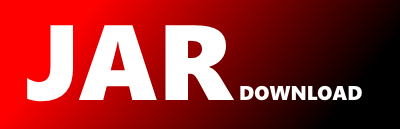
software.amazon.awssdk.services.ecs.model.ContainerDependency Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies. When a
* dependency is defined for container startup, for container shutdown it is reversed.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent to enable container
* dependencies. However, we recommend using the latest container agent version. For information about checking your
* agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you are using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If your
* container instances are launched from version 20190301
or later, then they contain the required versions
* of the container agent and ecs-init
. For more information, see Amazon ECS-optimized Linux
* AMI in the Amazon Elastic Container Service Developer Guide.
*
*
*
* If you are using tasks that use the Fargate launch type, container dependency parameters are not supported.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ContainerDependency implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CONTAINER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ContainerDependency::containerName)).setter(setter(Builder::containerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerName").build()).build();
private static final SdkField CONDITION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ContainerDependency::conditionAsString)).setter(setter(Builder::condition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("condition").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTAINER_NAME_FIELD,
CONDITION_FIELD));
private static final long serialVersionUID = 1L;
private final String containerName;
private final String condition;
private ContainerDependency(BuilderImpl builder) {
this.containerName = builder.containerName;
this.condition = builder.condition;
}
/**
*
* The name of a container.
*
*
* @return The name of a container.
*/
public String containerName() {
return containerName;
}
/**
*
* The dependency condition of the container. The following are the available conditions and their behavior:
*
*
* -
*
* START
- This condition emulates the behavior of links and volumes today. It validates that a
* dependent container is started before permitting other containers to start.
*
*
* -
*
* COMPLETE
- This condition validates that a dependent container runs to completion (exits) before
* permitting other containers to start. This can be useful for nonessential containers that run a script and then
* exit.
*
*
* -
*
* SUCCESS
- This condition is the same as COMPLETE
, but it also requires that the
* container exits with a zero
status.
*
*
* -
*
* HEALTHY
- This condition validates that the dependent container passes its Docker health check
* before permitting other containers to start. This requires that the dependent container has health checks
* configured. This condition is confirmed only at task startup.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #condition} will
* return {@link ContainerCondition#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #conditionAsString}.
*
*
* @return The dependency condition of the container. The following are the available conditions and their
* behavior:
*
* -
*
* START
- This condition emulates the behavior of links and volumes today. It validates that a
* dependent container is started before permitting other containers to start.
*
*
* -
*
* COMPLETE
- This condition validates that a dependent container runs to completion (exits)
* before permitting other containers to start. This can be useful for nonessential containers that run a
* script and then exit.
*
*
* -
*
* SUCCESS
- This condition is the same as COMPLETE
, but it also requires that the
* container exits with a zero
status.
*
*
* -
*
* HEALTHY
- This condition validates that the dependent container passes its Docker health
* check before permitting other containers to start. This requires that the dependent container has health
* checks configured. This condition is confirmed only at task startup.
*
*
* @see ContainerCondition
*/
public ContainerCondition condition() {
return ContainerCondition.fromValue(condition);
}
/**
*
* The dependency condition of the container. The following are the available conditions and their behavior:
*
*
* -
*
* START
- This condition emulates the behavior of links and volumes today. It validates that a
* dependent container is started before permitting other containers to start.
*
*
* -
*
* COMPLETE
- This condition validates that a dependent container runs to completion (exits) before
* permitting other containers to start. This can be useful for nonessential containers that run a script and then
* exit.
*
*
* -
*
* SUCCESS
- This condition is the same as COMPLETE
, but it also requires that the
* container exits with a zero
status.
*
*
* -
*
* HEALTHY
- This condition validates that the dependent container passes its Docker health check
* before permitting other containers to start. This requires that the dependent container has health checks
* configured. This condition is confirmed only at task startup.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #condition} will
* return {@link ContainerCondition#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #conditionAsString}.
*
*
* @return The dependency condition of the container. The following are the available conditions and their
* behavior:
*
* -
*
* START
- This condition emulates the behavior of links and volumes today. It validates that a
* dependent container is started before permitting other containers to start.
*
*
* -
*
* COMPLETE
- This condition validates that a dependent container runs to completion (exits)
* before permitting other containers to start. This can be useful for nonessential containers that run a
* script and then exit.
*
*
* -
*
* SUCCESS
- This condition is the same as COMPLETE
, but it also requires that the
* container exits with a zero
status.
*
*
* -
*
* HEALTHY
- This condition validates that the dependent container passes its Docker health
* check before permitting other containers to start. This requires that the dependent container has health
* checks configured. This condition is confirmed only at task startup.
*
*
* @see ContainerCondition
*/
public String conditionAsString() {
return condition;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(containerName());
hashCode = 31 * hashCode + Objects.hashCode(conditionAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ContainerDependency)) {
return false;
}
ContainerDependency other = (ContainerDependency) obj;
return Objects.equals(containerName(), other.containerName())
&& Objects.equals(conditionAsString(), other.conditionAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ContainerDependency").add("ContainerName", containerName())
.add("Condition", conditionAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "containerName":
return Optional.ofNullable(clazz.cast(containerName()));
case "condition":
return Optional.ofNullable(clazz.cast(conditionAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function