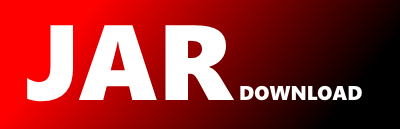
software.amazon.awssdk.services.ecs.model.EFSAuthorizationConfig Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The authorization configuration details for the Amazon EFS file system.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EFSAuthorizationConfig implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ACCESS_POINT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("accessPointId").getter(getter(EFSAuthorizationConfig::accessPointId))
.setter(setter(Builder::accessPointId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("accessPointId").build()).build();
private static final SdkField IAM_FIELD = SdkField. builder(MarshallingType.STRING).memberName("iam")
.getter(getter(EFSAuthorizationConfig::iamAsString)).setter(setter(Builder::iam))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("iam").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCESS_POINT_ID_FIELD,
IAM_FIELD));
private static final long serialVersionUID = 1L;
private final String accessPointId;
private final String iam;
private EFSAuthorizationConfig(BuilderImpl builder) {
this.accessPointId = builder.accessPointId;
this.iam = builder.iam;
}
/**
*
* The Amazon EFS access point ID to use. If an access point is specified, the root directory value specified in the
* EFSVolumeConfiguration
must either be omitted or set to /
which will enforce the path
* set on the EFS access point. If an access point is used, transit encryption must be on in the
* EFSVolumeConfiguration
. For more information, see Working with Amazon EFS access points
* in the Amazon Elastic File System User Guide.
*
*
* @return The Amazon EFS access point ID to use. If an access point is specified, the root directory value
* specified in the EFSVolumeConfiguration
must either be omitted or set to /
* which will enforce the path set on the EFS access point. If an access point is used, transit encryption
* must be on in the EFSVolumeConfiguration
. For more information, see Working with Amazon EFS access
* points in the Amazon Elastic File System User Guide.
*/
public final String accessPointId() {
return accessPointId;
}
/**
*
* Determines whether to use the Amazon ECS task role defined in a task definition when mounting the Amazon EFS file
* system. If it is turned on, transit encryption must be turned on in the EFSVolumeConfiguration
. If
* this parameter is omitted, the default value of DISABLED
is used. For more information, see Using
* Amazon EFS access points in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #iam} will return
* {@link EFSAuthorizationConfigIAM#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #iamAsString}.
*
*
* @return Determines whether to use the Amazon ECS task role defined in a task definition when mounting the Amazon
* EFS file system. If it is turned on, transit encryption must be turned on in the
* EFSVolumeConfiguration
. If this parameter is omitted, the default value of
* DISABLED
is used. For more information, see Using Amazon EFS access points in the Amazon Elastic Container Service Developer Guide.
* @see EFSAuthorizationConfigIAM
*/
public final EFSAuthorizationConfigIAM iam() {
return EFSAuthorizationConfigIAM.fromValue(iam);
}
/**
*
* Determines whether to use the Amazon ECS task role defined in a task definition when mounting the Amazon EFS file
* system. If it is turned on, transit encryption must be turned on in the EFSVolumeConfiguration
. If
* this parameter is omitted, the default value of DISABLED
is used. For more information, see Using
* Amazon EFS access points in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #iam} will return
* {@link EFSAuthorizationConfigIAM#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #iamAsString}.
*
*
* @return Determines whether to use the Amazon ECS task role defined in a task definition when mounting the Amazon
* EFS file system. If it is turned on, transit encryption must be turned on in the
* EFSVolumeConfiguration
. If this parameter is omitted, the default value of
* DISABLED
is used. For more information, see Using Amazon EFS access points in the Amazon Elastic Container Service Developer Guide.
* @see EFSAuthorizationConfigIAM
*/
public final String iamAsString() {
return iam;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(accessPointId());
hashCode = 31 * hashCode + Objects.hashCode(iamAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EFSAuthorizationConfig)) {
return false;
}
EFSAuthorizationConfig other = (EFSAuthorizationConfig) obj;
return Objects.equals(accessPointId(), other.accessPointId()) && Objects.equals(iamAsString(), other.iamAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EFSAuthorizationConfig").add("AccessPointId", accessPointId()).add("Iam", iamAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "accessPointId":
return Optional.ofNullable(clazz.cast(accessPointId()));
case "iam":
return Optional.ofNullable(clazz.cast(iamAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function