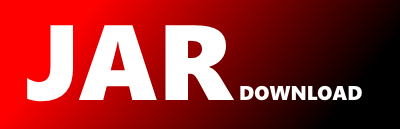
software.amazon.awssdk.services.ecs.model.ManagedScaling Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The managed scaling settings for the Auto Scaling group capacity provider.
*
*
* When managed scaling is turned on, Amazon ECS manages the scale-in and scale-out actions of the Auto Scaling group.
* Amazon ECS manages a target tracking scaling policy using an Amazon ECS managed CloudWatch metric with the specified
* targetCapacity
value as the target value for the metric. For more information, see Using managed scaling in the Amazon Elastic Container Service Developer Guide.
*
*
* If managed scaling is off, the user must manage the scaling of the Auto Scaling group.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ManagedScaling implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(ManagedScaling::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField TARGET_CAPACITY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("targetCapacity").getter(getter(ManagedScaling::targetCapacity)).setter(setter(Builder::targetCapacity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetCapacity").build()).build();
private static final SdkField MINIMUM_SCALING_STEP_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("minimumScalingStepSize").getter(getter(ManagedScaling::minimumScalingStepSize))
.setter(setter(Builder::minimumScalingStepSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("minimumScalingStepSize").build())
.build();
private static final SdkField MAXIMUM_SCALING_STEP_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("maximumScalingStepSize").getter(getter(ManagedScaling::maximumScalingStepSize))
.setter(setter(Builder::maximumScalingStepSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maximumScalingStepSize").build())
.build();
private static final SdkField INSTANCE_WARMUP_PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("instanceWarmupPeriod").getter(getter(ManagedScaling::instanceWarmupPeriod))
.setter(setter(Builder::instanceWarmupPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("instanceWarmupPeriod").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays
.asList(STATUS_FIELD, TARGET_CAPACITY_FIELD, MINIMUM_SCALING_STEP_SIZE_FIELD, MAXIMUM_SCALING_STEP_SIZE_FIELD,
INSTANCE_WARMUP_PERIOD_FIELD));
private static final long serialVersionUID = 1L;
private final String status;
private final Integer targetCapacity;
private final Integer minimumScalingStepSize;
private final Integer maximumScalingStepSize;
private final Integer instanceWarmupPeriod;
private ManagedScaling(BuilderImpl builder) {
this.status = builder.status;
this.targetCapacity = builder.targetCapacity;
this.minimumScalingStepSize = builder.minimumScalingStepSize;
this.maximumScalingStepSize = builder.maximumScalingStepSize;
this.instanceWarmupPeriod = builder.instanceWarmupPeriod;
}
/**
*
* Determines whether to use managed scaling for the capacity provider.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ManagedScalingStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return Determines whether to use managed scaling for the capacity provider.
* @see ManagedScalingStatus
*/
public final ManagedScalingStatus status() {
return ManagedScalingStatus.fromValue(status);
}
/**
*
* Determines whether to use managed scaling for the capacity provider.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ManagedScalingStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return Determines whether to use managed scaling for the capacity provider.
* @see ManagedScalingStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The target capacity utilization as a percentage for the capacity provider. The specified value must be greater
* than 0
and less than or equal to 100
. For example, if you want the capacity provider to
* maintain 10% spare capacity, then that means the utilization is 90%, so use a targetCapacity
of
* 90
. The default value of 100
percent results in the Amazon EC2 instances in your Auto
* Scaling group being completely used.
*
*
* @return The target capacity utilization as a percentage for the capacity provider. The specified value must be
* greater than 0
and less than or equal to 100
. For example, if you want the
* capacity provider to maintain 10% spare capacity, then that means the utilization is 90%, so use a
* targetCapacity
of 90
. The default value of 100
percent results in
* the Amazon EC2 instances in your Auto Scaling group being completely used.
*/
public final Integer targetCapacity() {
return targetCapacity;
}
/**
*
* The minimum number of Amazon EC2 instances that Amazon ECS will scale out at one time. The scale in process is
* not affected by this parameter If this parameter is omitted, the default value of 1
is used.
*
*
* When additional capacity is required, Amazon ECS will scale up the minimum scaling step size even if the actual
* demand is less than the minimum scaling step size.
*
*
* If you use a capacity provider with an Auto Scaling group configured with more than one Amazon EC2 instance type
* or Availability Zone, Amazon ECS will scale up by the exact minimum scaling step size value and will ignore both
* the maximum scaling step size as well as the capacity demand.
*
*
* @return The minimum number of Amazon EC2 instances that Amazon ECS will scale out at one time. The scale in
* process is not affected by this parameter If this parameter is omitted, the default value of
* 1
is used.
*
* When additional capacity is required, Amazon ECS will scale up the minimum scaling step size even if the
* actual demand is less than the minimum scaling step size.
*
*
* If you use a capacity provider with an Auto Scaling group configured with more than one Amazon EC2
* instance type or Availability Zone, Amazon ECS will scale up by the exact minimum scaling step size value
* and will ignore both the maximum scaling step size as well as the capacity demand.
*/
public final Integer minimumScalingStepSize() {
return minimumScalingStepSize;
}
/**
*
* The maximum number of Amazon EC2 instances that Amazon ECS will scale out at one time. The scale in process is
* not affected by this parameter. If this parameter is omitted, the default value of 10000
is used.
*
*
* @return The maximum number of Amazon EC2 instances that Amazon ECS will scale out at one time. The scale in
* process is not affected by this parameter. If this parameter is omitted, the default value of
* 10000
is used.
*/
public final Integer maximumScalingStepSize() {
return maximumScalingStepSize;
}
/**
*
* The period of time, in seconds, after a newly launched Amazon EC2 instance can contribute to CloudWatch metrics
* for Auto Scaling group. If this parameter is omitted, the default value of 300
seconds is used.
*
*
* @return The period of time, in seconds, after a newly launched Amazon EC2 instance can contribute to CloudWatch
* metrics for Auto Scaling group. If this parameter is omitted, the default value of 300
* seconds is used.
*/
public final Integer instanceWarmupPeriod() {
return instanceWarmupPeriod;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(targetCapacity());
hashCode = 31 * hashCode + Objects.hashCode(minimumScalingStepSize());
hashCode = 31 * hashCode + Objects.hashCode(maximumScalingStepSize());
hashCode = 31 * hashCode + Objects.hashCode(instanceWarmupPeriod());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ManagedScaling)) {
return false;
}
ManagedScaling other = (ManagedScaling) obj;
return Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(targetCapacity(), other.targetCapacity())
&& Objects.equals(minimumScalingStepSize(), other.minimumScalingStepSize())
&& Objects.equals(maximumScalingStepSize(), other.maximumScalingStepSize())
&& Objects.equals(instanceWarmupPeriod(), other.instanceWarmupPeriod());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ManagedScaling").add("Status", statusAsString()).add("TargetCapacity", targetCapacity())
.add("MinimumScalingStepSize", minimumScalingStepSize()).add("MaximumScalingStepSize", maximumScalingStepSize())
.add("InstanceWarmupPeriod", instanceWarmupPeriod()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "targetCapacity":
return Optional.ofNullable(clazz.cast(targetCapacity()));
case "minimumScalingStepSize":
return Optional.ofNullable(clazz.cast(minimumScalingStepSize()));
case "maximumScalingStepSize":
return Optional.ofNullable(clazz.cast(maximumScalingStepSize()));
case "instanceWarmupPeriod":
return Optional.ofNullable(clazz.cast(instanceWarmupPeriod()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function