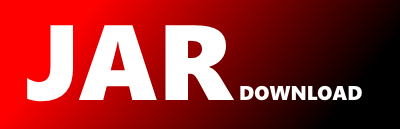
software.amazon.awssdk.services.ecs.model.TaskOverride Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The overrides that are associated with a task.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TaskOverride implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField> CONTAINER_OVERRIDES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("containerOverrides")
.getter(getter(TaskOverride::containerOverrides))
.setter(setter(Builder::containerOverrides))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerOverrides").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ContainerOverride::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CPU_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cpu")
.getter(getter(TaskOverride::cpu)).setter(setter(Builder::cpu))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cpu").build()).build();
private static final SdkField> INFERENCE_ACCELERATOR_OVERRIDES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("inferenceAcceleratorOverrides")
.getter(getter(TaskOverride::inferenceAcceleratorOverrides))
.setter(setter(Builder::inferenceAcceleratorOverrides))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inferenceAcceleratorOverrides")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InferenceAcceleratorOverride::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField EXECUTION_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("executionRoleArn").getter(getter(TaskOverride::executionRoleArn))
.setter(setter(Builder::executionRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionRoleArn").build()).build();
private static final SdkField MEMORY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("memory")
.getter(getter(TaskOverride::memory)).setter(setter(Builder::memory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memory").build()).build();
private static final SdkField TASK_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskRoleArn").getter(getter(TaskOverride::taskRoleArn)).setter(setter(Builder::taskRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskRoleArn").build()).build();
private static final SdkField EPHEMERAL_STORAGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ephemeralStorage")
.getter(getter(TaskOverride::ephemeralStorage)).setter(setter(Builder::ephemeralStorage))
.constructor(EphemeralStorage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ephemeralStorage").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTAINER_OVERRIDES_FIELD,
CPU_FIELD, INFERENCE_ACCELERATOR_OVERRIDES_FIELD, EXECUTION_ROLE_ARN_FIELD, MEMORY_FIELD, TASK_ROLE_ARN_FIELD,
EPHEMERAL_STORAGE_FIELD));
private static final long serialVersionUID = 1L;
private final List containerOverrides;
private final String cpu;
private final List inferenceAcceleratorOverrides;
private final String executionRoleArn;
private final String memory;
private final String taskRoleArn;
private final EphemeralStorage ephemeralStorage;
private TaskOverride(BuilderImpl builder) {
this.containerOverrides = builder.containerOverrides;
this.cpu = builder.cpu;
this.inferenceAcceleratorOverrides = builder.inferenceAcceleratorOverrides;
this.executionRoleArn = builder.executionRoleArn;
this.memory = builder.memory;
this.taskRoleArn = builder.taskRoleArn;
this.ephemeralStorage = builder.ephemeralStorage;
}
/**
* For responses, this returns true if the service returned a value for the ContainerOverrides property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasContainerOverrides() {
return containerOverrides != null && !(containerOverrides instanceof SdkAutoConstructList);
}
/**
*
* One or more container overrides that are sent to a task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasContainerOverrides} method.
*
*
* @return One or more container overrides that are sent to a task.
*/
public final List containerOverrides() {
return containerOverrides;
}
/**
*
* The CPU override for the task.
*
*
* @return The CPU override for the task.
*/
public final String cpu() {
return cpu;
}
/**
* For responses, this returns true if the service returned a value for the InferenceAcceleratorOverrides property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasInferenceAcceleratorOverrides() {
return inferenceAcceleratorOverrides != null && !(inferenceAcceleratorOverrides instanceof SdkAutoConstructList);
}
/**
*
* The Elastic Inference accelerator override for the task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInferenceAcceleratorOverrides} method.
*
*
* @return The Elastic Inference accelerator override for the task.
*/
public final List inferenceAcceleratorOverrides() {
return inferenceAcceleratorOverrides;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role override for the task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the task execution role override for the task. For more information,
* see Amazon
* ECS task execution IAM role in the Amazon Elastic Container Service Developer Guide.
*/
public final String executionRoleArn() {
return executionRoleArn;
}
/**
*
* The memory override for the task.
*
*
* @return The memory override for the task.
*/
public final String memory() {
return memory;
}
/**
*
* The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in this task
* are granted the permissions that are specified in this role. For more information, see IAM Role for Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the role that containers in this task can assume. All containers in
* this task are granted the permissions that are specified in this role. For more information, see IAM Role for
* Tasks in the Amazon Elastic Container Service Developer Guide.
*/
public final String taskRoleArn() {
return taskRoleArn;
}
/**
*
* The ephemeral storage setting override for the task.
*
*
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
*
* @return The ephemeral storage setting override for the task.
*
* This parameter is only supported for tasks hosted on Fargate that use the following platform versions:
*
*
* -
*
* Linux platform version 1.4.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*/
public final EphemeralStorage ephemeralStorage() {
return ephemeralStorage;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasContainerOverrides() ? containerOverrides() : null);
hashCode = 31 * hashCode + Objects.hashCode(cpu());
hashCode = 31 * hashCode + Objects.hashCode(hasInferenceAcceleratorOverrides() ? inferenceAcceleratorOverrides() : null);
hashCode = 31 * hashCode + Objects.hashCode(executionRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(memory());
hashCode = 31 * hashCode + Objects.hashCode(taskRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(ephemeralStorage());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TaskOverride)) {
return false;
}
TaskOverride other = (TaskOverride) obj;
return hasContainerOverrides() == other.hasContainerOverrides()
&& Objects.equals(containerOverrides(), other.containerOverrides()) && Objects.equals(cpu(), other.cpu())
&& hasInferenceAcceleratorOverrides() == other.hasInferenceAcceleratorOverrides()
&& Objects.equals(inferenceAcceleratorOverrides(), other.inferenceAcceleratorOverrides())
&& Objects.equals(executionRoleArn(), other.executionRoleArn()) && Objects.equals(memory(), other.memory())
&& Objects.equals(taskRoleArn(), other.taskRoleArn())
&& Objects.equals(ephemeralStorage(), other.ephemeralStorage());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("TaskOverride")
.add("ContainerOverrides", hasContainerOverrides() ? containerOverrides() : null)
.add("Cpu", cpu())
.add("InferenceAcceleratorOverrides", hasInferenceAcceleratorOverrides() ? inferenceAcceleratorOverrides() : null)
.add("ExecutionRoleArn", executionRoleArn()).add("Memory", memory()).add("TaskRoleArn", taskRoleArn())
.add("EphemeralStorage", ephemeralStorage()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "containerOverrides":
return Optional.ofNullable(clazz.cast(containerOverrides()));
case "cpu":
return Optional.ofNullable(clazz.cast(cpu()));
case "inferenceAcceleratorOverrides":
return Optional.ofNullable(clazz.cast(inferenceAcceleratorOverrides()));
case "executionRoleArn":
return Optional.ofNullable(clazz.cast(executionRoleArn()));
case "memory":
return Optional.ofNullable(clazz.cast(memory()));
case "taskRoleArn":
return Optional.ofNullable(clazz.cast(taskRoleArn()));
case "ephemeralStorage":
return Optional.ofNullable(clazz.cast(ephemeralStorage()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function