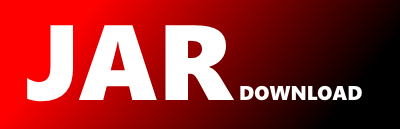
software.amazon.awssdk.services.ecs.model.ContainerOverride Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The overrides that should be sent to a container. An empty container override can be passed in. An example of an
* empty container override would be {"containerOverrides": [ ] }
. If a non-empty container override is
* specified, the name
parameter must be included.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ContainerOverride implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ContainerOverride::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField> COMMAND_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ContainerOverride::command))
.setter(setter(Builder::command))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("command").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> ENVIRONMENT_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ContainerOverride::environment))
.setter(setter(Builder::environment))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("environment").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(KeyValuePair::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CPU_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(ContainerOverride::cpu)).setter(setter(Builder::cpu))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cpu").build()).build();
private static final SdkField MEMORY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(ContainerOverride::memory)).setter(setter(Builder::memory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memory").build()).build();
private static final SdkField MEMORY_RESERVATION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(ContainerOverride::memoryReservation)).setter(setter(Builder::memoryReservation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memoryReservation").build()).build();
private static final SdkField> RESOURCE_REQUIREMENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(ContainerOverride::resourceRequirements))
.setter(setter(Builder::resourceRequirements))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceRequirements").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ResourceRequirement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, COMMAND_FIELD,
ENVIRONMENT_FIELD, CPU_FIELD, MEMORY_FIELD, MEMORY_RESERVATION_FIELD, RESOURCE_REQUIREMENTS_FIELD));
private static final long serialVersionUID = 1L;
private final String name;
private final List command;
private final List environment;
private final Integer cpu;
private final Integer memory;
private final Integer memoryReservation;
private final List resourceRequirements;
private ContainerOverride(BuilderImpl builder) {
this.name = builder.name;
this.command = builder.command;
this.environment = builder.environment;
this.cpu = builder.cpu;
this.memory = builder.memory;
this.memoryReservation = builder.memoryReservation;
this.resourceRequirements = builder.resourceRequirements;
}
/**
*
* The name of the container that receives the override. This parameter is required if any override is specified.
*
*
* @return The name of the container that receives the override. This parameter is required if any override is
* specified.
*/
public String name() {
return name;
}
/**
*
* The command to send to the container that overrides the default command from the Docker image or the task
* definition. You must also specify a container name.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The command to send to the container that overrides the default command from the Docker image or the task
* definition. You must also specify a container name.
*/
public List command() {
return command;
}
/**
*
* The environment variables to send to the container. You can add new environment variables, which are added to the
* container at launch, or you can override the existing environment variables from the Docker image or the task
* definition. You must also specify a container name.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The environment variables to send to the container. You can add new environment variables, which are
* added to the container at launch, or you can override the existing environment variables from the Docker
* image or the task definition. You must also specify a container name.
*/
public List environment() {
return environment;
}
/**
*
* The number of cpu
units reserved for the container, instead of the default value from the task
* definition. You must also specify a container name.
*
*
* @return The number of cpu
units reserved for the container, instead of the default value from the
* task definition. You must also specify a container name.
*/
public Integer cpu() {
return cpu;
}
/**
*
* The hard limit (in MiB) of memory to present to the container, instead of the default value from the task
* definition. If your container attempts to exceed the memory specified here, the container is killed. You must
* also specify a container name.
*
*
* @return The hard limit (in MiB) of memory to present to the container, instead of the default value from the task
* definition. If your container attempts to exceed the memory specified here, the container is killed. You
* must also specify a container name.
*/
public Integer memory() {
return memory;
}
/**
*
* The soft limit (in MiB) of memory to reserve for the container, instead of the default value from the task
* definition. You must also specify a container name.
*
*
* @return The soft limit (in MiB) of memory to reserve for the container, instead of the default value from the
* task definition. You must also specify a container name.
*/
public Integer memoryReservation() {
return memoryReservation;
}
/**
*
* The type and amount of a resource to assign to a container, instead of the default value from the task
* definition. The only supported resource is a GPU.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The type and amount of a resource to assign to a container, instead of the default value from the task
* definition. The only supported resource is a GPU.
*/
public List resourceRequirements() {
return resourceRequirements;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(command());
hashCode = 31 * hashCode + Objects.hashCode(environment());
hashCode = 31 * hashCode + Objects.hashCode(cpu());
hashCode = 31 * hashCode + Objects.hashCode(memory());
hashCode = 31 * hashCode + Objects.hashCode(memoryReservation());
hashCode = 31 * hashCode + Objects.hashCode(resourceRequirements());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ContainerOverride)) {
return false;
}
ContainerOverride other = (ContainerOverride) obj;
return Objects.equals(name(), other.name()) && Objects.equals(command(), other.command())
&& Objects.equals(environment(), other.environment()) && Objects.equals(cpu(), other.cpu())
&& Objects.equals(memory(), other.memory()) && Objects.equals(memoryReservation(), other.memoryReservation())
&& Objects.equals(resourceRequirements(), other.resourceRequirements());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ContainerOverride").add("Name", name()).add("Command", command())
.add("Environment", environment()).add("Cpu", cpu()).add("Memory", memory())
.add("MemoryReservation", memoryReservation()).add("ResourceRequirements", resourceRequirements()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "command":
return Optional.ofNullable(clazz.cast(command()));
case "environment":
return Optional.ofNullable(clazz.cast(environment()));
case "cpu":
return Optional.ofNullable(clazz.cast(cpu()));
case "memory":
return Optional.ofNullable(clazz.cast(memory()));
case "memoryReservation":
return Optional.ofNullable(clazz.cast(memoryReservation()));
case "resourceRequirements":
return Optional.ofNullable(clazz.cast(resourceRequirements()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function