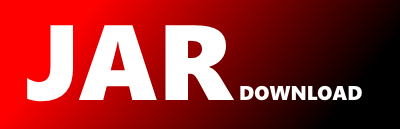
software.amazon.awssdk.services.ecs.model.TaskDefinition Maven / Gradle / Ivy
Show all versions of ecs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ecs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The details of a task definition which describes the container and volume definitions of an Amazon Elastic Container
* Service task. You can specify which Docker images to use, the required resources, and other configurations related to
* launching the task definition through an Amazon ECS service or task.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TaskDefinition implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TASK_DEFINITION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::taskDefinitionArn)).setter(setter(Builder::taskDefinitionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskDefinitionArn").build()).build();
private static final SdkField> CONTAINER_DEFINITIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(TaskDefinition::containerDefinitions))
.setter(setter(Builder::containerDefinitions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("containerDefinitions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ContainerDefinition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FAMILY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::family)).setter(setter(Builder::family))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("family").build()).build();
private static final SdkField TASK_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::taskRoleArn)).setter(setter(Builder::taskRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskRoleArn").build()).build();
private static final SdkField EXECUTION_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::executionRoleArn)).setter(setter(Builder::executionRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("executionRoleArn").build()).build();
private static final SdkField NETWORK_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::networkModeAsString)).setter(setter(Builder::networkMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("networkMode").build()).build();
private static final SdkField REVISION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.getter(getter(TaskDefinition::revision)).setter(setter(Builder::revision))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("revision").build()).build();
private static final SdkField> VOLUMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(TaskDefinition::volumes))
.setter(setter(Builder::volumes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("volumes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Volume::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField> REQUIRES_ATTRIBUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(TaskDefinition::requiresAttributes))
.setter(setter(Builder::requiresAttributes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("requiresAttributes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Attribute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PLACEMENT_CONSTRAINTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(TaskDefinition::placementConstraints))
.setter(setter(Builder::placementConstraints))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("placementConstraints").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TaskDefinitionPlacementConstraint::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> COMPATIBILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(TaskDefinition::compatibilitiesAsStrings))
.setter(setter(Builder::compatibilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("compatibilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> REQUIRES_COMPATIBILITIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(TaskDefinition::requiresCompatibilitiesAsStrings))
.setter(setter(Builder::requiresCompatibilitiesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("requiresCompatibilities").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CPU_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::cpu)).setter(setter(Builder::cpu))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("cpu").build()).build();
private static final SdkField MEMORY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::memory)).setter(setter(Builder::memory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("memory").build()).build();
private static final SdkField PID_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::pidModeAsString)).setter(setter(Builder::pidMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pidMode").build()).build();
private static final SdkField IPC_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(TaskDefinition::ipcModeAsString)).setter(setter(Builder::ipcMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ipcMode").build()).build();
private static final SdkField PROXY_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(TaskDefinition::proxyConfiguration))
.setter(setter(Builder::proxyConfiguration)).constructor(ProxyConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("proxyConfiguration").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TASK_DEFINITION_ARN_FIELD,
CONTAINER_DEFINITIONS_FIELD, FAMILY_FIELD, TASK_ROLE_ARN_FIELD, EXECUTION_ROLE_ARN_FIELD, NETWORK_MODE_FIELD,
REVISION_FIELD, VOLUMES_FIELD, STATUS_FIELD, REQUIRES_ATTRIBUTES_FIELD, PLACEMENT_CONSTRAINTS_FIELD,
COMPATIBILITIES_FIELD, REQUIRES_COMPATIBILITIES_FIELD, CPU_FIELD, MEMORY_FIELD, PID_MODE_FIELD, IPC_MODE_FIELD,
PROXY_CONFIGURATION_FIELD));
private static final long serialVersionUID = 1L;
private final String taskDefinitionArn;
private final List containerDefinitions;
private final String family;
private final String taskRoleArn;
private final String executionRoleArn;
private final String networkMode;
private final Integer revision;
private final List volumes;
private final String status;
private final List requiresAttributes;
private final List placementConstraints;
private final List compatibilities;
private final List requiresCompatibilities;
private final String cpu;
private final String memory;
private final String pidMode;
private final String ipcMode;
private final ProxyConfiguration proxyConfiguration;
private TaskDefinition(BuilderImpl builder) {
this.taskDefinitionArn = builder.taskDefinitionArn;
this.containerDefinitions = builder.containerDefinitions;
this.family = builder.family;
this.taskRoleArn = builder.taskRoleArn;
this.executionRoleArn = builder.executionRoleArn;
this.networkMode = builder.networkMode;
this.revision = builder.revision;
this.volumes = builder.volumes;
this.status = builder.status;
this.requiresAttributes = builder.requiresAttributes;
this.placementConstraints = builder.placementConstraints;
this.compatibilities = builder.compatibilities;
this.requiresCompatibilities = builder.requiresCompatibilities;
this.cpu = builder.cpu;
this.memory = builder.memory;
this.pidMode = builder.pidMode;
this.ipcMode = builder.ipcMode;
this.proxyConfiguration = builder.proxyConfiguration;
}
/**
*
* The full Amazon Resource Name (ARN) of the task definition.
*
*
* @return The full Amazon Resource Name (ARN) of the task definition.
*/
public String taskDefinitionArn() {
return taskDefinitionArn;
}
/**
*
* A list of container definitions in JSON format that describe the different containers that make up your task. For
* more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of container definitions in JSON format that describe the different containers that make up your
* task. For more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*/
public List containerDefinitions() {
return containerDefinitions;
}
/**
*
* The name of a family that this task definition is registered to. A family groups multiple versions of a task
* definition. Amazon ECS gives the first task definition that you registered to a family a revision number of 1.
* Amazon ECS gives sequential revision numbers to each task definition that you add.
*
*
* @return The name of a family that this task definition is registered to. A family groups multiple versions of a
* task definition. Amazon ECS gives the first task definition that you registered to a family a revision
* number of 1. Amazon ECS gives sequential revision numbers to each task definition that you add.
*/
public String family() {
return family;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role that
* grants containers in the task permission to call AWS APIs on your behalf. For more information, see Amazon ECS Task Role in
* the Amazon Elastic Container Service Developer Guide.
*
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you launch the
* Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code in order to take
* advantage of the feature. For more information, see Windows IAM Roles
* for Tasks in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the AWS Identity and Access Management (IAM) role
* that grants containers in the task permission to call AWS APIs on your behalf. For more information, see
* Amazon ECS Task
* Role in the Amazon Elastic Container Service Developer Guide.
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you
* launch the Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code in
* order to take advantage of the feature. For more information, see Windows
* IAM Roles for Tasks in the Amazon Elastic Container Service Developer Guide.
*/
public String taskRoleArn() {
return taskRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role that containers in this task can assume. All containers
* in this task are granted the permissions that are specified in this role.
*
*
* @return The Amazon Resource Name (ARN) of the task execution role that containers in this task can assume. All
* containers in this task are granted the permissions that are specified in this role.
*/
public String executionRoleArn() {
return executionRoleArn;
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. The default Docker network mode is
* bridge
. If you are using the Fargate launch type, the awsvpc
network mode is required.
* If you are using the EC2 launch type, any network mode can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks containers do
* not have external connectivity. The host
and awsvpc
network modes offer the highest
* networking performance for containers because they use the EC2 network stack instead of the virtualized network
* stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
*
* Currently, only Amazon ECS-optimized AMIs, other Amazon Linux variants with the ecs-init
package, or
* AWS Fargate infrastructure support the awsvpc
network mode.
*
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* Docker for Windows uses different network modes than Docker for Linux. When you register a task definition with
* Windows containers, you must not specify a network mode. If you use the console to register a task definition
* with Windows containers, you must choose the <default>
network mode object.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkMode} will
* return {@link NetworkMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkModeAsString}.
*
*
* @return The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. The default Docker network mode is
* bridge
. If you are using the Fargate launch type, the awsvpc
network mode is
* required. If you are using the EC2 launch type, any network mode can be used. If the network mode is set
* to none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of
* dynamic host port mappings.
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
*
* Currently, only Amazon ECS-optimized AMIs, other Amazon Linux variants with the ecs-init
* package, or AWS Fargate infrastructure support the awsvpc
network mode.
*
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* Docker for Windows uses different network modes than Docker for Linux. When you register a task
* definition with Windows containers, you must not specify a network mode. If you use the console to
* register a task definition with Windows containers, you must choose the <default>
* network mode object.
*
*
* For more information, see Network settings in the
* Docker run reference.
* @see NetworkMode
*/
public NetworkMode networkMode() {
return NetworkMode.fromValue(networkMode);
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. The default Docker network mode is
* bridge
. If you are using the Fargate launch type, the awsvpc
network mode is required.
* If you are using the EC2 launch type, any network mode can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks containers do
* not have external connectivity. The host
and awsvpc
network modes offer the highest
* networking performance for containers because they use the EC2 network stack instead of the virtualized network
* stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
*
* Currently, only Amazon ECS-optimized AMIs, other Amazon Linux variants with the ecs-init
package, or
* AWS Fargate infrastructure support the awsvpc
network mode.
*
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* Docker for Windows uses different network modes than Docker for Linux. When you register a task definition with
* Windows containers, you must not specify a network mode. If you use the console to register a task definition
* with Windows containers, you must choose the <default>
network mode object.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #networkMode} will
* return {@link NetworkMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #networkModeAsString}.
*
*
* @return The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. The default Docker network mode is
* bridge
. If you are using the Fargate launch type, the awsvpc
network mode is
* required. If you are using the EC2 launch type, any network mode can be used. If the network mode is set
* to none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of
* dynamic host port mappings.
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
*
* Currently, only Amazon ECS-optimized AMIs, other Amazon Linux variants with the ecs-init
* package, or AWS Fargate infrastructure support the awsvpc
network mode.
*
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* Docker for Windows uses different network modes than Docker for Linux. When you register a task
* definition with Windows containers, you must not specify a network mode. If you use the console to
* register a task definition with Windows containers, you must choose the <default>
* network mode object.
*
*
* For more information, see Network settings in the
* Docker run reference.
* @see NetworkMode
*/
public String networkModeAsString() {
return networkMode;
}
/**
*
* The revision of the task in a particular family. The revision is a version number of a task definition in a
* family. When you register a task definition for the first time, the revision is 1
. Each time that
* you register a new revision of a task definition in the same family, the revision value always increases by one,
* even if you have deregistered previous revisions in this family.
*
*
* @return The revision of the task in a particular family. The revision is a version number of a task definition in
* a family. When you register a task definition for the first time, the revision is 1
. Each
* time that you register a new revision of a task definition in the same family, the revision value always
* increases by one, even if you have deregistered previous revisions in this family.
*/
public Integer revision() {
return revision;
}
/**
*
* The list of volume definitions for the task.
*
*
* If your tasks are using the Fargate launch type, the host
and sourcePath
parameters are
* not supported.
*
*
* For more information about volume definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The list of volume definitions for the task.
*
* If your tasks are using the Fargate launch type, the host
and sourcePath
* parameters are not supported.
*
*
* For more information about volume definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*/
public List volumes() {
return volumes;
}
/**
*
* The status of the task definition.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TaskDefinitionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the task definition.
* @see TaskDefinitionStatus
*/
public TaskDefinitionStatus status() {
return TaskDefinitionStatus.fromValue(status);
}
/**
*
* The status of the task definition.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TaskDefinitionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the task definition.
* @see TaskDefinitionStatus
*/
public String statusAsString() {
return status;
}
/**
*
* The container instance attributes required by your task. This field is not valid if you are using the Fargate
* launch type for your task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The container instance attributes required by your task. This field is not valid if you are using the
* Fargate launch type for your task.
*/
public List requiresAttributes() {
return requiresAttributes;
}
/**
*
* An array of placement constraint objects to use for tasks. This field is not valid if you are using the Fargate
* launch type for your task.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of placement constraint objects to use for tasks. This field is not valid if you are using the
* Fargate launch type for your task.
*/
public List placementConstraints() {
return placementConstraints;
}
/**
*
* The launch type to use with your task. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*/
public List compatibilities() {
return CompatibilityListCopier.copyStringToEnum(compatibilities);
}
/**
*
* The launch type to use with your task. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*/
public List compatibilitiesAsStrings() {
return compatibilities;
}
/**
*
* The launch type the task requires. If no value is specified, it will default to EC2
. Valid values
* include EC2
and FARGATE
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The launch type the task requires. If no value is specified, it will default to EC2
. Valid
* values include EC2
and FARGATE
.
*/
public List requiresCompatibilities() {
return CompatibilityListCopier.copyStringToEnum(requiresCompatibilities);
}
/**
*
* The launch type the task requires. If no value is specified, it will default to EC2
. Valid values
* include EC2
and FARGATE
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The launch type the task requires. If no value is specified, it will default to EC2
. Valid
* values include EC2
and FARGATE
.
*/
public List requiresCompatibilitiesAsStrings() {
return requiresCompatibilities;
}
/**
*
* The number of cpu
units used by the task. If you are using the EC2 launch type, this field is
* optional and any value can be used. If you are using the Fargate launch type, this field is required and you must
* use one of the following values, which determines your range of valid values for the memory
* parameter:
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6
* GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024
* (1 GB)
*
*
*
*
* @return The number of cpu
units used by the task. If you are using the EC2 launch type, this field
* is optional and any value can be used. If you are using the Fargate launch type, this field is required
* and you must use one of the following values, which determines your range of valid values for the
* memory
parameter:
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB),
* 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: Between 4096 (4 GB) and 16384 (16 GB) in increments
* of 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: Between 8192 (8 GB) and 30720 (30 GB) in increments
* of 1024 (1 GB)
*
*
*/
public String cpu() {
return cpu;
}
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* If using the EC2 launch type, this field is optional and any value can be used. If a task-level memory value is
* specified then the container-level memory value is optional.
*
*
* If using the Fargate launch type, this field is required and you must use one of the following values, which
* determines your range of valid values for the cpu
parameter:
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values: 2048 (2
* vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values: 4096 (4
* vCPU)
*
*
*
*
* @return The amount (in MiB) of memory used by the task.
*
* If using the EC2 launch type, this field is optional and any value can be used. If a task-level memory
* value is specified then the container-level memory value is optional.
*
*
* If using the Fargate launch type, this field is required and you must use one of the following values,
* which determines your range of valid values for the cpu
parameter:
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 2048 (2 vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 4096 (4 vCPU)
*
*
*/
public String memory() {
return memory;
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. If host
is specified, then all containers within the tasks that specified the
* host
PID mode on the same container instance share the same process namespace with the host Amazon
* EC2 instance. If task
is specified, all containers within the specified task share the same process
* namespace. If no value is specified, the default is a private namespace. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, be aware that there is a heightened risk of undesired process
* namespace expose. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pidMode} will
* return {@link PidMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pidModeAsString}.
*
*
* @return The process namespace to use for the containers in the task. The valid values are host
or
* task
. If host
is specified, then all containers within the tasks that specified
* the host
PID mode on the same container instance share the same process namespace with the
* host Amazon EC2 instance. If task
is specified, all containers within the specified task
* share the same process namespace. If no value is specified, the default is a private namespace. For more
* information, see PID
* settings in the Docker run reference.
*
* If the host
PID mode is used, be aware that there is a heightened risk of undesired process
* namespace expose. For more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
* @see PidMode
*/
public PidMode pidMode() {
return PidMode.fromValue(pidMode);
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. If host
is specified, then all containers within the tasks that specified the
* host
PID mode on the same container instance share the same process namespace with the host Amazon
* EC2 instance. If task
is specified, all containers within the specified task share the same process
* namespace. If no value is specified, the default is a private namespace. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, be aware that there is a heightened risk of undesired process
* namespace expose. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pidMode} will
* return {@link PidMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pidModeAsString}.
*
*
* @return The process namespace to use for the containers in the task. The valid values are host
or
* task
. If host
is specified, then all containers within the tasks that specified
* the host
PID mode on the same container instance share the same process namespace with the
* host Amazon EC2 instance. If task
is specified, all containers within the specified task
* share the same process namespace. If no value is specified, the default is a private namespace. For more
* information, see PID
* settings in the Docker run reference.
*
* If the host
PID mode is used, be aware that there is a heightened risk of undesired process
* namespace expose. For more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
* @see PidMode
*/
public String pidModeAsString() {
return pidMode;
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipcMode} will
* return {@link IpcMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipcModeAsString}.
*
*
* @return The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within
* the tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
* @see IpcMode
*/
public IpcMode ipcMode() {
return IpcMode.fromValue(ipcMode);
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #ipcMode} will
* return {@link IpcMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #ipcModeAsString}.
*
*
* @return The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within
* the tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
* @see IpcMode
*/
public String ipcModeAsString() {
return ipcMode;
}
/**
*
* The configuration details for the App Mesh proxy.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least version
* 1.26.0-1 of the ecs-init
package to enable a proxy configuration. If your container instances are
* launched from the Amazon ECS-optimized AMI version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The configuration details for the App Mesh proxy.
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least
* version 1.26.0-1 of the ecs-init
package to enable a proxy configuration. If your container
* instances are launched from the Amazon ECS-optimized AMI version 20190301
or later, then
* they contain the required versions of the container agent and ecs-init
. For more
* information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*/
public ProxyConfiguration proxyConfiguration() {
return proxyConfiguration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(taskDefinitionArn());
hashCode = 31 * hashCode + Objects.hashCode(containerDefinitions());
hashCode = 31 * hashCode + Objects.hashCode(family());
hashCode = 31 * hashCode + Objects.hashCode(taskRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(executionRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(networkModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(revision());
hashCode = 31 * hashCode + Objects.hashCode(volumes());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(requiresAttributes());
hashCode = 31 * hashCode + Objects.hashCode(placementConstraints());
hashCode = 31 * hashCode + Objects.hashCode(compatibilitiesAsStrings());
hashCode = 31 * hashCode + Objects.hashCode(requiresCompatibilitiesAsStrings());
hashCode = 31 * hashCode + Objects.hashCode(cpu());
hashCode = 31 * hashCode + Objects.hashCode(memory());
hashCode = 31 * hashCode + Objects.hashCode(pidModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(ipcModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(proxyConfiguration());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TaskDefinition)) {
return false;
}
TaskDefinition other = (TaskDefinition) obj;
return Objects.equals(taskDefinitionArn(), other.taskDefinitionArn())
&& Objects.equals(containerDefinitions(), other.containerDefinitions())
&& Objects.equals(family(), other.family()) && Objects.equals(taskRoleArn(), other.taskRoleArn())
&& Objects.equals(executionRoleArn(), other.executionRoleArn())
&& Objects.equals(networkModeAsString(), other.networkModeAsString())
&& Objects.equals(revision(), other.revision()) && Objects.equals(volumes(), other.volumes())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(requiresAttributes(), other.requiresAttributes())
&& Objects.equals(placementConstraints(), other.placementConstraints())
&& Objects.equals(compatibilitiesAsStrings(), other.compatibilitiesAsStrings())
&& Objects.equals(requiresCompatibilitiesAsStrings(), other.requiresCompatibilitiesAsStrings())
&& Objects.equals(cpu(), other.cpu()) && Objects.equals(memory(), other.memory())
&& Objects.equals(pidModeAsString(), other.pidModeAsString())
&& Objects.equals(ipcModeAsString(), other.ipcModeAsString())
&& Objects.equals(proxyConfiguration(), other.proxyConfiguration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("TaskDefinition").add("TaskDefinitionArn", taskDefinitionArn())
.add("ContainerDefinitions", containerDefinitions()).add("Family", family()).add("TaskRoleArn", taskRoleArn())
.add("ExecutionRoleArn", executionRoleArn()).add("NetworkMode", networkModeAsString())
.add("Revision", revision()).add("Volumes", volumes()).add("Status", statusAsString())
.add("RequiresAttributes", requiresAttributes()).add("PlacementConstraints", placementConstraints())
.add("Compatibilities", compatibilitiesAsStrings())
.add("RequiresCompatibilities", requiresCompatibilitiesAsStrings()).add("Cpu", cpu()).add("Memory", memory())
.add("PidMode", pidModeAsString()).add("IpcMode", ipcModeAsString())
.add("ProxyConfiguration", proxyConfiguration()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "taskDefinitionArn":
return Optional.ofNullable(clazz.cast(taskDefinitionArn()));
case "containerDefinitions":
return Optional.ofNullable(clazz.cast(containerDefinitions()));
case "family":
return Optional.ofNullable(clazz.cast(family()));
case "taskRoleArn":
return Optional.ofNullable(clazz.cast(taskRoleArn()));
case "executionRoleArn":
return Optional.ofNullable(clazz.cast(executionRoleArn()));
case "networkMode":
return Optional.ofNullable(clazz.cast(networkModeAsString()));
case "revision":
return Optional.ofNullable(clazz.cast(revision()));
case "volumes":
return Optional.ofNullable(clazz.cast(volumes()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "requiresAttributes":
return Optional.ofNullable(clazz.cast(requiresAttributes()));
case "placementConstraints":
return Optional.ofNullable(clazz.cast(placementConstraints()));
case "compatibilities":
return Optional.ofNullable(clazz.cast(compatibilitiesAsStrings()));
case "requiresCompatibilities":
return Optional.ofNullable(clazz.cast(requiresCompatibilitiesAsStrings()));
case "cpu":
return Optional.ofNullable(clazz.cast(cpu()));
case "memory":
return Optional.ofNullable(clazz.cast(memory()));
case "pidMode":
return Optional.ofNullable(clazz.cast(pidModeAsString()));
case "ipcMode":
return Optional.ofNullable(clazz.cast(ipcModeAsString()));
case "proxyConfiguration":
return Optional.ofNullable(clazz.cast(proxyConfiguration()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you
* launch the Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code in
* order to take advantage of the feature. For more information, see Windows
* IAM Roles for Tasks in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder taskRoleArn(String taskRoleArn);
/**
*
* The Amazon Resource Name (ARN) of the task execution role that containers in this task can assume. All
* containers in this task are granted the permissions that are specified in this role.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the task execution role that containers in this task can assume. All
* containers in this task are granted the permissions that are specified in this role.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder executionRoleArn(String executionRoleArn);
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. The default Docker network mode is
* bridge
. If you are using the Fargate launch type, the awsvpc
network mode is
* required. If you are using the EC2 launch type, any network mode can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks containers
* do not have external connectivity. The host
and awsvpc
network modes offer the
* highest networking performance for containers because they use the EC2 network stack instead of the
* virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly
* to the corresponding host port (for the host
network mode) or the attached elastic network
* interface port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port
* mappings.
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition.
* For more information, see Task Networking
* in the Amazon Elastic Container Service Developer Guide.
*
*
*
* Currently, only Amazon ECS-optimized AMIs, other Amazon Linux variants with the ecs-init
* package, or AWS Fargate infrastructure support the awsvpc
network mode.
*
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* Docker for Windows uses different network modes than Docker for Linux. When you register a task definition
* with Windows containers, you must not specify a network mode. If you use the console to register a task
* definition with Windows containers, you must choose the <default>
network mode object.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* @param networkMode
* The Docker networking mode to use for the containers in the task. The valid values are
* none
, bridge
, awsvpc
, and host
. The default Docker
* network mode is bridge
. If you are using the Fargate launch type, the awsvpc
* network mode is required. If you are using the EC2 launch type, any network mode can be used. If the
* network mode is set to none
, you cannot specify port mappings in your container
* definitions, and the tasks containers do not have external connectivity. The host
and
* awsvpc
network modes offer the highest networking performance for containers because they
* use the EC2 network stack instead of the virtualized network stack provided by the bridge
* mode.
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached
* elastic network interface port (for the awsvpc
network mode), so you cannot take
* advantage of dynamic host port mappings.
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and
* you must specify a NetworkConfiguration value when you create a service or run a task with the
* task definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
*
* Currently, only Amazon ECS-optimized AMIs, other Amazon Linux variants with the ecs-init
* package, or AWS Fargate infrastructure support the awsvpc
network mode.
*
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* Docker for Windows uses different network modes than Docker for Linux. When you register a task
* definition with Windows containers, you must not specify a network mode. If you use the console to
* register a task definition with Windows containers, you must choose the <default>
* network mode object.
*
*
* For more information, see Network settings in the
* Docker run reference.
* @see NetworkMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see NetworkMode
*/
Builder networkMode(String networkMode);
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. The default Docker network mode is
* bridge
. If you are using the Fargate launch type, the awsvpc
network mode is
* required. If you are using the EC2 launch type, any network mode can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks containers
* do not have external connectivity. The host
and awsvpc
network modes offer the
* highest networking performance for containers because they use the EC2 network stack instead of the
* virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly
* to the corresponding host port (for the host
network mode) or the attached elastic network
* interface port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port
* mappings.
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition.
* For more information, see Task Networking
* in the Amazon Elastic Container Service Developer Guide.
*
*
*
* Currently, only Amazon ECS-optimized AMIs, other Amazon Linux variants with the ecs-init
* package, or AWS Fargate infrastructure support the awsvpc
network mode.
*
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* Docker for Windows uses different network modes than Docker for Linux. When you register a task definition
* with Windows containers, you must not specify a network mode. If you use the console to register a task
* definition with Windows containers, you must choose the <default>
network mode object.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* @param networkMode
* The Docker networking mode to use for the containers in the task. The valid values are
* none
, bridge
, awsvpc
, and host
. The default Docker
* network mode is bridge
. If you are using the Fargate launch type, the awsvpc
* network mode is required. If you are using the EC2 launch type, any network mode can be used. If the
* network mode is set to none
, you cannot specify port mappings in your container
* definitions, and the tasks containers do not have external connectivity. The host
and
* awsvpc
network modes offer the highest networking performance for containers because they
* use the EC2 network stack instead of the virtualized network stack provided by the bridge
* mode.
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached
* elastic network interface port (for the awsvpc
network mode), so you cannot take
* advantage of dynamic host port mappings.
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and
* you must specify a NetworkConfiguration value when you create a service or run a task with the
* task definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
*
* Currently, only Amazon ECS-optimized AMIs, other Amazon Linux variants with the ecs-init
* package, or AWS Fargate infrastructure support the awsvpc
network mode.
*
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* Docker for Windows uses different network modes than Docker for Linux. When you register a task
* definition with Windows containers, you must not specify a network mode. If you use the console to
* register a task definition with Windows containers, you must choose the <default>
* network mode object.
*
*
* For more information, see Network settings in the
* Docker run reference.
* @see NetworkMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see NetworkMode
*/
Builder networkMode(NetworkMode networkMode);
/**
*
* The revision of the task in a particular family. The revision is a version number of a task definition in a
* family. When you register a task definition for the first time, the revision is 1
. Each time
* that you register a new revision of a task definition in the same family, the revision value always increases
* by one, even if you have deregistered previous revisions in this family.
*
*
* @param revision
* The revision of the task in a particular family. The revision is a version number of a task definition
* in a family. When you register a task definition for the first time, the revision is 1
.
* Each time that you register a new revision of a task definition in the same family, the revision value
* always increases by one, even if you have deregistered previous revisions in this family.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder revision(Integer revision);
/**
*
* The list of volume definitions for the task.
*
*
* If your tasks are using the Fargate launch type, the host
and sourcePath
parameters
* are not supported.
*
*
* For more information about volume definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param volumes
* The list of volume definitions for the task.
*
* If your tasks are using the Fargate launch type, the host
and sourcePath
* parameters are not supported.
*
*
* For more information about volume definition parameters and defaults, see Amazon ECS
* Task Definitions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder volumes(Collection volumes);
/**
*
* The list of volume definitions for the task.
*
*
* If your tasks are using the Fargate launch type, the host
and sourcePath
parameters
* are not supported.
*
*
* For more information about volume definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param volumes
* The list of volume definitions for the task.
*
* If your tasks are using the Fargate launch type, the host
and sourcePath
* parameters are not supported.
*
*
* For more information about volume definition parameters and defaults, see Amazon ECS
* Task Definitions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder volumes(Volume... volumes);
/**
*
* The list of volume definitions for the task.
*
*
* If your tasks are using the Fargate launch type, the host
and sourcePath
parameters
* are not supported.
*
*
* For more information about volume definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to
* create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its
* result is passed to {@link #volumes(List)}.
*
* @param volumes
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #volumes(List)
*/
Builder volumes(Consumer... volumes);
/**
*
* The status of the task definition.
*
*
* @param status
* The status of the task definition.
* @see TaskDefinitionStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskDefinitionStatus
*/
Builder status(String status);
/**
*
* The status of the task definition.
*
*
* @param status
* The status of the task definition.
* @see TaskDefinitionStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskDefinitionStatus
*/
Builder status(TaskDefinitionStatus status);
/**
*
* The container instance attributes required by your task. This field is not valid if you are using the Fargate
* launch type for your task.
*
*
* @param requiresAttributes
* The container instance attributes required by your task. This field is not valid if you are using the
* Fargate launch type for your task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder requiresAttributes(Collection requiresAttributes);
/**
*
* The container instance attributes required by your task. This field is not valid if you are using the Fargate
* launch type for your task.
*
*
* @param requiresAttributes
* The container instance attributes required by your task. This field is not valid if you are using the
* Fargate launch type for your task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder requiresAttributes(Attribute... requiresAttributes);
/**
*
* The container instance attributes required by your task. This field is not valid if you are using the Fargate
* launch type for your task.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to
* create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its
* result is passed to {@link #requiresAttributes(List)}.
*
* @param requiresAttributes
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #requiresAttributes(List)
*/
Builder requiresAttributes(Consumer... requiresAttributes);
/**
*
* An array of placement constraint objects to use for tasks. This field is not valid if you are using the
* Fargate launch type for your task.
*
*
* @param placementConstraints
* An array of placement constraint objects to use for tasks. This field is not valid if you are using
* the Fargate launch type for your task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder placementConstraints(Collection placementConstraints);
/**
*
* An array of placement constraint objects to use for tasks. This field is not valid if you are using the
* Fargate launch type for your task.
*
*
* @param placementConstraints
* An array of placement constraint objects to use for tasks. This field is not valid if you are using
* the Fargate launch type for your task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder placementConstraints(TaskDefinitionPlacementConstraint... placementConstraints);
/**
*
* An array of placement constraint objects to use for tasks. This field is not valid if you are using the
* Fargate launch type for your task.
*
* This is a convenience that creates an instance of the {@link List.Builder}
* avoiding the need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is
* called immediately and its result is passed to {@link
* #placementConstraints(List)}.
*
* @param placementConstraints
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #placementConstraints(List)
*/
Builder placementConstraints(Consumer... placementConstraints);
/**
*
* The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param compatibilities
* The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder compatibilitiesWithStrings(Collection compatibilities);
/**
*
* The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param compatibilities
* The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder compatibilitiesWithStrings(String... compatibilities);
/**
*
* The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param compatibilities
* The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder compatibilities(Collection compatibilities);
/**
*
* The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param compatibilities
* The launch type to use with your task. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder compatibilities(Compatibility... compatibilities);
/**
*
* The launch type the task requires. If no value is specified, it will default to EC2
. Valid
* values include EC2
and FARGATE
.
*
*
* @param requiresCompatibilities
* The launch type the task requires. If no value is specified, it will default to EC2
.
* Valid values include EC2
and FARGATE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder requiresCompatibilitiesWithStrings(Collection requiresCompatibilities);
/**
*
* The launch type the task requires. If no value is specified, it will default to EC2
. Valid
* values include EC2
and FARGATE
.
*
*
* @param requiresCompatibilities
* The launch type the task requires. If no value is specified, it will default to EC2
.
* Valid values include EC2
and FARGATE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder requiresCompatibilitiesWithStrings(String... requiresCompatibilities);
/**
*
* The launch type the task requires. If no value is specified, it will default to EC2
. Valid
* values include EC2
and FARGATE
.
*
*
* @param requiresCompatibilities
* The launch type the task requires. If no value is specified, it will default to EC2
.
* Valid values include EC2
and FARGATE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder requiresCompatibilities(Collection requiresCompatibilities);
/**
*
* The launch type the task requires. If no value is specified, it will default to EC2
. Valid
* values include EC2
and FARGATE
.
*
*
* @param requiresCompatibilities
* The launch type the task requires. If no value is specified, it will default to EC2
.
* Valid values include EC2
and FARGATE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder requiresCompatibilities(Compatibility... requiresCompatibilities);
/**
*
* The number of cpu
units used by the task. If you are using the EC2 launch type, this field is
* optional and any value can be used. If you are using the Fargate launch type, this field is required and you
* must use one of the following values, which determines your range of valid values for the memory
* parameter:
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB),
* 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: Between 4096 (4 GB) and 16384 (16 GB) in increments of
* 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: Between 8192 (8 GB) and 30720 (30 GB) in increments of
* 1024 (1 GB)
*
*
*
*
* @param cpu
* The number of cpu
units used by the task. If you are using the EC2 launch type, this
* field is optional and any value can be used. If you are using the Fargate launch type, this field is
* required and you must use one of the following values, which determines your range of valid values for
* the memory
parameter:
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4
* GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5
* GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: Between 4096 (4 GB) and 16384 (16 GB) in
* increments of 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: Between 8192 (8 GB) and 30720 (30 GB) in
* increments of 1024 (1 GB)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder cpu(String cpu);
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* If using the EC2 launch type, this field is optional and any value can be used. If a task-level memory value
* is specified then the container-level memory value is optional.
*
*
* If using the Fargate launch type, this field is required and you must use one of the following values, which
* determines your range of valid values for the cpu
parameter:
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values: 2048
* (2 vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values: 4096
* (4 vCPU)
*
*
*
*
* @param memory
* The amount (in MiB) of memory used by the task.
*
* If using the EC2 launch type, this field is optional and any value can be used. If a task-level memory
* value is specified then the container-level memory value is optional.
*
*
* If using the Fargate launch type, this field is required and you must use one of the following values,
* which determines your range of valid values for the cpu
parameter:
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
* values: 2048 (2 vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
* values: 4096 (4 vCPU)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder memory(String memory);
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. If host
is specified, then all containers within the tasks that specified the
* host
PID mode on the same container instance share the same process namespace with the host
* Amazon EC2 instance. If task
is specified, all containers within the specified task share the
* same process namespace. If no value is specified, the default is a private namespace. For more information,
* see PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, be aware that there is a heightened risk of undesired process
* namespace expose. For more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
*
*
* @param pidMode
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. If host
is specified, then all containers within the tasks that
* specified the host
PID mode on the same container instance share the same process
* namespace with the host Amazon EC2 instance. If task
is specified, all containers within
* the specified task share the same process namespace. If no value is specified, the default is a
* private namespace. For more information, see PID settings in the
* Docker run reference.
*
* If the host
PID mode is used, be aware that there is a heightened risk of undesired
* process namespace expose. For more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
* @see PidMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see PidMode
*/
Builder pidMode(String pidMode);
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. If host
is specified, then all containers within the tasks that specified the
* host
PID mode on the same container instance share the same process namespace with the host
* Amazon EC2 instance. If task
is specified, all containers within the specified task share the
* same process namespace. If no value is specified, the default is a private namespace. For more information,
* see PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, be aware that there is a heightened risk of undesired process
* namespace expose. For more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
*
*
* @param pidMode
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. If host
is specified, then all containers within the tasks that
* specified the host
PID mode on the same container instance share the same process
* namespace with the host Amazon EC2 instance. If task
is specified, all containers within
* the specified task share the same process namespace. If no value is specified, the default is a
* private namespace. For more information, see PID settings in the
* Docker run reference.
*
* If the host
PID mode is used, be aware that there is a heightened risk of undesired
* process namespace expose. For more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
* @see PidMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see PidMode
*/
Builder pidMode(PidMode pidMode);
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the
* tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within the
* containers of a task are private and not shared with other containers in a task or on the container instance.
* If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon setting on the
* container instance. For more information, see IPC settings in the Docker run
* reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the
* task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
*
*
* @param ipcMode
* The IPC resource namespace to use for the containers in the task. The valid values are
* host
, task
, or none
. If host
is specified, then
* all containers within the tasks that specified the host
IPC mode on the same container
* instance share the same IPC resources with the host Amazon EC2 instance. If task
is
* specified, all containers within the specified task share the same IPC resources. If none
* is specified, then IPC resources within the containers of a task are private and not shared with other
* containers in a task or on the container instance. If no value is specified, then the IPC resource
* namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC
* settings in the Docker run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers
* in the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
* are not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
* will apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
* @see IpcMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpcMode
*/
Builder ipcMode(String ipcMode);
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the
* tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within the
* containers of a task are private and not shared with other containers in a task or on the container instance.
* If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon setting on the
* container instance. For more information, see IPC settings in the Docker run
* reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the
* task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
*
*
* @param ipcMode
* The IPC resource namespace to use for the containers in the task. The valid values are
* host
, task
, or none
. If host
is specified, then
* all containers within the tasks that specified the host
IPC mode on the same container
* instance share the same IPC resources with the host Amazon EC2 instance. If task
is
* specified, all containers within the specified task share the same IPC resources. If none
* is specified, then IPC resources within the containers of a task are private and not shared with other
* containers in a task or on the container instance. If no value is specified, then the IPC resource
* namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC
* settings in the Docker run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers
* in the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
* are not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
* will apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks using the Fargate launch type.
*
* @see IpcMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpcMode
*/
Builder ipcMode(IpcMode ipcMode);
/**
*
* The configuration details for the App Mesh proxy.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least
* version 1.26.0-1 of the ecs-init
package to enable a proxy configuration. If your container
* instances are launched from the Amazon ECS-optimized AMI version 20190301
or later, then they
* contain the required versions of the container agent and ecs-init
. For more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* @param proxyConfiguration
* The configuration details for the App Mesh proxy.
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at
* least version 1.26.0-1 of the ecs-init
package to enable a proxy configuration. If your
* container instances are launched from the Amazon ECS-optimized AMI version 20190301
or
* later, then they contain the required versions of the container agent and ecs-init
. For
* more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder proxyConfiguration(ProxyConfiguration proxyConfiguration);
/**
*
* The configuration details for the App Mesh proxy.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least
* version 1.26.0-1 of the ecs-init
package to enable a proxy configuration. If your container
* instances are launched from the Amazon ECS-optimized AMI version 20190301
or later, then they
* contain the required versions of the container agent and ecs-init
. For more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
* This is a convenience that creates an instance of the {@link ProxyConfiguration.Builder} avoiding the need to
* create one manually via {@link ProxyConfiguration#builder()}.
*
* When the {@link Consumer} completes, {@link ProxyConfiguration.Builder#build()} is called immediately and its
* result is passed to {@link #proxyConfiguration(ProxyConfiguration)}.
*
* @param proxyConfiguration
* a consumer that will call methods on {@link ProxyConfiguration.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #proxyConfiguration(ProxyConfiguration)
*/
default Builder proxyConfiguration(Consumer proxyConfiguration) {
return proxyConfiguration(ProxyConfiguration.builder().applyMutation(proxyConfiguration).build());
}
}
static final class BuilderImpl implements Builder {
private String taskDefinitionArn;
private List containerDefinitions = DefaultSdkAutoConstructList.getInstance();
private String family;
private String taskRoleArn;
private String executionRoleArn;
private String networkMode;
private Integer revision;
private List volumes = DefaultSdkAutoConstructList.getInstance();
private String status;
private List requiresAttributes = DefaultSdkAutoConstructList.getInstance();
private List placementConstraints = DefaultSdkAutoConstructList.getInstance();
private List compatibilities = DefaultSdkAutoConstructList.getInstance();
private List requiresCompatibilities = DefaultSdkAutoConstructList.getInstance();
private String cpu;
private String memory;
private String pidMode;
private String ipcMode;
private ProxyConfiguration proxyConfiguration;
private BuilderImpl() {
}
private BuilderImpl(TaskDefinition model) {
taskDefinitionArn(model.taskDefinitionArn);
containerDefinitions(model.containerDefinitions);
family(model.family);
taskRoleArn(model.taskRoleArn);
executionRoleArn(model.executionRoleArn);
networkMode(model.networkMode);
revision(model.revision);
volumes(model.volumes);
status(model.status);
requiresAttributes(model.requiresAttributes);
placementConstraints(model.placementConstraints);
compatibilitiesWithStrings(model.compatibilities);
requiresCompatibilitiesWithStrings(model.requiresCompatibilities);
cpu(model.cpu);
memory(model.memory);
pidMode(model.pidMode);
ipcMode(model.ipcMode);
proxyConfiguration(model.proxyConfiguration);
}
public final String getTaskDefinitionArn() {
return taskDefinitionArn;
}
@Override
public final Builder taskDefinitionArn(String taskDefinitionArn) {
this.taskDefinitionArn = taskDefinitionArn;
return this;
}
public final void setTaskDefinitionArn(String taskDefinitionArn) {
this.taskDefinitionArn = taskDefinitionArn;
}
public final Collection getContainerDefinitions() {
return containerDefinitions != null ? containerDefinitions.stream().map(ContainerDefinition::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder containerDefinitions(Collection containerDefinitions) {
this.containerDefinitions = ContainerDefinitionsCopier.copy(containerDefinitions);
return this;
}
@Override
@SafeVarargs
public final Builder containerDefinitions(ContainerDefinition... containerDefinitions) {
containerDefinitions(Arrays.asList(containerDefinitions));
return this;
}
@Override
@SafeVarargs
public final Builder containerDefinitions(Consumer... containerDefinitions) {
containerDefinitions(Stream.of(containerDefinitions).map(c -> ContainerDefinition.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setContainerDefinitions(Collection containerDefinitions) {
this.containerDefinitions = ContainerDefinitionsCopier.copyFromBuilder(containerDefinitions);
}
public final String getFamily() {
return family;
}
@Override
public final Builder family(String family) {
this.family = family;
return this;
}
public final void setFamily(String family) {
this.family = family;
}
public final String getTaskRoleArn() {
return taskRoleArn;
}
@Override
public final Builder taskRoleArn(String taskRoleArn) {
this.taskRoleArn = taskRoleArn;
return this;
}
public final void setTaskRoleArn(String taskRoleArn) {
this.taskRoleArn = taskRoleArn;
}
public final String getExecutionRoleArn() {
return executionRoleArn;
}
@Override
public final Builder executionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
return this;
}
public final void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
public final String getNetworkModeAsString() {
return networkMode;
}
@Override
public final Builder networkMode(String networkMode) {
this.networkMode = networkMode;
return this;
}
@Override
public final Builder networkMode(NetworkMode networkMode) {
this.networkMode(networkMode == null ? null : networkMode.toString());
return this;
}
public final void setNetworkMode(String networkMode) {
this.networkMode = networkMode;
}
public final Integer getRevision() {
return revision;
}
@Override
public final Builder revision(Integer revision) {
this.revision = revision;
return this;
}
public final void setRevision(Integer revision) {
this.revision = revision;
}
public final Collection getVolumes() {
return volumes != null ? volumes.stream().map(Volume::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder volumes(Collection volumes) {
this.volumes = VolumeListCopier.copy(volumes);
return this;
}
@Override
@SafeVarargs
public final Builder volumes(Volume... volumes) {
volumes(Arrays.asList(volumes));
return this;
}
@Override
@SafeVarargs
public final Builder volumes(Consumer... volumes) {
volumes(Stream.of(volumes).map(c -> Volume.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setVolumes(Collection volumes) {
this.volumes = VolumeListCopier.copyFromBuilder(volumes);
}
public final String getStatusAsString() {
return status;
}
@Override
public final Builder status(String status) {
this.status = status;
return this;
}
@Override
public final Builder status(TaskDefinitionStatus status) {
this.status(status == null ? null : status.toString());
return this;
}
public final void setStatus(String status) {
this.status = status;
}
public final Collection getRequiresAttributes() {
return requiresAttributes != null ? requiresAttributes.stream().map(Attribute::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder requiresAttributes(Collection requiresAttributes) {
this.requiresAttributes = RequiresAttributesCopier.copy(requiresAttributes);
return this;
}
@Override
@SafeVarargs
public final Builder requiresAttributes(Attribute... requiresAttributes) {
requiresAttributes(Arrays.asList(requiresAttributes));
return this;
}
@Override
@SafeVarargs
public final Builder requiresAttributes(Consumer... requiresAttributes) {
requiresAttributes(Stream.of(requiresAttributes).map(c -> Attribute.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setRequiresAttributes(Collection requiresAttributes) {
this.requiresAttributes = RequiresAttributesCopier.copyFromBuilder(requiresAttributes);
}
public final Collection getPlacementConstraints() {
return placementConstraints != null ? placementConstraints.stream().map(TaskDefinitionPlacementConstraint::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder placementConstraints(Collection placementConstraints) {
this.placementConstraints = TaskDefinitionPlacementConstraintsCopier.copy(placementConstraints);
return this;
}
@Override
@SafeVarargs
public final Builder placementConstraints(TaskDefinitionPlacementConstraint... placementConstraints) {
placementConstraints(Arrays.asList(placementConstraints));
return this;
}
@Override
@SafeVarargs
public final Builder placementConstraints(Consumer... placementConstraints) {
placementConstraints(Stream.of(placementConstraints)
.map(c -> TaskDefinitionPlacementConstraint.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setPlacementConstraints(Collection placementConstraints) {
this.placementConstraints = TaskDefinitionPlacementConstraintsCopier.copyFromBuilder(placementConstraints);
}
public final Collection getCompatibilitiesAsStrings() {
return compatibilities;
}
@Override
public final Builder compatibilitiesWithStrings(Collection compatibilities) {
this.compatibilities = CompatibilityListCopier.copy(compatibilities);
return this;
}
@Override
@SafeVarargs
public final Builder compatibilitiesWithStrings(String... compatibilities) {
compatibilitiesWithStrings(Arrays.asList(compatibilities));
return this;
}
@Override
public final Builder compatibilities(Collection compatibilities) {
this.compatibilities = CompatibilityListCopier.copyEnumToString(compatibilities);
return this;
}
@Override
@SafeVarargs
public final Builder compatibilities(Compatibility... compatibilities) {
compatibilities(Arrays.asList(compatibilities));
return this;
}
public final void setCompatibilitiesWithStrings(Collection compatibilities) {
this.compatibilities = CompatibilityListCopier.copy(compatibilities);
}
public final Collection getRequiresCompatibilitiesAsStrings() {
return requiresCompatibilities;
}
@Override
public final Builder requiresCompatibilitiesWithStrings(Collection requiresCompatibilities) {
this.requiresCompatibilities = CompatibilityListCopier.copy(requiresCompatibilities);
return this;
}
@Override
@SafeVarargs
public final Builder requiresCompatibilitiesWithStrings(String... requiresCompatibilities) {
requiresCompatibilitiesWithStrings(Arrays.asList(requiresCompatibilities));
return this;
}
@Override
public final Builder requiresCompatibilities(Collection requiresCompatibilities) {
this.requiresCompatibilities = CompatibilityListCopier.copyEnumToString(requiresCompatibilities);
return this;
}
@Override
@SafeVarargs
public final Builder requiresCompatibilities(Compatibility... requiresCompatibilities) {
requiresCompatibilities(Arrays.asList(requiresCompatibilities));
return this;
}
public final void setRequiresCompatibilitiesWithStrings(Collection requiresCompatibilities) {
this.requiresCompatibilities = CompatibilityListCopier.copy(requiresCompatibilities);
}
public final String getCpu() {
return cpu;
}
@Override
public final Builder cpu(String cpu) {
this.cpu = cpu;
return this;
}
public final void setCpu(String cpu) {
this.cpu = cpu;
}
public final String getMemory() {
return memory;
}
@Override
public final Builder memory(String memory) {
this.memory = memory;
return this;
}
public final void setMemory(String memory) {
this.memory = memory;
}
public final String getPidModeAsString() {
return pidMode;
}
@Override
public final Builder pidMode(String pidMode) {
this.pidMode = pidMode;
return this;
}
@Override
public final Builder pidMode(PidMode pidMode) {
this.pidMode(pidMode == null ? null : pidMode.toString());
return this;
}
public final void setPidMode(String pidMode) {
this.pidMode = pidMode;
}
public final String getIpcModeAsString() {
return ipcMode;
}
@Override
public final Builder ipcMode(String ipcMode) {
this.ipcMode = ipcMode;
return this;
}
@Override
public final Builder ipcMode(IpcMode ipcMode) {
this.ipcMode(ipcMode == null ? null : ipcMode.toString());
return this;
}
public final void setIpcMode(String ipcMode) {
this.ipcMode = ipcMode;
}
public final ProxyConfiguration.Builder getProxyConfiguration() {
return proxyConfiguration != null ? proxyConfiguration.toBuilder() : null;
}
@Override
public final Builder proxyConfiguration(ProxyConfiguration proxyConfiguration) {
this.proxyConfiguration = proxyConfiguration;
return this;
}
public final void setProxyConfiguration(ProxyConfiguration.BuilderImpl proxyConfiguration) {
this.proxyConfiguration = proxyConfiguration != null ? proxyConfiguration.build() : null;
}
@Override
public TaskDefinition build() {
return new TaskDefinition(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}