param protocol
* The protocol used for the port mapping. Valid values are tcp
and udp
. The
* default is tcp
.
* @see TransportProtocol
* @return Returns a reference to this object so that method calls can be chained together.
* @see TransportProtocol
*/
Builder protocol(String protocol);
/**
*
* The protocol used for the port mapping. Valid values are tcp
and udp
. The default
* is tcp
.
*
*
* @param protocol
* The protocol used for the port mapping. Valid values are tcp
and udp
. The
* default is tcp
.
* @see TransportProtocol
* @return Returns a reference to this object so that method calls can be chained together.
* @see TransportProtocol
*/
Builder protocol(TransportProtocol protocol);
/**
*
* The name that's used for the port mapping. This parameter only applies to Service Connect. This parameter is
* the name that you use in the serviceConnectConfiguration
of a service. The name can include up
* to 64 characters. The characters can include lowercase letters, numbers, underscores (_), and hyphens (-).
* The name can't start with a hyphen.
*
*
* For more information, see Service Connect
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param name
* The name that's used for the port mapping. This parameter only applies to Service Connect. This
* parameter is the name that you use in the serviceConnectConfiguration
of a service. The
* name can include up to 64 characters. The characters can include lowercase letters, numbers,
* underscores (_), and hyphens (-). The name can't start with a hyphen.
*
* For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
*
* The application protocol that's used for the port mapping. This parameter only applies to Service Connect. We
* recommend that you set this parameter to be consistent with the protocol that your application uses. If you
* set this parameter, Amazon ECS adds protocol-specific connection handling to the Service Connect proxy. If
* you set this parameter, Amazon ECS adds protocol-specific telemetry in the Amazon ECS console and CloudWatch.
*
*
* If you don't set a value for this parameter, then TCP is used. However, Amazon ECS doesn't add
* protocol-specific telemetry for TCP.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect
* to services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are
* supported with Service Connect. For more information, see Service Connect
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param appProtocol
* The application protocol that's used for the port mapping. This parameter only applies to Service
* Connect. We recommend that you set this parameter to be consistent with the protocol that your
* application uses. If you set this parameter, Amazon ECS adds protocol-specific connection handling to
* the Service Connect proxy. If you set this parameter, Amazon ECS adds protocol-specific telemetry in
* the Amazon ECS console and CloudWatch.
*
* If you don't set a value for this parameter, then TCP is used. However, Amazon ECS doesn't add
* protocol-specific telemetry for TCP.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
* @see ApplicationProtocol
* @return Returns a reference to this object so that method calls can be chained together.
* @see ApplicationProtocol
*/
Builder appProtocol(String appProtocol);
/**
*
* The application protocol that's used for the port mapping. This parameter only applies to Service Connect. We
* recommend that you set this parameter to be consistent with the protocol that your application uses. If you
* set this parameter, Amazon ECS adds protocol-specific connection handling to the Service Connect proxy. If
* you set this parameter, Amazon ECS adds protocol-specific telemetry in the Amazon ECS console and CloudWatch.
*
*
* If you don't set a value for this parameter, then TCP is used. However, Amazon ECS doesn't add
* protocol-specific telemetry for TCP.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect
* to services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are
* supported with Service Connect. For more information, see Service Connect
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param appProtocol
* The application protocol that's used for the port mapping. This parameter only applies to Service
* Connect. We recommend that you set this parameter to be consistent with the protocol that your
* application uses. If you set this parameter, Amazon ECS adds protocol-specific connection handling to
* the Service Connect proxy. If you set this parameter, Amazon ECS adds protocol-specific telemetry in
* the Amazon ECS console and CloudWatch.
*
* If you don't set a value for this parameter, then TCP is used. However, Amazon ECS doesn't add
* protocol-specific telemetry for TCP.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
* @see ApplicationProtocol
* @return Returns a reference to this object so that method calls can be chained together.
* @see ApplicationProtocol
*/
Builder appProtocol(ApplicationProtocol appProtocol);
/**
*
* The port number range on the container that's bound to the dynamically mapped host port range.
*
*
* The following rules apply when you specify a containerPortRange
:
*
*
* -
*
* You must use either the bridge
network mode or the awsvpc
network mode.
*
*
* -
*
* This parameter is available for both the EC2 and Fargate launch types.
*
*
* -
*
* This parameter is available for both the Linux and Windows operating systems.
*
*
* -
*
* The container instance must have at least version 1.67.0 of the container agent and at least version 1.67.0-1
* of the ecs-init
package
*
*
* -
*
* You can specify a maximum of 100 port ranges per container.
*
*
* -
*
* You do not specify a hostPortRange
. The value of the hostPortRange
is set as
* follows:
*
*
* -
*
* For containers in a task with the awsvpc
network mode, the hostPort
is set to the
* same value as the containerPort
. This is a static mapping strategy.
*
*
* -
*
* For containers in a task with the bridge
network mode, the Amazon ECS agent finds open host
* ports from the default ephemeral range and passes it to docker to bind them to the container ports.
*
*
*
*
* -
*
* The containerPortRange
valid values are between 1 and 65535.
*
*
* -
*
* A port can only be included in one port mapping per container.
*
*
* -
*
* You cannot specify overlapping port ranges.
*
*
* -
*
* The first port in the range must be less than last port in the range.
*
*
* -
*
* Docker recommends that you turn off the docker-proxy in the Docker daemon config file when you have a large
* number of ports.
*
*
* For more information, see Issue #11185 on the Github
* website.
*
*
* For information about how to turn off the docker-proxy in the Docker daemon config file, see Docker daemon in the Amazon ECS Developer Guide.
*
*
*
*
* You can call
* DescribeTasks
to view the hostPortRange
which are the host ports that are
* bound to the container ports.
*
*
* @param containerPortRange
* The port number range on the container that's bound to the dynamically mapped host port range.
*
* The following rules apply when you specify a containerPortRange
:
*
*
* -
*
* You must use either the bridge
network mode or the awsvpc
network mode.
*
*
* -
*
* This parameter is available for both the EC2 and Fargate launch types.
*
*
* -
*
* This parameter is available for both the Linux and Windows operating systems.
*
*
* -
*
* The container instance must have at least version 1.67.0 of the container agent and at least version
* 1.67.0-1 of the ecs-init
package
*
*
* -
*
* You can specify a maximum of 100 port ranges per container.
*
*
* -
*
* You do not specify a hostPortRange
. The value of the hostPortRange
is set as
* follows:
*
*
* -
*
* For containers in a task with the awsvpc
network mode, the hostPort
is set
* to the same value as the containerPort
. This is a static mapping strategy.
*
*
* -
*
* For containers in a task with the bridge
network mode, the Amazon ECS agent finds open
* host ports from the default ephemeral range and passes it to docker to bind them to the container
* ports.
*
*
*
*
* -
*
* The containerPortRange
valid values are between 1 and 65535.
*
*
* -
*
* A port can only be included in one port mapping per container.
*
*
* -
*
* You cannot specify overlapping port ranges.
*
*
* -
*
* The first port in the range must be less than last port in the range.
*
*
* -
*
* Docker recommends that you turn off the docker-proxy in the Docker daemon config file when you have a
* large number of ports.
*
*
* For more information, see Issue #11185 on the
* Github website.
*
*
* For information about how to turn off the docker-proxy in the Docker daemon config file, see Docker daemon in the Amazon ECS Developer Guide.
*
*
*
*
* You can call
* DescribeTasks
to view the hostPortRange
which are the host ports that
* are bound to the container ports.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder containerPortRange(String containerPortRange);
}
static final class BuilderImpl implements Builder {
private Integer containerPort;
private Integer hostPort;
private String protocol;
private String name;
private String appProtocol;
private String containerPortRange;
private BuilderImpl() {
}
private BuilderImpl(PortMapping model) {
containerPort(model.containerPort);
hostPort(model.hostPort);
protocol(model.protocol);
name(model.name);
appProtocol(model.appProtocol);
containerPortRange(model.containerPortRange);
}
public final Integer getContainerPort() {
return containerPort;
}
public final void setContainerPort(Integer containerPort) {
this.containerPort = containerPort;
}
@Override
public final Builder containerPort(Integer containerPort) {
this.containerPort = containerPort;
return this;
}
public final Integer getHostPort() {
return hostPort;
}
public final void setHostPort(Integer hostPort) {
this.hostPort = hostPort;
}
@Override
public final Builder hostPort(Integer hostPort) {
this.hostPort = hostPort;
return this;
}
public final String getProtocol() {
return protocol;
}
public final void setProtocol(String protocol) {
this.protocol = protocol;
}
@Override
public final Builder protocol(String protocol) {
this.protocol = protocol;
return this;
}
@Override
public final Builder protocol(TransportProtocol protocol) {
this.protocol(protocol == null ? null : protocol.toString());
return this;
}
public final String getName() {
return name;
}
public final void setName(String name) {
this.name = name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final String getAppProtocol() {
return appProtocol;
}
public final void setAppProtocol(String appProtocol) {
this.appProtocol = appProtocol;
}
@Override
public final Builder appProtocol(String appProtocol) {
this.appProtocol = appProtocol;
return this;
}
@Override
public final Builder appProtocol(ApplicationProtocol appProtocol) {
this.appProtocol(appProtocol == null ? null : appProtocol.toString());
return this;
}
public final String getContainerPortRange() {
return containerPortRange;
}
public final void setContainerPortRange(String containerPortRange) {
this.containerPortRange = containerPortRange;
}
@Override
public final Builder containerPortRange(String containerPortRange) {
this.containerPortRange = containerPortRange;
return this;
}
@Override
public PortMapping build() {
return new PortMapping(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}