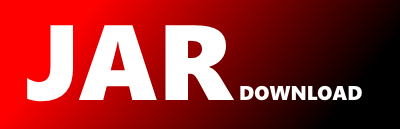
software.amazon.awssdk.services.ecs.model.RunTaskRequest Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class RunTaskRequest extends EcsRequest implements ToCopyableBuilder {
private static final SdkField CLUSTER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RunTaskRequest::cluster)).setter(setter(Builder::cluster))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cluster").build()).build();
private static final SdkField TASK_DEFINITION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RunTaskRequest::taskDefinition)).setter(setter(Builder::taskDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinition").build()).build();
private static final SdkField OVERRIDES_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(RunTaskRequest::overrides)).setter(setter(Builder::overrides)).constructor(TaskOverride::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("overrides").build()).build();
private static final SdkField COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(RunTaskRequest::count)).setter(setter(Builder::count))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("count").build()).build();
private static final SdkField STARTED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RunTaskRequest::startedBy)).setter(setter(Builder::startedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startedBy").build()).build();
private static final SdkField GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RunTaskRequest::group)).setter(setter(Builder::group))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("group").build()).build();
private static final SdkField> PLACEMENT_CONSTRAINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(RunTaskRequest::placementConstraints))
.setter(setter(Builder::placementConstraints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementConstraints").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementConstraint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PLACEMENT_STRATEGY_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(RunTaskRequest::placementStrategy))
.setter(setter(Builder::placementStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementStrategy").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PlacementStrategy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField LAUNCH_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RunTaskRequest::launchTypeAsString)).setter(setter(Builder::launchType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("launchType").build()).build();
private static final SdkField PLATFORM_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RunTaskRequest::platformVersion)).setter(setter(Builder::platformVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("platformVersion").build()).build();
private static final SdkField NETWORK_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(RunTaskRequest::networkConfiguration))
.setter(setter(Builder::networkConfiguration)).constructor(NetworkConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkConfiguration").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(RunTaskRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ENABLE_ECS_MANAGED_TAGS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(RunTaskRequest::enableECSManagedTags)).setter(setter(Builder::enableECSManagedTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enableECSManagedTags").build())
.build();
private static final SdkField PROPAGATE_TAGS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RunTaskRequest::propagateTagsAsString)).setter(setter(Builder::propagateTags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("propagateTags").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_FIELD,
TASK_DEFINITION_FIELD, OVERRIDES_FIELD, COUNT_FIELD, STARTED_BY_FIELD, GROUP_FIELD, PLACEMENT_CONSTRAINTS_FIELD,
PLACEMENT_STRATEGY_FIELD, LAUNCH_TYPE_FIELD, PLATFORM_VERSION_FIELD, NETWORK_CONFIGURATION_FIELD, TAGS_FIELD,
ENABLE_ECS_MANAGED_TAGS_FIELD, PROPAGATE_TAGS_FIELD));
private final String cluster;
private final String taskDefinition;
private final TaskOverride overrides;
private final Integer count;
private final String startedBy;
private final String group;
private final List placementConstraints;
private final List placementStrategy;
private final String launchType;
private final String platformVersion;
private final NetworkConfiguration networkConfiguration;
private final List tags;
private final Boolean enableECSManagedTags;
private final String propagateTags;
private RunTaskRequest(BuilderImpl builder) {
super(builder);
this.cluster = builder.cluster;
this.taskDefinition = builder.taskDefinition;
this.overrides = builder.overrides;
this.count = builder.count;
this.startedBy = builder.startedBy;
this.group = builder.group;
this.placementConstraints = builder.placementConstraints;
this.placementStrategy = builder.placementStrategy;
this.launchType = builder.launchType;
this.platformVersion = builder.platformVersion;
this.networkConfiguration = builder.networkConfiguration;
this.tags = builder.tags;
this.enableECSManagedTags = builder.enableECSManagedTags;
this.propagateTags = builder.propagateTags;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not specify
* a cluster, the default cluster is assumed.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not
* specify a cluster, the default cluster is assumed.
*/
public String cluster() {
return cluster;
}
/**
*
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision is used.
*
*
* @return The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision
* is used.
*/
public String taskDefinition() {
return taskDefinition;
}
/**
*
* A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a command
override. You can also override
* existing environment variables (that are specified in the task definition or Docker image) on a container or add
* new environment variables to it with an environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters of the
* override structure.
*
*
*
* @return A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container
* (that is specified in the task definition or Docker image) with a command
override. You can
* also override existing environment variables (that are specified in the task definition or Docker image)
* on a container or add new environment variables to it with an environment
override.
*
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters
* of the override structure.
*
*/
public TaskOverride overrides() {
return overrides;
}
/**
*
* The number of instantiations of the specified task to place on your cluster. You can specify up to 10 tasks per
* call.
*
*
* @return The number of instantiations of the specified task to place on your cluster. You can specify up to 10
* tasks per call.
*/
public Integer count() {
return count;
}
/**
*
* An optional tag specified when a task is started. For example, if you automatically trigger a task to run a batch
* process job, you could apply a unique identifier for that job to your task with the startedBy
* parameter. You can then identify which tasks belong to that job by filtering the results of a ListTasks
* call with the startedBy
value. Up to 36 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed.
*
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the deployment
* ID of the service that starts it.
*
*
* @return An optional tag specified when a task is started. For example, if you automatically trigger a task to run
* a batch process job, you could apply a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks belong to that job by filtering the
* results of a ListTasks call with the startedBy
value. Up to 36 letters (uppercase and
* lowercase), numbers, hyphens, and underscores are allowed.
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the
* deployment ID of the service that starts it.
*/
public String startedBy() {
return startedBy;
}
/**
*
* The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*
*
* @return The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*/
public String group() {
return group;
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
*/
public List placementConstraints() {
return placementConstraints;
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
*/
public List placementStrategy() {
return placementStrategy;
}
/**
*
* The launch type on which to run your task. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type on which to run your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public LaunchType launchType() {
return LaunchType.fromValue(launchType);
}
/**
*
* The launch type on which to run your task. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #launchType} will
* return {@link LaunchType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #launchTypeAsString}.
*
*
* @return The launch type on which to run your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public String launchTypeAsString() {
return launchType;
}
/**
*
* The platform version the task should run. A platform version is only specified for tasks using the Fargate launch
* type. If one is not specified, the LATEST
platform version is used by default. For more information,
* see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version the task should run. A platform version is only specified for tasks using the
* Fargate launch type. If one is not specified, the LATEST
platform version is used by
* default. For more information, see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public String platformVersion() {
return platformVersion;
}
/**
*
* The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported for
* other network modes. For more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported
* for other network modes. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*/
public NetworkConfiguration networkConfiguration() {
return networkConfiguration;
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define. Tag keys can have a maximum character length of 128 characters, and
* tag values can have a maximum length of 256 characters.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define. Tag keys can have a maximum character length of 128
* characters, and tag values can have a maximum length of 256 characters.
*/
public List tags() {
return tags;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean enableECSManagedTags() {
return enableECSManagedTags;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
*
* An error will be received if you specify the SERVICE
option when running a task.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
*
* An error will be received if you specify the SERVICE
option when running a task.
*
* @see PropagateTags
*/
public PropagateTags propagateTags() {
return PropagateTags.fromValue(propagateTags);
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
*
* An error will be received if you specify the SERVICE
option when running a task.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #propagateTags}
* will return {@link PropagateTags#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #propagateTagsAsString}.
*
*
* @return Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
*
* An error will be received if you specify the SERVICE
option when running a task.
*
* @see PropagateTags
*/
public String propagateTagsAsString() {
return propagateTags;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(cluster());
hashCode = 31 * hashCode + Objects.hashCode(taskDefinition());
hashCode = 31 * hashCode + Objects.hashCode(overrides());
hashCode = 31 * hashCode + Objects.hashCode(count());
hashCode = 31 * hashCode + Objects.hashCode(startedBy());
hashCode = 31 * hashCode + Objects.hashCode(group());
hashCode = 31 * hashCode + Objects.hashCode(placementConstraints());
hashCode = 31 * hashCode + Objects.hashCode(placementStrategy());
hashCode = 31 * hashCode + Objects.hashCode(launchTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(platformVersion());
hashCode = 31 * hashCode + Objects.hashCode(networkConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(enableECSManagedTags());
hashCode = 31 * hashCode + Objects.hashCode(propagateTagsAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RunTaskRequest)) {
return false;
}
RunTaskRequest other = (RunTaskRequest) obj;
return Objects.equals(cluster(), other.cluster()) && Objects.equals(taskDefinition(), other.taskDefinition())
&& Objects.equals(overrides(), other.overrides()) && Objects.equals(count(), other.count())
&& Objects.equals(startedBy(), other.startedBy()) && Objects.equals(group(), other.group())
&& Objects.equals(placementConstraints(), other.placementConstraints())
&& Objects.equals(placementStrategy(), other.placementStrategy())
&& Objects.equals(launchTypeAsString(), other.launchTypeAsString())
&& Objects.equals(platformVersion(), other.platformVersion())
&& Objects.equals(networkConfiguration(), other.networkConfiguration()) && Objects.equals(tags(), other.tags())
&& Objects.equals(enableECSManagedTags(), other.enableECSManagedTags())
&& Objects.equals(propagateTagsAsString(), other.propagateTagsAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("RunTaskRequest").add("Cluster", cluster()).add("TaskDefinition", taskDefinition())
.add("Overrides", overrides()).add("Count", count()).add("StartedBy", startedBy()).add("Group", group())
.add("PlacementConstraints", placementConstraints()).add("PlacementStrategy", placementStrategy())
.add("LaunchType", launchTypeAsString()).add("PlatformVersion", platformVersion())
.add("NetworkConfiguration", networkConfiguration()).add("Tags", tags())
.add("EnableECSManagedTags", enableECSManagedTags()).add("PropagateTags", propagateTagsAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "cluster":
return Optional.ofNullable(clazz.cast(cluster()));
case "taskDefinition":
return Optional.ofNullable(clazz.cast(taskDefinition()));
case "overrides":
return Optional.ofNullable(clazz.cast(overrides()));
case "count":
return Optional.ofNullable(clazz.cast(count()));
case "startedBy":
return Optional.ofNullable(clazz.cast(startedBy()));
case "group":
return Optional.ofNullable(clazz.cast(group()));
case "placementConstraints":
return Optional.ofNullable(clazz.cast(placementConstraints()));
case "placementStrategy":
return Optional.ofNullable(clazz.cast(placementStrategy()));
case "launchType":
return Optional.ofNullable(clazz.cast(launchTypeAsString()));
case "platformVersion":
return Optional.ofNullable(clazz.cast(platformVersion()));
case "networkConfiguration":
return Optional.ofNullable(clazz.cast(networkConfiguration()));
case "tags":
return Optional.ofNullable(clazz.cast(tags()));
case "enableECSManagedTags":
return Optional.ofNullable(clazz.cast(enableECSManagedTags()));
case "propagateTags":
return Optional.ofNullable(clazz.cast(propagateTagsAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function