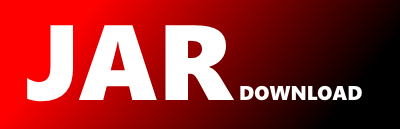
software.amazon.awssdk.services.ecs.model.ContainerImage Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details about the container image a service revision uses.
*
*
* To ensure that all tasks in a service use the same container image, Amazon ECS resolves container image names and any
* image tags specified in the task definition to container image digests.
*
*
* After the container image digest has been established, Amazon ECS uses the digest to start any other desired tasks,
* and for any future service and service revision updates. This leads to all tasks in a service always running
* identical container images, resulting in version consistency for your software. For more information, see Container image resolution in the Amazon ECS Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ContainerImage implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CONTAINER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerName").getter(getter(ContainerImage::containerName)).setter(setter(Builder::containerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerName").build()).build();
private static final SdkField IMAGE_DIGEST_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("imageDigest").getter(getter(ContainerImage::imageDigest)).setter(setter(Builder::imageDigest))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("imageDigest").build()).build();
private static final SdkField IMAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("image")
.getter(getter(ContainerImage::image)).setter(setter(Builder::image))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("image").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTAINER_NAME_FIELD,
IMAGE_DIGEST_FIELD, IMAGE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("containerName", CONTAINER_NAME_FIELD);
put("imageDigest", IMAGE_DIGEST_FIELD);
put("image", IMAGE_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String containerName;
private final String imageDigest;
private final String image;
private ContainerImage(BuilderImpl builder) {
this.containerName = builder.containerName;
this.imageDigest = builder.imageDigest;
this.image = builder.image;
}
/**
*
* The name of the container.
*
*
* @return The name of the container.
*/
public final String containerName() {
return containerName;
}
/**
*
* The container image digest.
*
*
* @return The container image digest.
*/
public final String imageDigest() {
return imageDigest;
}
/**
*
* The container image.
*
*
* @return The container image.
*/
public final String image() {
return image;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(containerName());
hashCode = 31 * hashCode + Objects.hashCode(imageDigest());
hashCode = 31 * hashCode + Objects.hashCode(image());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ContainerImage)) {
return false;
}
ContainerImage other = (ContainerImage) obj;
return Objects.equals(containerName(), other.containerName()) && Objects.equals(imageDigest(), other.imageDigest())
&& Objects.equals(image(), other.image());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ContainerImage").add("ContainerName", containerName()).add("ImageDigest", imageDigest())
.add("Image", image()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "containerName":
return Optional.ofNullable(clazz.cast(containerName()));
case "imageDigest":
return Optional.ofNullable(clazz.cast(imageDigest()));
case "image":
return Optional.ofNullable(clazz.cast(image()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function