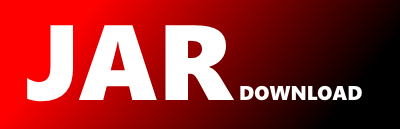
software.amazon.awssdk.services.ecs.DefaultEcsAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.ecs.internal.EcsServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.ecs.model.AccessDeniedException;
import software.amazon.awssdk.services.ecs.model.AttributeLimitExceededException;
import software.amazon.awssdk.services.ecs.model.BlockedException;
import software.amazon.awssdk.services.ecs.model.ClientException;
import software.amazon.awssdk.services.ecs.model.ClusterContainsContainerInstancesException;
import software.amazon.awssdk.services.ecs.model.ClusterContainsServicesException;
import software.amazon.awssdk.services.ecs.model.ClusterContainsTasksException;
import software.amazon.awssdk.services.ecs.model.ClusterNotFoundException;
import software.amazon.awssdk.services.ecs.model.ConflictException;
import software.amazon.awssdk.services.ecs.model.CreateCapacityProviderRequest;
import software.amazon.awssdk.services.ecs.model.CreateCapacityProviderResponse;
import software.amazon.awssdk.services.ecs.model.CreateClusterRequest;
import software.amazon.awssdk.services.ecs.model.CreateClusterResponse;
import software.amazon.awssdk.services.ecs.model.CreateServiceRequest;
import software.amazon.awssdk.services.ecs.model.CreateServiceResponse;
import software.amazon.awssdk.services.ecs.model.CreateTaskSetRequest;
import software.amazon.awssdk.services.ecs.model.CreateTaskSetResponse;
import software.amazon.awssdk.services.ecs.model.DeleteAccountSettingRequest;
import software.amazon.awssdk.services.ecs.model.DeleteAccountSettingResponse;
import software.amazon.awssdk.services.ecs.model.DeleteAttributesRequest;
import software.amazon.awssdk.services.ecs.model.DeleteAttributesResponse;
import software.amazon.awssdk.services.ecs.model.DeleteCapacityProviderRequest;
import software.amazon.awssdk.services.ecs.model.DeleteCapacityProviderResponse;
import software.amazon.awssdk.services.ecs.model.DeleteClusterRequest;
import software.amazon.awssdk.services.ecs.model.DeleteClusterResponse;
import software.amazon.awssdk.services.ecs.model.DeleteServiceRequest;
import software.amazon.awssdk.services.ecs.model.DeleteServiceResponse;
import software.amazon.awssdk.services.ecs.model.DeleteTaskDefinitionsRequest;
import software.amazon.awssdk.services.ecs.model.DeleteTaskDefinitionsResponse;
import software.amazon.awssdk.services.ecs.model.DeleteTaskSetRequest;
import software.amazon.awssdk.services.ecs.model.DeleteTaskSetResponse;
import software.amazon.awssdk.services.ecs.model.DeregisterContainerInstanceRequest;
import software.amazon.awssdk.services.ecs.model.DeregisterContainerInstanceResponse;
import software.amazon.awssdk.services.ecs.model.DeregisterTaskDefinitionRequest;
import software.amazon.awssdk.services.ecs.model.DeregisterTaskDefinitionResponse;
import software.amazon.awssdk.services.ecs.model.DescribeCapacityProvidersRequest;
import software.amazon.awssdk.services.ecs.model.DescribeCapacityProvidersResponse;
import software.amazon.awssdk.services.ecs.model.DescribeClustersRequest;
import software.amazon.awssdk.services.ecs.model.DescribeClustersResponse;
import software.amazon.awssdk.services.ecs.model.DescribeContainerInstancesRequest;
import software.amazon.awssdk.services.ecs.model.DescribeContainerInstancesResponse;
import software.amazon.awssdk.services.ecs.model.DescribeServicesRequest;
import software.amazon.awssdk.services.ecs.model.DescribeServicesResponse;
import software.amazon.awssdk.services.ecs.model.DescribeTaskDefinitionRequest;
import software.amazon.awssdk.services.ecs.model.DescribeTaskDefinitionResponse;
import software.amazon.awssdk.services.ecs.model.DescribeTaskSetsRequest;
import software.amazon.awssdk.services.ecs.model.DescribeTaskSetsResponse;
import software.amazon.awssdk.services.ecs.model.DescribeTasksRequest;
import software.amazon.awssdk.services.ecs.model.DescribeTasksResponse;
import software.amazon.awssdk.services.ecs.model.DiscoverPollEndpointRequest;
import software.amazon.awssdk.services.ecs.model.DiscoverPollEndpointResponse;
import software.amazon.awssdk.services.ecs.model.EcsException;
import software.amazon.awssdk.services.ecs.model.ExecuteCommandRequest;
import software.amazon.awssdk.services.ecs.model.ExecuteCommandResponse;
import software.amazon.awssdk.services.ecs.model.GetTaskProtectionRequest;
import software.amazon.awssdk.services.ecs.model.GetTaskProtectionResponse;
import software.amazon.awssdk.services.ecs.model.InvalidParameterException;
import software.amazon.awssdk.services.ecs.model.LimitExceededException;
import software.amazon.awssdk.services.ecs.model.ListAccountSettingsRequest;
import software.amazon.awssdk.services.ecs.model.ListAccountSettingsResponse;
import software.amazon.awssdk.services.ecs.model.ListAttributesRequest;
import software.amazon.awssdk.services.ecs.model.ListAttributesResponse;
import software.amazon.awssdk.services.ecs.model.ListClustersRequest;
import software.amazon.awssdk.services.ecs.model.ListClustersResponse;
import software.amazon.awssdk.services.ecs.model.ListContainerInstancesRequest;
import software.amazon.awssdk.services.ecs.model.ListContainerInstancesResponse;
import software.amazon.awssdk.services.ecs.model.ListServicesByNamespaceRequest;
import software.amazon.awssdk.services.ecs.model.ListServicesByNamespaceResponse;
import software.amazon.awssdk.services.ecs.model.ListServicesRequest;
import software.amazon.awssdk.services.ecs.model.ListServicesResponse;
import software.amazon.awssdk.services.ecs.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ecs.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesRequest;
import software.amazon.awssdk.services.ecs.model.ListTaskDefinitionFamiliesResponse;
import software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsRequest;
import software.amazon.awssdk.services.ecs.model.ListTaskDefinitionsResponse;
import software.amazon.awssdk.services.ecs.model.ListTasksRequest;
import software.amazon.awssdk.services.ecs.model.ListTasksResponse;
import software.amazon.awssdk.services.ecs.model.MissingVersionException;
import software.amazon.awssdk.services.ecs.model.NamespaceNotFoundException;
import software.amazon.awssdk.services.ecs.model.NoUpdateAvailableException;
import software.amazon.awssdk.services.ecs.model.PlatformTaskDefinitionIncompatibilityException;
import software.amazon.awssdk.services.ecs.model.PlatformUnknownException;
import software.amazon.awssdk.services.ecs.model.PutAccountSettingDefaultRequest;
import software.amazon.awssdk.services.ecs.model.PutAccountSettingDefaultResponse;
import software.amazon.awssdk.services.ecs.model.PutAccountSettingRequest;
import software.amazon.awssdk.services.ecs.model.PutAccountSettingResponse;
import software.amazon.awssdk.services.ecs.model.PutAttributesRequest;
import software.amazon.awssdk.services.ecs.model.PutAttributesResponse;
import software.amazon.awssdk.services.ecs.model.PutClusterCapacityProvidersRequest;
import software.amazon.awssdk.services.ecs.model.PutClusterCapacityProvidersResponse;
import software.amazon.awssdk.services.ecs.model.RegisterContainerInstanceRequest;
import software.amazon.awssdk.services.ecs.model.RegisterContainerInstanceResponse;
import software.amazon.awssdk.services.ecs.model.RegisterTaskDefinitionRequest;
import software.amazon.awssdk.services.ecs.model.RegisterTaskDefinitionResponse;
import software.amazon.awssdk.services.ecs.model.ResourceInUseException;
import software.amazon.awssdk.services.ecs.model.ResourceNotFoundException;
import software.amazon.awssdk.services.ecs.model.RunTaskRequest;
import software.amazon.awssdk.services.ecs.model.RunTaskResponse;
import software.amazon.awssdk.services.ecs.model.ServerException;
import software.amazon.awssdk.services.ecs.model.ServiceNotActiveException;
import software.amazon.awssdk.services.ecs.model.ServiceNotFoundException;
import software.amazon.awssdk.services.ecs.model.StartTaskRequest;
import software.amazon.awssdk.services.ecs.model.StartTaskResponse;
import software.amazon.awssdk.services.ecs.model.StopTaskRequest;
import software.amazon.awssdk.services.ecs.model.StopTaskResponse;
import software.amazon.awssdk.services.ecs.model.SubmitAttachmentStateChangesRequest;
import software.amazon.awssdk.services.ecs.model.SubmitAttachmentStateChangesResponse;
import software.amazon.awssdk.services.ecs.model.SubmitContainerStateChangeRequest;
import software.amazon.awssdk.services.ecs.model.SubmitContainerStateChangeResponse;
import software.amazon.awssdk.services.ecs.model.SubmitTaskStateChangeRequest;
import software.amazon.awssdk.services.ecs.model.SubmitTaskStateChangeResponse;
import software.amazon.awssdk.services.ecs.model.TagResourceRequest;
import software.amazon.awssdk.services.ecs.model.TagResourceResponse;
import software.amazon.awssdk.services.ecs.model.TargetNotConnectedException;
import software.amazon.awssdk.services.ecs.model.TargetNotFoundException;
import software.amazon.awssdk.services.ecs.model.TaskSetNotFoundException;
import software.amazon.awssdk.services.ecs.model.UnsupportedFeatureException;
import software.amazon.awssdk.services.ecs.model.UntagResourceRequest;
import software.amazon.awssdk.services.ecs.model.UntagResourceResponse;
import software.amazon.awssdk.services.ecs.model.UpdateCapacityProviderRequest;
import software.amazon.awssdk.services.ecs.model.UpdateCapacityProviderResponse;
import software.amazon.awssdk.services.ecs.model.UpdateClusterRequest;
import software.amazon.awssdk.services.ecs.model.UpdateClusterResponse;
import software.amazon.awssdk.services.ecs.model.UpdateClusterSettingsRequest;
import software.amazon.awssdk.services.ecs.model.UpdateClusterSettingsResponse;
import software.amazon.awssdk.services.ecs.model.UpdateContainerAgentRequest;
import software.amazon.awssdk.services.ecs.model.UpdateContainerAgentResponse;
import software.amazon.awssdk.services.ecs.model.UpdateContainerInstancesStateRequest;
import software.amazon.awssdk.services.ecs.model.UpdateContainerInstancesStateResponse;
import software.amazon.awssdk.services.ecs.model.UpdateInProgressException;
import software.amazon.awssdk.services.ecs.model.UpdateServicePrimaryTaskSetRequest;
import software.amazon.awssdk.services.ecs.model.UpdateServicePrimaryTaskSetResponse;
import software.amazon.awssdk.services.ecs.model.UpdateServiceRequest;
import software.amazon.awssdk.services.ecs.model.UpdateServiceResponse;
import software.amazon.awssdk.services.ecs.model.UpdateTaskProtectionRequest;
import software.amazon.awssdk.services.ecs.model.UpdateTaskProtectionResponse;
import software.amazon.awssdk.services.ecs.model.UpdateTaskSetRequest;
import software.amazon.awssdk.services.ecs.model.UpdateTaskSetResponse;
import software.amazon.awssdk.services.ecs.transform.CreateCapacityProviderRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.CreateClusterRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.CreateServiceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.CreateTaskSetRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeleteAccountSettingRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeleteAttributesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeleteCapacityProviderRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeleteClusterRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeleteServiceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeleteTaskDefinitionsRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeleteTaskSetRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeregisterContainerInstanceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DeregisterTaskDefinitionRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DescribeCapacityProvidersRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DescribeClustersRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DescribeContainerInstancesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DescribeServicesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DescribeTaskDefinitionRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DescribeTaskSetsRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DescribeTasksRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.DiscoverPollEndpointRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ExecuteCommandRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.GetTaskProtectionRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListAccountSettingsRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListAttributesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListClustersRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListContainerInstancesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListServicesByNamespaceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListServicesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListTaskDefinitionFamiliesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListTaskDefinitionsRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.ListTasksRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.PutAccountSettingDefaultRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.PutAccountSettingRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.PutAttributesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.PutClusterCapacityProvidersRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.RegisterContainerInstanceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.RegisterTaskDefinitionRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.RunTaskRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.StartTaskRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.StopTaskRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.SubmitAttachmentStateChangesRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.SubmitContainerStateChangeRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.SubmitTaskStateChangeRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateCapacityProviderRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateClusterRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateClusterSettingsRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateContainerAgentRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateContainerInstancesStateRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateServicePrimaryTaskSetRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateServiceRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateTaskProtectionRequestMarshaller;
import software.amazon.awssdk.services.ecs.transform.UpdateTaskSetRequestMarshaller;
import software.amazon.awssdk.services.ecs.waiters.EcsAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link EcsAsyncClient}.
*
* @see EcsAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultEcsAsyncClient implements EcsAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultEcsAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultEcsAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Creates a new capacity provider. Capacity providers are associated with an Amazon ECS cluster and are used in
* capacity provider strategies to facilitate cluster auto scaling.
*
*
* Only capacity providers that use an Auto Scaling group can be created. Amazon ECS tasks on Fargate use the
* FARGATE
and FARGATE_SPOT
capacity providers. These providers are available to all
* accounts in the Amazon Web Services Regions that Fargate supports.
*
*
* @param createCapacityProviderRequest
* @return A Java Future containing the result of the CreateCapacityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - LimitExceededException The limit for the resource was exceeded.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateCapacityProvider
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createCapacityProvider(
CreateCapacityProviderRequest createCapacityProviderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCapacityProviderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCapacityProviderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCapacityProvider");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateCapacityProviderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCapacityProvider").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateCapacityProviderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createCapacityProviderRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a default
cluster when you
* launch your first container instance. However, you can create your own cluster with a unique name with the
* CreateCluster
action.
*
*
*
* When you call the CreateCluster API operation, Amazon ECS attempts to create the Amazon ECS service-linked
* role for your account. This is so that it can manage required resources in other Amazon Web Services services on
* your behalf. However, if the user that makes the call doesn't have permissions to create the service-linked role,
* it isn't created. For more information, see Using
* service-linked roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @param createClusterRequest
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - NamespaceNotFoundException The specified namespace wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateCluster
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createCluster(CreateClusterRequest createClusterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createClusterRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCluster");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCluster").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateClusterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createClusterRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Runs and maintains your desired number of tasks from a specified task definition. If the number of tasks running
* in a service drops below the desiredCount
, Amazon ECS runs another copy of the task in the specified
* cluster. To update an existing service, see the UpdateService action.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
*
* In addition to maintaining the desired count of tasks in your service, you can optionally run your service behind
* one or more load balancers. The load balancers distribute traffic across the tasks that are associated with the
* service. For more information, see Service load
* balancing in the Amazon Elastic Container Service Developer Guide.
*
*
* Tasks for services that don't use a load balancer are considered healthy if they're in the RUNNING
* state. Tasks for services that use a load balancer are considered healthy if they're in the RUNNING
* state and are reported as healthy by the load balancer.
*
*
* There are two service scheduler strategies available:
*
*
* -
*
* REPLICA
- The replica scheduling strategy places and maintains your desired number of tasks across
* your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task
* placement strategies and constraints to customize task placement decisions. For more information, see Service scheduler
* concepts in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* DAEMON
- The daemon scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that you specify in your cluster. The service scheduler also
* evaluates the task placement constraints for running tasks. It also stops tasks that don't meet the placement
* constraints. When using this strategy, you don't need to specify a desired number of tasks, a task placement
* strategy, or use Service Auto Scaling policies. For more information, see Service scheduler
* concepts in the Amazon Elastic Container Service Developer Guide.
*
*
*
*
* You can optionally specify a deployment configuration for your service. The deployment is initiated by changing
* properties. For example, the deployment might be initiated by the task definition or by your desired count of a
* service. This is done with an UpdateService operation. The default value for a replica service for
* minimumHealthyPercent
is 100%. The default value for a daemon service for
* minimumHealthyPercent
is 0%.
*
*
* If a service uses the ECS
deployment controller, the minimum healthy percent represents a lower
* limit on the number of tasks in a service that must remain in the RUNNING
state during a deployment.
* Specifically, it represents it as a percentage of your desired number of tasks (rounded up to the nearest
* integer). This happens when any of your container instances are in the DRAINING
state if the service
* contains tasks using the EC2 launch type. Using this parameter, you can deploy without using additional cluster
* capacity. For example, if you set your service to have desired number of four tasks and a minimum healthy percent
* of 50%, the scheduler might stop two existing tasks to free up cluster capacity before starting two new tasks. If
* they're in the RUNNING
state, tasks for services that don't use a load balancer are considered
* healthy . If they're in the RUNNING
state and reported as healthy by the load balancer, tasks for
* services that do use a load balancer are considered healthy . The default value for minimum healthy
* percent is 100%.
*
*
* If a service uses the ECS
deployment controller, the maximum percent parameter represents an
* upper limit on the number of tasks in a service that are allowed in the RUNNING
or
* PENDING
state during a deployment. Specifically, it represents it as a percentage of the desired
* number of tasks (rounded down to the nearest integer). This happens when any of your container instances are in
* the DRAINING
state if the service contains tasks using the EC2 launch type. Using this parameter,
* you can define the deployment batch size. For example, if your service has a desired number of four tasks and a
* maximum percent value of 200%, the scheduler may start four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available). The default value for maximum percent is
* 200%.
*
*
* If a service uses either the CODE_DEPLOY
or EXTERNAL
deployment controller types and
* tasks that use the EC2 launch type, the minimum healthy percent and maximum percent values are used
* only to define the lower and upper limit on the number of the tasks in the service that remain in the
* RUNNING
state. This is while the container instances are in the DRAINING
state. If the
* tasks in the service use the Fargate launch type, the minimum healthy percent and maximum percent values aren't
* used. This is the case even if they're currently visible when describing your service.
*
*
* When creating a service that uses the EXTERNAL
deployment controller, you can specify only
* parameters that aren't controlled at the task set level. The only required parameter is the service name. You
* control your services using the CreateTaskSet operation. For more information, see Amazon ECS deployment
* types in the Amazon Elastic Container Service Developer Guide.
*
*
* When the service scheduler launches new tasks, it determines task placement. For information about task placement
* and task placement strategies, see Amazon ECS task
* placement in the Amazon Elastic Container Service Developer Guide.
*
*
* @param createServiceRequest
* @return A Java Future containing the result of the CreateService operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - NamespaceNotFoundException The specified namespace wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateService
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createService(CreateServiceRequest createServiceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createServiceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateService");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateService").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateServiceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createServiceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Create a task set in the specified cluster and service. This is used when a service uses the
* EXTERNAL
deployment controller type. For more information, see Amazon ECS deployment
* types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param createTaskSetRequest
* @return A Java Future containing the result of the CreateTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - PlatformUnknownException The specified platform version doesn't exist.
* - PlatformTaskDefinitionIncompatibilityException The specified platform version doesn't satisfy the
* required capabilities of the task definition.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - NamespaceNotFoundException The specified namespace wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.CreateTaskSet
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createTaskSet(CreateTaskSetRequest createTaskSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createTaskSetRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createTaskSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateTaskSet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateTaskSetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateTaskSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateTaskSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createTaskSetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables an account setting for a specified user, role, or the root user for an account.
*
*
* @param deleteAccountSettingRequest
* @return A Java Future containing the result of the DeleteAccountSetting operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteAccountSetting
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAccountSetting(
DeleteAccountSettingRequest deleteAccountSettingRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAccountSettingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAccountSettingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAccountSetting");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAccountSettingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAccountSetting").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAccountSettingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAccountSettingRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes one or more custom attributes from an Amazon ECS resource.
*
*
* @param deleteAttributesRequest
* @return A Java Future containing the result of the DeleteAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotFoundException The specified target wasn't found. You can view your available container
* instances with ListContainerInstances. Amazon ECS container instances are cluster-specific and
* Region-specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteAttributes
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteAttributes(DeleteAttributesRequest deleteAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteAttributesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified capacity provider.
*
*
*
* The FARGATE
and FARGATE_SPOT
capacity providers are reserved and can't be deleted. You
* can disassociate them from a cluster using either the PutClusterCapacityProviders API or by deleting the
* cluster.
*
*
*
* Prior to a capacity provider being deleted, the capacity provider must be removed from the capacity provider
* strategy from all services. The UpdateService API can be used to remove a capacity provider from a
* service's capacity provider strategy. When updating a service, the forceNewDeployment
option can be
* used to ensure that any tasks using the Amazon EC2 instance capacity provided by the capacity provider are
* transitioned to use the capacity from the remaining capacity providers. Only capacity providers that aren't
* associated with a cluster can be deleted. To remove a capacity provider from a cluster, you can either use
* PutClusterCapacityProviders or delete the cluster.
*
*
* @param deleteCapacityProviderRequest
* @return A Java Future containing the result of the DeleteCapacityProvider operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteCapacityProvider
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteCapacityProvider(
DeleteCapacityProviderRequest deleteCapacityProviderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCapacityProviderRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCapacityProviderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCapacityProvider");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteCapacityProviderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCapacityProvider").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteCapacityProviderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteCapacityProviderRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified cluster. The cluster transitions to the INACTIVE
state. Clusters with an
* INACTIVE
status might remain discoverable in your account for a period of time. However, this
* behavior is subject to change in the future. We don't recommend that you rely on INACTIVE
clusters
* persisting.
*
*
* You must deregister all container instances from this cluster before you may delete it. You can list the
* container instances in a cluster with ListContainerInstances and deregister them with
* DeregisterContainerInstance.
*
*
* @param deleteClusterRequest
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ClusterContainsContainerInstancesException You can't delete a cluster that has registered container
* instances. First, deregister the container instances before you can delete the cluster. For more
* information, see DeregisterContainerInstance.
* - ClusterContainsServicesException You can't delete a cluster that contains services. First, update the
* service to reduce its desired task count to 0, and then delete the service. For more information, see
* UpdateService and DeleteService.
* - ClusterContainsTasksException You can't delete a cluster that has active tasks.
* - UpdateInProgressException There's already a current Amazon ECS container agent update in progress on
* the container instance that's specified. If the container agent becomes disconnected while it's in a
* transitional stage, such as
PENDING
or STAGING
, the update process can get
* stuck in that state. However, when the agent reconnects, it resumes where it stopped previously.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteCluster
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteCluster(DeleteClusterRequest deleteClusterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteClusterRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCluster");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCluster").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteClusterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteClusterRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified service within a cluster. You can delete a service if you have no running tasks in it and the
* desired task count is zero. If the service is actively maintaining tasks, you can't delete it, and you must
* update the service to a desired task count of zero. For more information, see UpdateService.
*
*
*
* When you delete a service, if there are still running tasks that require cleanup, the service status moves from
* ACTIVE
to DRAINING
, and the service is no longer visible in the console or in the
* ListServices API operation. After all tasks have transitioned to either STOPPING
or
* STOPPED
status, the service status moves from DRAINING
to INACTIVE
.
* Services in the DRAINING
or INACTIVE
status can still be viewed with the
* DescribeServices API operation. However, in the future, INACTIVE
services may be cleaned up
* and purged from Amazon ECS record keeping, and DescribeServices calls on those services return a
* ServiceNotFoundException
error.
*
*
*
* If you attempt to create a new service with the same name as an existing service in either ACTIVE
or
* DRAINING
status, you receive an error.
*
*
*
* @param deleteServiceRequest
* @return A Java Future containing the result of the DeleteService operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteService
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteService(DeleteServiceRequest deleteServiceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteServiceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteServiceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteService");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteServiceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteService").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteServiceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteServiceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes one or more task definitions.
*
*
* You must deregister a task definition revision before you delete it. For more information, see DeregisterTaskDefinition.
*
*
* When you delete a task definition revision, it is immediately transitions from the INACTIVE
to
* DELETE_IN_PROGRESS
. Existing tasks and services that reference a DELETE_IN_PROGRESS
* task definition revision continue to run without disruption. Existing services that reference a
* DELETE_IN_PROGRESS
task definition revision can still scale up or down by modifying the service's
* desired count.
*
*
* You can't use a DELETE_IN_PROGRESS
task definition revision to run new tasks or create new services.
* You also can't update an existing service to reference a DELETE_IN_PROGRESS
task definition
* revision.
*
*
* A task definition revision will stay in DELETE_IN_PROGRESS
status until all the associated tasks and
* services have been terminated.
*
*
* When you delete all INACTIVE
task definition revisions, the task definition name is not displayed in
* the console and not returned in the API. If a task definition revisions are in the
* DELETE_IN_PROGRESS
state, the task definition name is displayed in the console and returned in the
* API. The task definition name is retained by Amazon ECS and the revision is incremented the next time you create
* a task definition with that name.
*
*
* @param deleteTaskDefinitionsRequest
* @return A Java Future containing the result of the DeleteTaskDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ServerException These errors are usually caused by a server issue.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteTaskDefinitions
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteTaskDefinitions(
DeleteTaskDefinitionsRequest deleteTaskDefinitionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteTaskDefinitionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteTaskDefinitionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteTaskDefinitions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteTaskDefinitionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteTaskDefinitions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteTaskDefinitionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteTaskDefinitionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified task set within a service. This is used when a service uses the EXTERNAL
* deployment controller type. For more information, see Amazon ECS deployment
* types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param deleteTaskSetRequest
* @return A Java Future containing the result of the DeleteTaskSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - TaskSetNotFoundException The specified task set wasn't found. You can view your available task sets
* with DescribeTaskSets. Task sets are specific to each cluster, service and Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeleteTaskSet
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteTaskSet(DeleteTaskSetRequest deleteTaskSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteTaskSetRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteTaskSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteTaskSet");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteTaskSetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteTaskSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteTaskSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteTaskSetRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deregisters an Amazon ECS container instance from the specified cluster. This instance is no longer available to
* run tasks.
*
*
* If you intend to use the container instance for some other purpose after deregistration, we recommend that you
* stop all of the tasks running on the container instance before deregistration. That prevents any orphaned tasks
* from consuming resources.
*
*
* Deregistering a container instance removes the instance from a cluster, but it doesn't terminate the EC2
* instance. If you are finished using the instance, be sure to terminate it in the Amazon EC2 console to stop
* billing.
*
*
*
* If you terminate a running container instance, Amazon ECS automatically deregisters the instance from your
* cluster (stopped container instances or instances with disconnected agents aren't automatically deregistered when
* terminated).
*
*
*
* @param deregisterContainerInstanceRequest
* @return A Java Future containing the result of the DeregisterContainerInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeregisterContainerInstance
* @see AWS API Documentation
*/
@Override
public CompletableFuture deregisterContainerInstance(
DeregisterContainerInstanceRequest deregisterContainerInstanceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterContainerInstanceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterContainerInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterContainerInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterContainerInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterContainerInstance").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeregisterContainerInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deregisterContainerInstanceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deregisters the specified task definition by family and revision. Upon deregistration, the task definition is
* marked as INACTIVE
. Existing tasks and services that reference an INACTIVE
task
* definition continue to run without disruption. Existing services that reference an INACTIVE
task
* definition can still scale up or down by modifying the service's desired count. If you want to delete a task
* definition revision, you must first deregister the task definition revision.
*
*
* You can't use an INACTIVE
task definition to run new tasks or create new services, and you can't
* update an existing service to reference an INACTIVE
task definition. However, there may be up to a
* 10-minute window following deregistration where these restrictions have not yet taken effect.
*
*
*
* At this time, INACTIVE
task definitions remain discoverable in your account indefinitely. However,
* this behavior is subject to change in the future. We don't recommend that you rely on INACTIVE
task
* definitions persisting beyond the lifecycle of any associated tasks and services.
*
*
*
* You must deregister a task definition revision before you delete it. For more information, see DeleteTaskDefinitions.
*
*
* @param deregisterTaskDefinitionRequest
* @return A Java Future containing the result of the DeregisterTaskDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DeregisterTaskDefinition
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deregisterTaskDefinition(
DeregisterTaskDefinitionRequest deregisterTaskDefinitionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterTaskDefinitionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterTaskDefinitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterTaskDefinition");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterTaskDefinitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterTaskDefinition").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeregisterTaskDefinitionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deregisterTaskDefinitionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes one or more of your capacity providers.
*
*
* @param describeCapacityProvidersRequest
* @return A Java Future containing the result of the DescribeCapacityProviders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeCapacityProviders
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeCapacityProviders(
DescribeCapacityProvidersRequest describeCapacityProvidersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCapacityProvidersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCapacityProvidersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCapacityProviders");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeCapacityProvidersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCapacityProviders").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeCapacityProvidersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeCapacityProvidersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes one or more of your clusters.
*
*
* @param describeClustersRequest
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeClusters
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeClusters(DescribeClustersRequest describeClustersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeClustersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeClustersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeClusters");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeClustersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeClusters").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeClustersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeClustersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes one or more container instances. Returns metadata about each container instance requested.
*
*
* @param describeContainerInstancesRequest
* @return A Java Future containing the result of the DescribeContainerInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeContainerInstances
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeContainerInstances(
DescribeContainerInstancesRequest describeContainerInstancesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeContainerInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeContainerInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeContainerInstances");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeContainerInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeContainerInstances").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeContainerInstancesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeContainerInstancesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the specified services running in your cluster.
*
*
* @param describeServicesRequest
* @return A Java Future containing the result of the DescribeServices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeServices
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeServices(DescribeServicesRequest describeServicesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeServicesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeServicesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeServices");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeServicesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeServices").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeServicesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeServicesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a task definition. You can specify a family
and revision
to find information
* about a specific task definition, or you can simply specify the family to find the latest ACTIVE
* revision in that family.
*
*
*
* You can only describe INACTIVE
task definitions while an active task or service references them.
*
*
*
* @param describeTaskDefinitionRequest
* @return A Java Future containing the result of the DescribeTaskDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTaskDefinition
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeTaskDefinition(
DescribeTaskDefinitionRequest describeTaskDefinitionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeTaskDefinitionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTaskDefinitionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTaskDefinition");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeTaskDefinitionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTaskDefinition").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeTaskDefinitionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeTaskDefinitionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the task sets in the specified cluster and service. This is used when a service uses the
* EXTERNAL
deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param describeTaskSetsRequest
* @return A Java Future containing the result of the DescribeTaskSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - ServiceNotActiveException The specified service isn't active. You can't update a service that's
* inactive. If you have previously deleted a service, you can re-create it with CreateService.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTaskSets
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeTaskSets(DescribeTaskSetsRequest describeTaskSetsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeTaskSetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTaskSetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTaskSets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeTaskSetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTaskSets").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeTaskSetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeTaskSetsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a specified task or tasks.
*
*
* Currently, stopped tasks appear in the returned results for at least one hour.
*
*
* If you have tasks with tags, and then delete the cluster, the tagged tasks are returned in the response. If you
* create a new cluster with the same name as the deleted cluster, the tagged tasks are not included in the
* response.
*
*
* @param describeTasksRequest
* @return A Java Future containing the result of the DescribeTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DescribeTasks
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeTasks(DescribeTasksRequest describeTasksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeTasksRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTasksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTasks");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeTasksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTasks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeTasksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeTasksRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Returns an endpoint for the Amazon ECS agent to poll for updates.
*
*
* @param discoverPollEndpointRequest
* @return A Java Future containing the result of the DiscoverPollEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.DiscoverPollEndpoint
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture discoverPollEndpoint(
DiscoverPollEndpointRequest discoverPollEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(discoverPollEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, discoverPollEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DiscoverPollEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DiscoverPollEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DiscoverPollEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DiscoverPollEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(discoverPollEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Runs a command remotely on a container within a task.
*
*
* If you use a condition key in your IAM policy to refine the conditions for the policy statement, for example
* limit the actions to a specific cluster, you receive an AccessDeniedException
when there is a
* mismatch between the condition key value and the corresponding parameter value.
*
*
* For information about required permissions and considerations, see Using Amazon ECS Exec for
* debugging in the Amazon ECS Developer Guide.
*
*
* @param executeCommandRequest
* @return A Java Future containing the result of the ExecuteCommand operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotConnectedException The execute command cannot run. This error can be caused by any of the
* following configuration issues:
*
* -
*
* Incorrect IAM permissions
*
*
* -
*
* The SSM agent is not installed or is not running
*
*
* -
*
* There is an interface Amazon VPC endpoint for Amazon ECS, but there is not one for Systems Manager
* Session Manager
*
*
*
*
* For information about how to troubleshoot the issues, see Troubleshooting issues
* with ECS Exec in the Amazon Elastic Container Service Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ExecuteCommand
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture executeCommand(ExecuteCommandRequest executeCommandRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(executeCommandRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, executeCommandRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ExecuteCommand");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ExecuteCommandResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ExecuteCommand").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ExecuteCommandRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(executeCommandRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the protection status of tasks in an Amazon ECS service.
*
*
* @param getTaskProtectionRequest
* @return A Java Future containing the result of the GetTaskProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have authorization to perform the requested action.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ResourceNotFoundException The specified resource wasn't found.
* - ServerException These errors are usually caused by a server issue.
* - UnsupportedFeatureException The specified task isn't supported in this Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.GetTaskProtection
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getTaskProtection(GetTaskProtectionRequest getTaskProtectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getTaskProtectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getTaskProtectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetTaskProtection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetTaskProtectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetTaskProtection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetTaskProtectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getTaskProtectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the account settings for a specified principal.
*
*
* @param listAccountSettingsRequest
* @return A Java Future containing the result of the ListAccountSettings operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAccountSettings
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAccountSettings(
ListAccountSettingsRequest listAccountSettingsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAccountSettingsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAccountSettingsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAccountSettings");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAccountSettingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAccountSettings").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAccountSettingsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAccountSettingsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the attributes for Amazon ECS resources within a specified target type and cluster. When you specify a
* target type and cluster, ListAttributes
returns a list of attribute objects, one for each attribute
* on each resource. You can filter the list of results to a single attribute name to only return results that have
* that name. You can also filter the results by attribute name and value. You can do this, for example, to see
* which container instances in a cluster are running a Linux AMI (ecs.os-type=linux
).
*
*
* @param listAttributesRequest
* @return A Java Future containing the result of the ListAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListAttributes
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAttributes(ListAttributesRequest listAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAttributesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAttributesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of existing clusters.
*
*
* @param listClustersRequest
* @return A Java Future containing the result of the ListClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListClusters
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listClusters(ListClustersRequest listClustersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listClustersRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listClustersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListClusters");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListClustersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListClusters").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListClustersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listClustersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of container instances in a specified cluster. You can filter the results of a
* ListContainerInstances
operation with cluster query language statements inside the
* filter
parameter. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
* @param listContainerInstancesRequest
* @return A Java Future containing the result of the ListContainerInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListContainerInstances
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listContainerInstances(
ListContainerInstancesRequest listContainerInstancesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listContainerInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listContainerInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListContainerInstances");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListContainerInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListContainerInstances").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListContainerInstancesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listContainerInstancesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of services. You can filter the results by cluster, launch type, and scheduling strategy.
*
*
* @param listServicesRequest
* @return A Java Future containing the result of the ListServices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListServices
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listServices(ListServicesRequest listServicesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listServicesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listServicesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListServices");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListServicesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListServices").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListServicesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listServicesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This operation lists all of the services that are associated with a Cloud Map namespace. This list might include
* services in different clusters. In contrast, ListServices
can only list services in one cluster at a
* time. If you need to filter the list of services in a single cluster by various parameters, use
* ListServices
. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param listServicesByNamespaceRequest
* @return A Java Future containing the result of the ListServicesByNamespace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - NamespaceNotFoundException The specified namespace wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListServicesByNamespace
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listServicesByNamespace(
ListServicesByNamespaceRequest listServicesByNamespaceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listServicesByNamespaceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listServicesByNamespaceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListServicesByNamespace");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListServicesByNamespaceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListServicesByNamespace").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListServicesByNamespaceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listServicesByNamespaceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List the tags for an Amazon ECS resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of task definition families that are registered to your account. This list includes task
* definition families that no longer have any ACTIVE
task definition revisions.
*
*
* You can filter out task definition families that don't contain any ACTIVE
task definition revisions
* by setting the status
parameter to ACTIVE
. You can also filter the results with the
* familyPrefix
parameter.
*
*
* @param listTaskDefinitionFamiliesRequest
* @return A Java Future containing the result of the ListTaskDefinitionFamilies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitionFamilies
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTaskDefinitionFamilies(
ListTaskDefinitionFamiliesRequest listTaskDefinitionFamiliesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTaskDefinitionFamiliesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTaskDefinitionFamiliesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTaskDefinitionFamilies");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTaskDefinitionFamiliesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTaskDefinitionFamilies").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTaskDefinitionFamiliesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTaskDefinitionFamiliesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of task definitions that are registered to your account. You can filter the results by family name
* with the familyPrefix
parameter or by status with the status
parameter.
*
*
* @param listTaskDefinitionsRequest
* @return A Java Future containing the result of the ListTaskDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTaskDefinitions
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listTaskDefinitions(
ListTaskDefinitionsRequest listTaskDefinitionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTaskDefinitionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTaskDefinitionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTaskDefinitions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTaskDefinitionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTaskDefinitions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTaskDefinitionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTaskDefinitionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of tasks. You can filter the results by cluster, task definition family, container instance,
* launch type, what IAM principal started the task, or by the desired status of the task.
*
*
* Recently stopped tasks might appear in the returned results.
*
*
* @param listTasksRequest
* @return A Java Future containing the result of the ListTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - ServiceNotFoundException The specified service wasn't found. You can view your available services
* with ListServices. Amazon ECS services are cluster specific and Region specific.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.ListTasks
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listTasks(ListTasksRequest listTasksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTasksRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTasksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTasks");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListTasksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListTasks")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTasksRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(listTasksRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Modifies an account setting. Account settings are set on a per-Region basis.
*
*
* If you change the root user account setting, the default settings are reset for users and roles that do not have
* specified individual account settings. For more information, see Account Settings
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When you specify serviceLongArnFormat
, taskLongArnFormat
, or
* containerInstanceLongArnFormat
, the Amazon Resource Name (ARN) and resource ID format of the
* resource type for a specified user, role, or the root user for an account is affected. The opt-in and opt-out
* account setting must be set for each Amazon ECS resource separately. The ARN and resource ID format of a resource
* is defined by the opt-in status of the user or role that created the resource. You must turn on this setting to
* use Amazon ECS features such as resource tagging.
*
*
* When you specify awsvpcTrunking
, the elastic network interface (ENI) limit for any new container
* instances that support the feature is changed. If awsvpcTrunking
is turned on, any new container
* instances that support the feature are launched have the increased ENI limits available to them. For more
* information, see Elastic Network
* Interface Trunking in the Amazon Elastic Container Service Developer Guide.
*
*
* When you specify containerInsights
, the default setting indicating whether Amazon Web Services
* CloudWatch Container Insights is turned on for your clusters is changed. If containerInsights
is
* turned on, any new clusters that are created will have Container Insights turned on unless you disable it during
* cluster creation. For more information, see CloudWatch
* Container Insights in the Amazon Elastic Container Service Developer Guide.
*
*
* Amazon ECS is introducing tagging authorization for resource creation. Users must have permissions for actions
* that create the resource, such as ecsCreateCluster
. If tags are specified when you create a
* resource, Amazon Web Services performs additional authorization to verify if users or roles have permissions to
* create tags. Therefore, you must grant explicit permissions to use the ecs:TagResource
action. For
* more information, see Grant
* permission to tag resources on creation in the Amazon ECS Developer Guide.
*
*
* When Amazon Web Services determines that a security or infrastructure update is needed for an Amazon ECS task
* hosted on Fargate, the tasks need to be stopped and new tasks launched to replace them. Use
* fargateTaskRetirementWaitPeriod
to configure the wait time to retire a Fargate task. For information
* about the Fargate tasks maintenance, see Amazon Web Services
* Fargate task maintenance in the Amazon ECS Developer Guide.
*
*
* The guardDutyActivate
parameter is read-only in Amazon ECS and indicates whether Amazon ECS Runtime
* Monitoring is enabled or disabled by your security administrator in your Amazon ECS account. Amazon GuardDuty
* controls this account setting on your behalf. For more information, see Protecting
* Amazon ECS workloads with Amazon ECS Runtime Monitoring.
*
*
* @param putAccountSettingRequest
* @return A Java Future containing the result of the PutAccountSetting operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAccountSetting
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putAccountSetting(PutAccountSettingRequest putAccountSettingRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAccountSettingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAccountSettingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAccountSetting");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAccountSettingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAccountSetting").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutAccountSettingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putAccountSettingRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Modifies an account setting for all users on an account for whom no individual account setting has been
* specified. Account settings are set on a per-Region basis.
*
*
* @param putAccountSettingDefaultRequest
* @return A Java Future containing the result of the PutAccountSettingDefault operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServerException These errors are usually caused by a server issue.
* - ClientException These errors are usually caused by a client action. This client action might be using
* an action or resource on behalf of a user that doesn't have permissions to use the action or resource.
* Or, it might be specifying an identifier that isn't valid.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAccountSettingDefault
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture putAccountSettingDefault(
PutAccountSettingDefaultRequest putAccountSettingDefaultRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAccountSettingDefaultRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAccountSettingDefaultRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAccountSettingDefault");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAccountSettingDefaultResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAccountSettingDefault").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutAccountSettingDefaultRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putAccountSettingDefaultRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Create or update an attribute on an Amazon ECS resource. If the attribute doesn't exist, it's created. If the
* attribute exists, its value is replaced with the specified value. To delete an attribute, use
* DeleteAttributes. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
* @param putAttributesRequest
* @return A Java Future containing the result of the PutAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ClusterNotFoundException The specified cluster wasn't found. You can view your available clusters
* with ListClusters. Amazon ECS clusters are Region specific.
* - TargetNotFoundException The specified target wasn't found. You can view your available container
* instances with ListContainerInstances. Amazon ECS container instances are cluster-specific and
* Region-specific.
* - AttributeLimitExceededException You can apply up to 10 custom attributes for each resource. You can
* view the attributes of a resource with ListAttributes. You can remove existing attributes on a
* resource with DeleteAttributes.
* - InvalidParameterException The specified parameter isn't valid. Review the available parameters for
* the API request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - EcsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample EcsAsyncClient.PutAttributes
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putAttributes(PutAttributesRequest putAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putAttributesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ECS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams