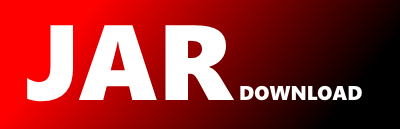
software.amazon.awssdk.services.ecs.model.LinuxParameters Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Linux-specific options that are applied to the container, such as Linux KernelCapabilities.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LinuxParameters implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CAPABILITIES_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(LinuxParameters::capabilities))
.setter(setter(Builder::capabilities)).constructor(KernelCapabilities::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("capabilities").build()).build();
private static final SdkField> DEVICES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(LinuxParameters::devices))
.setter(setter(Builder::devices))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("devices").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Device::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INIT_PROCESS_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(LinuxParameters::initProcessEnabled)).setter(setter(Builder::initProcessEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("initProcessEnabled").build())
.build();
private static final SdkField SHARED_MEMORY_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(LinuxParameters::sharedMemorySize)).setter(setter(Builder::sharedMemorySize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sharedMemorySize").build()).build();
private static final SdkField> TMPFS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(LinuxParameters::tmpfs))
.setter(setter(Builder::tmpfs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("tmpfs").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tmpfs::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField MAX_SWAP_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(LinuxParameters::maxSwap)).setter(setter(Builder::maxSwap))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maxSwap").build()).build();
private static final SdkField SWAPPINESS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(LinuxParameters::swappiness)).setter(setter(Builder::swappiness))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("swappiness").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CAPABILITIES_FIELD,
DEVICES_FIELD, INIT_PROCESS_ENABLED_FIELD, SHARED_MEMORY_SIZE_FIELD, TMPFS_FIELD, MAX_SWAP_FIELD, SWAPPINESS_FIELD));
private static final long serialVersionUID = 1L;
private final KernelCapabilities capabilities;
private final List devices;
private final Boolean initProcessEnabled;
private final Integer sharedMemorySize;
private final List tmpfs;
private final Integer maxSwap;
private final Integer swappiness;
private LinuxParameters(BuilderImpl builder) {
this.capabilities = builder.capabilities;
this.devices = builder.devices;
this.initProcessEnabled = builder.initProcessEnabled;
this.sharedMemorySize = builder.sharedMemorySize;
this.tmpfs = builder.tmpfs;
this.maxSwap = builder.maxSwap;
this.swappiness = builder.swappiness;
}
/**
*
* The Linux capabilities for the container that are added to or dropped from the default configuration provided by
* Docker.
*
*
*
* If you are using tasks that use the Fargate launch type, capabilities
is supported but the
* add
parameter is not supported.
*
*
*
* @return The Linux capabilities for the container that are added to or dropped from the default configuration
* provided by Docker.
*
* If you are using tasks that use the Fargate launch type, capabilities
is supported but the
* add
parameter is not supported.
*
*/
public KernelCapabilities capabilities() {
return capabilities;
}
/**
*
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section of the
* Docker Remote API and the --device
option to
* docker run.
*
*
*
* If you are using tasks that use the Fargate launch type, the devices
parameter is not supported.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section
* of the Docker Remote API and the
* --device
option to docker
* run.
*
* If you are using tasks that use the Fargate launch type, the devices
parameter is not
* supported.
*
*/
public List devices() {
return devices;
}
/**
*
* Run an init
process inside the container that forwards signals and reaps processes. This parameter
* maps to the --init
option to docker run.
* This parameter requires version 1.25 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
* @return Run an init
process inside the container that forwards signals and reaps processes. This
* parameter maps to the --init
option to docker run. This parameter requires version 1.25
* of the Docker Remote API or greater on your container instance. To check the Docker Remote API version on
* your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*/
public Boolean initProcessEnabled() {
return initProcessEnabled;
}
/**
*
* The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker run.
*
*
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is not
* supported.
*
*
*
* @return The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker
* run.
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is
* not supported.
*
*/
public Integer sharedMemorySize() {
return sharedMemorySize;
}
/**
*
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
*
*
* If you are using tasks that use the Fargate launch type, the tmpfs
parameter is not supported.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker
* run.
*
* If you are using tasks that use the Fargate launch type, the tmpfs
parameter is not
* supported.
*
*/
public List tmpfs() {
return tmpfs;
}
/**
*
* The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker run where
* the value would be the sum of the container memory plus the maxSwap
value.
*
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted values
* are 0
or any positive integer. If the maxSwap
parameter is omitted, the container will
* use the swap configuration for the container instance it is running on. A maxSwap
value must be set
* for the swappiness
parameter to be used.
*
*
*
* If you are using tasks that use the Fargate launch type, the maxSwap
parameter is not supported.
*
*
*
* @return The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker
* run where the value would be the sum of the container memory plus the maxSwap
value.
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted
* values are 0
or any positive integer. If the maxSwap
parameter is omitted, the
* container will use the swap configuration for the container instance it is running on. A
* maxSwap
value must be set for the swappiness
parameter to be used.
*
*
*
* If you are using tasks that use the Fargate launch type, the maxSwap
parameter is not
* supported.
*
*/
public Integer maxSwap() {
return maxSwap;
}
/**
*
* This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
value of
* 100
will cause pages to be swapped very aggressively. Accepted values are whole numbers between
* 0
and 100
. If the swappiness
parameter is not specified, a default value
* of 60
is used. If a value is not specified for maxSwap
then this parameter is ignored.
* This parameter maps to the --memory-swappiness
option to docker run.
*
*
*
* If you are using tasks that use the Fargate launch type, the swappiness
parameter is not supported.
*
*
*
* @return This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
* value of 100
will cause pages to be swapped very aggressively. Accepted values are whole
* numbers between 0
and 100
. If the swappiness
parameter is not
* specified, a default value of 60
is used. If a value is not specified for
* maxSwap
then this parameter is ignored. This parameter maps to the
* --memory-swappiness
option to docker
* run.
*
* If you are using tasks that use the Fargate launch type, the swappiness
parameter is not
* supported.
*
*/
public Integer swappiness() {
return swappiness;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(capabilities());
hashCode = 31 * hashCode + Objects.hashCode(devices());
hashCode = 31 * hashCode + Objects.hashCode(initProcessEnabled());
hashCode = 31 * hashCode + Objects.hashCode(sharedMemorySize());
hashCode = 31 * hashCode + Objects.hashCode(tmpfs());
hashCode = 31 * hashCode + Objects.hashCode(maxSwap());
hashCode = 31 * hashCode + Objects.hashCode(swappiness());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LinuxParameters)) {
return false;
}
LinuxParameters other = (LinuxParameters) obj;
return Objects.equals(capabilities(), other.capabilities()) && Objects.equals(devices(), other.devices())
&& Objects.equals(initProcessEnabled(), other.initProcessEnabled())
&& Objects.equals(sharedMemorySize(), other.sharedMemorySize()) && Objects.equals(tmpfs(), other.tmpfs())
&& Objects.equals(maxSwap(), other.maxSwap()) && Objects.equals(swappiness(), other.swappiness());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("LinuxParameters").add("Capabilities", capabilities()).add("Devices", devices())
.add("InitProcessEnabled", initProcessEnabled()).add("SharedMemorySize", sharedMemorySize())
.add("Tmpfs", tmpfs()).add("MaxSwap", maxSwap()).add("Swappiness", swappiness()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "capabilities":
return Optional.ofNullable(clazz.cast(capabilities()));
case "devices":
return Optional.ofNullable(clazz.cast(devices()));
case "initProcessEnabled":
return Optional.ofNullable(clazz.cast(initProcessEnabled()));
case "sharedMemorySize":
return Optional.ofNullable(clazz.cast(sharedMemorySize()));
case "tmpfs":
return Optional.ofNullable(clazz.cast(tmpfs()));
case "maxSwap":
return Optional.ofNullable(clazz.cast(maxSwap()));
case "swappiness":
return Optional.ofNullable(clazz.cast(swappiness()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function