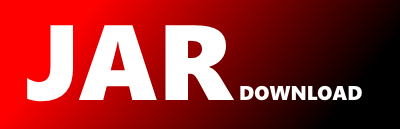
software.amazon.awssdk.services.ecs.model.PutClusterCapacityProvidersRequest Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutClusterCapacityProvidersRequest extends EcsRequest implements
ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("cluster")
.getter(getter(PutClusterCapacityProvidersRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField> CAPACITY_PROVIDERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviders")
.getter(getter(PutClusterCapacityProvidersRequest::capacityProviders))
.setter(setter(Builder::capacityProviders))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviders").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> DEFAULT_CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("defaultCapacityProviderStrategy")
.getter(getter(PutClusterCapacityProvidersRequest::defaultCapacityProviderStrategy))
.setter(setter(Builder::defaultCapacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("defaultCapacityProviderStrategy")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD,
CAPACITY_PROVIDERS_FIELD, DEFAULT_CAPACITY_PROVIDER_STRATEGY_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("cluster", CLUSTER_FIELD);
put("capacityProviders", CAPACITY_PROVIDERS_FIELD);
put("defaultCapacityProviderStrategy", DEFAULT_CAPACITY_PROVIDER_STRATEGY_FIELD);
}
});
private final String cluster;
private final List capacityProviders;
private final List defaultCapacityProviderStrategy;
private PutClusterCapacityProvidersRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.capacityProviders = builder.capacityProviders;
this.defaultCapacityProviderStrategy = builder.defaultCapacityProviderStrategy;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster to modify the capacity provider settings for. If
* you don't specify a cluster, the default cluster is assumed.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster to modify the capacity provider settings
* for. If you don't specify a cluster, the default cluster is assumed.
*/
public final String cluster() {
return cluster;
}
/**
* For responses, this returns true if the service returned a value for the CapacityProviders property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCapacityProviders() {
return capacityProviders != null && !(capacityProviders instanceof SdkAutoConstructList);
}
/**
*
* The name of one or more capacity providers to associate with the cluster.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be created.
* New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
capacity
* providers. The Fargate capacity providers are available to all accounts and only need to be associated with a
* cluster to be used.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapacityProviders} method.
*
*
* @return The name of one or more capacity providers to associate with the cluster.
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be
* created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
* capacity providers. The Fargate capacity providers are available to all accounts and only need to be
* associated with a cluster to be used.
*/
public final List capacityProviders() {
return capacityProviders;
}
/**
* For responses, this returns true if the service returned a value for the DefaultCapacityProviderStrategy
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasDefaultCapacityProviderStrategy() {
return defaultCapacityProviderStrategy != null && !(defaultCapacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The capacity provider strategy to use by default for the cluster.
*
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is specified then
* the default capacity provider strategy for the cluster is used.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the base
and
* weight
to assign to them. A capacity provider must be associated with the cluster to be used in a
* capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider with a cluster. Only capacity
* providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be created.
* New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
capacity
* providers. The Fargate capacity providers are available to all accounts and only need to be associated with a
* cluster to be used.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDefaultCapacityProviderStrategy}
* method.
*
*
* @return The capacity provider strategy to use by default for the cluster.
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is
* specified then the default capacity provider strategy for the cluster is used.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the base
* and weight
to assign to them. A capacity provider must be associated with the cluster to be
* used in a capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider with a cluster. Only
* capacity providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be
* created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
* capacity providers. The Fargate capacity providers are available to all accounts and only need to be
* associated with a cluster to be used.
*/
public final List defaultCapacityProviderStrategy() {
return defaultCapacityProviderStrategy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviders() ? capacityProviders() : null);
hashCode = 31 * hashCode
+ Objects.hashCode(hasDefaultCapacityProviderStrategy() ? defaultCapacityProviderStrategy() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutClusterCapacityProvidersRequest)) {
return false;
}
PutClusterCapacityProvidersRequest other = (PutClusterCapacityProvidersRequest) obj;
return Objects.equals(cluster(), other.cluster()) && hasCapacityProviders() == other.hasCapacityProviders()
&& Objects.equals(capacityProviders(), other.capacityProviders())
&& hasDefaultCapacityProviderStrategy() == other.hasDefaultCapacityProviderStrategy()
&& Objects.equals(defaultCapacityProviderStrategy(), other.defaultCapacityProviderStrategy());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("PutClusterCapacityProvidersRequest")
.add("Cluster", cluster())
.add("CapacityProviders", hasCapacityProviders() ? capacityProviders() : null)
.add("DefaultCapacityProviderStrategy",
hasDefaultCapacityProviderStrategy() ? defaultCapacityProviderStrategy() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "capacityProviders":
return Optional.ofNullable(clazz.cast(capacityProviders()));
case "defaultCapacityProviderStrategy":
return Optional.ofNullable(clazz.cast(defaultCapacityProviderStrategy()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already
* be created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or
* FARGATE_SPOT
capacity providers. The Fargate capacity providers are available to all
* accounts and only need to be associated with a cluster to be used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capacityProviders(Collection capacityProviders);
/**
*
* The name of one or more capacity providers to associate with the cluster.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be
* created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
* capacity providers. The Fargate capacity providers are available to all accounts and only need to be
* associated with a cluster to be used.
*
*
* @param capacityProviders
* The name of one or more capacity providers to associate with the cluster.
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already
* be created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or
* FARGATE_SPOT
capacity providers. The Fargate capacity providers are available to all
* accounts and only need to be associated with a cluster to be used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder capacityProviders(String... capacityProviders);
/**
*
* The capacity provider strategy to use by default for the cluster.
*
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is specified
* then the default capacity provider strategy for the cluster is used.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the base
and
* weight
to assign to them. A capacity provider must be associated with the cluster to be used in
* a capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider with a cluster. Only capacity
* providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be
* created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
* capacity providers. The Fargate capacity providers are available to all accounts and only need to be
* associated with a cluster to be used.
*
*
* @param defaultCapacityProviderStrategy
* The capacity provider strategy to use by default for the cluster.
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is
* specified then the default capacity provider strategy for the cluster is used.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the
* base
and weight
to assign to them. A capacity provider must be associated
* with the cluster to be used in a capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider with a cluster. Only
* capacity providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already
* be created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or
* FARGATE_SPOT
capacity providers. The Fargate capacity providers are available to all
* accounts and only need to be associated with a cluster to be used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder defaultCapacityProviderStrategy(Collection defaultCapacityProviderStrategy);
/**
*
* The capacity provider strategy to use by default for the cluster.
*
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is specified
* then the default capacity provider strategy for the cluster is used.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the base
and
* weight
to assign to them. A capacity provider must be associated with the cluster to be used in
* a capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider with a cluster. Only capacity
* providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be
* created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
* capacity providers. The Fargate capacity providers are available to all accounts and only need to be
* associated with a cluster to be used.
*
*
* @param defaultCapacityProviderStrategy
* The capacity provider strategy to use by default for the cluster.
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is
* specified then the default capacity provider strategy for the cluster is used.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the
* base
and weight
to assign to them. A capacity provider must be associated
* with the cluster to be used in a capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider with a cluster. Only
* capacity providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already
* be created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or
* FARGATE_SPOT
capacity providers. The Fargate capacity providers are available to all
* accounts and only need to be associated with a cluster to be used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder defaultCapacityProviderStrategy(CapacityProviderStrategyItem... defaultCapacityProviderStrategy);
/**
*
* The capacity provider strategy to use by default for the cluster.
*
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is specified
* then the default capacity provider strategy for the cluster is used.
*
*
* A capacity provider strategy consists of one or more capacity providers along with the base
and
* weight
to assign to them. A capacity provider must be associated with the cluster to be used in
* a capacity provider strategy. The PutClusterCapacityProviders API is used to associate a capacity provider with a cluster. Only capacity
* providers with an ACTIVE
or UPDATING
status can be used.
*
*
* If specifying a capacity provider that uses an Auto Scaling group, the capacity provider must already be
* created. New capacity providers can be created with the CreateCapacityProvider API operation.
*
*
* To use a Fargate capacity provider, specify either the FARGATE
or FARGATE_SPOT
* capacity providers. The Fargate capacity providers are available to all accounts and only need to be
* associated with a cluster to be used.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem.Builder} avoiding the need to
* create one manually via
* {@link software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem.Builder#build()} is called
* immediately and its result is passed to {@link
* #defaultCapacityProviderStrategy(List)}.
*
* @param defaultCapacityProviderStrategy
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.ecs.model.CapacityProviderStrategyItem.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #defaultCapacityProviderStrategy(java.util.Collection)
*/
Builder defaultCapacityProviderStrategy(Consumer... defaultCapacityProviderStrategy);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends EcsRequest.BuilderImpl implements Builder {
private String cluster;
private List capacityProviders = DefaultSdkAutoConstructList.getInstance();
private List defaultCapacityProviderStrategy = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(PutClusterCapacityProvidersRequest model) {
super(model);
cluster(model.cluster);
capacityProviders(model.capacityProviders);
defaultCapacityProviderStrategy(model.defaultCapacityProviderStrategy);
}
public final String getCluster() {
return cluster;
}
public final void setCluster(String cluster) {
this.cluster = cluster;
}
@Override
public final Builder cluster(String cluster) {
this.cluster = cluster;
return this;
}
public final Collection getCapacityProviders() {
if (capacityProviders instanceof SdkAutoConstructList) {
return null;
}
return capacityProviders;
}
public final void setCapacityProviders(Collection capacityProviders) {
this.capacityProviders = StringListCopier.copy(capacityProviders);
}
@Override
public final Builder capacityProviders(Collection capacityProviders) {
this.capacityProviders = StringListCopier.copy(capacityProviders);
return this;
}
@Override
@SafeVarargs
public final Builder capacityProviders(String... capacityProviders) {
capacityProviders(Arrays.asList(capacityProviders));
return this;
}
public final List getDefaultCapacityProviderStrategy() {
List result = CapacityProviderStrategyCopier
.copyToBuilder(this.defaultCapacityProviderStrategy);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setDefaultCapacityProviderStrategy(
Collection defaultCapacityProviderStrategy) {
this.defaultCapacityProviderStrategy = CapacityProviderStrategyCopier
.copyFromBuilder(defaultCapacityProviderStrategy);
}
@Override
public final Builder defaultCapacityProviderStrategy(
Collection defaultCapacityProviderStrategy) {
this.defaultCapacityProviderStrategy = CapacityProviderStrategyCopier.copy(defaultCapacityProviderStrategy);
return this;
}
@Override
@SafeVarargs
public final Builder defaultCapacityProviderStrategy(CapacityProviderStrategyItem... defaultCapacityProviderStrategy) {
defaultCapacityProviderStrategy(Arrays.asList(defaultCapacityProviderStrategy));
return this;
}
@Override
@SafeVarargs
public final Builder defaultCapacityProviderStrategy(
Consumer... defaultCapacityProviderStrategy) {
defaultCapacityProviderStrategy(Stream.of(defaultCapacityProviderStrategy)
.map(c -> CapacityProviderStrategyItem.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public PutClusterCapacityProvidersRequest build() {
return new PutClusterCapacityProvidersRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}