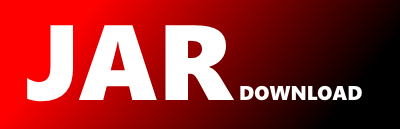
software.amazon.awssdk.services.ecs.model.ServiceConnectService Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The Service Connect service object configuration. For more information, see Service Connect in the
* Amazon Elastic Container Service Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServiceConnectService implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField PORT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("portName").getter(getter(ServiceConnectService::portName)).setter(setter(Builder::portName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("portName").build()).build();
private static final SdkField DISCOVERY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("discoveryName").getter(getter(ServiceConnectService::discoveryName))
.setter(setter(Builder::discoveryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("discoveryName").build()).build();
private static final SdkField> CLIENT_ALIASES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("clientAliases")
.getter(getter(ServiceConnectService::clientAliases))
.setter(setter(Builder::clientAliases))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientAliases").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceConnectClientAlias::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INGRESS_PORT_OVERRIDE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ingressPortOverride").getter(getter(ServiceConnectService::ingressPortOverride))
.setter(setter(Builder::ingressPortOverride))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ingressPortOverride").build())
.build();
private static final SdkField TIMEOUT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("timeout")
.getter(getter(ServiceConnectService::timeout)).setter(setter(Builder::timeout))
.constructor(TimeoutConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeout").build()).build();
private static final SdkField TLS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("tls")
.getter(getter(ServiceConnectService::tls)).setter(setter(Builder::tls))
.constructor(ServiceConnectTlsConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tls").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PORT_NAME_FIELD,
DISCOVERY_NAME_FIELD, CLIENT_ALIASES_FIELD, INGRESS_PORT_OVERRIDE_FIELD, TIMEOUT_FIELD, TLS_FIELD));
private static final long serialVersionUID = 1L;
private final String portName;
private final String discoveryName;
private final List clientAliases;
private final Integer ingressPortOverride;
private final TimeoutConfiguration timeout;
private final ServiceConnectTlsConfiguration tls;
private ServiceConnectService(BuilderImpl builder) {
this.portName = builder.portName;
this.discoveryName = builder.discoveryName;
this.clientAliases = builder.clientAliases;
this.ingressPortOverride = builder.ingressPortOverride;
this.timeout = builder.timeout;
this.tls = builder.tls;
}
/**
*
* The portName
must match the name of one of the portMappings
from all the containers in
* the task definition of this Amazon ECS service.
*
*
* @return The portName
must match the name of one of the portMappings
from all the
* containers in the task definition of this Amazon ECS service.
*/
public final String portName() {
return portName;
}
/**
*
* The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this Amazon
* ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64 characters. The
* name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name can't start with a
* hyphen.
*
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used in
* portName.namespace
.
*
*
* @return The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this
* Amazon ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64
* characters. The name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name
* can't start with a hyphen.
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used
* in portName.namespace
.
*/
public final String discoveryName() {
return discoveryName;
}
/**
* For responses, this returns true if the service returned a value for the ClientAliases property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasClientAliases() {
return clientAliases != null && !(clientAliases instanceof SdkAutoConstructList);
}
/**
*
* The list of client aliases for this Service Connect service. You use these to assign names that can be used by
* client applications. The maximum number of client aliases that you can have in this list is 1.
*
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients") can use
* to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with one
* port
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasClientAliases} method.
*
*
* @return The list of client aliases for this Service Connect service. You use these to assign names that can be
* used by client applications. The maximum number of client aliases that you can have in this list is
* 1.
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients")
* can use to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with
* one port
.
*/
public final List clientAliases() {
return clientAliases;
}
/**
*
* The port number for the Service Connect proxy to listen on.
*
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security groups
* to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container port
* number is in the portMapping
in the task definition. In bridge mode, the default value is the
* ephemeral port of the Service Connect proxy.
*
*
* @return The port number for the Service Connect proxy to listen on.
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security
* groups to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container
* port number is in the portMapping
in the task definition. In bridge mode, the default value
* is the ephemeral port of the Service Connect proxy.
*/
public final Integer ingressPortOverride() {
return ingressPortOverride;
}
/**
*
* A reference to an object that represents the configured timeouts for Service Connect.
*
*
* @return A reference to an object that represents the configured timeouts for Service Connect.
*/
public final TimeoutConfiguration timeout() {
return timeout;
}
/**
*
* A reference to an object that represents a Transport Layer Security (TLS) configuration.
*
*
* @return A reference to an object that represents a Transport Layer Security (TLS) configuration.
*/
public final ServiceConnectTlsConfiguration tls() {
return tls;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(portName());
hashCode = 31 * hashCode + Objects.hashCode(discoveryName());
hashCode = 31 * hashCode + Objects.hashCode(hasClientAliases() ? clientAliases() : null);
hashCode = 31 * hashCode + Objects.hashCode(ingressPortOverride());
hashCode = 31 * hashCode + Objects.hashCode(timeout());
hashCode = 31 * hashCode + Objects.hashCode(tls());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceConnectService)) {
return false;
}
ServiceConnectService other = (ServiceConnectService) obj;
return Objects.equals(portName(), other.portName()) && Objects.equals(discoveryName(), other.discoveryName())
&& hasClientAliases() == other.hasClientAliases() && Objects.equals(clientAliases(), other.clientAliases())
&& Objects.equals(ingressPortOverride(), other.ingressPortOverride())
&& Objects.equals(timeout(), other.timeout()) && Objects.equals(tls(), other.tls());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServiceConnectService").add("PortName", portName()).add("DiscoveryName", discoveryName())
.add("ClientAliases", hasClientAliases() ? clientAliases() : null)
.add("IngressPortOverride", ingressPortOverride()).add("Timeout", timeout()).add("Tls", tls()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "portName":
return Optional.ofNullable(clazz.cast(portName()));
case "discoveryName":
return Optional.ofNullable(clazz.cast(discoveryName()));
case "clientAliases":
return Optional.ofNullable(clazz.cast(clientAliases()));
case "ingressPortOverride":
return Optional.ofNullable(clazz.cast(ingressPortOverride()));
case "timeout":
return Optional.ofNullable(clazz.cast(timeout()));
case "tls":
return Optional.ofNullable(clazz.cast(tls()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function