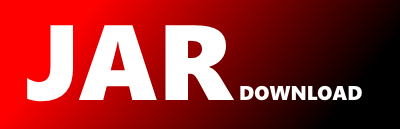
software.amazon.awssdk.services.ecs.model.Session Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The AWS Java SDK for the Amazon EC2 Container Service holds the client classes that are used for
communicating with the
Amazon EC2 Container Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details for the execute command session.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Session implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SESSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sessionId").getter(getter(Session::sessionId)).setter(setter(Builder::sessionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sessionId").build()).build();
private static final SdkField STREAM_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("streamUrl").getter(getter(Session::streamUrl)).setter(setter(Builder::streamUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("streamUrl").build()).build();
private static final SdkField TOKEN_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("tokenValue").getter(getter(Session::tokenValue)).setter(setter(Builder::tokenValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tokenValue").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SESSION_ID_FIELD,
STREAM_URL_FIELD, TOKEN_VALUE_FIELD));
private static final long serialVersionUID = 1L;
private final String sessionId;
private final String streamUrl;
private final String tokenValue;
private Session(BuilderImpl builder) {
this.sessionId = builder.sessionId;
this.streamUrl = builder.streamUrl;
this.tokenValue = builder.tokenValue;
}
/**
*
* The ID of the execute command session.
*
*
* @return The ID of the execute command session.
*/
public final String sessionId() {
return sessionId;
}
/**
*
* A URL to the managed agent on the container that the SSM Session Manager client uses to send commands and receive
* output from the container.
*
*
* @return A URL to the managed agent on the container that the SSM Session Manager client uses to send commands and
* receive output from the container.
*/
public final String streamUrl() {
return streamUrl;
}
/**
*
* An encrypted token value containing session and caller information. It's used to authenticate the connection to
* the container.
*
*
* @return An encrypted token value containing session and caller information. It's used to authenticate the
* connection to the container.
*/
public final String tokenValue() {
return tokenValue;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(sessionId());
hashCode = 31 * hashCode + Objects.hashCode(streamUrl());
hashCode = 31 * hashCode + Objects.hashCode(tokenValue());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Session)) {
return false;
}
Session other = (Session) obj;
return Objects.equals(sessionId(), other.sessionId()) && Objects.equals(streamUrl(), other.streamUrl())
&& Objects.equals(tokenValue(), other.tokenValue());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Session").add("SessionId", sessionId()).add("StreamUrl", streamUrl())
.add("TokenValue", tokenValue() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "sessionId":
return Optional.ofNullable(clazz.cast(sessionId()));
case "streamUrl":
return Optional.ofNullable(clazz.cast(streamUrl()));
case "tokenValue":
return Optional.ofNullable(clazz.cast(tokenValue()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy