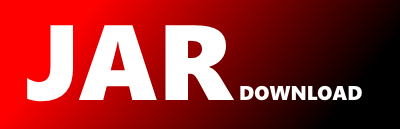
software.amazon.awssdk.services.ecs.model.LoadBalancer Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The load balancer configuration to use with a service or task set.
*
*
* When you add, update, or remove a load balancer configuration, Amazon ECS starts a new deployment with the updated
* Elastic Load Balancing configuration. This causes tasks to register to and deregister from load balancers.
*
*
* We recommend that you verify this on a test environment before you update the Elastic Load Balancing configuration.
*
*
* A service-linked role is required for services that use multiple target groups. For more information, see Using
* service-linked roles in the Amazon Elastic Container Service Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LoadBalancer implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TARGET_GROUP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("targetGroupArn").getter(getter(LoadBalancer::targetGroupArn)).setter(setter(Builder::targetGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetGroupArn").build()).build();
private static final SdkField LOAD_BALANCER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("loadBalancerName").getter(getter(LoadBalancer::loadBalancerName))
.setter(setter(Builder::loadBalancerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("loadBalancerName").build()).build();
private static final SdkField CONTAINER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("containerName").getter(getter(LoadBalancer::containerName)).setter(setter(Builder::containerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerName").build()).build();
private static final SdkField CONTAINER_PORT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("containerPort").getter(getter(LoadBalancer::containerPort)).setter(setter(Builder::containerPort))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerPort").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TARGET_GROUP_ARN_FIELD,
LOAD_BALANCER_NAME_FIELD, CONTAINER_NAME_FIELD, CONTAINER_PORT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String targetGroupArn;
private final String loadBalancerName;
private final String containerName;
private final Integer containerPort;
private LoadBalancer(BuilderImpl builder) {
this.targetGroupArn = builder.targetGroupArn;
this.loadBalancerName = builder.loadBalancerName;
this.containerName = builder.containerName;
this.containerPort = builder.containerPort;
}
/**
*
* The full Amazon Resource Name (ARN) of the Elastic Load Balancing target group or groups associated with a
* service or task set.
*
*
* A target group ARN is only specified when using an Application Load Balancer or Network Load Balancer.
*
*
* For services using the ECS
deployment controller, you can specify one or multiple target groups. For
* more information, see Registering multiple target groups with a service in the Amazon Elastic Container Service Developer
* Guide.
*
*
* For services using the CODE_DEPLOY
deployment controller, you're required to define two target
* groups for the load balancer. For more information, see Blue/green
* deployment with CodeDeploy in the Amazon Elastic Container Service Developer Guide.
*
*
*
* If your service's task definition uses the awsvpc
network mode, you must choose ip
as
* the target type, not instance
. Do this when creating your target groups because tasks that use the
* awsvpc
network mode are associated with an elastic network interface, not an Amazon EC2 instance.
* This network mode is required for the Fargate launch type.
*
*
*
* @return The full Amazon Resource Name (ARN) of the Elastic Load Balancing target group or groups associated with
* a service or task set.
*
* A target group ARN is only specified when using an Application Load Balancer or Network Load Balancer.
*
*
* For services using the ECS
deployment controller, you can specify one or multiple target
* groups. For more information, see Registering multiple target groups with a service in the Amazon Elastic Container Service
* Developer Guide.
*
*
* For services using the CODE_DEPLOY
deployment controller, you're required to define two
* target groups for the load balancer. For more information, see Blue/green deployment with CodeDeploy in the Amazon Elastic Container Service Developer
* Guide.
*
*
*
* If your service's task definition uses the awsvpc
network mode, you must choose
* ip
as the target type, not instance
. Do this when creating your target groups
* because tasks that use the awsvpc
network mode are associated with an elastic network
* interface, not an Amazon EC2 instance. This network mode is required for the Fargate launch type.
*
*/
public final String targetGroupArn() {
return targetGroupArn;
}
/**
*
* The name of the load balancer to associate with the Amazon ECS service or task set.
*
*
* If you are using an Application Load Balancer or a Network Load Balancer the load balancer name parameter should
* be omitted.
*
*
* @return The name of the load balancer to associate with the Amazon ECS service or task set.
*
* If you are using an Application Load Balancer or a Network Load Balancer the load balancer name parameter
* should be omitted.
*/
public final String loadBalancerName() {
return loadBalancerName;
}
/**
*
* The name of the container (as it appears in a container definition) to associate with the load balancer.
*
*
* You need to specify the container name when configuring the target group for an Amazon ECS load balancer.
*
*
* @return The name of the container (as it appears in a container definition) to associate with the load
* balancer.
*
* You need to specify the container name when configuring the target group for an Amazon ECS load balancer.
*/
public final String containerName() {
return containerName;
}
/**
*
* The port on the container to associate with the load balancer. This port must correspond to a
* containerPort
in the task definition the tasks in the service are using. For tasks that use the EC2
* launch type, the container instance they're launched on must allow ingress traffic on the hostPort
* of the port mapping.
*
*
* @return The port on the container to associate with the load balancer. This port must correspond to a
* containerPort
in the task definition the tasks in the service are using. For tasks that use
* the EC2 launch type, the container instance they're launched on must allow ingress traffic on the
* hostPort
of the port mapping.
*/
public final Integer containerPort() {
return containerPort;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(targetGroupArn());
hashCode = 31 * hashCode + Objects.hashCode(loadBalancerName());
hashCode = 31 * hashCode + Objects.hashCode(containerName());
hashCode = 31 * hashCode + Objects.hashCode(containerPort());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LoadBalancer)) {
return false;
}
LoadBalancer other = (LoadBalancer) obj;
return Objects.equals(targetGroupArn(), other.targetGroupArn())
&& Objects.equals(loadBalancerName(), other.loadBalancerName())
&& Objects.equals(containerName(), other.containerName())
&& Objects.equals(containerPort(), other.containerPort());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LoadBalancer").add("TargetGroupArn", targetGroupArn())
.add("LoadBalancerName", loadBalancerName()).add("ContainerName", containerName())
.add("ContainerPort", containerPort()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "targetGroupArn":
return Optional.ofNullable(clazz.cast(targetGroupArn()));
case "loadBalancerName":
return Optional.ofNullable(clazz.cast(loadBalancerName()));
case "containerName":
return Optional.ofNullable(clazz.cast(containerName()));
case "containerPort":
return Optional.ofNullable(clazz.cast(containerPort()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("targetGroupArn", TARGET_GROUP_ARN_FIELD);
map.put("loadBalancerName", LOAD_BALANCER_NAME_FIELD);
map.put("containerName", CONTAINER_NAME_FIELD);
map.put("containerPort", CONTAINER_PORT_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function