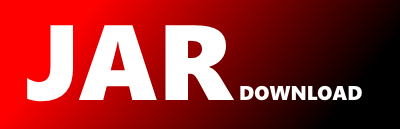
software.amazon.awssdk.services.ecs.model.ContainerInstance Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An Amazon EC2 or External instance that's running the Amazon ECS agent and has been registered with a cluster.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ContainerInstance implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CONTAINER_INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerInstanceArn").getter(getter(ContainerInstance::containerInstanceArn))
.setter(setter(Builder::containerInstanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerInstanceArn").build())
.build();
private static final SdkField EC2_INSTANCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ec2InstanceId").getter(getter(ContainerInstance::ec2InstanceId)).setter(setter(Builder::ec2InstanceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ec2InstanceId").build()).build();
private static final SdkField CAPACITY_PROVIDER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("capacityProviderName").getter(getter(ContainerInstance::capacityProviderName))
.setter(setter(Builder::capacityProviderName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderName").build())
.build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.LONG).memberName("version")
.getter(getter(ContainerInstance::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("version").build()).build();
private static final SdkField VERSION_INFO_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("versionInfo").getter(getter(ContainerInstance::versionInfo)).setter(setter(Builder::versionInfo))
.constructor(VersionInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("versionInfo").build()).build();
private static final SdkField> REMAINING_RESOURCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("remainingResources")
.getter(getter(ContainerInstance::remainingResources))
.setter(setter(Builder::remainingResources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("remainingResources").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Resource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> REGISTERED_RESOURCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("registeredResources")
.getter(getter(ContainerInstance::registeredResources))
.setter(setter(Builder::registeredResources))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registeredResources").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Resource::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(ContainerInstance::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("statusReason").getter(getter(ContainerInstance::statusReason)).setter(setter(Builder::statusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("statusReason").build()).build();
private static final SdkField AGENT_CONNECTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("agentConnected").getter(getter(ContainerInstance::agentConnected))
.setter(setter(Builder::agentConnected))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("agentConnected").build()).build();
private static final SdkField RUNNING_TASKS_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("runningTasksCount").getter(getter(ContainerInstance::runningTasksCount))
.setter(setter(Builder::runningTasksCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("runningTasksCount").build()).build();
private static final SdkField PENDING_TASKS_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("pendingTasksCount").getter(getter(ContainerInstance::pendingTasksCount))
.setter(setter(Builder::pendingTasksCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pendingTasksCount").build()).build();
private static final SdkField AGENT_UPDATE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("agentUpdateStatus").getter(getter(ContainerInstance::agentUpdateStatusAsString))
.setter(setter(Builder::agentUpdateStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("agentUpdateStatus").build()).build();
private static final SdkField> ATTRIBUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("attributes")
.getter(getter(ContainerInstance::attributes))
.setter(setter(Builder::attributes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attributes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Attribute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField REGISTERED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("registeredAt").getter(getter(ContainerInstance::registeredAt)).setter(setter(Builder::registeredAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("registeredAt").build()).build();
private static final SdkField> ATTACHMENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("attachments")
.getter(getter(ContainerInstance::attachments))
.setter(setter(Builder::attachments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("attachments").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Attachment::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("tags")
.getter(getter(ContainerInstance::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField HEALTH_STATUS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("healthStatus")
.getter(getter(ContainerInstance::healthStatus)).setter(setter(Builder::healthStatus))
.constructor(ContainerInstanceHealthStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("healthStatus").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTAINER_INSTANCE_ARN_FIELD,
EC2_INSTANCE_ID_FIELD, CAPACITY_PROVIDER_NAME_FIELD, VERSION_FIELD, VERSION_INFO_FIELD, REMAINING_RESOURCES_FIELD,
REGISTERED_RESOURCES_FIELD, STATUS_FIELD, STATUS_REASON_FIELD, AGENT_CONNECTED_FIELD, RUNNING_TASKS_COUNT_FIELD,
PENDING_TASKS_COUNT_FIELD, AGENT_UPDATE_STATUS_FIELD, ATTRIBUTES_FIELD, REGISTERED_AT_FIELD, ATTACHMENTS_FIELD,
TAGS_FIELD, HEALTH_STATUS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("containerInstanceArn", CONTAINER_INSTANCE_ARN_FIELD);
put("ec2InstanceId", EC2_INSTANCE_ID_FIELD);
put("capacityProviderName", CAPACITY_PROVIDER_NAME_FIELD);
put("version", VERSION_FIELD);
put("versionInfo", VERSION_INFO_FIELD);
put("remainingResources", REMAINING_RESOURCES_FIELD);
put("registeredResources", REGISTERED_RESOURCES_FIELD);
put("status", STATUS_FIELD);
put("statusReason", STATUS_REASON_FIELD);
put("agentConnected", AGENT_CONNECTED_FIELD);
put("runningTasksCount", RUNNING_TASKS_COUNT_FIELD);
put("pendingTasksCount", PENDING_TASKS_COUNT_FIELD);
put("agentUpdateStatus", AGENT_UPDATE_STATUS_FIELD);
put("attributes", ATTRIBUTES_FIELD);
put("registeredAt", REGISTERED_AT_FIELD);
put("attachments", ATTACHMENTS_FIELD);
put("tags", TAGS_FIELD);
put("healthStatus", HEALTH_STATUS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String containerInstanceArn;
private final String ec2InstanceId;
private final String capacityProviderName;
private final Long version;
private final VersionInfo versionInfo;
private final List remainingResources;
private final List registeredResources;
private final String status;
private final String statusReason;
private final Boolean agentConnected;
private final Integer runningTasksCount;
private final Integer pendingTasksCount;
private final String agentUpdateStatus;
private final List attributes;
private final Instant registeredAt;
private final List attachments;
private final List tags;
private final ContainerInstanceHealthStatus healthStatus;
private ContainerInstance(BuilderImpl builder) {
this.containerInstanceArn = builder.containerInstanceArn;
this.ec2InstanceId = builder.ec2InstanceId;
this.capacityProviderName = builder.capacityProviderName;
this.version = builder.version;
this.versionInfo = builder.versionInfo;
this.remainingResources = builder.remainingResources;
this.registeredResources = builder.registeredResources;
this.status = builder.status;
this.statusReason = builder.statusReason;
this.agentConnected = builder.agentConnected;
this.runningTasksCount = builder.runningTasksCount;
this.pendingTasksCount = builder.pendingTasksCount;
this.agentUpdateStatus = builder.agentUpdateStatus;
this.attributes = builder.attributes;
this.registeredAt = builder.registeredAt;
this.attachments = builder.attachments;
this.tags = builder.tags;
this.healthStatus = builder.healthStatus;
}
/**
*
* The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see
* Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*/
public final String containerInstanceArn() {
return containerInstanceArn;
}
/**
*
* The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
*
*
* @return The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
*/
public final String ec2InstanceId() {
return ec2InstanceId;
}
/**
*
* The capacity provider that's associated with the container instance.
*
*
* @return The capacity provider that's associated with the container instance.
*/
public final String capacityProviderName() {
return capacityProviderName;
}
/**
*
* The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS container
* instance state with CloudWatch Events, you can compare the version of a container instance reported by the Amazon
* ECS APIs with the version reported in CloudWatch Events for the container instance (inside the
* detail
object) to verify that the version in your event stream is current.
*
*
* @return The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS
* container instance state with CloudWatch Events, you can compare the version of a container instance
* reported by the Amazon ECS APIs with the version reported in CloudWatch Events for the container instance
* (inside the detail
object) to verify that the version in your event stream is current.
*/
public final Long version() {
return version;
}
/**
*
* The version information for the Amazon ECS container agent and Docker daemon running on the container instance.
*
*
* @return The version information for the Amazon ECS container agent and Docker daemon running on the container
* instance.
*/
public final VersionInfo versionInfo() {
return versionInfo;
}
/**
* For responses, this returns true if the service returned a value for the RemainingResources property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRemainingResources() {
return remainingResources != null && !(remainingResources instanceof SdkAutoConstructList);
}
/**
*
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't already
* allocated to tasks and is therefore available for new tasks. For port resource types, this parameter describes
* the ports that were reserved by the Amazon ECS container agent (at instance registration time) and any task
* containers that have reserved port mappings on the host (with the host
or bridge
* network mode). Any port that's not specified here is available for new tasks.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRemainingResources} method.
*
*
* @return For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't
* already allocated to tasks and is therefore available for new tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent (at instance
* registration time) and any task containers that have reserved port mappings on the host (with the
* host
or bridge
network mode). Any port that's not specified here is available
* for new tasks.
*/
public final List remainingResources() {
return remainingResources;
}
/**
* For responses, this returns true if the service returned a value for the RegisteredResources property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasRegisteredResources() {
return registeredResources != null && !(registeredResources instanceof SdkAutoConstructList);
}
/**
*
* For CPU and memory resource types, this parameter describes the amount of each resource that was available on the
* container instance when the container agent registered it with Amazon ECS. This value represents the total amount
* of CPU and memory that can be allocated on this container instance to tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent when it registered the
* container instance with Amazon ECS.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasRegisteredResources} method.
*
*
* @return For CPU and memory resource types, this parameter describes the amount of each resource that was
* available on the container instance when the container agent registered it with Amazon ECS. This value
* represents the total amount of CPU and memory that can be allocated on this container instance to tasks.
* For port resource types, this parameter describes the ports that were reserved by the Amazon ECS
* container agent when it registered the container instance with Amazon ECS.
*/
public final List registeredResources() {
return registeredResources;
}
/**
*
* The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
, or
* DRAINING
.
*
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly registered
* container instance will transition to a REGISTERING
status while the trunk elastic network interface
* is provisioned for the instance. If the registration fails, the instance will transition to a
* REGISTRATION_FAILED
status. You can describe the container instance and see the reason for failure
* in the statusReason
parameter. Once the container instance is terminated, the instance transitions
* to a DEREGISTERING
status while the trunk elastic network interface is deprovisioned. The instance
* then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The DRAINING
* indicates that new tasks aren't placed on the container instance and any service tasks running on the container
* instance are removed if possible. For more information, see Container
* instance draining in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
,
* or DRAINING
.
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly
* registered container instance will transition to a REGISTERING
status while the trunk
* elastic network interface is provisioned for the instance. If the registration fails, the instance will
* transition to a REGISTRATION_FAILED
status. You can describe the container instance and see
* the reason for failure in the statusReason
parameter. Once the container instance is
* terminated, the instance transitions to a DEREGISTERING
status while the trunk elastic
* network interface is deprovisioned. The instance then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The
* DRAINING
indicates that new tasks aren't placed on the container instance and any service
* tasks running on the container instance are removed if possible. For more information, see Container instance draining in the Amazon Elastic Container Service Developer Guide.
*/
public final String status() {
return status;
}
/**
*
* The reason that the container instance reached its current status.
*
*
* @return The reason that the container instance reached its current status.
*/
public final String statusReason() {
return statusReason;
}
/**
*
* This parameter returns true
if the agent is connected to Amazon ECS. An instance with an agent that
* may be unhealthy or stopped return false
. Only instances connected to an agent can accept task
* placement requests.
*
*
* @return This parameter returns true
if the agent is connected to Amazon ECS. An instance with an
* agent that may be unhealthy or stopped return false
. Only instances connected to an agent
* can accept task placement requests.
*/
public final Boolean agentConnected() {
return agentConnected;
}
/**
*
* The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
*
*
* @return The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
*/
public final Integer runningTasksCount() {
return runningTasksCount;
}
/**
*
* The number of tasks on the container instance that are in the PENDING
status.
*
*
* @return The number of tasks on the container instance that are in the PENDING
status.
*/
public final Integer pendingTasksCount() {
return pendingTasksCount;
}
/**
*
* The status of the most recent agent update. If an update wasn't ever requested, this value is NULL
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #agentUpdateStatus}
* will return {@link AgentUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #agentUpdateStatusAsString}.
*
*
* @return The status of the most recent agent update. If an update wasn't ever requested, this value is
* NULL
.
* @see AgentUpdateStatus
*/
public final AgentUpdateStatus agentUpdateStatus() {
return AgentUpdateStatus.fromValue(agentUpdateStatus);
}
/**
*
* The status of the most recent agent update. If an update wasn't ever requested, this value is NULL
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #agentUpdateStatus}
* will return {@link AgentUpdateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #agentUpdateStatusAsString}.
*
*
* @return The status of the most recent agent update. If an update wasn't ever requested, this value is
* NULL
.
* @see AgentUpdateStatus
*/
public final String agentUpdateStatusAsString() {
return agentUpdateStatus;
}
/**
* For responses, this returns true if the service returned a value for the Attributes property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasAttributes() {
return attributes != null && !(attributes instanceof SdkAutoConstructList);
}
/**
*
* The attributes set for the container instance, either by the Amazon ECS container agent at instance registration
* or manually with the PutAttributes
* operation.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttributes} method.
*
*
* @return The attributes set for the container instance, either by the Amazon ECS container agent at instance
* registration or manually with the PutAttributes
* operation.
*/
public final List attributes() {
return attributes;
}
/**
*
* The Unix timestamp for the time when the container instance was registered.
*
*
* @return The Unix timestamp for the time when the container instance was registered.
*/
public final Instant registeredAt() {
return registeredAt;
}
/**
* For responses, this returns true if the service returned a value for the Attachments property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAttachments() {
return attachments != null && !(attachments instanceof SdkAutoConstructList);
}
/**
*
* The resources attached to a container instance, such as an elastic network interface.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttachments} method.
*
*
* @return The resources attached to a container instance, such as an elastic network interface.
*/
public final List attachments() {
return attachments;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag consists
* of a key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The metadata that you apply to the container instance to help you categorize and organize them. Each tag
* consists of a key and an optional value. You define both.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete
* tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource
* limit.
*
*
*/
public final List tags() {
return tags;
}
/**
*
* An object representing the health status of the container instance.
*
*
* @return An object representing the health status of the container instance.
*/
public final ContainerInstanceHealthStatus healthStatus() {
return healthStatus;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(containerInstanceArn());
hashCode = 31 * hashCode + Objects.hashCode(ec2InstanceId());
hashCode = 31 * hashCode + Objects.hashCode(capacityProviderName());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(versionInfo());
hashCode = 31 * hashCode + Objects.hashCode(hasRemainingResources() ? remainingResources() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasRegisteredResources() ? registeredResources() : null);
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(statusReason());
hashCode = 31 * hashCode + Objects.hashCode(agentConnected());
hashCode = 31 * hashCode + Objects.hashCode(runningTasksCount());
hashCode = 31 * hashCode + Objects.hashCode(pendingTasksCount());
hashCode = 31 * hashCode + Objects.hashCode(agentUpdateStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasAttributes() ? attributes() : null);
hashCode = 31 * hashCode + Objects.hashCode(registeredAt());
hashCode = 31 * hashCode + Objects.hashCode(hasAttachments() ? attachments() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(healthStatus());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ContainerInstance)) {
return false;
}
ContainerInstance other = (ContainerInstance) obj;
return Objects.equals(containerInstanceArn(), other.containerInstanceArn())
&& Objects.equals(ec2InstanceId(), other.ec2InstanceId())
&& Objects.equals(capacityProviderName(), other.capacityProviderName())
&& Objects.equals(version(), other.version()) && Objects.equals(versionInfo(), other.versionInfo())
&& hasRemainingResources() == other.hasRemainingResources()
&& Objects.equals(remainingResources(), other.remainingResources())
&& hasRegisteredResources() == other.hasRegisteredResources()
&& Objects.equals(registeredResources(), other.registeredResources()) && Objects.equals(status(), other.status())
&& Objects.equals(statusReason(), other.statusReason())
&& Objects.equals(agentConnected(), other.agentConnected())
&& Objects.equals(runningTasksCount(), other.runningTasksCount())
&& Objects.equals(pendingTasksCount(), other.pendingTasksCount())
&& Objects.equals(agentUpdateStatusAsString(), other.agentUpdateStatusAsString())
&& hasAttributes() == other.hasAttributes() && Objects.equals(attributes(), other.attributes())
&& Objects.equals(registeredAt(), other.registeredAt()) && hasAttachments() == other.hasAttachments()
&& Objects.equals(attachments(), other.attachments()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(healthStatus(), other.healthStatus());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ContainerInstance").add("ContainerInstanceArn", containerInstanceArn())
.add("Ec2InstanceId", ec2InstanceId()).add("CapacityProviderName", capacityProviderName())
.add("Version", version()).add("VersionInfo", versionInfo())
.add("RemainingResources", hasRemainingResources() ? remainingResources() : null)
.add("RegisteredResources", hasRegisteredResources() ? registeredResources() : null).add("Status", status())
.add("StatusReason", statusReason()).add("AgentConnected", agentConnected())
.add("RunningTasksCount", runningTasksCount()).add("PendingTasksCount", pendingTasksCount())
.add("AgentUpdateStatus", agentUpdateStatusAsString()).add("Attributes", hasAttributes() ? attributes() : null)
.add("RegisteredAt", registeredAt()).add("Attachments", hasAttachments() ? attachments() : null)
.add("Tags", hasTags() ? tags() : null).add("HealthStatus", healthStatus()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "containerInstanceArn":
return Optional.ofNullable(clazz.cast(containerInstanceArn()));
case "ec2InstanceId":
return Optional.ofNullable(clazz.cast(ec2InstanceId()));
case "capacityProviderName":
return Optional.ofNullable(clazz.cast(capacityProviderName()));
case "version":
return Optional.ofNullable(clazz.cast(version()));
case "versionInfo":
return Optional.ofNullable(clazz.cast(versionInfo()));
case "remainingResources":
return Optional.ofNullable(clazz.cast(remainingResources()));
case "registeredResources":
return Optional.ofNullable(clazz.cast(registeredResources()));
case "status":
return Optional.ofNullable(clazz.cast(status()));
case "statusReason":
return Optional.ofNullable(clazz.cast(statusReason()));
case "agentConnected":
return Optional.ofNullable(clazz.cast(agentConnected()));
case "runningTasksCount":
return Optional.ofNullable(clazz.cast(runningTasksCount()));
case "pendingTasksCount":
return Optional.ofNullable(clazz.cast(pendingTasksCount()));
case "agentUpdateStatus":
return Optional.ofNullable(clazz.cast(agentUpdateStatusAsString()));
case "attributes":
return Optional.ofNullable(clazz.cast(attributes()));
case "registeredAt":
return Optional.ofNullable(clazz.cast(registeredAt()));
case "attachments":
return Optional.ofNullable(clazz.cast(attachments()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "healthStatus":
return Optional.ofNullable(clazz.cast(healthStatus()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function