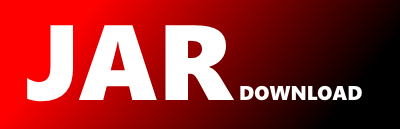
software.amazon.awssdk.services.ecs.model.ServiceRevision Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about the service revision.
*
*
* A service revision contains a record of the workload configuration Amazon ECS is attempting to deploy. Whenever you
* create or deploy a service, Amazon ECS automatically creates and captures the configuration that you're trying to
* deploy in the service revision. For information about service revisions, see Amazon ECS service
* revisions in the Amazon Elastic Container Service Developer Guide .
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServiceRevision implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField SERVICE_REVISION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceRevisionArn").getter(getter(ServiceRevision::serviceRevisionArn))
.setter(setter(Builder::serviceRevisionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRevisionArn").build())
.build();
private static final SdkField SERVICE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("serviceArn").getter(getter(ServiceRevision::serviceArn)).setter(setter(Builder::serviceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceArn").build()).build();
private static final SdkField CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("clusterArn").getter(getter(ServiceRevision::clusterArn)).setter(setter(Builder::clusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clusterArn").build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskDefinition").getter(getter(ServiceRevision::taskDefinition)).setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final SdkField> CAPACITY_PROVIDER_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("capacityProviderStrategy")
.getter(getter(ServiceRevision::capacityProviderStrategy))
.setter(setter(Builder::capacityProviderStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capacityProviderStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CapacityProviderStrategyItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("launchType").getter(getter(ServiceRevision::launchTypeAsString)).setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformVersion").getter(getter(ServiceRevision::platformVersion))
.setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField PLATFORM_FAMILY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformFamily").getter(getter(ServiceRevision::platformFamily)).setter(setter(Builder::platformFamily))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformFamily").build()).build();
private static final SdkField> LOAD_BALANCERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("loadBalancers")
.getter(getter(ServiceRevision::loadBalancers))
.setter(setter(Builder::loadBalancers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("loadBalancers").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(LoadBalancer::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SERVICE_REGISTRIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("serviceRegistries")
.getter(getter(ServiceRevision::serviceRegistries))
.setter(setter(Builder::serviceRegistries))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceRegistries").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceRegistry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("networkConfiguration")
.getter(getter(ServiceRevision::networkConfiguration)).setter(setter(Builder::networkConfiguration))
.constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField> CONTAINER_IMAGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("containerImages")
.getter(getter(ServiceRevision::containerImages))
.setter(setter(Builder::containerImages))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerImages").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ContainerImage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField GUARD_DUTY_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("guardDutyEnabled").getter(getter(ServiceRevision::guardDutyEnabled))
.setter(setter(Builder::guardDutyEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("guardDutyEnabled").build()).build();
private static final SdkField SERVICE_CONNECT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("serviceConnectConfiguration")
.getter(getter(ServiceRevision::serviceConnectConfiguration))
.setter(setter(Builder::serviceConnectConfiguration))
.constructor(ServiceConnectConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serviceConnectConfiguration")
.build()).build();
private static final SdkField> VOLUME_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("volumeConfigurations")
.getter(getter(ServiceRevision::volumeConfigurations))
.setter(setter(Builder::volumeConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("volumeConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ServiceVolumeConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FARGATE_EPHEMERAL_STORAGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("fargateEphemeralStorage")
.getter(getter(ServiceRevision::fargateEphemeralStorage)).setter(setter(Builder::fargateEphemeralStorage))
.constructor(DeploymentEphemeralStorage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fargateEphemeralStorage").build())
.build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdAt").getter(getter(ServiceRevision::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdAt").build()).build();
private static final SdkField> VPC_LATTICE_CONFIGURATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("vpcLatticeConfigurations")
.getter(getter(ServiceRevision::vpcLatticeConfigurations))
.setter(setter(Builder::vpcLatticeConfigurations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vpcLatticeConfigurations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(VpcLatticeConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SERVICE_REVISION_ARN_FIELD,
SERVICE_ARN_FIELD, CLUSTER_ARN_FIELD, TASK_DEFINITION_FIELD, CAPACITY_PROVIDER_STRATEGY_FIELD, LAUNCH_TYPE_FIELD,
PLATFORM_VERSION_FIELD, PLATFORM_FAMILY_FIELD, LOAD_BALANCERS_FIELD, SERVICE_REGISTRIES_FIELD,
NETWORK_CONFIGURATION_FIELD, CONTAINER_IMAGES_FIELD, GUARD_DUTY_ENABLED_FIELD, SERVICE_CONNECT_CONFIGURATION_FIELD,
VOLUME_CONFIGURATIONS_FIELD, FARGATE_EPHEMERAL_STORAGE_FIELD, CREATED_AT_FIELD, VPC_LATTICE_CONFIGURATIONS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("serviceRevisionArn", SERVICE_REVISION_ARN_FIELD);
put("serviceArn", SERVICE_ARN_FIELD);
put("clusterArn", CLUSTER_ARN_FIELD);
put("taskDefinition", TASK_DEFINITION_FIELD);
put("capacityProviderStrategy", CAPACITY_PROVIDER_STRATEGY_FIELD);
put("launchType", LAUNCH_TYPE_FIELD);
put("platformVersion", PLATFORM_VERSION_FIELD);
put("platformFamily", PLATFORM_FAMILY_FIELD);
put("loadBalancers", LOAD_BALANCERS_FIELD);
put("serviceRegistries", SERVICE_REGISTRIES_FIELD);
put("networkConfiguration", NETWORK_CONFIGURATION_FIELD);
put("containerImages", CONTAINER_IMAGES_FIELD);
put("guardDutyEnabled", GUARD_DUTY_ENABLED_FIELD);
put("serviceConnectConfiguration", SERVICE_CONNECT_CONFIGURATION_FIELD);
put("volumeConfigurations", VOLUME_CONFIGURATIONS_FIELD);
put("fargateEphemeralStorage", FARGATE_EPHEMERAL_STORAGE_FIELD);
put("createdAt", CREATED_AT_FIELD);
put("vpcLatticeConfigurations", VPC_LATTICE_CONFIGURATIONS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String serviceRevisionArn;
private final String serviceArn;
private final String clusterArn;
private final String taskDefinition;
private final List capacityProviderStrategy;
private final String launchType;
private final String platformVersion;
private final String platformFamily;
private final List loadBalancers;
private final List serviceRegistries;
private final NetworkConfiguration networkConfiguration;
private final List containerImages;
private final Boolean guardDutyEnabled;
private final ServiceConnectConfiguration serviceConnectConfiguration;
private final List volumeConfigurations;
private final DeploymentEphemeralStorage fargateEphemeralStorage;
private final Instant createdAt;
private final List vpcLatticeConfigurations;
private ServiceRevision(BuilderImpl builder) {
this.serviceRevisionArn = builder.serviceRevisionArn;
this.serviceArn = builder.serviceArn;
this.clusterArn = builder.clusterArn;
this.taskDefinition = builder.taskDefinition;
this.capacityProviderStrategy = builder.capacityProviderStrategy;
this.launchType = builder.launchType;
this.platformVersion = builder.platformVersion;
this.platformFamily = builder.platformFamily;
this.loadBalancers = builder.loadBalancers;
this.serviceRegistries = builder.serviceRegistries;
this.networkConfiguration = builder.networkConfiguration;
this.containerImages = builder.containerImages;
this.guardDutyEnabled = builder.guardDutyEnabled;
this.serviceConnectConfiguration = builder.serviceConnectConfiguration;
this.volumeConfigurations = builder.volumeConfigurations;
this.fargateEphemeralStorage = builder.fargateEphemeralStorage;
this.createdAt = builder.createdAt;
this.vpcLatticeConfigurations = builder.vpcLatticeConfigurations;
}
/**
*
* The ARN of the service revision.
*
*
* @return The ARN of the service revision.
*/
public final String serviceRevisionArn() {
return serviceRevisionArn;
}
/**
*
* The ARN of the service for the service revision.
*
*
* @return The ARN of the service for the service revision.
*/
public final String serviceArn() {
return serviceArn;
}
/**
*
* The ARN of the cluster that hosts the service.
*
*
* @return The ARN of the cluster that hosts the service.
*/
public final String clusterArn() {
return clusterArn;
}
/**
*
* The task definition the service revision uses.
*
*
* @return The task definition the service revision uses.
*/
public final String taskDefinition() {
return taskDefinition;
}
/**
* For responses, this returns true if the service returned a value for the CapacityProviderStrategy property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasCapacityProviderStrategy() {
return capacityProviderStrategy != null && !(capacityProviderStrategy instanceof SdkAutoConstructList);
}
/**
*
* The capacity provider strategy the service revision uses.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCapacityProviderStrategy} method.
*
*
* @return The capacity provider strategy the service revision uses.
*/
public final List capacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* The launch type the service revision uses.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type the service revision uses.
* @see LaunchType
*/
public final LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The launch type the service revision uses.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type the service revision uses.
* @see LaunchType
*/
public final String launchTypeAsString() {
return launchType;
}
/**
*
* For the Fargate launch type, the platform version the service revision uses.
*
*
* @return For the Fargate launch type, the platform version the service revision uses.
*/
public final String platformVersion() {
return platformVersion;
}
/**
*
* The platform family the service revision uses.
*
*
* @return The platform family the service revision uses.
*/
public final String platformFamily() {
return platformFamily;
}
/**
* For responses, this returns true if the service returned a value for the LoadBalancers property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLoadBalancers() {
return loadBalancers != null && !(loadBalancers instanceof SdkAutoConstructList);
}
/**
*
* The load balancers the service revision uses.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLoadBalancers} method.
*
*
* @return The load balancers the service revision uses.
*/
public final List loadBalancers() {
return loadBalancers;
}
/**
* For responses, this returns true if the service returned a value for the ServiceRegistries property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasServiceRegistries() {
return serviceRegistries != null && !(serviceRegistries instanceof SdkAutoConstructList);
}
/**
*
* The service registries (for Service Discovery) the service revision uses.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasServiceRegistries} method.
*
*
* @return The service registries (for Service Discovery) the service revision uses.
*/
public final List serviceRegistries() {
return serviceRegistries;
}
/**
* Returns the value of the NetworkConfiguration property for this object.
*
* @return The value of the NetworkConfiguration property for this object.
*/
public final NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the ContainerImages property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasContainerImages() {
return containerImages != null && !(containerImages instanceof SdkAutoConstructList);
}
/**
*
* The container images the service revision uses.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasContainerImages} method.
*
*
* @return The container images the service revision uses.
*/
public final List containerImages() {
return containerImages;
}
/**
*
* Indicates whether Runtime Monitoring is turned on.
*
*
* @return Indicates whether Runtime Monitoring is turned on.
*/
public final Boolean guardDutyEnabled() {
return guardDutyEnabled;
}
/**
* Returns the value of the ServiceConnectConfiguration property for this object.
*
* @return The value of the ServiceConnectConfiguration property for this object.
*/
public final ServiceConnectConfiguration serviceConnectConfiguration() {
return serviceConnectConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the VolumeConfigurations property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVolumeConfigurations() {
return volumeConfigurations != null && !(volumeConfigurations instanceof SdkAutoConstructList);
}
/**
*
* The volumes that are configured at deployment that the service revision uses.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVolumeConfigurations} method.
*
*
* @return The volumes that are configured at deployment that the service revision uses.
*/
public final List volumeConfigurations() {
return volumeConfigurations;
}
/**
* Returns the value of the FargateEphemeralStorage property for this object.
*
* @return The value of the FargateEphemeralStorage property for this object.
*/
public final DeploymentEphemeralStorage fargateEphemeralStorage() {
return fargateEphemeralStorage;
}
/**
*
* The time that the service revision was created. The format is yyyy-mm-dd HH:mm:ss.SSSSS.
*
*
* @return The time that the service revision was created. The format is yyyy-mm-dd HH:mm:ss.SSSSS.
*/
public final Instant createdAt() {
return createdAt;
}
/**
* For responses, this returns true if the service returned a value for the VpcLatticeConfigurations property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasVpcLatticeConfigurations() {
return vpcLatticeConfigurations != null && !(vpcLatticeConfigurations instanceof SdkAutoConstructList);
}
/**
*
* The VPC Lattice configuration for the service revision.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVpcLatticeConfigurations} method.
*
*
* @return The VPC Lattice configuration for the service revision.
*/
public final List vpcLatticeConfigurations() {
return vpcLatticeConfigurations;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(serviceRevisionArn());
hashCode = 31 * hashCode + Objects.hashCode(serviceArn());
hashCode = 31 * hashCode + Objects.hashCode(clusterArn());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
hashCode = 31 * hashCode + Objects.hashCode(hasCapacityProviderStrategy() ? capacityProviderStrategy() : null);
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(platformFamily());
hashCode = 31 * hashCode + Objects.hashCode(hasLoadBalancers() ? loadBalancers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasServiceRegistries() ? serviceRegistries() : null);
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasContainerImages() ? containerImages() : null);
hashCode = 31 * hashCode + Objects.hashCode(guardDutyEnabled());
hashCode = 31 * hashCode + Objects.hashCode(serviceConnectConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasVolumeConfigurations() ? volumeConfigurations() : null);
hashCode = 31 * hashCode + Objects.hashCode(fargateEphemeralStorage());
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(hasVpcLatticeConfigurations() ? vpcLatticeConfigurations() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceRevision)) {
return false;
}
ServiceRevision other = (ServiceRevision) obj;
return Objects.equals(serviceRevisionArn(), other.serviceRevisionArn())
&& Objects.equals(serviceArn(), other.serviceArn()) && Objects.equals(clusterArn(), other.clusterArn())
&& Objects.equals(taskDefinition(), other.taskDefinition())
&& hasCapacityProviderStrategy() == other.hasCapacityProviderStrategy()
&& Objects.equals(capacityProviderStrategy(), other.capacityProviderStrategy())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString())
&& Objects.equals(platformVersion(), other.platformVersion())
&& Objects.equals(platformFamily(), other.platformFamily()) && hasLoadBalancers() == other.hasLoadBalancers()
&& Objects.equals(loadBalancers(), other.loadBalancers())
&& hasServiceRegistries() == other.hasServiceRegistries()
&& Objects.equals(serviceRegistries(), other.serviceRegistries())
&& Objects.equals(networkConfiguration(), other.networkConfiguration())
&& hasContainerImages() == other.hasContainerImages()
&& Objects.equals(containerImages(), other.containerImages())
&& Objects.equals(guardDutyEnabled(), other.guardDutyEnabled())
&& Objects.equals(serviceConnectConfiguration(), other.serviceConnectConfiguration())
&& hasVolumeConfigurations() == other.hasVolumeConfigurations()
&& Objects.equals(volumeConfigurations(), other.volumeConfigurations())
&& Objects.equals(fargateEphemeralStorage(), other.fargateEphemeralStorage())
&& Objects.equals(createdAt(), other.createdAt())
&& hasVpcLatticeConfigurations() == other.hasVpcLatticeConfigurations()
&& Objects.equals(vpcLatticeConfigurations(), other.vpcLatticeConfigurations());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServiceRevision").add("ServiceRevisionArn", serviceRevisionArn())
.add("ServiceArn", serviceArn()).add("ClusterArn", clusterArn()).add("TaskDefinition", taskDefinition())
.add("CapacityProviderStrategy", hasCapacityProviderStrategy() ? capacityProviderStrategy() : null)
.add("LaunchType", launchTypeAsString()).add("PlatformVersion", platformVersion())
.add("PlatformFamily", platformFamily()).add("LoadBalancers", hasLoadBalancers() ? loadBalancers() : null)
.add("ServiceRegistries", hasServiceRegistries() ? serviceRegistries() : null)
.add("NetworkConfiguration", networkConfiguration())
.add("ContainerImages", hasContainerImages() ? containerImages() : null)
.add("GuardDutyEnabled", guardDutyEnabled()).add("ServiceConnectConfiguration", serviceConnectConfiguration())
.add("VolumeConfigurations", hasVolumeConfigurations() ? volumeConfigurations() : null)
.add("FargateEphemeralStorage", fargateEphemeralStorage()).add("CreatedAt", createdAt())
.add("VpcLatticeConfigurations", hasVpcLatticeConfigurations() ? vpcLatticeConfigurations() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "serviceRevisionArn":
return Optional.ofNullable(clazz.cast(serviceRevisionArn()));
case "serviceArn":
return Optional.ofNullable(clazz.cast(serviceArn()));
case "clusterArn":
return Optional.ofNullable(clazz.cast(clusterArn()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
case "capacityProviderStrategy":
return Optional.ofNullable(clazz.cast(capacityProviderStrategy()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "platformFamily":
return Optional.ofNullable(clazz.cast(platformFamily()));
case "loadBalancers":
return Optional.ofNullable(clazz.cast(loadBalancers()));
case "serviceRegistries":
return Optional.ofNullable(clazz.cast(serviceRegistries()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "containerImages":
return Optional.ofNullable(clazz.cast(containerImages()));
case "guardDutyEnabled":
return Optional.ofNullable(clazz.cast(guardDutyEnabled()));
case "serviceConnectConfiguration":
return Optional.ofNullable(clazz.cast(serviceConnectConfiguration()));
case "volumeConfigurations":
return Optional.ofNullable(clazz.cast(volumeConfigurations()));
case "fargateEphemeralStorage":
return Optional.ofNullable(clazz.cast(fargateEphemeralStorage()));
case "createdAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "vpcLatticeConfigurations":
return Optional.ofNullable(clazz.cast(vpcLatticeConfigurations()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function