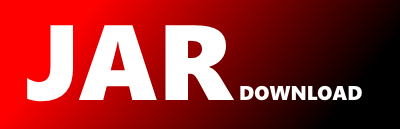
software.amazon.awssdk.services.ecs.model.SystemControl Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A list of namespaced kernel parameters to set in the container. This parameter maps to Sysctls
in the Create a container section of the Docker Remote API and the --sysctl
option to docker run. For example, you can
* configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived connections.
*
*
* We don't recommend that you specify network-related systemControls
parameters for multiple containers in
* a single task that also uses either the awsvpc
or host
network mode. Doing this has the
* following disadvantages:
*
*
* -
*
* For tasks that use the awsvpc
network mode including Fargate, if you set systemControls
for
* any container, it applies to all containers in the task. If you set different systemControls
for
* multiple containers in a single task, the container that's started last determines which systemControls
* take effect.
*
*
* -
*
* For tasks that use the host
network mode, the network namespace systemControls
aren't
* supported.
*
*
*
*
* If you're setting an IPC resource namespace to use for the containers in the task, the following conditions apply to
* your system controls. For more information, see IPC mode.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace systemControls
aren't supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace systemControls
values apply to all
* containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SystemControl implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("namespace").getter(getter(SystemControl::namespace)).setter(setter(Builder::namespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("namespace").build()).build();
private static final SdkField VALUE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("value")
.getter(getter(SystemControl::value)).setter(setter(Builder::value))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("value").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAMESPACE_FIELD, VALUE_FIELD));
private static final long serialVersionUID = 1L;
private final String namespace;
private final String value;
private SystemControl(BuilderImpl builder) {
this.namespace = builder.namespace;
this.value = builder.value;
}
/**
*
* The namespaced kernel parameter to set a value
for.
*
*
* @return The namespaced kernel parameter to set a value
for.
*/
public final String namespace() {
return namespace;
}
/**
*
* The namespaced kernel parameter to set a value
for.
*
*
* Valid IPC namespace values:
* "kernel.msgmax" | "kernel.msgmnb" | "kernel.msgmni" | "kernel.sem" | "kernel.shmall" | "kernel.shmmax" | "kernel.shmmni" | "kernel.shm_rmid_forced"
* , and Sysctls
that start with "fs.mqueue.*"
*
*
* Valid network namespace values: Sysctls
that start with "net.*"
*
*
* All of these values are supported by Fargate.
*
*
* @return The namespaced kernel parameter to set a value
for.
*
* Valid IPC namespace values:
* "kernel.msgmax" | "kernel.msgmnb" | "kernel.msgmni" | "kernel.sem" | "kernel.shmall" | "kernel.shmmax" | "kernel.shmmni" | "kernel.shm_rmid_forced"
* , and Sysctls
that start with "fs.mqueue.*"
*
*
* Valid network namespace values: Sysctls
that start with "net.*"
*
*
* All of these values are supported by Fargate.
*/
public final String value() {
return value;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(namespace());
hashCode = 31 * hashCode + Objects.hashCode(value());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SystemControl)) {
return false;
}
SystemControl other = (SystemControl) obj;
return Objects.equals(namespace(), other.namespace()) && Objects.equals(value(), other.value());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SystemControl").add("Namespace", namespace()).add("Value", value()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "namespace":
return Optional.ofNullable(clazz.cast(namespace()));
case "value":
return Optional.ofNullable(clazz.cast(value()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Valid IPC namespace values:
* "kernel.msgmax" | "kernel.msgmnb" | "kernel.msgmni" | "kernel.sem" | "kernel.shmall" | "kernel.shmmax" | "kernel.shmmni" | "kernel.shm_rmid_forced"
* , and Sysctls
that start with "fs.mqueue.*"
*
*
* Valid network namespace values: Sysctls
that start with "net.*"
*
*
* All of these values are supported by Fargate.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder value(String value);
}
static final class BuilderImpl implements Builder {
private String namespace;
private String value;
private BuilderImpl() {
}
private BuilderImpl(SystemControl model) {
namespace(model.namespace);
value(model.value);
}
public final String getNamespace() {
return namespace;
}
public final void setNamespace(String namespace) {
this.namespace = namespace;
}
@Override
public final Builder namespace(String namespace) {
this.namespace = namespace;
return this;
}
public final String getValue() {
return value;
}
public final void setValue(String value) {
this.value = value;
}
@Override
public final Builder value(String value) {
this.value = value;
return this;
}
@Override
public SystemControl build() {
return new SystemControl(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}