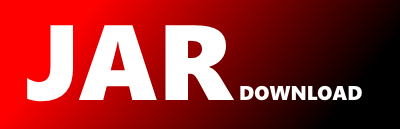
software.amazon.awssdk.services.ecs.model.Cluster Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A regional grouping of one or more container instances where you can run task requests. Each account receives a
* default cluster the first time you use the Amazon ECS service, but you may also create other clusters. Clusters may
* contain more than one instance type simultaneously.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Cluster implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clusterArn").getter(getter(Cluster::clusterArn)).setter(setter(Builder::clusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clusterArn").build()).build();
private static final SdkField CLUSTER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clusterName").getter(getter(Cluster::clusterName)).setter(setter(Builder::clusterName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clusterName").build()).build();
private static final SdkField CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("configuration")
.getter(getter(Cluster::configuration)).setter(setter(Builder::configuration))
.constructor(ClusterConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("configuration").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(Cluster::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField REGISTERED_CONTAINER_INSTANCES_COUNT_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("registeredContainerInstancesCount")
.getter(getter(Cluster::registeredContainerInstancesCount))
.setter(setter(Builder::registeredContainerInstancesCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registeredContainerInstancesCount")
.build()).build();
private static final SdkField RUNNING_TASKS_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("runningTasksCount").getter(getter(Cluster::runningTasksCount))
.setter(setter(Builder::runningTasksCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runningTasksCount").build()).build();
private static final SdkField PENDING_TASKS_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("pendingTasksCount").getter(getter(Cluster::pendingTasksCount))
.setter(setter(Builder::pendingTasksCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pendingTasksCount").build()).build();
private static final SdkField ACTIVE_SERVICES_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("activeServicesCount").getter(getter(Cluster::activeServicesCount))
.setter(setter(Builder::activeServicesCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("activeServicesCount").build())
.build();
private static final SdkField> STATISTICS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("statistics")
.getter(getter(Cluster::statistics))
.setter(setter(Builder::statistics))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("statistics").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(KeyValuePair::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(Cluster::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SETTINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("settings")
.getter(getter(Cluster::settings))
.setter(setter(Builder::settings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("settings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ClusterSetting::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CAPACITY_PROVIDERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviders")
.getter(getter(Cluster::capacityProviders))
.setter(setter(Builder::capacityProviders))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviders").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DEFAULT_CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("defaultCapacityProviderStrategy")
.getter(getter(Cluster::defaultCapacityProviderStrategy))
.setter(setter(Builder::defaultCapacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("defaultCapacityProviderStrategy")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ATTACHMENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("attachments")
.getter(getter(Cluster::attachments))
.setter(setter(Builder::attachments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attachments").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Attachment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ATTACHMENTS_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("attachmentsStatus").getter(getter(Cluster::attachmentsStatus))
.setter(setter(Builder::attachmentsStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attachmentsStatus").build()).build();
private static final SdkField SERVICE_CONNECT_DEFAULTS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("serviceConnectDefaults")
.getter(getter(Cluster::serviceConnectDefaults)).setter(setter(Builder::serviceConnectDefaults))
.constructor(ClusterServiceConnectDefaults::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceConnectDefaults").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_ARN_FIELD,
CLUSTER_NAME_FIELD, CONFIGURATION_FIELD, STATUS_FIELD, REGISTERED_CONTAINER_INSTANCES_COUNT_FIELD,
RUNNING_TASKS_COUNT_FIELD, PENDING_TASKS_COUNT_FIELD, ACTIVE_SERVICES_COUNT_FIELD, STATISTICS_FIELD, TAGS_FIELD,
SETTINGS_FIELD, CAPACITY_PROVIDERS_FIELD, DEFAULT_CAPACITY_PROVIDER_STRATEGY_FIELD, ATTACHMENTS_FIELD,
ATTACHMENTS_STATUS_FIELD, SERVICE_CONNECT_DEFAULTS_FIELD));
private static final long serialVersionUID = 1L;
private final String clusterArn;
private final String clusterName;
private final ClusterConfiguration configuration;
private final String status;
private final Integer registeredContainerInstancesCount;
private final Integer runningTasksCount;
private final Integer pendingTasksCount;
private final Integer activeServicesCount;
private final List statistics;
private final List tags;
private final List settings;
private final List capacityProviders;
private final List defaultCapacityProviderStrategy;
private final List attachments;
private final String attachmentsStatus;
private final ClusterServiceConnectDefaults serviceConnectDefaults;
private Cluster(BuilderImpl builder) {
this.clusterArn = builder.clusterArn;
this.clusterName = builder.clusterName;
this.configuration = builder.configuration;
this.status = builder.status;
this.registeredContainerInstancesCount = builder.registeredContainerInstancesCount;
this.runningTasksCount = builder.runningTasksCount;
this.pendingTasksCount = builder.pendingTasksCount;
this.activeServicesCount = builder.activeServicesCount;
this.statistics = builder.statistics;
this.tags = builder.tags;
this.settings = builder.settings;
this.capacityProviders = builder.capacityProviders;
this.defaultCapacityProviderStrategy = builder.defaultCapacityProviderStrategy;
this.attachments = builder.attachments;
this.attachmentsStatus = builder.attachmentsStatus;
this.serviceConnectDefaults = builder.serviceConnectDefaults;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the cluster. For more information about the ARN format, see Amazon
* Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) that identifies the cluster. For more information about the ARN format,
* see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*/
public final String clusterArn() {
return clusterArn;
}
/**
*
* A user-generated string that you use to identify your cluster.
*
*
* @return A user-generated string that you use to identify your cluster.
*/
public final String clusterName() {
return clusterName;
}
/**
*
* The execute command configuration for the cluster.
*
*
* @return The execute command configuration for the cluster.
*/
public final ClusterConfiguration configuration() {
return configuration;
}
/**
*
* The status of the cluster. The following are the possible states that are returned.
*
*
* - ACTIVE
* -
*
* The cluster is ready to accept tasks and if applicable you can register container instances with the cluster.
*
*
* - PROVISIONING
* -
*
* The cluster has capacity providers that are associated with it and the resources needed for the capacity provider
* are being created.
*
*
* - DEPROVISIONING
* -
*
* The cluster has capacity providers that are associated with it and the resources needed for the capacity provider
* are being deleted.
*
*
* - FAILED
* -
*
* The cluster has capacity providers that are associated with it and the resources needed for the capacity provider
* have failed to create.
*
*
* - INACTIVE
* -
*
* The cluster has been deleted. Clusters with an INACTIVE
status may remain discoverable in your
* account for a period of time. However, this behavior is subject to change in the future. We don't recommend that
* you rely on INACTIVE
clusters persisting.
*
*
*
*
* @return The status of the cluster. The following are the possible states that are returned.
*
* - ACTIVE
* -
*
* The cluster is ready to accept tasks and if applicable you can register container instances with the
* cluster.
*
*
* - PROVISIONING
* -
*
* The cluster has capacity providers that are associated with it and the resources needed for the capacity
* provider are being created.
*
*
* - DEPROVISIONING
* -
*
* The cluster has capacity providers that are associated with it and the resources needed for the capacity
* provider are being deleted.
*
*
* - FAILED
* -
*
* The cluster has capacity providers that are associated with it and the resources needed for the capacity
* provider have failed to create.
*
*
* - INACTIVE
* -
*
* The cluster has been deleted. Clusters with an INACTIVE
status may remain discoverable in
* your account for a period of time. However, this behavior is subject to change in the future. We don't
* recommend that you rely on INACTIVE
clusters persisting.
*
*
*/
public final String status() {
return status;
}
/**
*
* The number of container instances registered into the cluster. This includes container instances in both
* ACTIVE
and DRAINING
status.
*
*
* @return The number of container instances registered into the cluster. This includes container instances in both
* ACTIVE
and DRAINING
status.
*/
public final Integer registeredContainerInstancesCount() {
return registeredContainerInstancesCount;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @return The number of tasks in the cluster that are in the RUNNING
state.
*/
public final Integer runningTasksCount() {
return runningTasksCount;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @return The number of tasks in the cluster that are in the PENDING
state.
*/
public final Integer pendingTasksCount() {
return pendingTasksCount;
}
/**
*
* The number of services that are running on the cluster in an ACTIVE
state. You can view these
* services with ListServices.
*
*
* @return The number of services that are running on the cluster in an ACTIVE
state. You can view
* these services with ListServices.
*/
public final Integer activeServicesCount() {
return activeServicesCount;
}
/**
* For responses, this returns true if the service returned a value for the Statistics property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasStatistics() {
return statistics != null && !(statistics instanceof SdkAutoConstructList);
}
/**
*
* Additional information about your clusters that are separated by launch type. They include the following:
*
*
* -
*
* runningEC2TasksCount
*
*
* -
*
* RunningFargateTasksCount
*
*
* -
*
* pendingEC2TasksCount
*
*
* -
*
* pendingFargateTasksCount
*
*
* -
*
* activeEC2ServiceCount
*
*
* -
*
* activeFargateServiceCount
*
*
* -
*
* drainingEC2ServiceCount
*
*
* -
*
* drainingFargateServiceCount
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStatistics} method.
*
*
* @return Additional information about your clusters that are separated by launch type. They include the
* following:
*
* -
*
* runningEC2TasksCount
*
*
* -
*
* RunningFargateTasksCount
*
*
* -
*
* pendingEC2TasksCount
*
*
* -
*
* pendingFargateTasksCount
*
*
* -
*
* activeEC2ServiceCount
*
*
* -
*
* activeFargateServiceCount
*
*
* -
*
* drainingEC2ServiceCount
*
*
* -
*
* drainingFargateServiceCount
*
*
*/
public final List statistics() {
return statistics;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the cluster to help you categorize and organize them. Each tag consists of a key
* and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The metadata that you apply to the cluster to help you categorize and organize them. Each tag consists of
* a key and an optional value. You define both.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete
* tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource
* limit.
*
*
*/
public final List tags() {
return tags;
}
/**
* For responses, this returns true if the service returned a value for the Settings property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasSettings() {
return settings != null && !(settings instanceof SdkAutoConstructList);
}
/**
*
* The settings for the cluster. This parameter indicates whether CloudWatch Container Insights is on or off for a
* cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSettings} method.
*
*
* @return The settings for the cluster. This parameter indicates whether CloudWatch Container Insights is on or off
* for a cluster.
*/
public final List settings() {
return settings;
}
/**
* For responses, this returns true if the service returned a value for the CapacityProviders property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCapacityProviders() {
return capacityProviders != null && !(capacityProviders instanceof SdkAutoConstructList);
}
/**
*
* The capacity providers associated with the cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapacityProviders} method.
*
*
* @return The capacity providers associated with the cluster.
*/
public final List capacityProviders() {
return capacityProviders;
}
/**
* For responses, this returns true if the service returned a value for the DefaultCapacityProviderStrategy
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasDefaultCapacityProviderStrategy() {
return defaultCapacityProviderStrategy != null && !(defaultCapacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The default capacity provider strategy for the cluster. When services or tasks are run in the cluster with no
* launch type or capacity provider strategy specified, the default capacity provider strategy is used.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDefaultCapacityProviderStrategy}
* method.
*
*
* @return The default capacity provider strategy for the cluster. When services or tasks are run in the cluster
* with no launch type or capacity provider strategy specified, the default capacity provider strategy is
* used.
*/
public final List defaultCapacityProviderStrategy() {
return defaultCapacityProviderStrategy;
}
/**
* For responses, this returns true if the service returned a value for the Attachments property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAttachments() {
return attachments != null && !(attachments instanceof SdkAutoConstructList);
}
/**
*
* The resources attached to a cluster. When using a capacity provider with a cluster, the capacity provider and
* associated resources are returned as cluster attachments.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttachments} method.
*
*
* @return The resources attached to a cluster. When using a capacity provider with a cluster, the capacity provider
* and associated resources are returned as cluster attachments.
*/
public final List attachments() {
return attachments;
}
/**
*
* The status of the capacity providers associated with the cluster. The following are the states that are returned.
*
*
* - UPDATE_IN_PROGRESS
* -
*
* The available capacity providers for the cluster are updating.
*
*
* - UPDATE_COMPLETE
* -
*
* The capacity providers have successfully updated.
*
*
* - UPDATE_FAILED
* -
*
* The capacity provider updates failed.
*
*
*
*
* @return The status of the capacity providers associated with the cluster. The following are the states that are
* returned.
*
* - UPDATE_IN_PROGRESS
* -
*
* The available capacity providers for the cluster are updating.
*
*
* - UPDATE_COMPLETE
* -
*
* The capacity providers have successfully updated.
*
*
* - UPDATE_FAILED
* -
*
* The capacity provider updates failed.
*
*
*/
public final String attachmentsStatus() {
return attachmentsStatus;
}
/**
*
* Use this parameter to set a default Service Connect namespace. After you set a default Service Connect namespace,
* any new services with Service Connect turned on that are created in the cluster are added as client services in
* the namespace. This setting only applies to new services that set the enabled
parameter to
* true
in the ServiceConnectConfiguration
. You can set the namespace of each service
* individually in the ServiceConnectConfiguration
to override this default parameter.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return Use this parameter to set a default Service Connect namespace. After you set a default Service Connect
* namespace, any new services with Service Connect turned on that are created in the cluster are added as
* client services in the namespace. This setting only applies to new services that set the
* enabled
parameter to true
in the ServiceConnectConfiguration
. You
* can set the namespace of each service individually in the ServiceConnectConfiguration
to
* override this default parameter.
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
*/
public final ClusterServiceConnectDefaults serviceConnectDefaults() {
return serviceConnectDefaults;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(clusterArn());
hashCode = 31 * hashCode + Objects.hashCode(clusterName());
hashCode = 31 * hashCode + Objects.hashCode(configuration());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(registeredContainerInstancesCount());
hashCode = 31 * hashCode + Objects.hashCode(runningTasksCount());
hashCode = 31 * hashCode + Objects.hashCode(pendingTasksCount());
hashCode = 31 * hashCode + Objects.hashCode(activeServicesCount());
hashCode = 31 * hashCode + Objects.hashCode(hasStatistics() ? statistics() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSettings() ? settings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviders() ? capacityProviders() : null);
hashCode = 31 * hashCode
+ Objects.hashCode(hasDefaultCapacityProviderStrategy() ? defaultCapacityProviderStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasAttachments() ? attachments() : null);
hashCode = 31 * hashCode + Objects.hashCode(attachmentsStatus());
hashCode = 31 * hashCode + Objects.hashCode(serviceConnectDefaults());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Cluster)) {
return false;
}
Cluster other = (Cluster) obj;
return Objects.equals(clusterArn(), other.clusterArn()) && Objects.equals(clusterName(), other.clusterName())
&& Objects.equals(configuration(), other.configuration()) && Objects.equals(status(), other.status())
&& Objects.equals(registeredContainerInstancesCount(), other.registeredContainerInstancesCount())
&& Objects.equals(runningTasksCount(), other.runningTasksCount())
&& Objects.equals(pendingTasksCount(), other.pendingTasksCount())
&& Objects.equals(activeServicesCount(), other.activeServicesCount()) && hasStatistics() == other.hasStatistics()
&& Objects.equals(statistics(), other.statistics()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && hasSettings() == other.hasSettings()
&& Objects.equals(settings(), other.settings()) && hasCapacityProviders() == other.hasCapacityProviders()
&& Objects.equals(capacityProviders(), other.capacityProviders())
&& hasDefaultCapacityProviderStrategy() == other.hasDefaultCapacityProviderStrategy()
&& Objects.equals(defaultCapacityProviderStrategy(), other.defaultCapacityProviderStrategy())
&& hasAttachments() == other.hasAttachments() && Objects.equals(attachments(), other.attachments())
&& Objects.equals(attachmentsStatus(), other.attachmentsStatus())
&& Objects.equals(serviceConnectDefaults(), other.serviceConnectDefaults());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("Cluster")
.add("ClusterArn", clusterArn())
.add("ClusterName", clusterName())
.add("Configuration", configuration())
.add("Status", status())
.add("RegisteredContainerInstancesCount", registeredContainerInstancesCount())
.add("RunningTasksCount", runningTasksCount())
.add("PendingTasksCount", pendingTasksCount())
.add("ActiveServicesCount", activeServicesCount())
.add("Statistics", hasStatistics() ? statistics() : null)
.add("Tags", hasTags() ? tags() : null)
.add("Settings", hasSettings() ? settings() : null)
.add("CapacityProviders", hasCapacityProviders() ? capacityProviders() : null)
.add("DefaultCapacityProviderStrategy",
hasDefaultCapacityProviderStrategy() ? defaultCapacityProviderStrategy() : null)
.add("Attachments", hasAttachments() ? attachments() : null).add("AttachmentsStatus", attachmentsStatus())
.add("ServiceConnectDefaults", serviceConnectDefaults()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "clusterArn":
return Optional.ofNullable(clazz.cast(clusterArn()));
case "clusterName":
return Optional.ofNullable(clazz.cast(clusterName()));
case "configuration":
return Optional.ofNullable(clazz.cast(configuration()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "registeredContainerInstancesCount":
return Optional.ofNullable(clazz.cast(registeredContainerInstancesCount()));
case "runningTasksCount":
return Optional.ofNullable(clazz.cast(runningTasksCount()));
case "pendingTasksCount":
return Optional.ofNullable(clazz.cast(pendingTasksCount()));
case "activeServicesCount":
return Optional.ofNullable(clazz.cast(activeServicesCount()));
case "statistics":
return Optional.ofNullable(clazz.cast(statistics()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "settings":
return Optional.ofNullable(clazz.cast(settings()));
case "capacityProviders":
return Optional.ofNullable(clazz.cast(capacityProviders()));
case "defaultCapacityProviderStrategy":
return Optional.ofNullable(clazz.cast(defaultCapacityProviderStrategy()));
case "attachments":
return Optional.ofNullable(clazz.cast(attachments()));
case "attachmentsStatus":
return Optional.ofNullable(clazz.cast(attachmentsStatus()));
case "serviceConnectDefaults":
return Optional.ofNullable(clazz.cast(serviceConnectDefaults()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* runningEC2TasksCount
*
*
* -
*
* RunningFargateTasksCount
*
*
* -
*
* pendingEC2TasksCount
*
*
* -
*
* pendingFargateTasksCount
*
*
* -
*
* activeEC2ServiceCount
*
*
* -
*
* activeFargateServiceCount
*
*
* -
*
* drainingEC2ServiceCount
*
*
* -
*
* drainingFargateServiceCount
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statistics(Collection statistics);
/**
*
* Additional information about your clusters that are separated by launch type. They include the following:
*
*
* -
*
* runningEC2TasksCount
*
*
* -
*
* RunningFargateTasksCount
*
*
* -
*
* pendingEC2TasksCount
*
*
* -
*
* pendingFargateTasksCount
*
*
* -
*
* activeEC2ServiceCount
*
*
* -
*
* activeFargateServiceCount
*
*
* -
*
* drainingEC2ServiceCount
*
*
* -
*
* drainingFargateServiceCount
*
*
*
*
* @param statistics
* Additional information about your clusters that are separated by launch type. They include the
* following:
*
* -
*
* runningEC2TasksCount
*
*
* -
*
* RunningFargateTasksCount
*
*
* -
*
* pendingEC2TasksCount
*
*
* -
*
* pendingFargateTasksCount
*
*
* -
*
* activeEC2ServiceCount
*
*
* -
*
* activeFargateServiceCount
*
*
* -
*
* drainingEC2ServiceCount
*
*
* -
*
* drainingFargateServiceCount
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statistics(KeyValuePair... statistics);
/**
*
* Additional information about your clusters that are separated by launch type. They include the following:
*
*
* -
*
* runningEC2TasksCount
*
*
* -
*
* RunningFargateTasksCount
*
*
* -
*
* pendingEC2TasksCount
*
*
* -
*
* pendingFargateTasksCount
*
*
* -
*
* activeEC2ServiceCount
*
*
* -
*
* activeFargateServiceCount
*
*
* -
*
* drainingEC2ServiceCount
*
*
* -
*
* drainingFargateServiceCount
*
*
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ecs.model.KeyValuePair.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.ecs.model.KeyValuePair#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ecs.model.KeyValuePair.Builder#build()} is called immediately and its
* result is passed to {@link #statistics(List)}.
*
* @param statistics
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ecs.model.KeyValuePair.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #statistics(java.util.Collection)
*/
Builder statistics(Consumer... statistics);
/**
*
* The metadata that you apply to the cluster to help you categorize and organize them. Each tag consists of a
* key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys
* or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the cluster to help you categorize and organize them. Each tag consists
* of a key and an optional value. You define both.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services
* may have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and
* spaces representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or
* delete tag keys or values with this prefix. Tags with this prefix do not count against your tags per
* resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* The metadata that you apply to the cluster to help you categorize and organize them. Each tag consists of a
* key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys
* or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the cluster to help you categorize and organize them. Each tag consists
* of a key and an optional value. You define both.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services
* may have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and
* spaces representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or
* delete tag keys or values with this prefix. Tags with this prefix do not count against your tags per
* resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* The metadata that you apply to the cluster to help you categorize and organize them. Each tag consists of a
* key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys
* or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ecs.model.Tag.Builder} avoiding the need to create one manually via
* {@link software.amazon.awssdk.services.ecs.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes, {@link software.amazon.awssdk.services.ecs.model.Tag.Builder#build()} is
* called immediately and its result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on {@link software.amazon.awssdk.services.ecs.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
/**
*
* The settings for the cluster. This parameter indicates whether CloudWatch Container Insights is on or off for
* a cluster.
*
*
* @param settings
* The settings for the cluster. This parameter indicates whether CloudWatch Container Insights is on or
* off for a cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder settings(Collection settings);
/**
*
* The settings for the cluster. This parameter indicates whether CloudWatch Container Insights is on or off for
* a cluster.
*
*
* @param settings
* The settings for the cluster. This parameter indicates whether CloudWatch Container Insights is on or
* off for a cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder settings(ClusterSetting... settings);
/**
*
* The settings for the cluster. This parameter indicates whether CloudWatch Container Insights is on or off for
* a cluster.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ecs.model.ClusterSetting.Builder} avoiding the need to create one
* manually via {@link software.amazon.awssdk.services.ecs.model.ClusterSetting#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ecs.model.ClusterSetting.Builder#build()} is called immediately and
* its result is passed to {@link #settings(List)}.
*
* @param settings
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ecs.model.ClusterSetting.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #settings(java.util.Collection)
*/
Builder settings(Consumer... settings);
/**
*
* The capacity providers associated with the cluster.
*
*
* @param capacityProviders
* The capacity providers associated with the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capacityProviders(Collection capacityProviders);
/**
*
* The capacity providers associated with the cluster.
*
*
* @param capacityProviders
* The capacity providers associated with the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capacityProviders(String... capacityProviders);
/**
*
* The default capacity provider strategy for the cluster. When services or tasks are run in the cluster with no
* launch type or capacity provider strategy specified, the default capacity provider strategy is used.
*
*
* @param defaultCapacityProviderStrategy
* The default capacity provider strategy for the cluster. When services or tasks are run in the cluster
* with no launch type or capacity provider strategy specified, the default capacity provider strategy is
* used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder defaultCapacityProviderStrategy(Collection defaultCapacityProviderStrategy);
/**
*
* The default capacity provider strategy for the cluster. When services or tasks are run in the cluster with no
* launch type or capacity provider strategy specified, the default capacity provider strategy is used.
*
*
* @param defaultCapacityProviderStrategy
* The default capacity provider strategy for the cluster. When services or tasks are run in the cluster
* with no launch type or capacity provider strategy specified, the default capacity provider strategy is
* used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder defaultCapacityProviderStrategy(CapacityProviderStrategyItem... defaultCapacityProviderStrategy);
/**
*
* The default capacity provider strategy for the cluster. When services or tasks are run in the cluster with no
* launch type or capacity provider strategy specified, the default capacity provider strategy is used.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem.Builder} avoiding the need to
* create one manually via
* {@link software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem.Builder#build()} is called
* immediately and its result is passed to {@link
* #defaultCapacityProviderStrategy(List)}.
*
* @param defaultCapacityProviderStrategy
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #defaultCapacityProviderStrategy(java.util.Collection)
*/
Builder defaultCapacityProviderStrategy(Consumer... defaultCapacityProviderStrategy);
/**
*
* The resources attached to a cluster. When using a capacity provider with a cluster, the capacity provider and
* associated resources are returned as cluster attachments.
*
*
* @param attachments
* The resources attached to a cluster. When using a capacity provider with a cluster, the capacity
* provider and associated resources are returned as cluster attachments.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder attachments(Collection attachments);
/**
*
* The resources attached to a cluster. When using a capacity provider with a cluster, the capacity provider and
* associated resources are returned as cluster attachments.
*
*
* @param attachments
* The resources attached to a cluster. When using a capacity provider with a cluster, the capacity
* provider and associated resources are returned as cluster attachments.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder attachments(Attachment... attachments);
/**
*
* The resources attached to a cluster. When using a capacity provider with a cluster, the capacity provider and
* associated resources are returned as cluster attachments.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ecs.model.Attachment.Builder} avoiding the need to create one manually
* via {@link software.amazon.awssdk.services.ecs.model.Attachment#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ecs.model.Attachment.Builder#build()} is called immediately and its
* result is passed to {@link #attachments(List)}.
*
* @param attachments
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ecs.model.Attachment.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #attachments(java.util.Collection)
*/
Builder attachments(Consumer... attachments);
/**
*
* The status of the capacity providers associated with the cluster. The following are the states that are
* returned.
*
*
* - UPDATE_IN_PROGRESS
* -
*
* The available capacity providers for the cluster are updating.
*
*
* - UPDATE_COMPLETE
* -
*
* The capacity providers have successfully updated.
*
*
* - UPDATE_FAILED
* -
*
* The capacity provider updates failed.
*
*
*
*
* @param attachmentsStatus
* The status of the capacity providers associated with the cluster. The following are the states that
* are returned.
*
* - UPDATE_IN_PROGRESS
* -
*
* The available capacity providers for the cluster are updating.
*
*
* - UPDATE_COMPLETE
* -
*
* The capacity providers have successfully updated.
*
*
* - UPDATE_FAILED
* -
*
* The capacity provider updates failed.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder attachmentsStatus(String attachmentsStatus);
/**
*
* Use this parameter to set a default Service Connect namespace. After you set a default Service Connect
* namespace, any new services with Service Connect turned on that are created in the cluster are added as
* client services in the namespace. This setting only applies to new services that set the enabled
* parameter to true
in the ServiceConnectConfiguration
. You can set the namespace of
* each service individually in the ServiceConnectConfiguration
to override this default parameter.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect
* to services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are
* supported with Service Connect. For more information, see Service Connect
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param serviceConnectDefaults
* Use this parameter to set a default Service Connect namespace. After you set a default Service Connect
* namespace, any new services with Service Connect turned on that are created in the cluster are added
* as client services in the namespace. This setting only applies to new services that set the
* enabled
parameter to true
in the ServiceConnectConfiguration
.
* You can set the namespace of each service individually in the ServiceConnectConfiguration
* to override this default parameter.
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder serviceConnectDefaults(ClusterServiceConnectDefaults serviceConnectDefaults);
/**
*
* Use this parameter to set a default Service Connect namespace. After you set a default Service Connect
* namespace, any new services with Service Connect turned on that are created in the cluster are added as
* client services in the namespace. This setting only applies to new services that set the enabled
* parameter to true
in the ServiceConnectConfiguration
. You can set the namespace of
* each service individually in the ServiceConnectConfiguration
to override this default parameter.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect
* to services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are
* supported with Service Connect. For more information, see Service Connect
* in the Amazon Elastic Container Service Developer Guide.
*
* This is a convenience method that creates an instance of the {@link ClusterServiceConnectDefaults.Builder}
* avoiding the need to create one manually via {@link ClusterServiceConnectDefaults#builder()}.
*
*
* When the {@link Consumer} completes, {@link ClusterServiceConnectDefaults.Builder#build()} is called
* immediately and its result is passed to {@link #serviceConnectDefaults(ClusterServiceConnectDefaults)}.
*
* @param serviceConnectDefaults
* a consumer that will call methods on {@link ClusterServiceConnectDefaults.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #serviceConnectDefaults(ClusterServiceConnectDefaults)
*/
default Builder serviceConnectDefaults(Consumer serviceConnectDefaults) {
return serviceConnectDefaults(ClusterServiceConnectDefaults.builder().applyMutation(serviceConnectDefaults).build());
}
}
static final class BuilderImpl implements Builder {
private String clusterArn;
private String clusterName;
private ClusterConfiguration configuration;
private String status;
private Integer registeredContainerInstancesCount;
private Integer runningTasksCount;
private Integer pendingTasksCount;
private Integer activeServicesCount;
private List statistics = DefaultSdkAutoConstructList.getInstance();
private List tags = DefaultSdkAutoConstructList.getInstance();
private List settings = DefaultSdkAutoConstructList.getInstance();
private List capacityProviders = DefaultSdkAutoConstructList.getInstance();
private List defaultCapacityProviderStrategy = DefaultSdkAutoConstructList.getInstance();
private List attachments = DefaultSdkAutoConstructList.getInstance();
private String attachmentsStatus;
private ClusterServiceConnectDefaults serviceConnectDefaults;
private BuilderImpl() {
}
private BuilderImpl(Cluster model) {
clusterArn(model.clusterArn);
clusterName(model.clusterName);
configuration(model.configuration);
status(model.status);
registeredContainerInstancesCount(model.registeredContainerInstancesCount);
runningTasksCount(model.runningTasksCount);
pendingTasksCount(model.pendingTasksCount);
activeServicesCount(model.activeServicesCount);
statistics(model.statistics);
tags(model.tags);
settings(model.settings);
capacityProviders(model.capacityProviders);
defaultCapacityProviderStrategy(model.defaultCapacityProviderStrategy);
attachments(model.attachments);
attachmentsStatus(model.attachmentsStatus);
serviceConnectDefaults(model.serviceConnectDefaults);
}
public final String getClusterArn() {
return clusterArn;
}
public final void setClusterArn(String clusterArn) {
this.clusterArn = clusterArn;
}
@Override
public final Builder clusterArn(String clusterArn) {
this.clusterArn = clusterArn;
return this;
}
public final String getClusterName() {
return clusterName;
}
public final void setClusterName(String clusterName) {
this.clusterName = clusterName;
}
@Override
public final Builder clusterName(String clusterName) {
this.clusterName = clusterName;
return this;
}
public final ClusterConfiguration.Builder getConfiguration() {
return configuration != null ? configuration.toBuilder() : null;
}
public final void setConfiguration(ClusterConfiguration.BuilderImpl configuration) {
this.configuration = configuration != null ? configuration.build() : null;
}
@Override
public final Builder configuration(ClusterConfiguration configuration) {
this.configuration = configuration;
return this;
}
public final String getStatus() {
return status;
}
public final void setStatus(String status) {
this.status = status;
}
@Override
public final Builder status(String status) {
this.status = status;
return this;
}
public final Integer getRegisteredContainerInstancesCount() {
return registeredContainerInstancesCount;
}
public final void setRegisteredContainerInstancesCount(Integer registeredContainerInstancesCount) {
this.registeredContainerInstancesCount = registeredContainerInstancesCount;
}
@Override
public final Builder registeredContainerInstancesCount(Integer registeredContainerInstancesCount) {
this.registeredContainerInstancesCount = registeredContainerInstancesCount;
return this;
}
public final Integer getRunningTasksCount() {
return runningTasksCount;
}
public final void setRunningTasksCount(Integer runningTasksCount) {
this.runningTasksCount = runningTasksCount;
}
@Override
public final Builder runningTasksCount(Integer runningTasksCount) {
this.runningTasksCount = runningTasksCount;
return this;
}
public final Integer getPendingTasksCount() {
return pendingTasksCount;
}
public final void setPendingTasksCount(Integer pendingTasksCount) {
this.pendingTasksCount = pendingTasksCount;
}
@Override
public final Builder pendingTasksCount(Integer pendingTasksCount) {
this.pendingTasksCount = pendingTasksCount;
return this;
}
public final Integer getActiveServicesCount() {
return activeServicesCount;
}
public final void setActiveServicesCount(Integer activeServicesCount) {
this.activeServicesCount = activeServicesCount;
}
@Override
public final Builder activeServicesCount(Integer activeServicesCount) {
this.activeServicesCount = activeServicesCount;
return this;
}
public final List getStatistics() {
List result = StatisticsCopier.copyToBuilder(this.statistics);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setStatistics(Collection statistics) {
this.statistics = StatisticsCopier.copyFromBuilder(statistics);
}
@Override
public final Builder statistics(Collection statistics) {
this.statistics = StatisticsCopier.copy(statistics);
return this;
}
@Override
@SafeVarargs
public final Builder statistics(KeyValuePair... statistics) {
statistics(Arrays.asList(statistics));
return this;
}
@Override
@SafeVarargs
public final Builder statistics(Consumer... statistics) {
statistics(Stream.of(statistics).map(c -> KeyValuePair.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final List getTags() {
List result = TagsCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagsCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagsCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final List getSettings() {
List result = ClusterSettingsCopier.copyToBuilder(this.settings);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setSettings(Collection settings) {
this.settings = ClusterSettingsCopier.copyFromBuilder(settings);
}
@Override
@Transient
public final Builder settings(Collection settings) {
this.settings = ClusterSettingsCopier.copy(settings);
return this;
}
@Override
@Transient
@SafeVarargs
public final Builder settings(ClusterSetting... settings) {
settings(Arrays.asList(settings));
return this;
}
@Override
@Transient
@SafeVarargs
public final Builder settings(Consumer... settings) {
settings(Stream.of(settings).map(c -> ClusterSetting.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Collection getCapacityProviders() {
if (capacityProviders instanceof SdkAutoConstructList) {
return null;
}
return capacityProviders;
}
public final void setCapacityProviders(Collection capacityProviders) {
this.capacityProviders = StringListCopier.copy(capacityProviders);
}
@Override
public final Builder capacityProviders(Collection capacityProviders) {
this.capacityProviders = StringListCopier.copy(capacityProviders);
return this;
}
@Override
@SafeVarargs
public final Builder capacityProviders(String... capacityProviders) {
capacityProviders(Arrays.asList(capacityProviders));
return this;
}
public final List getDefaultCapacityProviderStrategy() {
List result = CapacityProviderStrategyCopier
.copyToBuilder(this.defaultCapacityProviderStrategy);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setDefaultCapacityProviderStrategy(
Collection defaultCapacityProviderStrategy) {
this.defaultCapacityProviderStrategy = CapacityProviderStrategyCopier
.copyFromBuilder(defaultCapacityProviderStrategy);
}
@Override
public final Builder defaultCapacityProviderStrategy(
Collection defaultCapacityProviderStrategy) {
this.defaultCapacityProviderStrategy = CapacityProviderStrategyCopier.copy(defaultCapacityProviderStrategy);
return this;
}
@Override
@SafeVarargs
public final Builder defaultCapacityProviderStrategy(CapacityProviderStrategyItem... defaultCapacityProviderStrategy) {
defaultCapacityProviderStrategy(Arrays.asList(defaultCapacityProviderStrategy));
return this;
}
@Override
@SafeVarargs
public final Builder defaultCapacityProviderStrategy(
Consumer... defaultCapacityProviderStrategy) {
defaultCapacityProviderStrategy(Stream.of(defaultCapacityProviderStrategy)
.map(c -> CapacityProviderStrategyItem.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final List getAttachments() {
List result = AttachmentsCopier.copyToBuilder(this.attachments);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setAttachments(Collection attachments) {
this.attachments = AttachmentsCopier.copyFromBuilder(attachments);
}
@Override
public final Builder attachments(Collection attachments) {
this.attachments = AttachmentsCopier.copy(attachments);
return this;
}
@Override
@SafeVarargs
public final Builder attachments(Attachment... attachments) {
attachments(Arrays.asList(attachments));
return this;
}
@Override
@SafeVarargs
public final Builder attachments(Consumer... attachments) {
attachments(Stream.of(attachments).map(c -> Attachment.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final String getAttachmentsStatus() {
return attachmentsStatus;
}
public final void setAttachmentsStatus(String attachmentsStatus) {
this.attachmentsStatus = attachmentsStatus;
}
@Override
public final Builder attachmentsStatus(String attachmentsStatus) {
this.attachmentsStatus = attachmentsStatus;
return this;
}
public final ClusterServiceConnectDefaults.Builder getServiceConnectDefaults() {
return serviceConnectDefaults != null ? serviceConnectDefaults.toBuilder() : null;
}
public final void setServiceConnectDefaults(ClusterServiceConnectDefaults.BuilderImpl serviceConnectDefaults) {
this.serviceConnectDefaults = serviceConnectDefaults != null ? serviceConnectDefaults.build() : null;
}
@Override
public final Builder serviceConnectDefaults(ClusterServiceConnectDefaults serviceConnectDefaults) {
this.serviceConnectDefaults = serviceConnectDefaults;
return this;
}
@Override
public Cluster build() {
return new Cluster(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}