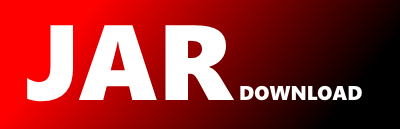
software.amazon.awssdk.services.ecs.model.ListServicesByNamespaceRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListServicesByNamespaceRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField NAMESPACE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("namespace").getter(getter(ListServicesByNamespaceRequest::namespace)).setter(setter(Builder::namespace))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("namespace").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("nextToken").getter(getter(ListServicesByNamespaceRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nextToken").build()).build();
private static final SdkField MAX_RESULTS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("maxResults").getter(getter(ListServicesByNamespaceRequest::maxResults))
.setter(setter(Builder::maxResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maxResults").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAMESPACE_FIELD,
NEXT_TOKEN_FIELD, MAX_RESULTS_FIELD));
private final String namespace;
private final String nextToken;
private final Integer maxResults;
private ListServicesByNamespaceRequest(BuilderImpl builder) {
super(builder);
this.namespace = builder.namespace;
this.nextToken = builder.nextToken;
this.maxResults = builder.maxResults;
}
/**
*
* The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace to list the services in.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace to list the services
* in.
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
*/
public final String namespace() {
return namespace;
}
/**
*
* The nextToken
value that's returned from a ListServicesByNamespace
request. It
* indicates that more results are available to fulfill the request and further calls are needed. If
* maxResults
is returned, it is possible the number of results is less than maxResults
.
*
*
* @return The nextToken
value that's returned from a ListServicesByNamespace
request. It
* indicates that more results are available to fulfill the request and further calls are needed. If
* maxResults
is returned, it is possible the number of results is less than
* maxResults
.
*/
public final String nextToken() {
return nextToken;
}
/**
*
* The maximum number of service results that ListServicesByNamespace
returns in paginated output. When
* this parameter is used, ListServicesByNamespace
only returns maxResults
results in a
* single page along with a nextToken
response element. The remaining results of the initial request
* can be seen by sending another ListServicesByNamespace
request with the returned
* nextToken
value. This value can be between 1 and 100. If this parameter isn't used, then
* ListServicesByNamespace
returns up to 10 results and a nextToken
value if applicable.
*
*
* @return The maximum number of service results that ListServicesByNamespace
returns in paginated
* output. When this parameter is used, ListServicesByNamespace
only returns
* maxResults
results in a single page along with a nextToken
response element.
* The remaining results of the initial request can be seen by sending another
* ListServicesByNamespace
request with the returned nextToken
value. This value
* can be between 1 and 100. If this parameter isn't used, then ListServicesByNamespace
returns
* up to 10 results and a nextToken
value if applicable.
*/
public final Integer maxResults() {
return maxResults;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(namespace());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(maxResults());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListServicesByNamespaceRequest)) {
return false;
}
ListServicesByNamespaceRequest other = (ListServicesByNamespaceRequest) obj;
return Objects.equals(namespace(), other.namespace()) && Objects.equals(nextToken(), other.nextToken())
&& Objects.equals(maxResults(), other.maxResults());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListServicesByNamespaceRequest").add("Namespace", namespace()).add("NextToken", nextToken())
.add("MaxResults", maxResults()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "namespace":
return Optional.ofNullable(clazz.cast(namespace()));
case "nextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "maxResults":
return Optional.ofNullable(clazz.cast(maxResults()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function