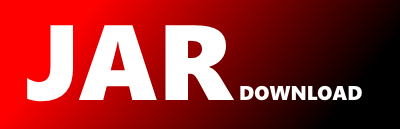
software.amazon.awssdk.services.ecs.model.ResourceRequirement Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The type and amount of a resource to assign to a container. The supported resource types are GPUs and Elastic
* Inference accelerators. For more information, see Working with GPUs on Amazon ECS
* or Working with Amazon Elastic
* Inference on Amazon ECS in the Amazon Elastic Container Service Developer Guide
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ResourceRequirement implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ResourceRequirement::value)).setter(setter(Builder::value))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("value").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(ResourceRequirement::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VALUE_FIELD, TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String value;
private final String type;
private ResourceRequirement(BuilderImpl builder) {
this.value = builder.value;
this.type = builder.type;
}
/**
*
* The value for the specified resource type.
*
*
* If the GPU
type is used, the value is the number of physical GPUs
the Amazon ECS
* container agent will reserve for the container. The number of GPUs reserved for all containers in a task should
* not exceed the number of available GPUs on the container instance the task is launched on.
*
*
* If the InferenceAccelerator
type is used, the value
should match the
* deviceName
for an InferenceAccelerator specified in a task definition.
*
*
* @return The value for the specified resource type.
*
* If the GPU
type is used, the value is the number of physical GPUs
the Amazon
* ECS container agent will reserve for the container. The number of GPUs reserved for all containers in a
* task should not exceed the number of available GPUs on the container instance the task is launched on.
*
*
* If the InferenceAccelerator
type is used, the value
should match the
* deviceName
for an InferenceAccelerator specified in a task definition.
*/
public String value() {
return value;
}
/**
*
* The type of resource to assign to a container. The supported values are GPU
or
* InferenceAccelerator
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of resource to assign to a container. The supported values are GPU
or
* InferenceAccelerator
.
* @see ResourceType
*/
public ResourceType type() {
return ResourceType.fromValue(type);
}
/**
*
* The type of resource to assign to a container. The supported values are GPU
or
* InferenceAccelerator
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type of resource to assign to a container. The supported values are GPU
or
* InferenceAccelerator
.
* @see ResourceType
*/
public String typeAsString() {
return type;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(value());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ResourceRequirement)) {
return false;
}
ResourceRequirement other = (ResourceRequirement) obj;
return Objects.equals(value(), other.value()) && Objects.equals(typeAsString(), other.typeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("ResourceRequirement").add("Value", value()).add("Type", typeAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "value":
return Optional.ofNullable(clazz.cast(value()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function