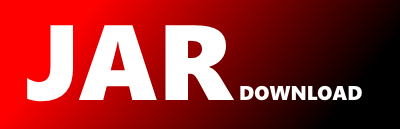
software.amazon.awssdk.services.ecs.model.ServiceManagedEBSVolumeConfiguration Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The configuration for the Amazon EBS volume that Amazon ECS creates and manages on your behalf. These settings are
* used to create each Amazon EBS volume, with one volume created for each task in the service.
*
*
* Many of these parameters map 1:1 with the Amazon EBS CreateVolume
API request parameters.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServiceManagedEBSVolumeConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("encrypted").getter(getter(ServiceManagedEBSVolumeConfiguration::encrypted))
.setter(setter(Builder::encrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encrypted").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("kmsKeyId").getter(getter(ServiceManagedEBSVolumeConfiguration::kmsKeyId))
.setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsKeyId").build()).build();
private static final SdkField VOLUME_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("volumeType").getter(getter(ServiceManagedEBSVolumeConfiguration::volumeType))
.setter(setter(Builder::volumeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("volumeType").build()).build();
private static final SdkField SIZE_IN_GIB_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("sizeInGiB").getter(getter(ServiceManagedEBSVolumeConfiguration::sizeInGiB))
.setter(setter(Builder::sizeInGiB))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sizeInGiB").build()).build();
private static final SdkField SNAPSHOT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("snapshotId").getter(getter(ServiceManagedEBSVolumeConfiguration::snapshotId))
.setter(setter(Builder::snapshotId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("snapshotId").build()).build();
private static final SdkField IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("iops")
.getter(getter(ServiceManagedEBSVolumeConfiguration::iops)).setter(setter(Builder::iops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("iops").build()).build();
private static final SdkField THROUGHPUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("throughput").getter(getter(ServiceManagedEBSVolumeConfiguration::throughput))
.setter(setter(Builder::throughput))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("throughput").build()).build();
private static final SdkField> TAG_SPECIFICATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tagSpecifications")
.getter(getter(ServiceManagedEBSVolumeConfiguration::tagSpecifications))
.setter(setter(Builder::tagSpecifications))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tagSpecifications").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EBSTagSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("roleArn").getter(getter(ServiceManagedEBSVolumeConfiguration::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("roleArn").build()).build();
private static final SdkField FILESYSTEM_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("filesystemType").getter(getter(ServiceManagedEBSVolumeConfiguration::filesystemTypeAsString))
.setter(setter(Builder::filesystemType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("filesystemType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENCRYPTED_FIELD,
KMS_KEY_ID_FIELD, VOLUME_TYPE_FIELD, SIZE_IN_GIB_FIELD, SNAPSHOT_ID_FIELD, IOPS_FIELD, THROUGHPUT_FIELD,
TAG_SPECIFICATIONS_FIELD, ROLE_ARN_FIELD, FILESYSTEM_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final Boolean encrypted;
private final String kmsKeyId;
private final String volumeType;
private final Integer sizeInGiB;
private final String snapshotId;
private final Integer iops;
private final Integer throughput;
private final List tagSpecifications;
private final String roleArn;
private final String filesystemType;
private ServiceManagedEBSVolumeConfiguration(BuilderImpl builder) {
this.encrypted = builder.encrypted;
this.kmsKeyId = builder.kmsKeyId;
this.volumeType = builder.volumeType;
this.sizeInGiB = builder.sizeInGiB;
this.snapshotId = builder.snapshotId;
this.iops = builder.iops;
this.throughput = builder.throughput;
this.tagSpecifications = builder.tagSpecifications;
this.roleArn = builder.roleArn;
this.filesystemType = builder.filesystemType;
}
/**
*
* Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by default.
* This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @return Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by
* default. This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public final Boolean encrypted() {
return encrypted;
}
/**
*
* The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for Amazon
* EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service key is specified,
* the default Amazon Web Services managed key for Amazon EBS volumes is used. This parameter maps 1:1 with the
* KmsKeyId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously. Therefore,
* if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but eventually fails.
*
*
*
* @return The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use
* for Amazon EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service
* key is specified, the default Amazon Web Services managed key for Amazon EBS volumes is used. This
* parameter maps 1:1 with the KmsKeyId
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously.
* Therefore, if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but
* eventually fails.
*
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API in the
* Amazon EC2 API Reference. For more information, see Amazon EBS volume types in
* the Amazon EC2 User Guide.
*
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*
*
* @return The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API
* in the Amazon EC2 API Reference. For more information, see Amazon EBS volume
* types in the Amazon EC2 User Guide.
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*/
public final String volumeType() {
return volumeType;
}
/**
*
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a snapshot
* ID, the snapshot size is used for the volume size by default. You can optionally specify a volume size greater
* than or equal to the snapshot size. This parameter maps 1:1 with the Size
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*
*
* @return The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a
* snapshot ID, the snapshot size is used for the volume size by default. You can optionally specify a
* volume size greater than or equal to the snapshot size. This parameter maps 1:1 with the
* Size
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*/
public final Integer sizeInGiB() {
return sizeInGiB;
}
/**
*
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume size.
* This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @return The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume
* size. This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public final String snapshotId() {
return snapshotId;
}
/**
*
* The number of I/O operations per second (IOPS). For gp3
, io1
, and io2
* volumes, this represents the number of IOPS that are provisioned for the volume. For gp2
volumes,
* this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits
* for bursting.
*
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
,
* sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @return The number of I/O operations per second (IOPS). For gp3
, io1
, and
* io2
volumes, this represents the number of IOPS that are provisioned for the volume. For
* gp2
volumes, this represents the baseline performance of the volume and the rate at which
* the volume accumulates I/O credits for bursting.
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
, sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public final Integer iops() {
return iops;
}
/**
*
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps 1:1 with
* the Throughput
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* This parameter is only supported for the gp3
volume type.
*
*
*
* @return The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps
* 1:1 with the Throughput
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* This parameter is only supported for the gp3
volume type.
*
*/
public final Integer throughput() {
return throughput;
}
/**
* For responses, this returns true if the service returned a value for the TagSpecifications property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTagSpecifications() {
return tagSpecifications != null && !(tagSpecifications instanceof SdkAutoConstructList);
}
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1 with
* the TagSpecifications.N
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTagSpecifications} method.
*
*
* @return The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps
* 1:1 with the TagSpecifications.N
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public final List tagSpecifications() {
return tagSpecifications;
}
/**
*
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role that is
* used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information, see Amazon ECS
* infrastructure IAM role in the Amazon ECS Developer Guide.
*
*
* @return The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role
* that is used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information,
* see Amazon
* ECS infrastructure IAM role in the Amazon ECS Developer Guide.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type mismatch,
* the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #filesystemType}
* will return {@link TaskFilesystemType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #filesystemTypeAsString}.
*
*
* @return The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type
* mismatch, the task will fail to start.
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If
* no value is specified, the xfs
filesystem type is used by default.
* @see TaskFilesystemType
*/
public final TaskFilesystemType filesystemType() {
return TaskFilesystemType.fromValue(filesystemType);
}
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type mismatch,
* the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #filesystemType}
* will return {@link TaskFilesystemType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #filesystemTypeAsString}.
*
*
* @return The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type
* mismatch, the task will fail to start.
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If
* no value is specified, the xfs
filesystem type is used by default.
* @see TaskFilesystemType
*/
public final String filesystemTypeAsString() {
return filesystemType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(encrypted());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(volumeType());
hashCode = 31 * hashCode + Objects.hashCode(sizeInGiB());
hashCode = 31 * hashCode + Objects.hashCode(snapshotId());
hashCode = 31 * hashCode + Objects.hashCode(iops());
hashCode = 31 * hashCode + Objects.hashCode(throughput());
hashCode = 31 * hashCode + Objects.hashCode(hasTagSpecifications() ? tagSpecifications() : null);
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(filesystemTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceManagedEBSVolumeConfiguration)) {
return false;
}
ServiceManagedEBSVolumeConfiguration other = (ServiceManagedEBSVolumeConfiguration) obj;
return Objects.equals(encrypted(), other.encrypted()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(volumeType(), other.volumeType()) && Objects.equals(sizeInGiB(), other.sizeInGiB())
&& Objects.equals(snapshotId(), other.snapshotId()) && Objects.equals(iops(), other.iops())
&& Objects.equals(throughput(), other.throughput()) && hasTagSpecifications() == other.hasTagSpecifications()
&& Objects.equals(tagSpecifications(), other.tagSpecifications()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(filesystemTypeAsString(), other.filesystemTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServiceManagedEBSVolumeConfiguration").add("Encrypted", encrypted()).add("KmsKeyId", kmsKeyId())
.add("VolumeType", volumeType()).add("SizeInGiB", sizeInGiB()).add("SnapshotId", snapshotId())
.add("Iops", iops()).add("Throughput", throughput())
.add("TagSpecifications", hasTagSpecifications() ? tagSpecifications() : null).add("RoleArn", roleArn())
.add("FilesystemType", filesystemTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "encrypted":
return Optional.ofNullable(clazz.cast(encrypted()));
case "kmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "volumeType":
return Optional.ofNullable(clazz.cast(volumeType()));
case "sizeInGiB":
return Optional.ofNullable(clazz.cast(sizeInGiB()));
case "snapshotId":
return Optional.ofNullable(clazz.cast(snapshotId()));
case "iops":
return Optional.ofNullable(clazz.cast(iops()));
case "throughput":
return Optional.ofNullable(clazz.cast(throughput()));
case "tagSpecifications":
return Optional.ofNullable(clazz.cast(tagSpecifications()));
case "roleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "filesystemType":
return Optional.ofNullable(clazz.cast(filesystemTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously.
* Therefore, if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but
* eventually fails.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder kmsKeyId(String kmsKeyId);
/**
*
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API in
* the Amazon EC2 API Reference. For more information, see Amazon EBS volume types
* in the Amazon EC2 User Guide.
*
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*
*
* @param volumeType
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume
* API in the Amazon EC2 API Reference. For more information, see Amazon EBS volume
* types in the Amazon EC2 User Guide.
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder volumeType(String volumeType);
/**
*
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a
* snapshot ID, the snapshot size is used for the volume size by default. You can optionally specify a volume
* size greater than or equal to the snapshot size. This parameter maps 1:1 with the Size
parameter
* of the CreateVolume
* API in the Amazon EC2 API Reference.
*
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*
*
* @param sizeInGiB
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify
* a snapshot ID, the snapshot size is used for the volume size by default. You can optionally specify a
* volume size greater than or equal to the snapshot size. This parameter maps 1:1 with the
* Size
parameter of the CreateVolume
* API in the Amazon EC2 API Reference.
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sizeInGiB(Integer sizeInGiB);
/**
*
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume
* size. This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API in
* the Amazon EC2 API Reference.
*
*
* @param snapshotId
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a
* volume size. This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume
* API in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder snapshotId(String snapshotId);
/**
*
* The number of I/O operations per second (IOPS). For gp3
, io1
, and io2
* volumes, this represents the number of IOPS that are provisioned for the volume. For gp2
* volumes, this represents the baseline performance of the volume and the rate at which the volume accumulates
* I/O credits for bursting.
*
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
,
* sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API in
* the Amazon EC2 API Reference.
*
*
* @param iops
* The number of I/O operations per second (IOPS). For gp3
, io1
, and
* io2
volumes, this represents the number of IOPS that are provisioned for the volume. For
* gp2
volumes, this represents the baseline performance of the volume and the rate at which
* the volume accumulates I/O credits for bursting.
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for
* st1
, sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume
* API in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder iops(Integer iops);
/**
*
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps 1:1
* with the Throughput
parameter of the CreateVolume API in
* the Amazon EC2 API Reference.
*
*
*
* This parameter is only supported for the gp3
volume type.
*
*
*
* @param throughput
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps
* 1:1 with the Throughput
parameter of the CreateVolume
* API in the Amazon EC2 API Reference.
*
* This parameter is only supported for the gp3
volume type.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder throughput(Integer throughput);
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1
* with the TagSpecifications.N
parameter of the CreateVolume API in
* the Amazon EC2 API Reference.
*
*
* @param tagSpecifications
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter
* maps 1:1 with the TagSpecifications.N
parameter of the CreateVolume
* API in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tagSpecifications(Collection tagSpecifications);
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1
* with the TagSpecifications.N
parameter of the CreateVolume API in
* the Amazon EC2 API Reference.
*
*
* @param tagSpecifications
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter
* maps 1:1 with the TagSpecifications.N
parameter of the CreateVolume
* API in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tagSpecifications(EBSTagSpecification... tagSpecifications);
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1
* with the TagSpecifications.N
parameter of the CreateVolume API in
* the Amazon EC2 API Reference.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ecs.model.EBSTagSpecification.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.ecs.model.EBSTagSpecification#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ecs.model.EBSTagSpecification.Builder#build()} is called immediately
* and its result is passed to {@link #tagSpecifications(List)}.
*
* @param tagSpecifications
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ecs.model.EBSTagSpecification.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tagSpecifications(java.util.Collection)
*/
Builder tagSpecifications(Consumer... tagSpecifications);
/**
*
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role that is
* used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information, see
* Amazon ECS
* infrastructure IAM role in the Amazon ECS Developer Guide.
*
*
* @param roleArn
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role
* that is used to manage your Amazon Web Services infrastructure. We recommend using the Amazon
* ECS-managed AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For
* more information, see Amazon
* ECS infrastructure IAM role in the Amazon ECS Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleArn(String roleArn);
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type
* mismatch, the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*
* @param filesystemType
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the
* same filesystem type that the volume was using when the snapshot was created. If there is a filesystem
* type mismatch, the task will fail to start.
*
* The available filesystem types are
ext3
, ext4
, and xfs
.
* If no value is specified, the xfs
filesystem type is used by default.
* @see TaskFilesystemType
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskFilesystemType
*/
Builder filesystemType(String filesystemType);
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type
* mismatch, the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*
* @param filesystemType
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the
* same filesystem type that the volume was using when the snapshot was created. If there is a filesystem
* type mismatch, the task will fail to start.
*
* The available filesystem types are
ext3
, ext4
, and xfs
.
* If no value is specified, the xfs
filesystem type is used by default.
* @see TaskFilesystemType
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskFilesystemType
*/
Builder filesystemType(TaskFilesystemType filesystemType);
}
static final class BuilderImpl implements Builder {
private Boolean encrypted;
private String kmsKeyId;
private String volumeType;
private Integer sizeInGiB;
private String snapshotId;
private Integer iops;
private Integer throughput;
private List tagSpecifications = DefaultSdkAutoConstructList.getInstance();
private String roleArn;
private String filesystemType;
private BuilderImpl() {
}
private BuilderImpl(ServiceManagedEBSVolumeConfiguration model) {
encrypted(model.encrypted);
kmsKeyId(model.kmsKeyId);
volumeType(model.volumeType);
sizeInGiB(model.sizeInGiB);
snapshotId(model.snapshotId);
iops(model.iops);
throughput(model.throughput);
tagSpecifications(model.tagSpecifications);
roleArn(model.roleArn);
filesystemType(model.filesystemType);
}
public final Boolean getEncrypted() {
return encrypted;
}
public final void setEncrypted(Boolean encrypted) {
this.encrypted = encrypted;
}
@Override
public final Builder encrypted(Boolean encrypted) {
this.encrypted = encrypted;
return this;
}
public final String getKmsKeyId() {
return kmsKeyId;
}
public final void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
@Override
public final Builder kmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
return this;
}
public final String getVolumeType() {
return volumeType;
}
public final void setVolumeType(String volumeType) {
this.volumeType = volumeType;
}
@Override
public final Builder volumeType(String volumeType) {
this.volumeType = volumeType;
return this;
}
public final Integer getSizeInGiB() {
return sizeInGiB;
}
public final void setSizeInGiB(Integer sizeInGiB) {
this.sizeInGiB = sizeInGiB;
}
@Override
public final Builder sizeInGiB(Integer sizeInGiB) {
this.sizeInGiB = sizeInGiB;
return this;
}
public final String getSnapshotId() {
return snapshotId;
}
public final void setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
}
@Override
public final Builder snapshotId(String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
public final Integer getIops() {
return iops;
}
public final void setIops(Integer iops) {
this.iops = iops;
}
@Override
public final Builder iops(Integer iops) {
this.iops = iops;
return this;
}
public final Integer getThroughput() {
return throughput;
}
public final void setThroughput(Integer throughput) {
this.throughput = throughput;
}
@Override
public final Builder throughput(Integer throughput) {
this.throughput = throughput;
return this;
}
public final List getTagSpecifications() {
List result = EBSTagSpecificationsCopier.copyToBuilder(this.tagSpecifications);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTagSpecifications(Collection tagSpecifications) {
this.tagSpecifications = EBSTagSpecificationsCopier.copyFromBuilder(tagSpecifications);
}
@Override
public final Builder tagSpecifications(Collection tagSpecifications) {
this.tagSpecifications = EBSTagSpecificationsCopier.copy(tagSpecifications);
return this;
}
@Override
@SafeVarargs
public final Builder tagSpecifications(EBSTagSpecification... tagSpecifications) {
tagSpecifications(Arrays.asList(tagSpecifications));
return this;
}
@Override
@SafeVarargs
public final Builder tagSpecifications(Consumer... tagSpecifications) {
tagSpecifications(Stream.of(tagSpecifications).map(c -> EBSTagSpecification.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final String getRoleArn() {
return roleArn;
}
public final void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
@Override
public final Builder roleArn(String roleArn) {
this.roleArn = roleArn;
return this;
}
public final String getFilesystemType() {
return filesystemType;
}
public final void setFilesystemType(String filesystemType) {
this.filesystemType = filesystemType;
}
@Override
public final Builder filesystemType(String filesystemType) {
this.filesystemType = filesystemType;
return this;
}
@Override
public final Builder filesystemType(TaskFilesystemType filesystemType) {
this.filesystemType(filesystemType == null ? null : filesystemType.toString());
return this;
}
@Override
public ServiceManagedEBSVolumeConfiguration build() {
return new ServiceManagedEBSVolumeConfiguration(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}