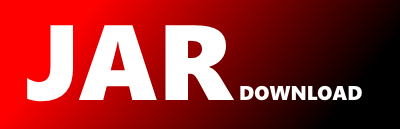
software.amazon.awssdk.services.ecs.endpoints.internal.EcsResolveEndpointInterceptor Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.endpoints.internal;
import java.time.Duration;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletionException;
import java.util.function.Supplier;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.auth.signer.Aws4Signer;
import software.amazon.awssdk.auth.signer.SignerLoader;
import software.amazon.awssdk.awscore.AwsExecutionAttribute;
import software.amazon.awssdk.awscore.endpoints.AwsEndpointAttribute;
import software.amazon.awssdk.awscore.endpoints.authscheme.EndpointAuthScheme;
import software.amazon.awssdk.awscore.endpoints.authscheme.SigV4AuthScheme;
import software.amazon.awssdk.awscore.endpoints.authscheme.SigV4aAuthScheme;
import software.amazon.awssdk.awscore.util.SignerOverrideUtils;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.SelectedAuthScheme;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.interceptor.Context;
import software.amazon.awssdk.core.interceptor.ExecutionAttributes;
import software.amazon.awssdk.core.interceptor.ExecutionInterceptor;
import software.amazon.awssdk.core.interceptor.SdkExecutionAttribute;
import software.amazon.awssdk.core.interceptor.SdkInternalExecutionAttribute;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.signer.Signer;
import software.amazon.awssdk.endpoints.Endpoint;
import software.amazon.awssdk.http.SdkHttpRequest;
import software.amazon.awssdk.http.auth.aws.signer.AwsV4HttpSigner;
import software.amazon.awssdk.http.auth.aws.signer.AwsV4aHttpSigner;
import software.amazon.awssdk.http.auth.aws.signer.RegionSet;
import software.amazon.awssdk.http.auth.spi.scheme.AuthSchemeOption;
import software.amazon.awssdk.identity.spi.Identity;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.services.ecs.endpoints.EcsEndpointParams;
import software.amazon.awssdk.services.ecs.endpoints.EcsEndpointProvider;
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
public final class EcsResolveEndpointInterceptor implements ExecutionInterceptor {
private final EndpointAuthSchemeStrategy endpointAuthSchemeStrategy;
public EcsResolveEndpointInterceptor() {
EndpointAuthSchemeStrategyFactory endpointAuthSchemeStrategyFactory = new DefaultEndpointAuthSchemeStrategyFactory();
this.endpointAuthSchemeStrategy = endpointAuthSchemeStrategyFactory.endpointAuthSchemeStrategy();
}
@Override
public SdkRequest modifyRequest(Context.ModifyRequest context, ExecutionAttributes executionAttributes) {
SdkRequest result = context.request();
if (AwsEndpointProviderUtils.endpointIsDiscovered(executionAttributes)) {
return result;
}
EcsEndpointProvider provider = (EcsEndpointProvider) executionAttributes
.getAttribute(SdkInternalExecutionAttribute.ENDPOINT_PROVIDER);
try {
long resolveEndpointStart = System.nanoTime();
Endpoint endpoint = provider.resolveEndpoint(ruleParams(result, executionAttributes)).join();
Duration resolveEndpointDuration = Duration.ofNanos(System.nanoTime() - resolveEndpointStart);
Optional metricCollector = executionAttributes
.getOptionalAttribute(SdkExecutionAttribute.API_CALL_METRIC_COLLECTOR);
metricCollector.ifPresent(mc -> mc.reportMetric(CoreMetric.ENDPOINT_RESOLVE_DURATION, resolveEndpointDuration));
if (!AwsEndpointProviderUtils.disableHostPrefixInjection(executionAttributes)) {
Optional hostPrefix = hostPrefix(executionAttributes.getAttribute(SdkExecutionAttribute.OPERATION_NAME),
result);
if (hostPrefix.isPresent()) {
endpoint = AwsEndpointProviderUtils.addHostPrefix(endpoint, hostPrefix.get());
}
}
List endpointAuthSchemes = endpoint.attribute(AwsEndpointAttribute.AUTH_SCHEMES);
SelectedAuthScheme> selectedAuthScheme = executionAttributes
.getAttribute(SdkInternalExecutionAttribute.SELECTED_AUTH_SCHEME);
if (endpointAuthSchemes != null && selectedAuthScheme != null) {
selectedAuthScheme = authSchemeWithEndpointSignerProperties(endpointAuthSchemes, selectedAuthScheme);
executionAttributes.putAttribute(SdkInternalExecutionAttribute.SELECTED_AUTH_SCHEME, selectedAuthScheme);
}
if (endpointAuthSchemes != null) {
EndpointAuthScheme chosenAuthScheme = endpointAuthSchemeStrategy.chooseAuthScheme(endpointAuthSchemes);
Supplier signerProvider = signerProvider(chosenAuthScheme);
result = SignerOverrideUtils.overrideSignerIfNotOverridden(result, executionAttributes, signerProvider);
}
executionAttributes.putAttribute(SdkInternalExecutionAttribute.RESOLVED_ENDPOINT, endpoint);
return result;
} catch (CompletionException e) {
Throwable cause = e.getCause();
if (cause instanceof SdkClientException) {
throw (SdkClientException) cause;
} else {
throw SdkClientException.create("Endpoint resolution failed", cause);
}
}
}
@Override
public SdkHttpRequest modifyHttpRequest(Context.ModifyHttpRequest context, ExecutionAttributes executionAttributes) {
Endpoint resolvedEndpoint = executionAttributes.getAttribute(SdkInternalExecutionAttribute.RESOLVED_ENDPOINT);
if (resolvedEndpoint.headers().isEmpty()) {
return context.httpRequest();
}
SdkHttpRequest.Builder httpRequestBuilder = context.httpRequest().toBuilder();
resolvedEndpoint.headers().forEach((name, values) -> {
values.forEach(v -> httpRequestBuilder.appendHeader(name, v));
});
return httpRequestBuilder.build();
}
public static EcsEndpointParams ruleParams(SdkRequest request, ExecutionAttributes executionAttributes) {
EcsEndpointParams.Builder builder = EcsEndpointParams.builder();
builder.region(AwsEndpointProviderUtils.regionBuiltIn(executionAttributes));
builder.useDualStack(AwsEndpointProviderUtils.dualStackEnabledBuiltIn(executionAttributes));
builder.useFips(AwsEndpointProviderUtils.fipsEnabledBuiltIn(executionAttributes));
builder.endpoint(AwsEndpointProviderUtils.endpointBuiltIn(executionAttributes));
setContextParams(builder, executionAttributes.getAttribute(AwsExecutionAttribute.OPERATION_NAME), request);
setStaticContextParams(builder, executionAttributes.getAttribute(AwsExecutionAttribute.OPERATION_NAME));
return builder.build();
}
private static void setContextParams(EcsEndpointParams.Builder params, String operationName, SdkRequest request) {
}
private static void setStaticContextParams(EcsEndpointParams.Builder params, String operationName) {
}
private SelectedAuthScheme authSchemeWithEndpointSignerProperties(
List endpointAuthSchemes, SelectedAuthScheme selectedAuthScheme) {
for (EndpointAuthScheme endpointAuthScheme : endpointAuthSchemes) {
AuthSchemeOption.Builder option = selectedAuthScheme.authSchemeOption().toBuilder();
if (endpointAuthScheme instanceof SigV4AuthScheme) {
SigV4AuthScheme v4AuthScheme = (SigV4AuthScheme) endpointAuthScheme;
if (v4AuthScheme.isDisableDoubleEncodingSet()) {
option.putSignerProperty(AwsV4HttpSigner.DOUBLE_URL_ENCODE, !v4AuthScheme.disableDoubleEncoding());
}
if (v4AuthScheme.signingRegion() != null) {
option.putSignerProperty(AwsV4HttpSigner.REGION_NAME, v4AuthScheme.signingRegion());
}
if (v4AuthScheme.signingName() != null) {
option.putSignerProperty(AwsV4HttpSigner.SERVICE_SIGNING_NAME, v4AuthScheme.signingName());
}
return new SelectedAuthScheme<>(selectedAuthScheme.identity(), selectedAuthScheme.signer(), option.build());
}
if (endpointAuthScheme instanceof SigV4aAuthScheme) {
SigV4aAuthScheme v4aAuthScheme = (SigV4aAuthScheme) endpointAuthScheme;
if (v4aAuthScheme.isDisableDoubleEncodingSet()) {
option.putSignerProperty(AwsV4aHttpSigner.DOUBLE_URL_ENCODE, !v4aAuthScheme.disableDoubleEncoding());
}
if (v4aAuthScheme.signingRegionSet() != null) {
RegionSet regionSet = RegionSet.create(v4aAuthScheme.signingRegionSet());
option.putSignerProperty(AwsV4aHttpSigner.REGION_SET, regionSet);
}
if (v4aAuthScheme.signingName() != null) {
option.putSignerProperty(AwsV4aHttpSigner.SERVICE_SIGNING_NAME, v4aAuthScheme.signingName());
}
return new SelectedAuthScheme<>(selectedAuthScheme.identity(), selectedAuthScheme.signer(), option.build());
}
throw new IllegalArgumentException("Endpoint auth scheme '" + endpointAuthScheme.name()
+ "' cannot be mapped to the SDK auth scheme. Was it declared in the service's model?");
}
return selectedAuthScheme;
}
private static Optional hostPrefix(String operationName, SdkRequest request) {
return Optional.empty();
}
private Supplier signerProvider(EndpointAuthScheme authScheme) {
switch (authScheme.name()) {
case "sigv4":
return Aws4Signer::create;
case "sigv4a":
return SignerLoader::getSigV4aSigner;
default:
break;
}
throw SdkClientException.create("Don't know how to create signer for auth scheme: " + authScheme.name());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy