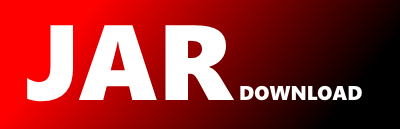
software.amazon.awssdk.services.ecs.model.UpdateServiceRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateServiceRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(UpdateServiceRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField SERVICE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("service")
.getter(getter(UpdateServiceRequest::service)).setter(setter(Builder::service))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("service").build()).build();
private static final SdkField DESIRED_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("desiredCount").getter(getter(UpdateServiceRequest::desiredCount)).setter(setter(Builder::desiredCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("desiredCount").build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinition").getter(getter(UpdateServiceRequest::taskDefinition))
.setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final SdkField> CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviderStrategy")
.getter(getter(UpdateServiceRequest::capacityProviderStrategy))
.setter(setter(Builder::capacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DEPLOYMENT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("deploymentConfiguration")
.getter(getter(UpdateServiceRequest::deploymentConfiguration)).setter(setter(Builder::deploymentConfiguration))
.constructor(DeploymentConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deploymentConfiguration").build())
.build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkConfiguration")
.getter(getter(UpdateServiceRequest::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField> PLACEMENT_CONSTRAINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("placementConstraints")
.getter(getter(UpdateServiceRequest::placementConstraints))
.setter(setter(Builder::placementConstraints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementConstraints").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementConstraint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PLACEMENT_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("placementStrategy")
.getter(getter(UpdateServiceRequest::placementStrategy))
.setter(setter(Builder::placementStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementStrategy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformVersion").getter(getter(UpdateServiceRequest::platformVersion))
.setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField FORCE_NEW_DEPLOYMENT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("forceNewDeployment").getter(getter(UpdateServiceRequest::forceNewDeployment))
.setter(setter(Builder::forceNewDeployment))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("forceNewDeployment").build())
.build();
private static final SdkField HEALTH_CHECK_GRACE_PERIOD_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("healthCheckGracePeriodSeconds")
.getter(getter(UpdateServiceRequest::healthCheckGracePeriodSeconds))
.setter(setter(Builder::healthCheckGracePeriodSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("healthCheckGracePeriodSeconds")
.build()).build();
private static final SdkField ENABLE_EXECUTE_COMMAND_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableExecuteCommand").getter(getter(UpdateServiceRequest::enableExecuteCommand))
.setter(setter(Builder::enableExecuteCommand))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableExecuteCommand").build())
.build();
private static final SdkField ENABLE_ECS_MANAGED_TAGS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enableECSManagedTags").getter(getter(UpdateServiceRequest::enableECSManagedTags))
.setter(setter(Builder::enableECSManagedTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableECSManagedTags").build())
.build();
private static final SdkField> LOAD_BALANCERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("loadBalancers")
.getter(getter(UpdateServiceRequest::loadBalancers))
.setter(setter(Builder::loadBalancers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("loadBalancers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LoadBalancer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PROPAGATE_TAGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("propagateTags").getter(getter(UpdateServiceRequest::propagateTagsAsString))
.setter(setter(Builder::propagateTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("propagateTags").build()).build();
private static final SdkField> SERVICE_REGISTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("serviceRegistries")
.getter(getter(UpdateServiceRequest::serviceRegistries))
.setter(setter(Builder::serviceRegistries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRegistries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceRegistry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SERVICE_CONNECT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("serviceConnectConfiguration")
.getter(getter(UpdateServiceRequest::serviceConnectConfiguration))
.setter(setter(Builder::serviceConnectConfiguration))
.constructor(ServiceConnectConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceConnectConfiguration")
.build()).build();
private static final SdkField> VOLUME_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("volumeConfigurations")
.getter(getter(UpdateServiceRequest::volumeConfigurations))
.setter(setter(Builder::volumeConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("volumeConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceVolumeConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD, SERVICE_FIELD,
DESIRED_COUNT_FIELD, TASK_DEFINITION_FIELD, CAPACITY_PROVIDER_STRATEGY_FIELD, DEPLOYMENT_CONFIGURATION_FIELD,
NETWORK_CONFIGURATION_FIELD, PLACEMENT_CONSTRAINTS_FIELD, PLACEMENT_STRATEGY_FIELD, PLATFORM_VERSION_FIELD,
FORCE_NEW_DEPLOYMENT_FIELD, HEALTH_CHECK_GRACE_PERIOD_SECONDS_FIELD, ENABLE_EXECUTE_COMMAND_FIELD,
ENABLE_ECS_MANAGED_TAGS_FIELD, LOAD_BALANCERS_FIELD, PROPAGATE_TAGS_FIELD, SERVICE_REGISTRIES_FIELD,
SERVICE_CONNECT_CONFIGURATION_FIELD, VOLUME_CONFIGURATIONS_FIELD));
private final String cluster;
private final String service;
private final Integer desiredCount;
private final String taskDefinition;
private final List capacityProviderStrategy;
private final DeploymentConfiguration deploymentConfiguration;
private final NetworkConfiguration networkConfiguration;
private final List placementConstraints;
private final List placementStrategy;
private final String platformVersion;
private final Boolean forceNewDeployment;
private final Integer healthCheckGracePeriodSeconds;
private final Boolean enableExecuteCommand;
private final Boolean enableECSManagedTags;
private final List loadBalancers;
private final String propagateTags;
private final List serviceRegistries;
private final ServiceConnectConfiguration serviceConnectConfiguration;
private final List volumeConfigurations;
private UpdateServiceRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.service = builder.service;
this.desiredCount = builder.desiredCount;
this.taskDefinition = builder.taskDefinition;
this.capacityProviderStrategy = builder.capacityProviderStrategy;
this.deploymentConfiguration = builder.deploymentConfiguration;
this.networkConfiguration = builder.networkConfiguration;
this.placementConstraints = builder.placementConstraints;
this.placementStrategy = builder.placementStrategy;
this.platformVersion = builder.platformVersion;
this.forceNewDeployment = builder.forceNewDeployment;
this.healthCheckGracePeriodSeconds = builder.healthCheckGracePeriodSeconds;
this.enableExecuteCommand = builder.enableExecuteCommand;
this.enableECSManagedTags = builder.enableECSManagedTags;
this.loadBalancers = builder.loadBalancers;
this.propagateTags = builder.propagateTags;
this.serviceRegistries = builder.serviceRegistries;
this.serviceConnectConfiguration = builder.serviceConnectConfiguration;
this.volumeConfigurations = builder.volumeConfigurations;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster that your service runs on. If you do not specify
* a cluster, the default cluster is assumed.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster that your service runs on. If you do not
* specify a cluster, the default cluster is assumed.
*/
public final String cluster() {
return cluster;
}
/**
*
* The name of the service to update.
*
*
* @return The name of the service to update.
*/
public final String service() {
return service;
}
/**
*
* The number of instantiations of the task to place and keep running in your service.
*
*
* @return The number of instantiations of the task to place and keep running in your service.
*/
public final Integer desiredCount() {
return desiredCount;
}
/**
*
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run in your service. If a revision
is not specified, the latest ACTIVE
* revision is used. If you modify the task definition with UpdateService
, Amazon ECS spawns a task
* with the new version of the task definition and then stops an old task after the new version is running.
*
*
* @return The family
and revision
(family:revision
) or full ARN of the task
* definition to run in your service. If a revision
is not specified, the latest
* ACTIVE
revision is used. If you modify the task definition with UpdateService
,
* Amazon ECS spawns a task with the new version of the task definition and then stops an old task after the
* new version is running.
*/
public final String taskDefinition() {
return taskDefinition;
}
/**
* For responses, this returns true if the service returned a value for the CapacityProviderStrategy property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCapacityProviderStrategy() {
return capacityProviderStrategy != null && !(capacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The capacity provider strategy to update the service to use.
*
*
* if the service uses the default capacity provider strategy for the cluster, the service can be updated to use one
* or more capacity providers as opposed to the default capacity provider strategy. However, when a service is using
* a capacity provider strategy that's not the default capacity provider strategy, the service can't be updated to
* use the cluster's default capacity provider strategy.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the base
and
* weight
to assign to them. A capacity provider must be associated with the cluster to be used in a
* capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider
* with a cluster. Only capacity providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be created.
* New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
capacity
* providers. The Fargate capacity providers are available to all accounts and only need to be associated with a
* cluster to be used.
*
*
* The PutClusterCapacityProviders API operation is used to update the list of available capacity providers
* for a cluster after the cluster is created.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapacityProviderStrategy} method.
*
*
* @return The capacity provider strategy to update the service to use.
*
* if the service uses the default capacity provider strategy for the cluster, the service can be updated to
* use one or more capacity providers as opposed to the default capacity provider strategy. However, when a
* service is using a capacity provider strategy that's not the default capacity provider strategy, the
* service can't be updated to use the cluster's default capacity provider strategy.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the base
* and weight
to assign to them. A capacity provider must be associated with the cluster to be
* used in a capacity provider strategy. The PutClusterCapacityProviders API is used to associate a
* capacity provider with a cluster. Only capacity providers with an ACTIVE
or
* UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be
* created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
* capacity providers. The Fargate capacity providers are available to all accounts and only need to be
* associated with a cluster to be used.
*
*
* The PutClusterCapacityProviders API operation is used to update the list of available capacity
* providers for a cluster after the cluster is created.
*
*/
public final List capacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @return Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
*/
public final DeploymentConfiguration deploymentConfiguration() {
return deploymentConfiguration;
}
/**
*
* An object representing the network configuration for the service.
*
*
* @return An object representing the network configuration for the service.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the PlacementConstraints property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPlacementConstraints() {
return placementConstraints != null && !(placementConstraints instanceof SdkAutoConstructList);
}
/**
*
* An array of task placement constraint objects to update the service to use. If no value is specified, the
* existing placement constraints for the service will remain unchanged. If this value is specified, it will
* override any existing placement constraints defined for the service. To remove all existing placement
* constraints, specify an empty array.
*
*
* You can specify a maximum of 10 constraints for each task. This limit includes constraints in the task definition
* and those specified at runtime.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPlacementConstraints} method.
*
*
* @return An array of task placement constraint objects to update the service to use. If no value is specified, the
* existing placement constraints for the service will remain unchanged. If this value is specified, it will
* override any existing placement constraints defined for the service. To remove all existing placement
* constraints, specify an empty array.
*
* You can specify a maximum of 10 constraints for each task. This limit includes constraints in the task
* definition and those specified at runtime.
*/
public final List placementConstraints() {
return placementConstraints;
}
/**
* For responses, this returns true if the service returned a value for the PlacementStrategy property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPlacementStrategy() {
return placementStrategy != null && !(placementStrategy instanceof SdkAutoConstructList);
}
/**
*
* The task placement strategy objects to update the service to use. If no value is specified, the existing
* placement strategy for the service will remain unchanged. If this value is specified, it will override the
* existing placement strategy defined for the service. To remove an existing placement strategy, specify an empty
* object.
*
*
* You can specify a maximum of five strategy rules for each service.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPlacementStrategy} method.
*
*
* @return The task placement strategy objects to update the service to use. If no value is specified, the existing
* placement strategy for the service will remain unchanged. If this value is specified, it will override
* the existing placement strategy defined for the service. To remove an existing placement strategy,
* specify an empty object.
*
* You can specify a maximum of five strategy rules for each service.
*/
public final List placementStrategy() {
return placementStrategy;
}
/**
*
* The platform version that your tasks in the service run on. A platform version is only specified for tasks using
* the Fargate launch type. If a platform version is not specified, the LATEST
platform version is
* used. For more information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version that your tasks in the service run on. A platform version is only specified for
* tasks using the Fargate launch type. If a platform version is not specified, the LATEST
* platform version is used. For more information, see Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* Determines whether to force a new deployment of the service. By default, deployments aren't forced. You can use
* this option to start a new deployment with no service definition changes. For example, you can update a service's
* tasks to use a newer Docker image with the same image/tag combination (my_image:latest
) or to roll
* Fargate tasks onto a newer platform version.
*
*
* @return Determines whether to force a new deployment of the service. By default, deployments aren't forced. You
* can use this option to start a new deployment with no service definition changes. For example, you can
* update a service's tasks to use a newer Docker image with the same image/tag combination (
* my_image:latest
) or to roll Fargate tasks onto a newer platform version.
*/
public final Boolean forceNewDeployment() {
return forceNewDeployment;
}
/**
*
* The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load Balancing
* target health checks after a task has first started. This is only valid if your service is configured to use a
* load balancer. If your service's tasks take a while to start and respond to Elastic Load Balancing health checks,
* you can specify a health check grace period of up to 2,147,483,647 seconds. During that time, the Amazon ECS
* service scheduler ignores the Elastic Load Balancing health check status. This grace period can prevent the ECS
* service scheduler from marking tasks as unhealthy and stopping them before they have time to come up.
*
*
* @return The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load
* Balancing target health checks after a task has first started. This is only valid if your service is
* configured to use a load balancer. If your service's tasks take a while to start and respond to Elastic
* Load Balancing health checks, you can specify a health check grace period of up to 2,147,483,647 seconds.
* During that time, the Amazon ECS service scheduler ignores the Elastic Load Balancing health check
* status. This grace period can prevent the ECS service scheduler from marking tasks as unhealthy and
* stopping them before they have time to come up.
*/
public final Integer healthCheckGracePeriodSeconds() {
return healthCheckGracePeriodSeconds;
}
/**
*
* If true
, this enables execute command functionality on all task containers.
*
*
* If you do not want to override the value that was set when the service was created, you can set this to
* null
when performing this action.
*
*
* @return If true
, this enables execute command functionality on all task containers.
*
* If you do not want to override the value that was set when the service was created, you can set this to
* null
when performing this action.
*/
public final Boolean enableExecuteCommand() {
return enableExecuteCommand;
}
/**
*
* Determines whether to turn on Amazon ECS managed tags for the tasks in the service. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* Only tasks launched after the update will reflect the update. To update the tags on all tasks, set
* forceNewDeployment
to true
, so that Amazon ECS starts new tasks with the updated tags.
*
*
* @return Determines whether to turn on Amazon ECS managed tags for the tasks in the service. For more information,
* see Tagging
* Your Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*
* Only tasks launched after the update will reflect the update. To update the tags on all tasks, set
* forceNewDeployment
to true
, so that Amazon ECS starts new tasks with the
* updated tags.
*/
public final Boolean enableECSManagedTags() {
return enableECSManagedTags;
}
/**
* For responses, this returns true if the service returned a value for the LoadBalancers property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLoadBalancers() {
return loadBalancers != null && !(loadBalancers instanceof SdkAutoConstructList);
}
/**
*
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container name,
* and the container port to access from the load balancer. The container name is as it appears in a container
* definition.
*
*
* When you add, update, or remove a load balancer configuration, Amazon ECS starts new tasks with the updated
* Elastic Load Balancing configuration, and then stops the old tasks when the new tasks are running.
*
*
* For services that use rolling updates, you can add, update, or remove Elastic Load Balancing target groups. You
* can update from a single target group to multiple target groups and from multiple target groups to a single
* target group.
*
*
* For services that use blue/green deployments, you can update Elastic Load Balancing target groups by using
* CreateDeployment
* through CodeDeploy. Note that multiple target groups are not supported for blue/green deployments. For more
* information see Register
* multiple target groups with a service in the Amazon Elastic Container Service Developer Guide.
*
*
* For services that use the external deployment controller, you can add, update, or remove load balancers by using
* CreateTaskSet.
* Note that multiple target groups are not supported for external deployments. For more information see Register
* multiple target groups with a service in the Amazon Elastic Container Service Developer Guide.
*
*
* You can remove existing loadBalancers
by passing an empty list.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLoadBalancers} method.
*
*
* @return A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container
* name, and the container port to access from the load balancer. The container name is as it appears in a
* container definition.
*
* When you add, update, or remove a load balancer configuration, Amazon ECS starts new tasks with the
* updated Elastic Load Balancing configuration, and then stops the old tasks when the new tasks are
* running.
*
*
* For services that use rolling updates, you can add, update, or remove Elastic Load Balancing target
* groups. You can update from a single target group to multiple target groups and from multiple target
* groups to a single target group.
*
*
* For services that use blue/green deployments, you can update Elastic Load Balancing target groups by
* using
* CreateDeployment
* through CodeDeploy. Note that multiple target groups are not supported for blue/green deployments. For
* more information see Register multiple target groups with a service in the Amazon Elastic Container Service Developer
* Guide.
*
*
* For services that use the external deployment controller, you can add, update, or remove load balancers
* by using CreateTaskSet
* . Note that multiple target groups are not supported for external deployments. For more information
* see Register multiple target groups with a service in the Amazon Elastic Container Service Developer
* Guide.
*
*
* You can remove existing loadBalancers
by passing an empty list.
*/
public final List loadBalancers() {
return loadBalancers;
}
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* Only tasks launched after the update will reflect the update. To update the tags on all tasks, set
* forceNewDeployment
to true
, so that Amazon ECS starts new tasks with the updated tags.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Determines whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
*
* Only tasks launched after the update will reflect the update. To update the tags on all tasks, set
* forceNewDeployment
to true
, so that Amazon ECS starts new tasks with the
* updated tags.
* @see PropagateTags
*/
public final PropagateTags propagateTags() {
return PropagateTags.fromValue(propagateTags);
}
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* Only tasks launched after the update will reflect the update. To update the tags on all tasks, set
* forceNewDeployment
to true
, so that Amazon ECS starts new tasks with the updated tags.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Determines whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
*
* Only tasks launched after the update will reflect the update. To update the tags on all tasks, set
* forceNewDeployment
to true
, so that Amazon ECS starts new tasks with the
* updated tags.
* @see PropagateTags
*/
public final String propagateTagsAsString() {
return propagateTags;
}
/**
* For responses, this returns true if the service returned a value for the ServiceRegistries property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasServiceRegistries() {
return serviceRegistries != null && !(serviceRegistries instanceof SdkAutoConstructList);
}
/**
*
* The details for the service discovery registries to assign to this service. For more information, see Service Discovery.
*
*
* When you add, update, or remove the service registries configuration, Amazon ECS starts new tasks with the
* updated service registries configuration, and then stops the old tasks when the new tasks are running.
*
*
* You can remove existing serviceRegistries
by passing an empty list.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasServiceRegistries} method.
*
*
* @return The details for the service discovery registries to assign to this service. For more information, see Service
* Discovery.
*
* When you add, update, or remove the service registries configuration, Amazon ECS starts new tasks with
* the updated service registries configuration, and then stops the old tasks when the new tasks are
* running.
*
*
* You can remove existing serviceRegistries
by passing an empty list.
*/
public final List serviceRegistries() {
return serviceRegistries;
}
/**
*
* The configuration for this service to discover and connect to services, and be discovered by, and connected from,
* other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The configuration for this service to discover and connect to services, and be discovered by, and
* connected from, other services within a namespace.
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
*/
public final ServiceConnectConfiguration serviceConnectConfiguration() {
return serviceConnectConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the VolumeConfigurations property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVolumeConfigurations() {
return volumeConfigurations != null && !(volumeConfigurations instanceof SdkAutoConstructList);
}
/**
*
* The details of the volume that was configuredAtLaunch
. You can configure the size, volumeType, IOPS,
* throughput, snapshot and encryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the name
* from the task definition. If set to null, no new deployment is triggered. Otherwise, if this configuration
* differs from the existing one, it triggers a new deployment.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVolumeConfigurations} method.
*
*
* @return The details of the volume that was configuredAtLaunch
. You can configure the size,
* volumeType, IOPS, throughput, snapshot and encryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the
* name
from the task definition. If set to null, no new deployment is triggered. Otherwise, if
* this configuration differs from the existing one, it triggers a new deployment.
*/
public final List volumeConfigurations() {
return volumeConfigurations;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(service());
hashCode = 31 * hashCode + Objects.hashCode(desiredCount());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviderStrategy() ? capacityProviderStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(deploymentConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementConstraints() ? placementConstraints() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementStrategy() ? placementStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(forceNewDeployment());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckGracePeriodSeconds());
hashCode = 31 * hashCode + Objects.hashCode(enableExecuteCommand());
hashCode = 31 * hashCode + Objects.hashCode(enableECSManagedTags());
hashCode = 31 * hashCode + Objects.hashCode(hasLoadBalancers() ? loadBalancers() : null);
hashCode = 31 * hashCode + Objects.hashCode(propagateTagsAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasServiceRegistries() ? serviceRegistries() : null);
hashCode = 31 * hashCode + Objects.hashCode(serviceConnectConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasVolumeConfigurations() ? volumeConfigurations() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateServiceRequest)) {
return false;
}
UpdateServiceRequest other = (UpdateServiceRequest) obj;
return Objects.equals(cluster(), other.cluster()) && Objects.equals(service(), other.service())
&& Objects.equals(desiredCount(), other.desiredCount())
&& Objects.equals(taskDefinition(), other.taskDefinition())
&& hasCapacityProviderStrategy() == other.hasCapacityProviderStrategy()
&& Objects.equals(capacityProviderStrategy(), other.capacityProviderStrategy())
&& Objects.equals(deploymentConfiguration(), other.deploymentConfiguration())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& hasPlacementConstraints() == other.hasPlacementConstraints()
&& Objects.equals(placementConstraints(), other.placementConstraints())
&& hasPlacementStrategy() == other.hasPlacementStrategy()
&& Objects.equals(placementStrategy(), other.placementStrategy())
&& Objects.equals(platformVersion(), other.platformVersion())
&& Objects.equals(forceNewDeployment(), other.forceNewDeployment())
&& Objects.equals(healthCheckGracePeriodSeconds(), other.healthCheckGracePeriodSeconds())
&& Objects.equals(enableExecuteCommand(), other.enableExecuteCommand())
&& Objects.equals(enableECSManagedTags(), other.enableECSManagedTags())
&& hasLoadBalancers() == other.hasLoadBalancers() && Objects.equals(loadBalancers(), other.loadBalancers())
&& Objects.equals(propagateTagsAsString(), other.propagateTagsAsString())
&& hasServiceRegistries() == other.hasServiceRegistries()
&& Objects.equals(serviceRegistries(), other.serviceRegistries())
&& Objects.equals(serviceConnectConfiguration(), other.serviceConnectConfiguration())
&& hasVolumeConfigurations() == other.hasVolumeConfigurations()
&& Objects.equals(volumeConfigurations(), other.volumeConfigurations());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateServiceRequest").add("Cluster", cluster()).add("Service", service())
.add("DesiredCount", desiredCount()).add("TaskDefinition", taskDefinition())
.add("CapacityProviderStrategy", hasCapacityProviderStrategy() ? capacityProviderStrategy() : null)
.add("DeploymentConfiguration", deploymentConfiguration()).add("NetworkConfiguration", networkConfiguration())
.add("PlacementConstraints", hasPlacementConstraints() ? placementConstraints() : null)
.add("PlacementStrategy", hasPlacementStrategy() ? placementStrategy() : null)
.add("PlatformVersion", platformVersion()).add("ForceNewDeployment", forceNewDeployment())
.add("HealthCheckGracePeriodSeconds", healthCheckGracePeriodSeconds())
.add("EnableExecuteCommand", enableExecuteCommand()).add("EnableECSManagedTags", enableECSManagedTags())
.add("LoadBalancers", hasLoadBalancers() ? loadBalancers() : null).add("PropagateTags", propagateTagsAsString())
.add("ServiceRegistries", hasServiceRegistries() ? serviceRegistries() : null)
.add("ServiceConnectConfiguration", serviceConnectConfiguration())
.add("VolumeConfigurations", hasVolumeConfigurations() ? volumeConfigurations() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "service":
return Optional.ofNullable(clazz.cast(service()));
case "desiredCount":
return Optional.ofNullable(clazz.cast(desiredCount()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
case "capacityProviderStrategy":
return Optional.ofNullable(clazz.cast(capacityProviderStrategy()));
case "deploymentConfiguration":
return Optional.ofNullable(clazz.cast(deploymentConfiguration()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "placementConstraints":
return Optional.ofNullable(clazz.cast(placementConstraints()));
case "placementStrategy":
return Optional.ofNullable(clazz.cast(placementStrategy()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "forceNewDeployment":
return Optional.ofNullable(clazz.cast(forceNewDeployment()));
case "healthCheckGracePeriodSeconds":
return Optional.ofNullable(clazz.cast(healthCheckGracePeriodSeconds()));
case "enableExecuteCommand":
return Optional.ofNullable(clazz.cast(enableExecuteCommand()));
case "enableECSManagedTags":
return Optional.ofNullable(clazz.cast(enableECSManagedTags()));
case "loadBalancers":
return Optional.ofNullable(clazz.cast(loadBalancers()));
case "propagateTags":
return Optional.ofNullable(clazz.cast(propagateTagsAsString()));
case "serviceRegistries":
return Optional.ofNullable(clazz.cast(serviceRegistries()));
case "serviceConnectConfiguration":
return Optional.ofNullable(clazz.cast(serviceConnectConfiguration()));
case "volumeConfigurations":
return Optional.ofNullable(clazz.cast(volumeConfigurations()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function