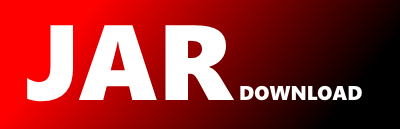
software.amazon.awssdk.services.ecs.model.ExecuteCommandRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecs Show documentation
Show all versions of ecs Show documentation
The AWS Java SDK for the Amazon EC2 Container Service holds the client classes that are used for
communicating with the
Amazon EC2 Container Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ExecuteCommandRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(ExecuteCommandRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField CONTAINER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("container").getter(getter(ExecuteCommandRequest::container)).setter(setter(Builder::container))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("container").build()).build();
private static final SdkField COMMAND_FIELD = SdkField. builder(MarshallingType.STRING).memberName("command")
.getter(getter(ExecuteCommandRequest::command)).setter(setter(Builder::command))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("command").build()).build();
private static final SdkField INTERACTIVE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("interactive").getter(getter(ExecuteCommandRequest::interactive)).setter(setter(Builder::interactive))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("interactive").build()).build();
private static final SdkField TASK_FIELD = SdkField. builder(MarshallingType.STRING).memberName("task")
.getter(getter(ExecuteCommandRequest::task)).setter(setter(Builder::task))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("task").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD,
CONTAINER_FIELD, COMMAND_FIELD, INTERACTIVE_FIELD, TASK_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("cluster", CLUSTER_FIELD);
put("container", CONTAINER_FIELD);
put("command", COMMAND_FIELD);
put("interactive", INTERACTIVE_FIELD);
put("task", TASK_FIELD);
}
});
private final String cluster;
private final String container;
private final String command;
private final Boolean interactive;
private final String task;
private ExecuteCommandRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.container = builder.container;
this.command = builder.command;
this.interactive = builder.interactive;
this.task = builder.task;
}
/**
*
* The Amazon Resource Name (ARN) or short name of the cluster the task is running in. If you do not specify a
* cluster, the default cluster is assumed.
*
*
* @return The Amazon Resource Name (ARN) or short name of the cluster the task is running in. If you do not specify
* a cluster, the default cluster is assumed.
*/
public final String cluster() {
return cluster;
}
/**
*
* The name of the container to execute the command on. A container name only needs to be specified for tasks
* containing multiple containers.
*
*
* @return The name of the container to execute the command on. A container name only needs to be specified for
* tasks containing multiple containers.
*/
public final String container() {
return container;
}
/**
*
* The command to run on the container.
*
*
* @return The command to run on the container.
*/
public final String command() {
return command;
}
/**
*
* Use this flag to run your command in interactive mode.
*
*
* @return Use this flag to run your command in interactive mode.
*/
public final Boolean interactive() {
return interactive;
}
/**
*
* The Amazon Resource Name (ARN) or ID of the task the container is part of.
*
*
* @return The Amazon Resource Name (ARN) or ID of the task the container is part of.
*/
public final String task() {
return task;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(container());
hashCode = 31 * hashCode + Objects.hashCode(command());
hashCode = 31 * hashCode + Objects.hashCode(interactive());
hashCode = 31 * hashCode + Objects.hashCode(task());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ExecuteCommandRequest)) {
return false;
}
ExecuteCommandRequest other = (ExecuteCommandRequest) obj;
return Objects.equals(cluster(), other.cluster()) && Objects.equals(container(), other.container())
&& Objects.equals(command(), other.command()) && Objects.equals(interactive(), other.interactive())
&& Objects.equals(task(), other.task());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ExecuteCommandRequest").add("Cluster", cluster()).add("Container", container())
.add("Command", command()).add("Interactive", interactive()).add("Task", task()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "container":
return Optional.ofNullable(clazz.cast(container()));
case "command":
return Optional.ofNullable(clazz.cast(command()));
case "interactive":
return Optional.ofNullable(clazz.cast(interactive()));
case "task":
return Optional.ofNullable(clazz.cast(task()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy