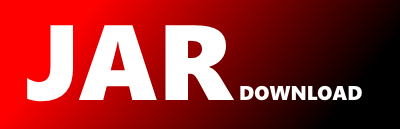
software.amazon.awssdk.services.ecs.model.TaskDefinition Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of a task definition which describes the container and volume definitions of an Amazon Elastic Container
* Service task. You can specify which Docker images to use, the required resources, and other configurations related to
* launching the task definition through an Amazon ECS service or task.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TaskDefinition implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TASK_DEFINITION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinitionArn").getter(getter(TaskDefinition::taskDefinitionArn))
.setter(setter(Builder::taskDefinitionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinitionArn").build()).build();
private static final SdkField> CONTAINER_DEFINITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("containerDefinitions")
.getter(getter(TaskDefinition::containerDefinitions))
.setter(setter(Builder::containerDefinitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerDefinitions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ContainerDefinition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FAMILY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("family")
.getter(getter(TaskDefinition::family)).setter(setter(Builder::family))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("family").build()).build();
private static final SdkField TASK_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskRoleArn").getter(getter(TaskDefinition::taskRoleArn)).setter(setter(Builder::taskRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskRoleArn").build()).build();
private static final SdkField EXECUTION_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("executionRoleArn").getter(getter(TaskDefinition::executionRoleArn))
.setter(setter(Builder::executionRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionRoleArn").build()).build();
private static final SdkField NETWORK_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("networkMode").getter(getter(TaskDefinition::networkModeAsString)).setter(setter(Builder::networkMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkMode").build()).build();
private static final SdkField REVISION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("revision").getter(getter(TaskDefinition::revision)).setter(setter(Builder::revision))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("revision").build()).build();
private static final SdkField> VOLUMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("volumes")
.getter(getter(TaskDefinition::volumes))
.setter(setter(Builder::volumes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("volumes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Volume::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(TaskDefinition::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField> REQUIRES_ATTRIBUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("requiresAttributes")
.getter(getter(TaskDefinition::requiresAttributes))
.setter(setter(Builder::requiresAttributes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("requiresAttributes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Attribute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PLACEMENT_CONSTRAINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("placementConstraints")
.getter(getter(TaskDefinition::placementConstraints))
.setter(setter(Builder::placementConstraints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementConstraints").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TaskDefinitionPlacementConstraint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> COMPATIBILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("compatibilities")
.getter(getter(TaskDefinition::compatibilitiesAsStrings))
.setter(setter(Builder::compatibilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("compatibilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RUNTIME_PLATFORM_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("runtimePlatform")
.getter(getter(TaskDefinition::runtimePlatform)).setter(setter(Builder::runtimePlatform))
.constructor(RuntimePlatform::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runtimePlatform").build()).build();
private static final SdkField> REQUIRES_COMPATIBILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("requiresCompatibilities")
.getter(getter(TaskDefinition::requiresCompatibilitiesAsStrings))
.setter(setter(Builder::requiresCompatibilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("requiresCompatibilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CPU_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cpu")
.getter(getter(TaskDefinition::cpu)).setter(setter(Builder::cpu))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cpu").build()).build();
private static final SdkField MEMORY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("memory")
.getter(getter(TaskDefinition::memory)).setter(setter(Builder::memory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memory").build()).build();
private static final SdkField> INFERENCE_ACCELERATORS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("inferenceAccelerators")
.getter(getter(TaskDefinition::inferenceAccelerators))
.setter(setter(Builder::inferenceAccelerators))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("inferenceAccelerators").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InferenceAccelerator::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PID_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("pidMode").getter(getter(TaskDefinition::pidModeAsString)).setter(setter(Builder::pidMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pidMode").build()).build();
private static final SdkField IPC_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ipcMode").getter(getter(TaskDefinition::ipcModeAsString)).setter(setter(Builder::ipcMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ipcMode").build()).build();
private static final SdkField PROXY_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("proxyConfiguration")
.getter(getter(TaskDefinition::proxyConfiguration)).setter(setter(Builder::proxyConfiguration))
.constructor(ProxyConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("proxyConfiguration").build())
.build();
private static final SdkField REGISTERED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("registeredAt").getter(getter(TaskDefinition::registeredAt)).setter(setter(Builder::registeredAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registeredAt").build()).build();
private static final SdkField DEREGISTERED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("deregisteredAt").getter(getter(TaskDefinition::deregisteredAt)).setter(setter(Builder::deregisteredAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deregisteredAt").build()).build();
private static final SdkField REGISTERED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("registeredBy").getter(getter(TaskDefinition::registeredBy)).setter(setter(Builder::registeredBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registeredBy").build()).build();
private static final SdkField EPHEMERAL_STORAGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ephemeralStorage")
.getter(getter(TaskDefinition::ephemeralStorage)).setter(setter(Builder::ephemeralStorage))
.constructor(EphemeralStorage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ephemeralStorage").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TASK_DEFINITION_ARN_FIELD,
CONTAINER_DEFINITIONS_FIELD, FAMILY_FIELD, TASK_ROLE_ARN_FIELD, EXECUTION_ROLE_ARN_FIELD, NETWORK_MODE_FIELD,
REVISION_FIELD, VOLUMES_FIELD, STATUS_FIELD, REQUIRES_ATTRIBUTES_FIELD, PLACEMENT_CONSTRAINTS_FIELD,
COMPATIBILITIES_FIELD, RUNTIME_PLATFORM_FIELD, REQUIRES_COMPATIBILITIES_FIELD, CPU_FIELD, MEMORY_FIELD,
INFERENCE_ACCELERATORS_FIELD, PID_MODE_FIELD, IPC_MODE_FIELD, PROXY_CONFIGURATION_FIELD, REGISTERED_AT_FIELD,
DEREGISTERED_AT_FIELD, REGISTERED_BY_FIELD, EPHEMERAL_STORAGE_FIELD));
private static final long serialVersionUID = 1L;
private final String taskDefinitionArn;
private final List containerDefinitions;
private final String family;
private final String taskRoleArn;
private final String executionRoleArn;
private final String networkMode;
private final Integer revision;
private final List volumes;
private final String status;
private final List requiresAttributes;
private final List placementConstraints;
private final List compatibilities;
private final RuntimePlatform runtimePlatform;
private final List requiresCompatibilities;
private final String cpu;
private final String memory;
private final List inferenceAccelerators;
private final String pidMode;
private final String ipcMode;
private final ProxyConfiguration proxyConfiguration;
private final Instant registeredAt;
private final Instant deregisteredAt;
private final String registeredBy;
private final EphemeralStorage ephemeralStorage;
private TaskDefinition(BuilderImpl builder) {
this.taskDefinitionArn = builder.taskDefinitionArn;
this.containerDefinitions = builder.containerDefinitions;
this.family = builder.family;
this.taskRoleArn = builder.taskRoleArn;
this.executionRoleArn = builder.executionRoleArn;
this.networkMode = builder.networkMode;
this.revision = builder.revision;
this.volumes = builder.volumes;
this.status = builder.status;
this.requiresAttributes = builder.requiresAttributes;
this.placementConstraints = builder.placementConstraints;
this.compatibilities = builder.compatibilities;
this.runtimePlatform = builder.runtimePlatform;
this.requiresCompatibilities = builder.requiresCompatibilities;
this.cpu = builder.cpu;
this.memory = builder.memory;
this.inferenceAccelerators = builder.inferenceAccelerators;
this.pidMode = builder.pidMode;
this.ipcMode = builder.ipcMode;
this.proxyConfiguration = builder.proxyConfiguration;
this.registeredAt = builder.registeredAt;
this.deregisteredAt = builder.deregisteredAt;
this.registeredBy = builder.registeredBy;
this.ephemeralStorage = builder.ephemeralStorage;
}
/**
*
* The full Amazon Resource Name (ARN) of the task definition.
*
*
* @return The full Amazon Resource Name (ARN) of the task definition.
*/
public final String taskDefinitionArn() {
return taskDefinitionArn;
}
/**
* For responses, this returns true if the service returned a value for the ContainerDefinitions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasContainerDefinitions() {
return containerDefinitions != null && !(containerDefinitions instanceof SdkAutoConstructList);
}
/**
*
* A list of container definitions in JSON format that describe the different containers that make up your task. For
* more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasContainerDefinitions} method.
*
*
* @return A list of container definitions in JSON format that describe the different containers that make up your
* task. For more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*/
public final List containerDefinitions() {
return containerDefinitions;
}
/**
*
* The name of a family that this task definition is registered to. Up to 255 characters are allowed. Letters (both
* uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are allowed.
*
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that you
* registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each task
* definition that you add.
*
*
* @return The name of a family that this task definition is registered to. Up to 255 characters are allowed.
* Letters (both uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are
* allowed.
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that
* you registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each
* task definition that you add.
*/
public final String family() {
return family;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information, see Amazon ECS Task Role
* in the Amazon Elastic Container Service Developer Guide.
*
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you launch the
* Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use the feature. For
* more information, see Windows IAM roles
* for tasks in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information,
* see Amazon ECS
* Task Role in the Amazon Elastic Container Service Developer Guide.
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you
* launch the Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use
* the feature. For more information, see Windows
* IAM roles for tasks in the Amazon Elastic Container Service Developer Guide.
*/
public final String taskRoleArn() {
return taskRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent permission
* to make Amazon Web Services API calls on your behalf. The task execution IAM role is required depending on the
* requirements of your task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent
* permission to make Amazon Web Services API calls on your behalf. The task execution IAM role is required
* depending on the requirements of your task. For more information, see Amazon
* ECS task execution IAM role in the Amazon Elastic Container Service Developer Guide.
*/
public final String executionRoleArn() {
return executionRoleArn;
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the default is
* bridge
.
*
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on Amazon
* EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows instances,
* <default>
or awsvpc
can be used. If the network mode is set to none
,
* you cannot specify port mappings in your container definitions, and the tasks containers do not have external
* connectivity. The host
and awsvpc
network modes offer the highest networking
* performance for containers because they use the EC2 network stack instead of the virtualized network stack
* provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0). It is
* considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkMode} will
* return {@link NetworkMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkModeAsString}.
*
*
* @return The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the
* default is bridge
.
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks
* on Amazon EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows
* instances, <default>
or awsvpc
can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of
* dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0).
* It is considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* For more information, see Network settings in the
* Docker run reference.
* @see NetworkMode
*/
public final NetworkMode networkMode() {
return NetworkMode.fromValue(networkMode);
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the default is
* bridge
.
*
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on Amazon
* EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows instances,
* <default>
or awsvpc
can be used. If the network mode is set to none
,
* you cannot specify port mappings in your container definitions, and the tasks containers do not have external
* connectivity. The host
and awsvpc
network modes offer the highest networking
* performance for containers because they use the EC2 network stack instead of the virtualized network stack
* provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0). It is
* considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkMode} will
* return {@link NetworkMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkModeAsString}.
*
*
* @return The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the
* default is bridge
.
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks
* on Amazon EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows
* instances, <default>
or awsvpc
can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of
* dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0).
* It is considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* For more information, see Network settings in the
* Docker run reference.
* @see NetworkMode
*/
public final String networkModeAsString() {
return networkMode;
}
/**
*
* The revision of the task in a particular family. The revision is a version number of a task definition in a
* family. When you register a task definition for the first time, the revision is 1
. Each time that
* you register a new revision of a task definition in the same family, the revision value always increases by one.
* This is even if you deregistered previous revisions in this family.
*
*
* @return The revision of the task in a particular family. The revision is a version number of a task definition in
* a family. When you register a task definition for the first time, the revision is 1
. Each
* time that you register a new revision of a task definition in the same family, the revision value always
* increases by one. This is even if you deregistered previous revisions in this family.
*/
public final Integer revision() {
return revision;
}
/**
* For responses, this returns true if the service returned a value for the Volumes property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasVolumes() {
return volumes != null && !(volumes instanceof SdkAutoConstructList);
}
/**
*
* The list of data volume definitions for the task. For more information, see Using data volumes in
* tasks in the Amazon Elastic Container Service Developer Guide.
*
*
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVolumes} method.
*
*
* @return The list of data volume definitions for the task. For more information, see Using data
* volumes in tasks in the Amazon Elastic Container Service Developer Guide.
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*/
public final List volumes() {
return volumes;
}
/**
*
* The status of the task definition.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TaskDefinitionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the task definition.
* @see TaskDefinitionStatus
*/
public final TaskDefinitionStatus status() {
return TaskDefinitionStatus.fromValue(status);
}
/**
*
* The status of the task definition.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TaskDefinitionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the task definition.
* @see TaskDefinitionStatus
*/
public final String statusAsString() {
return status;
}
/**
* For responses, this returns true if the service returned a value for the RequiresAttributes property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRequiresAttributes() {
return requiresAttributes != null && !(requiresAttributes instanceof SdkAutoConstructList);
}
/**
*
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply custom
* attributes. These are specified as key-value pairs using the Amazon ECS console or the PutAttributes API.
* These attributes are used when determining task placement for tasks hosted on Amazon EC2 instances. For more
* information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRequiresAttributes} method.
*
*
* @return The container instance attributes required by your task. When an Amazon EC2 instance is registered to
* your cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can
* apply custom attributes. These are specified as key-value pairs using the Amazon ECS console or the
* PutAttributes API. These attributes are used when determining task placement for tasks hosted on
* Amazon EC2 instances. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
* This parameter isn't supported for tasks run on Fargate.
*
*/
public final List requiresAttributes() {
return requiresAttributes;
}
/**
* For responses, this returns true if the service returned a value for the PlacementConstraints property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPlacementConstraints() {
return placementConstraints != null && !(placementConstraints instanceof SdkAutoConstructList);
}
/**
*
* An array of placement constraint objects to use for tasks.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPlacementConstraints} method.
*
*
* @return An array of placement constraint objects to use for tasks.
*
* This parameter isn't supported for tasks run on Fargate.
*
*/
public final List placementConstraints() {
return placementConstraints;
}
/**
*
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon
* ECS launch types in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCompatibilities} method.
*
*
* @return The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
*/
public final List compatibilities() {
return CompatibilityListCopier.copyStringToEnum(compatibilities);
}
/**
* For responses, this returns true if the service returned a value for the Compatibilities property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCompatibilities() {
return compatibilities != null && !(compatibilities instanceof SdkAutoConstructList);
}
/**
*
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon
* ECS launch types in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCompatibilities} method.
*
*
* @return The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
*/
public final List compatibilitiesAsStrings() {
return compatibilities;
}
/**
*
* The operating system that your task definitions are running on. A platform family is specified only for tasks
* using the Fargate launch type.
*
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
*
*
* @return The operating system that your task definitions are running on. A platform family is specified only for
* tasks using the Fargate launch type.
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
*/
public final RuntimePlatform runtimePlatform() {
return runtimePlatform;
}
/**
*
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRequiresCompatibilities} method.
*
*
* @return The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
*/
public final List requiresCompatibilities() {
return CompatibilityListCopier.copyStringToEnum(requiresCompatibilities);
}
/**
* For responses, this returns true if the service returned a value for the RequiresCompatibilities property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasRequiresCompatibilities() {
return requiresCompatibilities != null && !(requiresCompatibilities instanceof SdkAutoConstructList);
}
/**
*
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRequiresCompatibilities} method.
*
*
* @return The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
*/
public final List requiresCompatibilitiesAsStrings() {
return requiresCompatibilities;
}
/**
*
* The number of cpu
units used by the task. If you use the EC2 launch type, this field is optional.
* Any value can be used. If you use the Fargate launch type, this field is required. You must use one of the
* following values. The value that you choose determines your range of valid values for the memory
* parameter.
*
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6
* GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @return The number of cpu
units used by the task. If you use the EC2 launch type, this field is
* optional. Any value can be used. If you use the Fargate launch type, this field is required. You must use
* one of the following values. The value that you choose determines your range of valid values for the
* memory
parameter.
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB),
* 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*/
public final String cpu() {
return cpu;
}
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory value is
* specified, the container-level memory value is optional. For more information regarding container-level memory
* and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The value you
* choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values: 2048 (2
* vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values: 4096 (4
* vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @return The amount (in MiB) of memory used by the task.
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory
* value is specified, the container-level memory value is optional. For more information regarding
* container-level memory and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The
* value you choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 2048 (2 vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 4096 (4 vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*/
public final String memory() {
return memory;
}
/**
* For responses, this returns true if the service returned a value for the InferenceAccelerators property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasInferenceAccelerators() {
return inferenceAccelerators != null && !(inferenceAccelerators instanceof SdkAutoConstructList);
}
/**
*
* The Elastic Inference accelerator that's associated with the task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInferenceAccelerators} method.
*
*
* @return The Elastic Inference accelerator that's associated with the task.
*/
public final List inferenceAccelerators() {
return inferenceAccelerators;
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For example,
* monitoring sidecars might need pidMode
to access information about other containers running in the
* same task.
*
*
* If host
is specified, all containers within the tasks that specified the host
PID mode
* on the same container instance share the same process namespace with the host Amazon EC2 instance.
*
*
* If task
is specified, all containers within the specified task share the same process namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace exposure. For
* more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pidMode} will
* return {@link PidMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pidModeAsString}.
*
*
* @return The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For
* example, monitoring sidecars might need pidMode
to access information about other containers
* running in the same task.
*
* If host
is specified, all containers within the tasks that specified the host
* PID mode on the same container instance share the same process namespace with the host Amazon EC2
* instance.
*
*
* If task
is specified, all containers within the specified task share the same process
* namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information,
* see PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace
* exposure. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @see PidMode
*/
public final PidMode pidMode() {
return PidMode.fromValue(pidMode);
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For example,
* monitoring sidecars might need pidMode
to access information about other containers running in the
* same task.
*
*
* If host
is specified, all containers within the tasks that specified the host
PID mode
* on the same container instance share the same process namespace with the host Amazon EC2 instance.
*
*
* If task
is specified, all containers within the specified task share the same process namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace exposure. For
* more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pidMode} will
* return {@link PidMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pidModeAsString}.
*
*
* @return The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For
* example, monitoring sidecars might need pidMode
to access information about other containers
* running in the same task.
*
* If host
is specified, all containers within the tasks that specified the host
* PID mode on the same container instance share the same process namespace with the host Amazon EC2
* instance.
*
*
* If task
is specified, all containers within the specified task share the same process
* namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information,
* see PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace
* exposure. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @see PidMode
*/
public final String pidModeAsString() {
return pidMode;
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipcMode} will
* return {@link IpcMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipcModeAsString}.
*
*
* @return The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within
* the tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
* @see IpcMode
*/
public final IpcMode ipcMode() {
return IpcMode.fromValue(ipcMode);
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipcMode} will
* return {@link IpcMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipcModeAsString}.
*
*
* @return The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within
* the tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
* @see IpcMode
*/
public final String ipcModeAsString() {
return ipcMode;
}
/**
*
* The configuration details for the App Mesh proxy.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least version
* 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container instances are
* launched from the Amazon ECS optimized AMI version 20190301
or later, they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The configuration details for the App Mesh proxy.
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least
* version 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container
* instances are launched from the Amazon ECS optimized AMI version 20190301
or later, they
* contain the required versions of the container agent and ecs-init
. For more information, see
* Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*/
public final ProxyConfiguration proxyConfiguration() {
return proxyConfiguration;
}
/**
*
* The Unix timestamp for the time when the task definition was registered.
*
*
* @return The Unix timestamp for the time when the task definition was registered.
*/
public final Instant registeredAt() {
return registeredAt;
}
/**
*
* The Unix timestamp for the time when the task definition was deregistered.
*
*
* @return The Unix timestamp for the time when the task definition was deregistered.
*/
public final Instant deregisteredAt() {
return deregisteredAt;
}
/**
*
* The principal that registered the task definition.
*
*
* @return The principal that registered the task definition.
*/
public final String registeredBy() {
return registeredBy;
}
/**
*
* The ephemeral storage settings to use for tasks run with the task definition.
*
*
* @return The ephemeral storage settings to use for tasks run with the task definition.
*/
public final EphemeralStorage ephemeralStorage() {
return ephemeralStorage;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(taskDefinitionArn());
hashCode = 31 * hashCode + Objects.hashCode(hasContainerDefinitions() ? containerDefinitions() : null);
hashCode = 31 * hashCode + Objects.hashCode(family());
hashCode = 31 * hashCode + Objects.hashCode(taskRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(executionRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(networkModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(revision());
hashCode = 31 * hashCode + Objects.hashCode(hasVolumes() ? volumes() : null);
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasRequiresAttributes() ? requiresAttributes() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasPlacementConstraints() ? placementConstraints() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCompatibilities() ? compatibilitiesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(runtimePlatform());
hashCode = 31 * hashCode + Objects.hashCode(hasRequiresCompatibilities() ? requiresCompatibilitiesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(cpu());
hashCode = 31 * hashCode + Objects.hashCode(memory());
hashCode = 31 * hashCode + Objects.hashCode(hasInferenceAccelerators() ? inferenceAccelerators() : null);
hashCode = 31 * hashCode + Objects.hashCode(pidModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(ipcModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(proxyConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(registeredAt());
hashCode = 31 * hashCode + Objects.hashCode(deregisteredAt());
hashCode = 31 * hashCode + Objects.hashCode(registeredBy());
hashCode = 31 * hashCode + Objects.hashCode(ephemeralStorage());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TaskDefinition)) {
return false;
}
TaskDefinition other = (TaskDefinition) obj;
return Objects.equals(taskDefinitionArn(), other.taskDefinitionArn())
&& hasContainerDefinitions() == other.hasContainerDefinitions()
&& Objects.equals(containerDefinitions(), other.containerDefinitions())
&& Objects.equals(family(), other.family()) && Objects.equals(taskRoleArn(), other.taskRoleArn())
&& Objects.equals(executionRoleArn(), other.executionRoleArn())
&& Objects.equals(networkModeAsString(), other.networkModeAsString())
&& Objects.equals(revision(), other.revision()) && hasVolumes() == other.hasVolumes()
&& Objects.equals(volumes(), other.volumes()) && Objects.equals(statusAsString(), other.statusAsString())
&& hasRequiresAttributes() == other.hasRequiresAttributes()
&& Objects.equals(requiresAttributes(), other.requiresAttributes())
&& hasPlacementConstraints() == other.hasPlacementConstraints()
&& Objects.equals(placementConstraints(), other.placementConstraints())
&& hasCompatibilities() == other.hasCompatibilities()
&& Objects.equals(compatibilitiesAsStrings(), other.compatibilitiesAsStrings())
&& Objects.equals(runtimePlatform(), other.runtimePlatform())
&& hasRequiresCompatibilities() == other.hasRequiresCompatibilities()
&& Objects.equals(requiresCompatibilitiesAsStrings(), other.requiresCompatibilitiesAsStrings())
&& Objects.equals(cpu(), other.cpu()) && Objects.equals(memory(), other.memory())
&& hasInferenceAccelerators() == other.hasInferenceAccelerators()
&& Objects.equals(inferenceAccelerators(), other.inferenceAccelerators())
&& Objects.equals(pidModeAsString(), other.pidModeAsString())
&& Objects.equals(ipcModeAsString(), other.ipcModeAsString())
&& Objects.equals(proxyConfiguration(), other.proxyConfiguration())
&& Objects.equals(registeredAt(), other.registeredAt())
&& Objects.equals(deregisteredAt(), other.deregisteredAt())
&& Objects.equals(registeredBy(), other.registeredBy())
&& Objects.equals(ephemeralStorage(), other.ephemeralStorage());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TaskDefinition").add("TaskDefinitionArn", taskDefinitionArn())
.add("ContainerDefinitions", hasContainerDefinitions() ? containerDefinitions() : null).add("Family", family())
.add("TaskRoleArn", taskRoleArn()).add("ExecutionRoleArn", executionRoleArn())
.add("NetworkMode", networkModeAsString()).add("Revision", revision())
.add("Volumes", hasVolumes() ? volumes() : null).add("Status", statusAsString())
.add("RequiresAttributes", hasRequiresAttributes() ? requiresAttributes() : null)
.add("PlacementConstraints", hasPlacementConstraints() ? placementConstraints() : null)
.add("Compatibilities", hasCompatibilities() ? compatibilitiesAsStrings() : null)
.add("RuntimePlatform", runtimePlatform())
.add("RequiresCompatibilities", hasRequiresCompatibilities() ? requiresCompatibilitiesAsStrings() : null)
.add("Cpu", cpu()).add("Memory", memory())
.add("InferenceAccelerators", hasInferenceAccelerators() ? inferenceAccelerators() : null)
.add("PidMode", pidModeAsString()).add("IpcMode", ipcModeAsString())
.add("ProxyConfiguration", proxyConfiguration()).add("RegisteredAt", registeredAt())
.add("DeregisteredAt", deregisteredAt()).add("RegisteredBy", registeredBy())
.add("EphemeralStorage", ephemeralStorage()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "taskDefinitionArn":
return Optional.ofNullable(clazz.cast(taskDefinitionArn()));
case "containerDefinitions":
return Optional.ofNullable(clazz.cast(containerDefinitions()));
case "family":
return Optional.ofNullable(clazz.cast(family()));
case "taskRoleArn":
return Optional.ofNullable(clazz.cast(taskRoleArn()));
case "executionRoleArn":
return Optional.ofNullable(clazz.cast(executionRoleArn()));
case "networkMode":
return Optional.ofNullable(clazz.cast(networkModeAsString()));
case "revision":
return Optional.ofNullable(clazz.cast(revision()));
case "volumes":
return Optional.ofNullable(clazz.cast(volumes()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "requiresAttributes":
return Optional.ofNullable(clazz.cast(requiresAttributes()));
case "placementConstraints":
return Optional.ofNullable(clazz.cast(placementConstraints()));
case "compatibilities":
return Optional.ofNullable(clazz.cast(compatibilitiesAsStrings()));
case "runtimePlatform":
return Optional.ofNullable(clazz.cast(runtimePlatform()));
case "requiresCompatibilities":
return Optional.ofNullable(clazz.cast(requiresCompatibilitiesAsStrings()));
case "cpu":
return Optional.ofNullable(clazz.cast(cpu()));
case "memory":
return Optional.ofNullable(clazz.cast(memory()));
case "inferenceAccelerators":
return Optional.ofNullable(clazz.cast(inferenceAccelerators()));
case "pidMode":
return Optional.ofNullable(clazz.cast(pidModeAsString()));
case "ipcMode":
return Optional.ofNullable(clazz.cast(ipcModeAsString()));
case "proxyConfiguration":
return Optional.ofNullable(clazz.cast(proxyConfiguration()));
case "registeredAt":
return Optional.ofNullable(clazz.cast(registeredAt()));
case "deregisteredAt":
return Optional.ofNullable(clazz.cast(deregisteredAt()));
case "registeredBy":
return Optional.ofNullable(clazz.cast(registeredBy()));
case "ephemeralStorage":
return Optional.ofNullable(clazz.cast(ephemeralStorage()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function