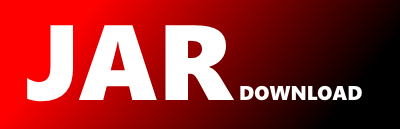
software.amazon.awssdk.services.ecs.model.Secret Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An object representing the secret to expose to your container. Secrets can be exposed to a container in the following
* ways:
*
*
* -
*
* To inject sensitive data into your containers as environment variables, use the secrets
container
* definition parameter.
*
*
* -
*
* To reference sensitive information in the log configuration of a container, use the secretOptions
* container definition parameter.
*
*
*
*
* For more information, see Specifying
* sensitive data in the Amazon Elastic Container Service Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Secret implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(Secret::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField VALUE_FROM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("valueFrom").getter(getter(Secret::valueFrom)).setter(setter(Builder::valueFrom))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("valueFrom").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, VALUE_FROM_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final String valueFrom;
private Secret(BuilderImpl builder) {
this.name = builder.name;
this.valueFrom = builder.valueFrom;
}
/**
*
* The name of the secret.
*
*
* @return The name of the secret.
*/
public final String name() {
return name;
}
/**
*
* The secret to expose to the container. The supported values are either the full ARN of the Secrets Manager secret
* or the full ARN of the parameter in the SSM Parameter Store.
*
*
* For information about the require Identity and Access Management permissions, see Required IAM permissions for Amazon ECS secrets (for Secrets Manager) or Required IAM permissions for Amazon ECS secrets (for Systems Manager Parameter store) in the Amazon
* Elastic Container Service Developer Guide.
*
*
*
* If the SSM Parameter Store parameter exists in the same Region as the task you're launching, then you can use
* either the full ARN or name of the parameter. If the parameter exists in a different Region, then the full ARN
* must be specified.
*
*
*
* @return The secret to expose to the container. The supported values are either the full ARN of the Secrets
* Manager secret or the full ARN of the parameter in the SSM Parameter Store.
*
* For information about the require Identity and Access Management permissions, see Required IAM permissions for Amazon ECS secrets (for Secrets Manager) or Required IAM permissions for Amazon ECS secrets (for Systems Manager Parameter store) in the
* Amazon Elastic Container Service Developer Guide.
*
*
*
* If the SSM Parameter Store parameter exists in the same Region as the task you're launching, then you can
* use either the full ARN or name of the parameter. If the parameter exists in a different Region, then the
* full ARN must be specified.
*
*/
public final String valueFrom() {
return valueFrom;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(valueFrom());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Secret)) {
return false;
}
Secret other = (Secret) obj;
return Objects.equals(name(), other.name()) && Objects.equals(valueFrom(), other.valueFrom());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Secret").add("Name", name()).add("ValueFrom", valueFrom()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "valueFrom":
return Optional.ofNullable(clazz.cast(valueFrom()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function